Galois is one of the strongest chess players of Byteforces. He has even invented a new variant of chess, which he named «PawnChess».
This new game is played on a board consisting of 8 rows and 8 columns. At the beginning of every game some black and white pawns are placed on the board. The number of black pawns placed is not necessarily equal to the number of white pawns placed.
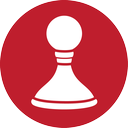
Lets enumerate rows and columns with integers from 1 to 8. Rows are numbered from top to bottom, while columns are numbered from left to right. Now we denote as (r, c) the cell located at the row r and at the column c.
There are always two players A and B playing the game. Player A plays with white pawns, while player B plays with black ones. The goal of player A is to put any of his pawns to the row 1, while player B tries to put any of his pawns to the row 8. As soon as any of the players completes his goal the game finishes immediately and the succeeded player is declared a winner.
Player A moves first and then they alternate turns. On his move player A must choose exactly one white pawn and move it one step upward and player B (at his turn) must choose exactly one black pawn and move it one step down. Any move is possible only if the targeted cell is empty. It's guaranteed that for any scenario of the game there will always be at least one move available for any of the players.
Moving upward means that the pawn located in (r, c) will go to the cell (r - 1, c), while moving down means the pawn located in (r, c) will go to the cell (r + 1, c). Again, the corresponding cell must be empty, i.e. not occupied by any other pawn of any color.
Given the initial disposition of the board, determine who wins the game if both players play optimally. Note that there will always be a winner due to the restriction that for any game scenario both players will have some moves available.
The input consists of the board description given in eight lines, each line contains eight characters. Character 'B' is used to denote a black pawn, and character 'W' represents a white pawn. Empty cell is marked with '.'.
It's guaranteed that there will not be white pawns on the first row neither black pawns on the last row.
Print 'A' if player A wins the game on the given board, and 'B' if player B will claim the victory. Again, it's guaranteed that there will always be a winner on the given board.
........ ........ .B....B. ....W... ........ ..W..... ........ ........
A
..B..... ..W..... ......B. ........ .....W.. ......B. ........ ........
B
In the first sample player A is able to complete his goal in 3 steps by always moving a pawn initially located at (4, 5). Player B needs at least 5 steps for any of his pawns to reach the row 8. Hence, player A will be the winner.
/*
简单的模拟题
给你一个8×8的矩阵 .表示可走的路 B表示black棋 W表示white
A下白棋 B下黑棋 A先走
A要让他的白棋到第一排 B要让他的黑棋到最后一排 A先走
数据保证有一方获胜 不存在打平的情况
如果棋子要前进的地方有别的棋子 这个棋子不能走过去
第二个样例可以很好的解释这一点 直接模拟一遍即可
*/
#include <cstdio>
#include <cstring>
#include <cmath>
#include <iostream>
#include <algorithm>
#include <string>
#include <cstdlib>
using namespace std;
char s[15][15];
int mp[15][15]={0};
char mark_1[15][15],mark_2[15][15];
int main(void)
{
for(int i=0;i<8;i++)
scanf("%s",s[i]);
int mi_1=10,mi_2=0;
int flag_1=0,flag_2=0;
for(int j=0;j<8;j++)
{
flag_1=0,flag_2=0;
int temp=-1;
for(int i=0;i<8;i++)
{
if(s[i][j]=='B')
{
if(flag_1==0) flag_1=1;
temp=i;
flag_2=0;
}
if(s[i][j]=='W')
{
flag_2=1;
temp=-1;
if(flag_1==1) continue; //说明有黑棋挡路
else if(flag_1==0)
{
flag_1=2;
mi_1=min(i,mi_1);
}
}
}
if(flag_2==0) mi_2=max(mi_2,temp);
}
//printf("%d %d\n",mi_1,7-mi_2);
if(mi_1<=7-mi_2) puts("A");
else puts("B");
return 0;
}
/*
数学题 找规律
题意:给你一个有n个顶点的凸多边形,每个点依次标号为1~n
从标号1开始到n不停的跟不相邻的点连接,不可以跟其他连的线相交
然后问你将这个凸多边形分成个几个部分。
*/
#include <cstdio>
#include <cstring>
#include <cmath>
#include <iostream>
#include <algorithm>
#include <string>
#include <cstdlib>
using namespace std;
int main()
{
long long n;
while(scanf("%I64d",&n)!=EOF)
printf("%I64d\n",(n-2)*(n-2));
return 0;
}
Vector Willman and Array Bolt are the two most famous athletes of Byteforces. They are going to compete in a race with a distance of Lmeters today.
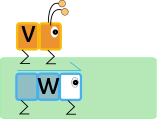
Willman and Bolt have exactly the same speed, so when they compete the result is always a tie. That is a problem for the organizers because they want a winner.
While watching previous races the organizers have noticed that Willman can perform only steps of length equal to w meters, and Bolt can perform only steps of length equal to b meters. Organizers decided to slightly change the rules of the race. Now, at the end of the racetrack there will be an abyss, and the winner will be declared the athlete, who manages to run farther from the starting point of the the racetrack (which is not the subject to change by any of the athletes).
Note that none of the athletes can run infinitely far, as they both will at some moment of time face the point, such that only one step further will cause them to fall in the abyss. In other words, the athlete will not fall into the abyss if the total length of all his steps will be less or equal to the chosen distance L.
Since the organizers are very fair, the are going to set the length of the racetrack as an integer chosen randomly and uniformly in range from 1 to t (both are included). What is the probability that Willman and Bolt tie again today?
The first line of the input contains three integers t, w and b (1 ≤ t, w, b ≤ 5·1018) — the maximum possible length of the racetrack, the length of Willman's steps and the length of Bolt's steps respectively.
Print the answer to the problem as an irreducible fraction . Follow the format of the samples output.
The fraction (p and q are integers, and both p ≥ 0 and q > 0 holds) is called irreducible, if there is no such integer d > 1, that both p andq are divisible by d.
10 3 2
3/10
7 1 2
3/7
In the first sample Willman and Bolt will tie in case 1, 6 or 7 are chosen as the length of the racetrack.
/*
题意:给你一个数t。问你在[1,t]里面有多少个整数num
满足num % w == num % b。
输出num/t
*/
#include <cstdio>
#include <cstring>
#include <cmath>
#include <iostream>
#include <algorithm>
#include <string>
#include <cstdlib>
using namespace std;
int main(void)
{
unsigned long long t,w,b,gcd,lcm,ans,temp;
cin>>t>>w>>b;
gcd=__gcd(w,b);
lcm=w/gcd;
ans=t/lcm/b;
temp=ans;
ans+=ans*(min(w,b)-1)+min(t-ans*lcm*b,min(w,b)-1);
gcd=__gcd(ans,t);
cout<<ans/gcd<<"/"<<t/gcd<<endl;
return 0;
}