Qt中的信号量来实现生产者和消费者两个线程同时访问同一块内存
#include <QSemaphore>
#include <QThread>
#include <QObject>
#include <iostream>
#include <thread>
#include <QRandomGenerator>
QSemaphore g_freeByte(100);
QSemaphore g_useByte;
std::vector<int> g_dataVec;
class ProducerData : public QThread
{
Q_OBJECT
public:
explicit ProducerData(QObject* parent = nullptr);
protected:
void run();
};
ProducerData::ProducerData(QObject* parent)
: QThread(parent)
{
}
void ProducerData::run()
{
g_dataVec.clear();
QRandomGenerator* pRand = QRandomGenerator::global();
while (true)
{
g_freeByte.acquire();
int info = pRand->bounded(1000);
std::cout << "生产者数据:" << info << std::endl;
g_dataVec.emplace_back(info);
g_useByte.release();
}
exec();
}
class Consumer : public QThread
{
Q_OBJECT
public:
explicit Consumer(QObject* parent = nullptr);
protected:
void run();
};
Consumer::Consumer(QObject* parent)
: QThread(parent)
{
}
void Consumer::run()
{
while (true)
{
g_useByte.acquire();
if (g_dataVec.empty())continue;
std::cout << "消费者拿走数据:"<<g_dataVec.front() << std::endl;
g_dataVec.erase(g_dataVec.begin());
g_freeByte.release();
msleep(200);
}
exec();
}
int main()
{
ProducerData pro;
pro.start();
Consumer con;
con.start();
return 0;
}
#pragma once
#include <queue>
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
class ProducerThread
{
public:
explicit ProducerThread(int task = 1);
void start();
private:
void producerData();
private:
int m_taskNum;
};
class ConsumerThread
{
public:
explicit class ConsumerThread(int taskNum = 1);
void start();
private:
void consumerData();
private:
int m_staskNum;
};
#include "DesignModel.h"
#include <random>
#include <chrono>
std::queue<int> g_queue;
std::mutex g_mutex;
std::condition_variable g_condition;
#define Product_Max 100
ProducerThread::ProducerThread(int task)
: m_taskNum(task)
{
}
void ProducerThread::start()
{
for (int i = 0; i < m_taskNum; i++)
{
std::thread thread(&ProducerThread::producerData, this);
thread.detach();
}
}
void ProducerThread::producerData()
{
srand(time(NULL));
while (true)
{
std::unique_lock<std::mutex> lock(g_mutex);
std::this_thread::sleep_for(std::chrono::milliseconds(300));
while (g_queue.size() > Product_Max)
{
g_condition.wait(lock);
}
int data = rand() % 1000 - 0;
g_queue.push(data);
std::cout << std::this_thread::get_id() << "生产数据:" << data << std::endl;
g_condition.notify_all();
}
}
ConsumerThread::ConsumerThread(int taskNum)
: m_staskNum(taskNum)
{
}
void ConsumerThread::start()
{
for (int i = 0; i < m_staskNum; i++)
{
std::thread thread(&ConsumerThread::consumerData, this);
thread.detach();
}
}
void ConsumerThread::consumerData()
{
srand(time(NULL));
while (true)
{
std::this_thread::sleep_for(std::chrono::milliseconds(800));
std::unique_lock<std::mutex> lock(g_mutex);
while (g_queue.empty())
{
g_condition.wait(lock);
}
std::cout << std::this_thread::get_id() << "消费数据:" << g_queue.front() << std::endl;
g_queue.pop();
g_condition.notify_all();
}
}
int main()
{
ProducerThread pro(8);
pro.start();
ConsumerThread con(10);
con.start();
system("pause");
return 0;
}
Qt用信号量,c++用条件变量实现生产者和消费者模式
于 2023-04-04 16:55:54 首次发布
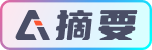