ToolBarManager是对SWT的ToolBar控件的封装,用它可以省去对这些底层控件的关注,就像使用了TableViewer,就不需要再去关心TableItem这些繁琐的细节一样。
在一般情况下我们都是向ToolBarManager里面添加Action,显示的效果就是一个Button。如果希望显示一个Combo或者Text这样的控 件,就不能向其中添加Action了,而且又不想使用ToolBar处理具体的ToolItem,以下是两种解决办法:
第一种:
构建自己的ControlContribution 就可以很容易的实现这样的功能。
首先要继承ControlContribution类,实现其protected Control createControl(Composite parent)方法,在这个方法中构建自己希望的控件,只要是Control的子类就都可以,然后将其添加到ToolBarManager里面即可。
以下即为在View的工具栏中添加自定义控件Combo
第一步、新建类 ComboContribution,并继承ControlContribution类,然后实现其protected Control createControl(Composite parent)方法,在这个方法中构建Combo控件
class
ComboContribution
extends
ControlContribution
...
{


public ComboContribution(String id)...{
super(id);
}

@Override

protected Control createControl(Composite parent)...{
Combo combo = new Combo(parent, SWT.READ_ONLY);

combo.setItems(new String[]...{ "First", "Secend", "Third" });

combo.addSelectionListener(new SelectionListener()...{


public void widgetDefaultSelected(SelectionEvent e) ...{
// TODO Auto-generated method stub
}


public void widgetSelected(SelectionEvent e) ...{
// TODO Auto-generated method stub
textSysInfo.append("View工具栏测试!");
}
});
return combo;
}
}
第二步、
private
void
initializeToolBar()中将其添加到ToolBarManager
/** */
/**
* Initialize the toolbar
*/

private
void
initializeToolBar()
...
{
IToolBarManager toolbarManager = getViewSite().getActionBars().getToolBarManager();

Action deleteAction = new Action()...{

public void run()...{
textSysInfo.setText("");
}
};
deleteAction.setText("清空");
deleteAction.setToolTipText("清空系统信息");
deleteAction.setImageDescriptor(PlatformUI.getWorkbench().
getSharedImages().getImageDescriptor(ISharedImages.IMG_TOOL_DELETE));
toolbarManager.add(deleteAction);
ComboContribution combo = new ComboContribution("Combo.contribution");
toolbarManager.add(combo);
}
第二种:
于第一种类似,只是实现的是 继承ContributionItem类,也是实现其protected Control createControl(Composite parent)方法,在这个方法中构建自定义控件。
代码如下:
class
ComboContribution2
extends
ContributionItem
...
{

private ToolItem toolitem;

private Combo fFindCombo;

private Button upFindbutton;

private Button downFindbutton;

private Button allFindbutton;


public ComboContribution2() ...{
super();
}

protected Control createControl(Composite parent) ...{
Composite composite = new Composite(parent, SWT.NONE);
//查询框
fFindCombo = new Combo(composite,SWT.NONE);
fFindCombo.setLocation(0, 2);
fFindCombo.setSize(130,20);
System.out.println(" fFindCombo == " + fFindCombo.getBounds());
//向上查
upFindbutton = new Button(composite, SWT.NONE);
upFindbutton.setLocation(135, 2);
upFindbutton.setSize(30,20);
upFindbutton.setText("上查");

upFindbutton.addSelectionListener(new SelectionListener()...{


public void widgetDefaultSelected(SelectionEvent e) ...{
// TODO 自动生成方法存根
}


public void widgetSelected(SelectionEvent e) ...{
fFindCombo.add(fFindCombo.getText());
}
});
System.out.println(" upFindbutton == " + upFindbutton.getBounds());
//向下查
downFindbutton = new Button(composite, SWT.NONE);
downFindbutton.setLocation(170, 2);
downFindbutton.setSize(30,20);
downFindbutton.setText("下查");
//全部查询
allFindbutton = new Button(composite, SWT.NONE);
allFindbutton.setLocation(205, 2);
allFindbutton.setSize(30,20);
allFindbutton.setText("全部");

toolitem.setWidth(240);

return composite;
}


public void fill(ToolBar parent, int index) ...{
toolitem = new ToolItem(parent, SWT.SEPARATOR, index);
Control control = createControl(parent);
toolitem.setControl(control);
}

}
然后同样在View的
private
void
initializeToolBar()方法中将其添加到ToolBarManager即可。
在一般情况下我们都是向ToolBarManager里面添加Action,显示的效果就是一个Button。如果希望显示一个Combo或者Text这样的控 件,就不能向其中添加Action了,而且又不想使用ToolBar处理具体的ToolItem,以下是两种解决办法:
第一种:
构建自己的ControlContribution 就可以很容易的实现这样的功能。
首先要继承ControlContribution类,实现其protected Control createControl(Composite parent)方法,在这个方法中构建自己希望的控件,只要是Control的子类就都可以,然后将其添加到ToolBarManager里面即可。
以下即为在View的工具栏中添加自定义控件Combo
第一步、新建类 ComboContribution,并继承ControlContribution类,然后实现其protected Control createControl(Composite parent)方法,在这个方法中构建Combo控件


















































getSharedImages().getImageDescriptor(ISharedImages.IMG_TOOL_DELETE));





于第一种类似,只是实现的是 继承ContributionItem类,也是实现其protected Control createControl(Composite parent)方法,在这个方法中构建自定义控件。
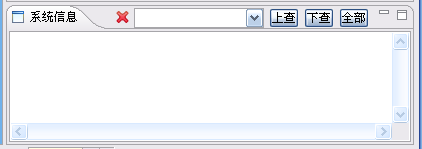
代码如下:










































































