//1.使用main函数的参数,实现一个整数计算器,程序可以接受三个参数,第一个参数“-a”选项执行加法,“-s”选项执行减法,“-m”选项执行乘法,
//“-d”选项执行除法,后面两个参数为操作数。
//例如:命令行参数输入:test.exe - a 1 2
//执行1 + 2输出3
//main函数参数解析运行时,需要在项目->属性->调试->命令行参数进行修改(格式为:-a 1 2)
#include<stdio.h>
#include<windows.h>
#include<stdlib.h>
int main(int argc, char *argv[])
{//将两个需要计算的数放进argv字符指针数组中
int num1 = atoi(argv[2]);//atoi函数功能:将字符串类型转换为int 型
int num2 = atoi(argv[3]);
if (argc != 4)
{
printf("参数输入错误");
return 1;
}
int ret = 0;
if (argv[1][0] == '-')//第二个参数判断
{
switch (argv[1][1])
{
case 'a':
ret = num1 + num2;
printf("%d\n", ret);
break;
case 's':
ret = num1 - num2;
printf("%d\n", ret);
break;
case 'm':
ret = num1*num2;
printf("%d\n", ret);
case 'd':
ret = num1 / num2;
printf("%d\n", ret);
default:
printf("第二个参数判断错误");
break;
}
}
system("pause");
return 0;
}
//下面这个程序是用atoi函数功能实现char*转为int型的
#include<stdio.h>
#include<windows.h>
#include<stdlib.h>
int main()
{
int n;
char *str = "12456.85";
n = atoi(str);
printf("string=%s integer=%d\n", str, n);
system("pause");
return 0;
}
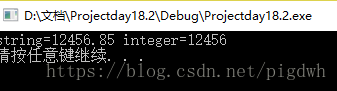
//2.写冒泡排序可以排序多个字符串。
//原理:根据多个字符串里面的字符比较,也就是根据其对应的ASC‖码进行比较,然后用冒泡排序法进行比较。
/*冒泡排序法的原理:设数组长度为N,从小到大排序。
1.比较相邻的前后二个数据,如果前面数据大于后面的数据,就将二个数据交换。
2.这样对数组的第0个数据到N - 1个数据进行一次遍历后,最大的一个数据就“沉”到数组第N - 1个位置。
3.N = N - 1,如果N不为0就重复前面二步,否则排序完成。*/
#include<stdio.h>
#include<windows.h>
#include<string.h>
#include<assert.h>
#pragma warning(disable:4996)
void Exchang(char *str1, char *str2)
{
assert(str1);
assert(str2);
char *temp;
temp= str1;
str1 = str2;
str2 = temp;
}
void BubbleSort(char *str[5], const int len)
{
assert(str);//校验
assert(len > 0);
int i = 0;
int j = 0;
int flage = 1;
for (; i < len - 1; i++)
{
flage = 0;
for (j = 0; j < len-i-1; j++)
{
if (strcmp(str[j], str[j + 1]) > 0)//用比较字符串来比较两个字符串的大小,strcmp(char const *s1,char const *s2);
//如果s1小于s2,strcmp函数返回一个小于0的值;如果大于,函数返回一个大于0的值,如果相等,函数返回0.
{
Exchang(str[j], str[i]);//交换两个字符串
//char *temp = str[j];
//str[j] = str[j + 1];
//str[j + 1] = temp;
flage = 1;
}
}
if (flage == 0);
{
break;
}
}
return 0;
}
int main()
{
char *str[] = { "abcd", "cdefg", "abcde", "bcdef", "cdefghab" };
int len = sizeof(str) / sizeof(str[0]);
BubbleSort(str, len);
int i = 0;
for (i = 0; i < len; i++)
{
printf("%s\n", str[i]);
}
printf("\n");
system("pause");
return 0;
}
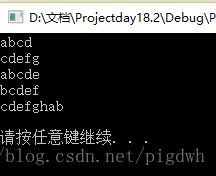