http://my.oschina.net/Tsybius2014/blog/294249?p=1
一、关于本文
本文中是一个类库,包括下面几个函数:
1)计算32位MD5码(大小写):Hash_MD5_32
2)计算16位MD5码(大小写):Hash_MD5_16
3)计算32位2重MD5码(大小写):Hash_2_MD5_32
4)计算16位2重MD5码(大小写):Hash_2_MD5_16
5)计算SHA-1码(大小写):Hash_SHA_1
6)计算SHA-256码(大小写):Hash_SHA_256
7)计算SHA-384码(大小写):Hash_SHA_384
8)计算SHA-512码(大小写):Hash_SHA_512
编译后被打包成文件HashTools.dll,其他程序可以在添加引用后对这些函数进行调用
二、类库中各函数代码
0)类库结构
1
2
3
4
5
6
7
8
9
10
11
12
13
|
using
System;
using
System.Collections.Generic;
using
System.Linq;
using
System.Text;
using
System.Threading.Tasks;
namespace
HashTools
{
public
class
HashHelper
{
//各个函数
}
}
|
1)计算32位MD5码(大小写):Hash_MD5_32
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
/// <summary>
/// 计算32位MD5码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_MD5_32(
string
word,
bool
toUpper =
true
)
{
try
{
System.Security.Cryptography.MD5CryptoServiceProvider MD5CSP
=
new
System.Security.Cryptography.MD5CryptoServiceProvider();
byte
[] bytValue = System.Text.Encoding.UTF8.GetBytes(word);
byte
[] bytHash = MD5CSP.ComputeHash(bytValue);
MD5CSP.Clear();
//根据计算得到的Hash码翻译为MD5码
string
sHash =
""
, sTemp =
""
;
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
//根据大小写规则决定返回的字符串
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
2)计算16位MD5码(大小写):Hash_MD5_16
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
/// <summary>
/// 计算16位MD5码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_MD5_16(
string
word,
bool
toUpper =
true
)
{
try
{
string
sHash = Hash_MD5_32(word).Substring(8, 16);
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
3)计算32位2重MD5码(大小写):Hash_2_MD5_32
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
|
/// <summary>
/// 计算32位2重MD5码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_2_MD5_32(
string
word,
bool
toUpper =
true
)
{
try
{
System.Security.Cryptography.MD5CryptoServiceProvider MD5CSP
=
new
System.Security.Cryptography.MD5CryptoServiceProvider();
byte
[] bytValue = System.Text.Encoding.UTF8.GetBytes(word);
byte
[] bytHash = MD5CSP.ComputeHash(bytValue);
//根据计算得到的Hash码翻译为MD5码
string
sHash =
""
, sTemp =
""
;
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
bytValue = System.Text.Encoding.UTF8.GetBytes(sHash);
bytHash = MD5CSP.ComputeHash(bytValue);
MD5CSP.Clear();
sHash =
""
;
//根据计算得到的Hash码翻译为MD5码
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
//根据大小写规则决定返回的字符串
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
4)计算16位2重MD5码(大小写):Hash_2_MD5_16
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
|
/// <summary>
/// 计算16位2重MD5码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_2_MD5_16(
string
word,
bool
toUpper =
true
)
{
try
{
System.Security.Cryptography.MD5CryptoServiceProvider MD5CSP
=
new
System.Security.Cryptography.MD5CryptoServiceProvider();
byte
[] bytValue = System.Text.Encoding.UTF8.GetBytes(word);
byte
[] bytHash = MD5CSP.ComputeHash(bytValue);
//根据计算得到的Hash码翻译为MD5码
string
sHash =
""
, sTemp =
""
;
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
sHash = sHash.Substring(8, 16);
bytValue = System.Text.Encoding.UTF8.GetBytes(sHash);
bytHash = MD5CSP.ComputeHash(bytValue);
MD5CSP.Clear();
sHash =
""
;
//根据计算得到的Hash码翻译为MD5码
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
sHash = sHash.Substring(8, 16);
//根据大小写规则决定返回的字符串
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
5)计算SHA-1码(大小写):Hash_SHA_1
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
/// <summary>
/// 计算SHA-1码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_SHA_1(
string
word,
bool
toUpper =
true
)
{
try
{
System.Security.Cryptography.SHA1CryptoServiceProvider SHA1CSP
=
new
System.Security.Cryptography.SHA1CryptoServiceProvider();
byte
[] bytValue = System.Text.Encoding.UTF8.GetBytes(word);
byte
[] bytHash = SHA1CSP.ComputeHash(bytValue);
SHA1CSP.Clear();
//根据计算得到的Hash码翻译为SHA-1码
string
sHash =
""
, sTemp =
""
;
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
//根据大小写规则决定返回的字符串
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
6)计算SHA-256码(大小写):Hash_SHA_256
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
/// <summary>
/// 计算SHA-256码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_SHA_256(
string
word,
bool
toUpper =
true
)
{
try
{
System.Security.Cryptography.SHA256CryptoServiceProvider SHA256CSP
=
new
System.Security.Cryptography.SHA256CryptoServiceProvider();
byte
[] bytValue = System.Text.Encoding.UTF8.GetBytes(word);
byte
[] bytHash = SHA256CSP.ComputeHash(bytValue);
SHA256CSP.Clear();
//根据计算得到的Hash码翻译为SHA-1码
string
sHash =
""
, sTemp =
""
;
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
//根据大小写规则决定返回的字符串
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
7)计算SHA-384码(大小写):Hash_SHA_384
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
/// <summary>
/// 计算SHA-384码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_SHA_384(
string
word,
bool
toUpper =
true
)
{
try
{
System.Security.Cryptography.SHA384CryptoServiceProvider SHA384CSP
=
new
System.Security.Cryptography.SHA384CryptoServiceProvider();
byte
[] bytValue = System.Text.Encoding.UTF8.GetBytes(word);
byte
[] bytHash = SHA384CSP.ComputeHash(bytValue);
SHA384CSP.Clear();
//根据计算得到的Hash码翻译为SHA-1码
string
sHash =
""
, sTemp =
""
;
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
//根据大小写规则决定返回的字符串
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
8)计算SHA-512码(大小写):Hash_SHA_512
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
/// <summary>
/// 计算SHA-512码
/// </summary>
/// <param name="word">字符串</param>
/// <param name="toUpper">返回哈希值格式 true:英文大写,false:英文小写</param>
/// <returns></returns>
public
static
string
Hash_SHA_512(
string
word,
bool
toUpper =
true
)
{
try
{
System.Security.Cryptography.SHA512CryptoServiceProvider SHA512CSP
=
new
System.Security.Cryptography.SHA512CryptoServiceProvider();
byte
[] bytValue = System.Text.Encoding.UTF8.GetBytes(word);
byte
[] bytHash = SHA512CSP.ComputeHash(bytValue);
SHA512CSP.Clear();
//根据计算得到的Hash码翻译为SHA-1码
string
sHash =
""
, sTemp =
""
;
for
(
int
counter = 0; counter < bytHash.Count(); counter++)
{
long
i = bytHash[counter] / 16;
if
(i > 9)
{
sTemp = ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp = ((
char
)(i + 0x30)).ToString();
}
i = bytHash[counter] % 16;
if
(i > 9)
{
sTemp += ((
char
)(i - 10 + 0x41)).ToString();
}
else
{
sTemp += ((
char
)(i + 0x30)).ToString();
}
sHash += sTemp;
}
//根据大小写规则决定返回的字符串
return
toUpper ? sHash : sHash.ToLower();
}
catch
(Exception ex)
{
throw
new
Exception(ex.Message);
}
}
|
三、函数调用
建立项目ComputeHash,添加对HashTools.dll库的引用。并添加代码:
1
|
using
HashTools;
|
然后在Main函数中添加下列代码:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
|
static
void
Main(
string
[] args)
{
Console.WriteLine(
"MD5 of \"abc\""
);
Console.WriteLine(
"MD5_32(Upper): {0}"
,
HashHelper.Hash_MD5_32(
"abc"
));
Console.WriteLine(
"MD5_32(Lower): {0}"
,
HashHelper.Hash_MD5_32(
"abc"
,
false
));
Console.WriteLine(
"MD5_16(Upper): {0}"
,
HashHelper.Hash_MD5_16(
"abc"
));
Console.WriteLine(
"MD5_16(Lower): {0}"
,
HashHelper.Hash_MD5_16(
"abc"
,
false
));
Console.WriteLine(
"2_MD5_32(Upper): {0}"
,
HashHelper.Hash_2_MD5_32(
"abc"
));
Console.WriteLine(
"2_MD5_32(Lower): {0}"
,
HashHelper.Hash_2_MD5_32(
"abc"
,
false
));
Console.WriteLine(
"2_MD5_32(Upper): {0}"
,
HashHelper.Hash_2_MD5_16(
"abc"
));
Console.WriteLine(
"2_MD5_32(Lower): {0}"
,
HashHelper.Hash_2_MD5_16(
"abc"
,
false
));
Console.WriteLine(
"SHA of \"abc\""
);
Console.WriteLine(
"SHA-1(Upper): {0}"
,
HashHelper.Hash_SHA_1(
"abc"
));
Console.WriteLine(
"SHA-1(Lower): {0}"
,
HashHelper.Hash_SHA_1(
"abc"
,
false
));
Console.WriteLine(
"SHA-256(Upper): {0}"
,
HashHelper.Hash_SHA_256(
"abc"
));
Console.WriteLine(
"SHA-256(Lower): {0}"
,
HashHelper.Hash_SHA_256(
"abc"
,
false
));
Console.WriteLine(
"SHA-384(Upper): {0}"
,
HashHelper.Hash_SHA_384(
"abc"
));
Console.WriteLine(
"SHA-384(Lower): {0}"
,
HashHelper.Hash_SHA_384(
"abc"
,
false
));
Console.WriteLine(
"SHA-512(Upper): {0}"
,
HashHelper.Hash_SHA_512(
"abc"
));
Console.WriteLine(
"SHA-512(Lower): {0}"
,
HashHelper.Hash_SHA_512(
"abc"
,
false
));
Console.ReadLine();
}
|
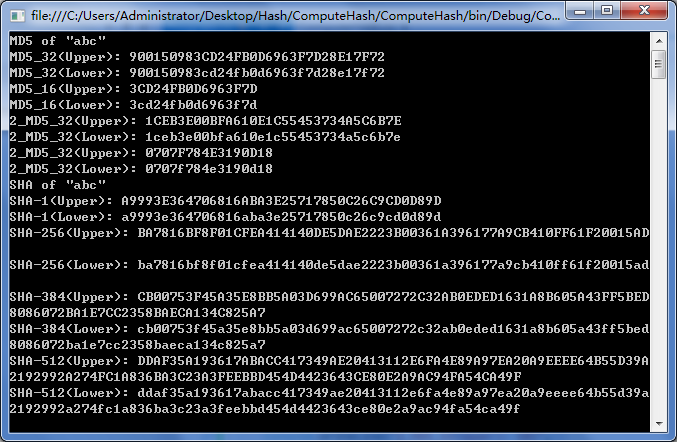
END