第一题:
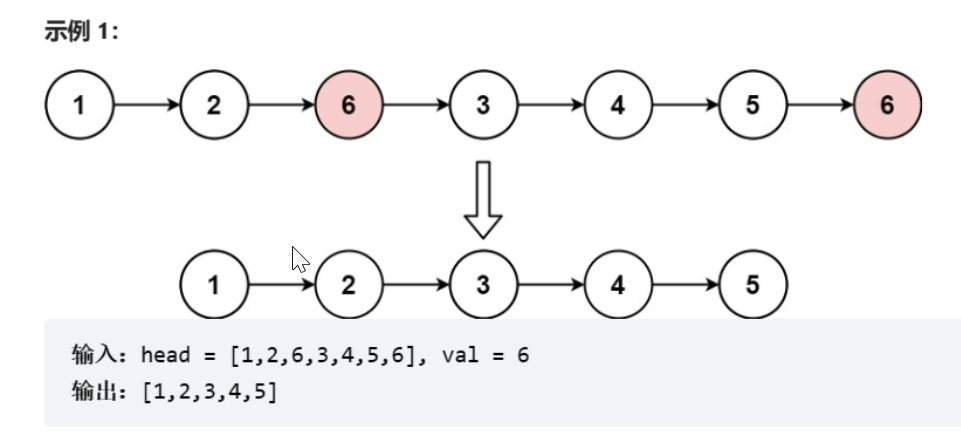
解题思路:遍历整个链表将满足条件的节点一一删除,首先定义一个prev指针和一个cur指针,prev用来保存cur前面一个节点,如果cur->val 等于val是,让prev的下一个节点指向cur的下一个节点,同时释放cur的空间,再让cur指向prev->next节点,完成一次删除。当cur等于NULL时遍历结束。
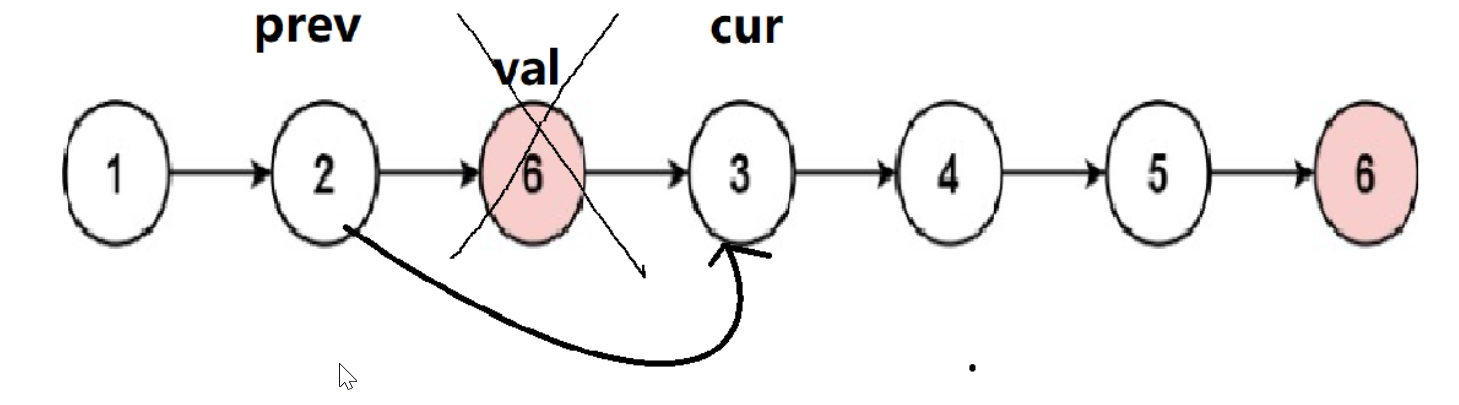
struct ListNode* removeElements(struct ListNode* head, int val){
struct ListNode * cur = head;
struct ListNode * prev = NULL;
while(cur)
{
if(cur->val != val)
{
prev = cur;
cur = cur->next;
}
else
{
if(prev == NULL)
{
head = cur->next;
free(cur);
cur = head;
}
else
{
prev->next = cur->next;
free(cur);
cur = prev->next;
}
}
}
return head;
}
第二题:
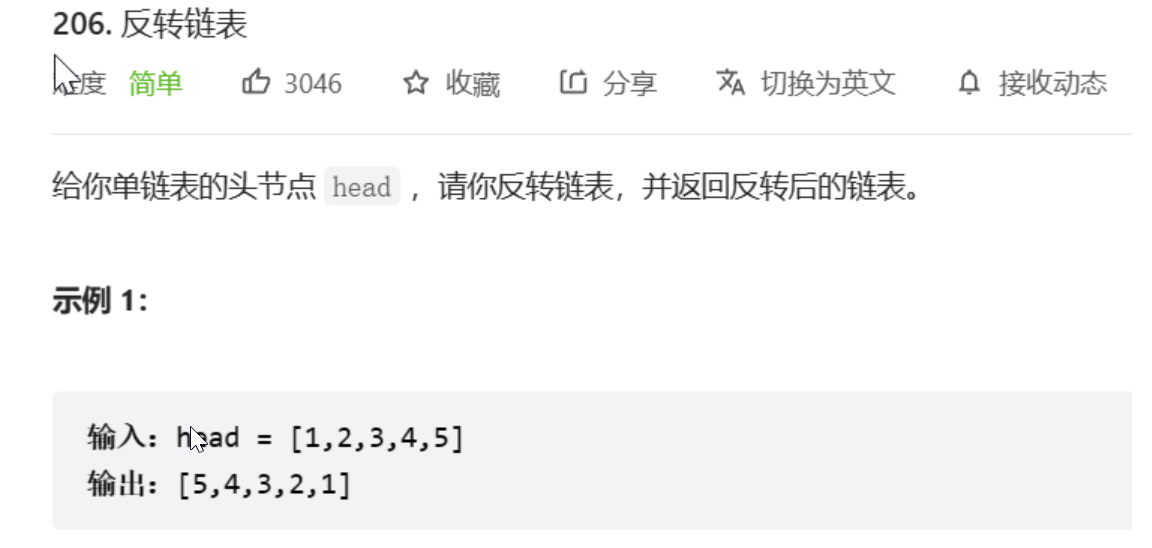
定义三个结构体指针变量,n1,n2,n3,n1初始化为空,n2指向头节点,n3指向头节点的后一个节点,第一步让n2指向n1,此时n2 与n3之间的节点断开,之后再让n1指n2, n2指向n3, n3也指向下一个节点,反转一个节点完成。一直到n2为空停下。返回n1。
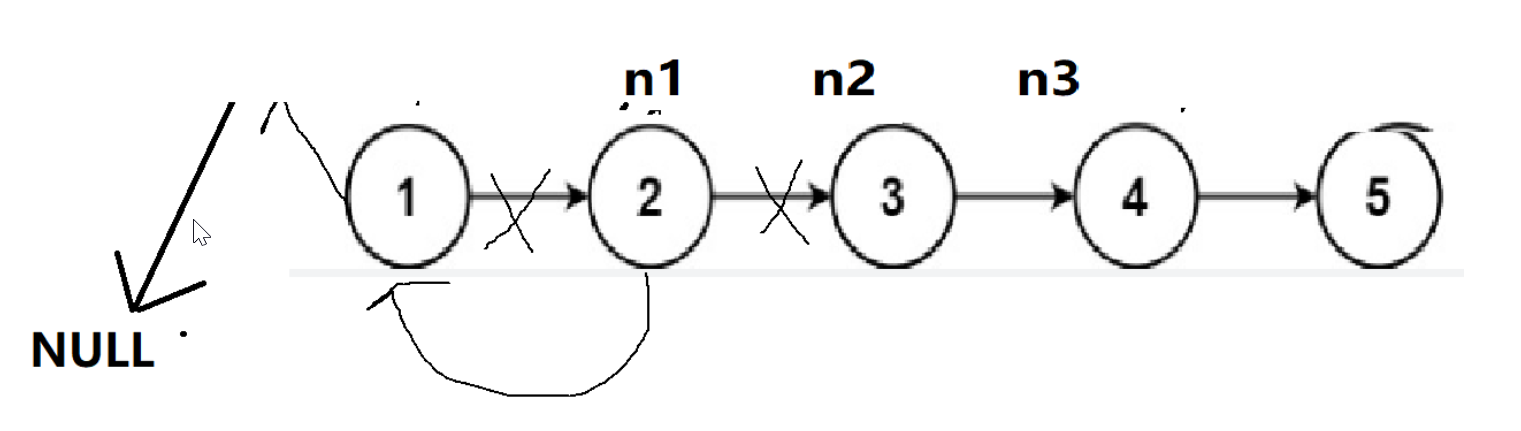
struct ListNode* reverseList(struct ListNode* head){
struct ListNode*n1,*n2,*n3;
if(head == NULL)
{
return NULL;
}
n1 = NULL;
n2 = head;
n3 = head->next;
while(n2)
{
n2->next = n1;
n1 = n2;
n2 = n3;
if(n3)
{
n3 = n3->next;
}
}
return n1;
}
第三题:
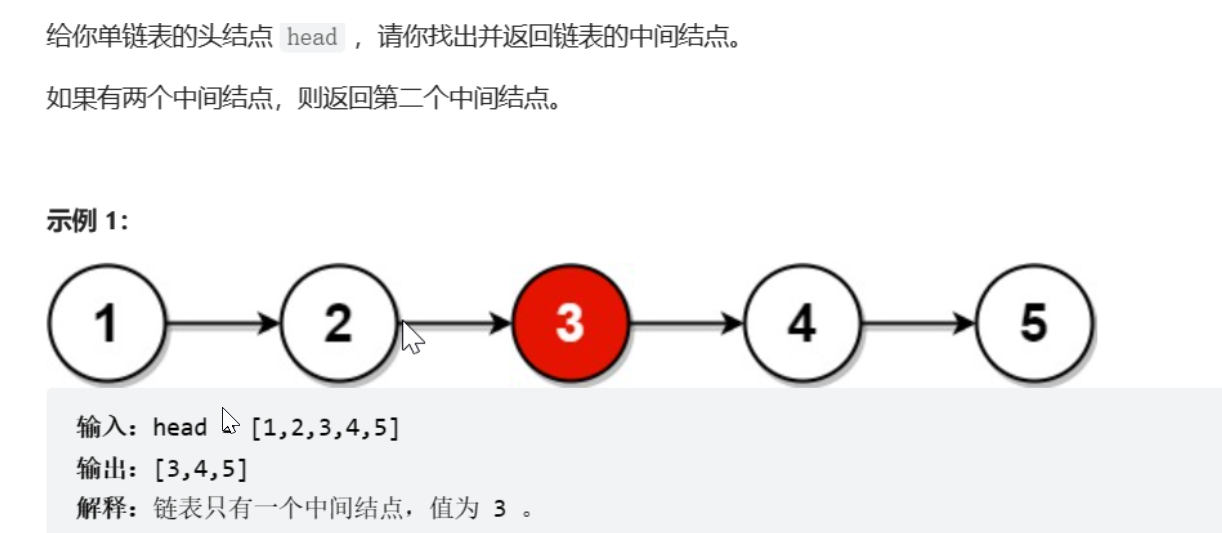
快慢指针问题,定义一个快指针,一次走两步,定义一个慢指针,一次走一步,当快指针刚好走到结尾,慢指针刚好在中间。
struct ListNode* middleNode(struct ListNode* head){
struct ListNode* fast = head;
struct ListNode* slow = head;
while(fast && fast->next)
{
slow = slow->next;
fast = fast->next->next;
}
return slow;
}
第四题:
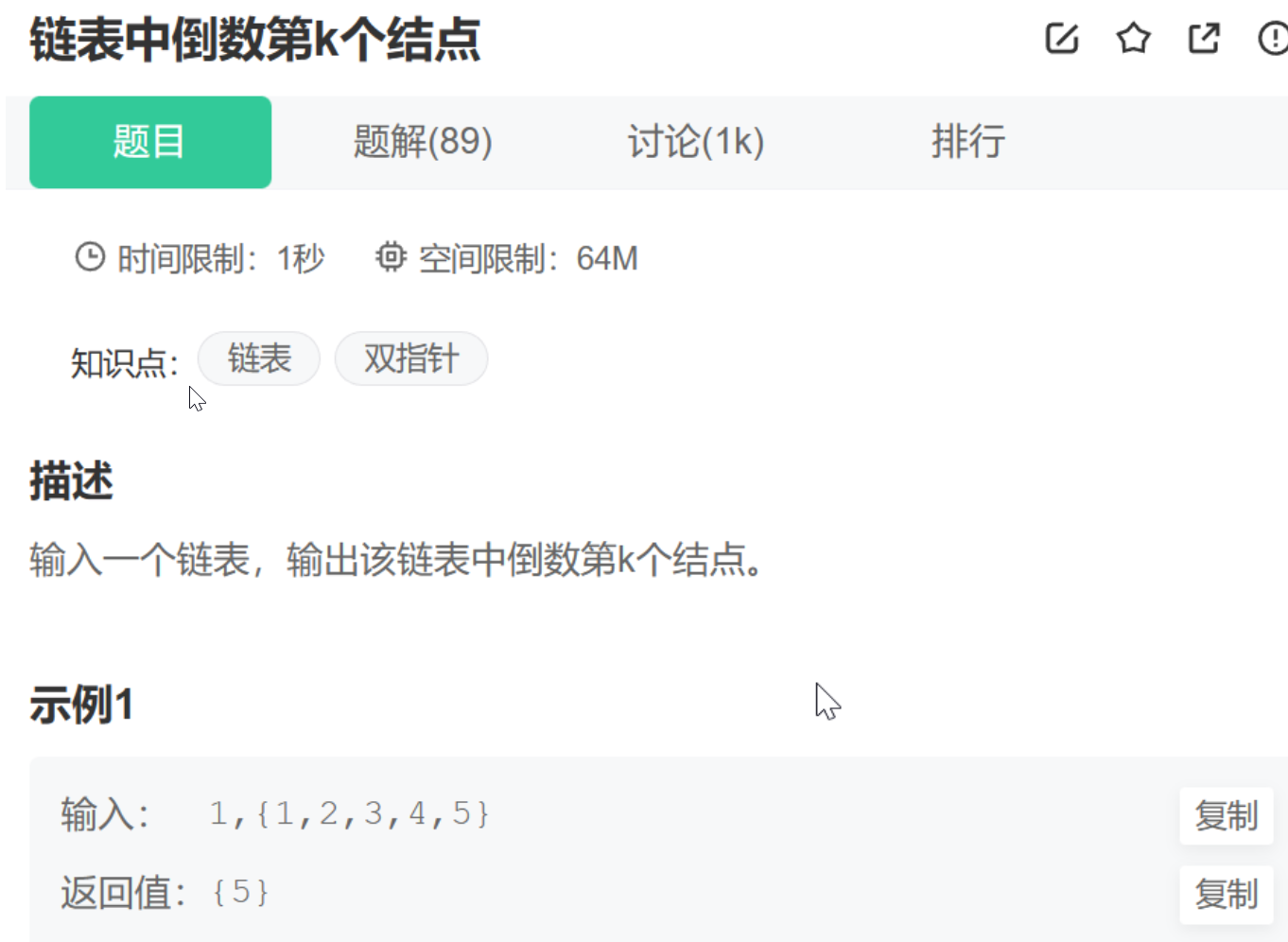
同样可以用快慢指针来解决,快指针先走k步,之后两个指针同时走,当快指针走到终点时,慢指针刚好到所需要的位置。
struct ListNode* FindKthToTail(struct ListNode* pListHead, int k ) {
// write code here
struct ListNode * fast = pListHead;
struct ListNode * slow = pListHead;
while(k--)
{
if(fast == NULL)
{
return NULL;
}
fast = fast->next;
}
while(fast)
{
slow = slow->next;
fast = fast->next;
}
return slow;
}
第五题:
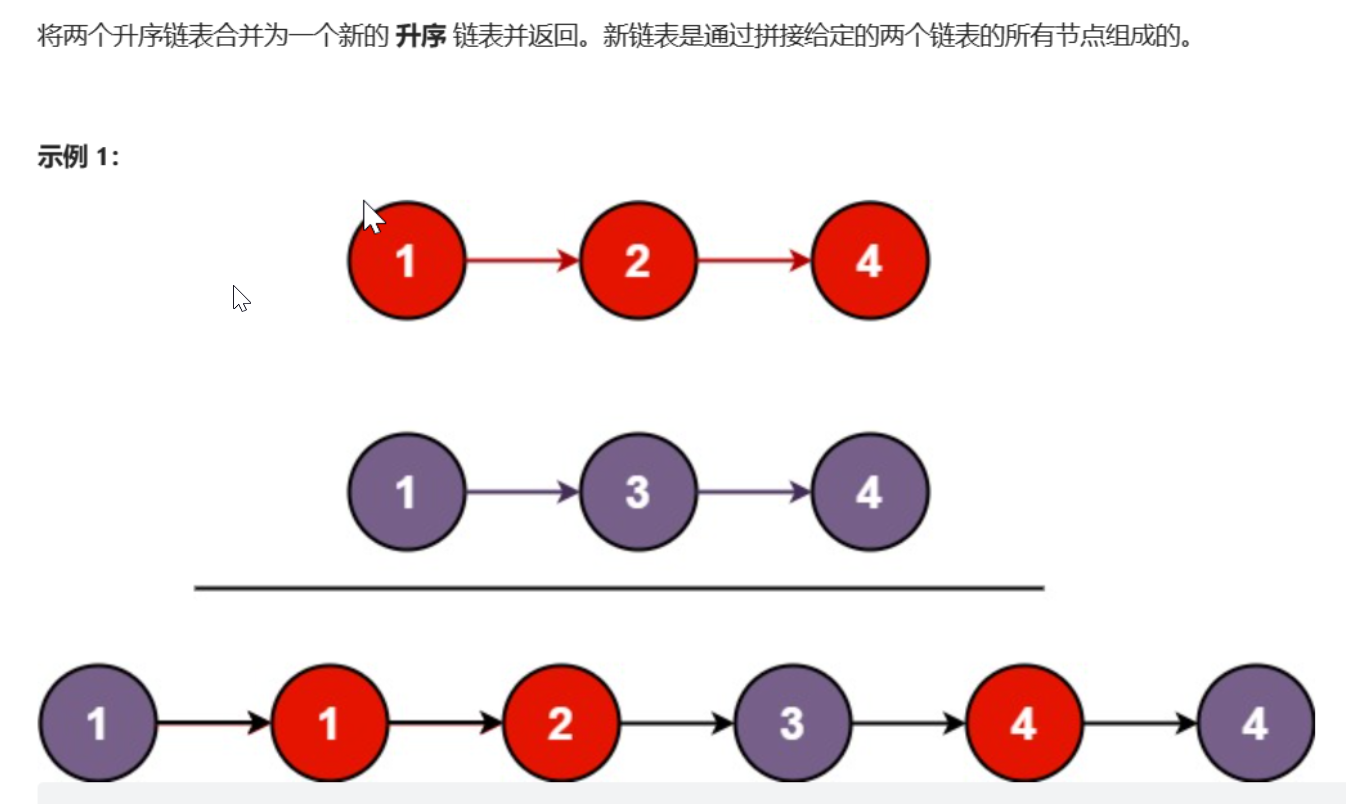
定义一个新链表,比较两个链表的数据,小的尾插到新链表中。
struct ListNode* mergeTwoLists(struct ListNode* list1, struct ListNode* list2){
if(list1 == NULL)
{
return list2;
}
if(list2 == NULL)
{
return list1;
}
struct ListNode* cur1 = list1,*cur2 = list2;
struct ListNode* head =NULL,*tail = NULL;
while(cur1 && cur2)
{
if(cur1->val < cur2->val)
{
if(head == NULL)
{
head = tail = cur1;
}
else
{
tail->next = cur1;
tail = tail->next;
}
cur1 = cur1->next;
}
else
{
if(head == NULL)
{
head =tail = cur2;
}
else
{
tail->next = cur2;
tail = tail->next;
}
cur2 = cur2->next;
}
}
if(cur1)
{
tail->next = cur1;
}
if(cur2)
{
tail->next = cur2;
}
return head;
}
第六题:
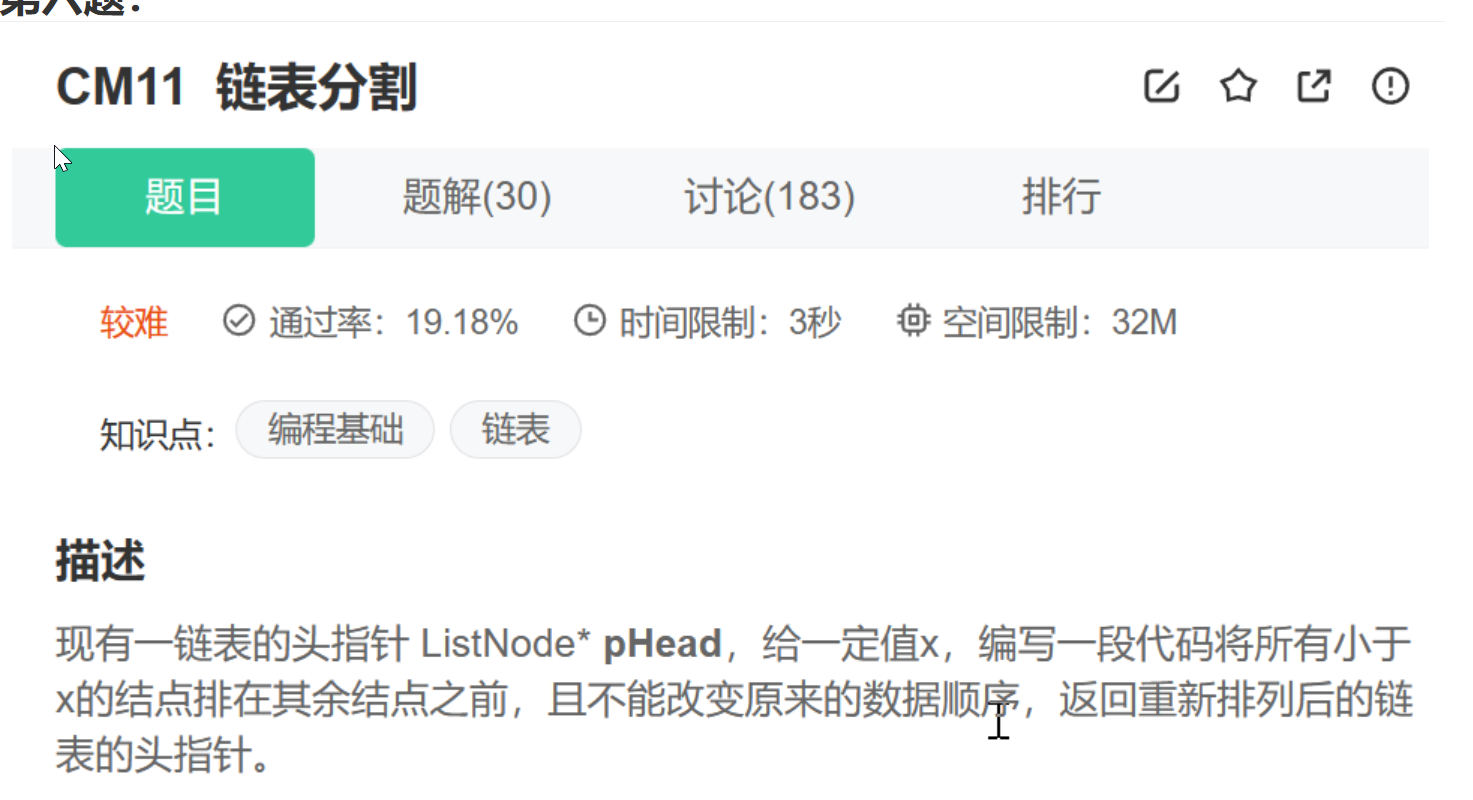
定义两个新的链表,将大于x的依次排列,将小于X的一次排列在另一个中,最后将大于X的链表尾插即可。
class Partition {
public:
ListNode* partition(ListNode* pHead, int x) {
// write code here
struct ListNode* gguard, *lguard, *ltail, *gtail;
gguard = gtail = (struct ListNode*)malloc(sizeof(struct ListNode ));
lguard = ltail = (struct ListNode*)malloc(sizeof(struct ListNode ));
gtail->next = ltail ->next = NULL;
struct ListNode* cur = pHead;
while(cur)
{
if(cur->val < x)
{
ltail->next = cur;
ltail = ltail->next;
}
else
{
gtail ->next = cur;
gtail = gtail->next;
}
cur = cur->next;
}
ltail->next =gguard->next;
gtail->next = NULL;
pHead = lguard->next;
free(gguard);
free(lguard);
return pHead;
}
};
第七题:
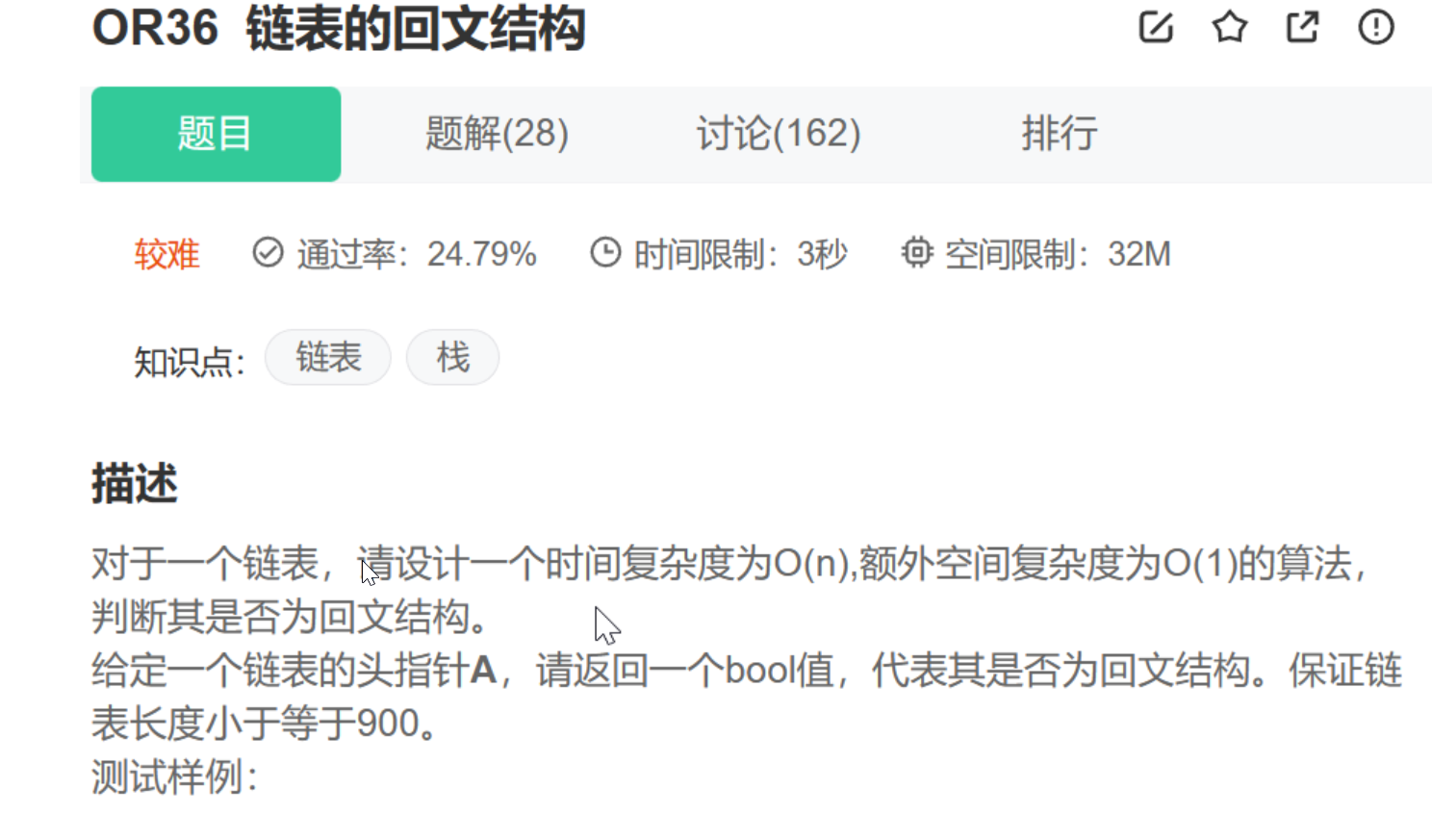
找中间点后反转再比较。
struct ListNode* reverseList(struct ListNode* head){
struct ListNode*n1,*n2,*n3;
if(head == NULL)
{
return NULL;
}
n1 = NULL;
n2 = head;
n3 = head->next;
while(n2)
{
n2->next = n1;
n1 = n2;
n2 = n3;
if(n3)
{
n3 = n3->next;
}
}
return n1;
}
struct ListNode* middleNode(struct ListNode* head){
struct ListNode* fast = head;
struct ListNode* slow = head;
while(fast && fast->next)
{
slow = slow->next;
fast = fast->next->next;
}
return slow;
}
class PalindromeList {
public:
bool chkPalindrome(ListNode* head) {
struct ListNode* rehead = middleNode(head);
struct ListNode* mid = reverseList(rehead);
while (head && rehead)
{
if(head->val != mid->val)
{
return false;
}
head = head->next;
rehead = rehead->next;
}
return true;
}
};
第八题:
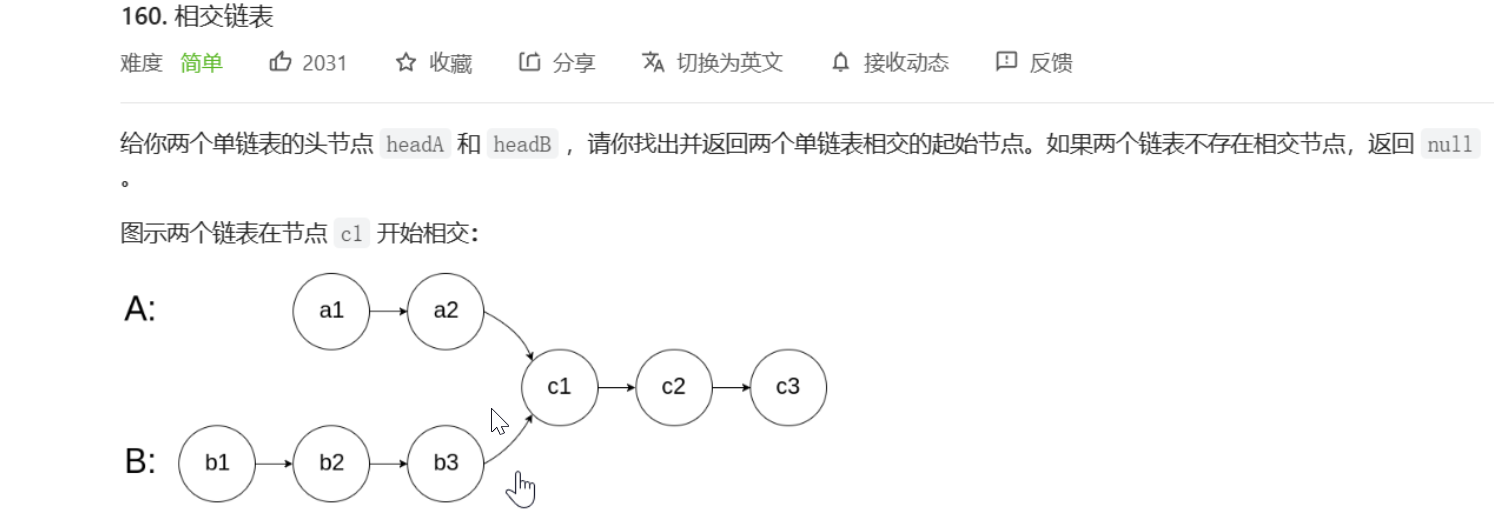
依然是快慢指针,快的先走,之后比较就行。
struct ListNode *getIntersectionNode(struct ListNode *headA, struct ListNode *headB) {
struct ListNode *cur1 =headA,*cur2 = headB;
struct ListNode *fast =headA,*slow = headB;
int count1 =0,count2 = 0;
while(cur1)
{
cur1 = cur1->next;
count1++;
}
while(cur2)
{
cur2 = cur2->next;
count2++;
}
if(cur1 != cur2)//判段是否有交点
{
return NULL;
}
int num = fabs(count1-count2);
if(count1 <count2)
{
fast = headB;
slow = headA;
}
while(num--)
{
fast = fast->next;
}
while(fast != slow)
{
fast =fast->next;
slow = slow->next;
}
return fast;
}