一、采用前后端分离开发
二、前端技术
1、vue基本语法
- 常见指令:
- v-bind 简写(冒号):
- v-model
- v-for
- v-if
- v-html
- 生命周期:
- created(),页面渲染之前
- mounted(),页面渲染之后
- 绑定事件:
- -v-on 简写@
- v-onclick == @click
- ES6规范,通过babel转成ES5,框架自带
2、Element-UI
3、Nodejs
-
Js运行环境,不需要浏览器直接运行代码,模拟服务器效果
4、NPM
包管理工具,类似Maven
- npm命令
- npm init初始化
- npm install 依赖名
5、Babel
转码器,ES6转换ES5代码
6、前端模块化
- 通过一个页面或js去调用另外一个页面的js文件的方法
- ES6语法不能在nodejs中直接使用,需要通过babel编译成ES5再执行
7、后台系统用vue-admin-templete
基于vue、Element-ui、9528端口
8、前台系统用Nuxt
- 基于vue、3000端口
- 服务器渲染技术
9、Echarts
-
图表工具
- 百度捐给Apache
你能做全栈吗?
- 不要直接回答能或不能;
- 说:项目中有80%的后端都是我写的,前端都是cv的,对于前端技术有所了解
三、后端技术
1、微服务架构
将项目拆分为独立的模块,每个模块都有其端口号,模块与模块之间没有关系,是通过远程调用实现。
2、SpringBoot
SpringBoot是什么东西?
- SpringBoot本质就是spring,只是快速构建Spring工程的脚手架
启动类包扫描机制
- 从外往里扫,也可以设置扫描机制,通过@ComponentScan(包路径)
- 当前包及其子包下的所有组件
- 放在配置类中也可以(@configuration)
SpringBoot配置文件类型
- properties
- yml
配置文件加载机制
- 先bootstrap
- 再application-dev;对应的环境如:dev、test、prod
- 最后application
3、SpringCloud
- 是很多框架的总称,使用这些框架实现微服务架构,基于springboot实现
- 组成的框架由:
- eureka服务发现与服务注册,nacos
- OpenFeign服务调用
- Hystrix熔断器
- Gateway网关
- Config配置中心,nacos
- Bus消息总线,nacos
- 项目中,使用阿里巴巴nacos,代替springcloud一些组件
- 注册中心
- 配置中心
- Feign
- 服务调用,一个微服务调用另外一个微服务,实现远程调用
- 熔断器
- Gateway网关,之前是zuul
- 版本
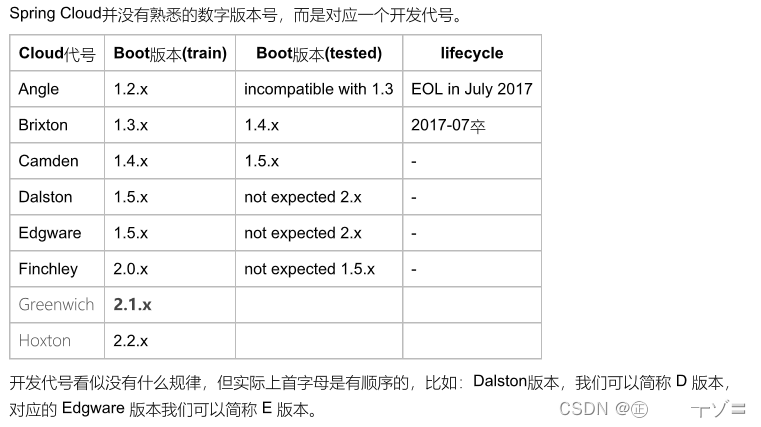
4、MyBatisPlus
- MyBatisPlus就是对MyBatis的增强,本身并没改变
- 自动填充
- 乐观锁
- 逻辑删除
- 代码生成器
5、EasyExcel
- 阿里巴巴提供操作Excel工具,效率高,代码简洁
为什么他效率高,代码简洁?
- 因为他封装了poi进行封装,采用SAX方法(一行一行操作)进行解析
- Dom 一次将所有数据放进内存中来
- 项目应用在添加课程分类,读取excel数据
6、SpringSecurity
-
项目整合框架实现权限管理功能
-
框架组成:
- 认证(登录)
- 授权(对用户授予权限)
- 登录认证过程
- 代码执行流程
1、认证过滤器(TokenLoginFilter)
- 继承UsernamePasswordAuthenticationFilter类
- spring官方建议构造器注入
public TokenLoginFilter(TokenManager tokenManager, RedisTemplate redisTemplate, AuthenticationManager authenticationManager) { this.tokenManager = tokenManager; this.redisTemplate = redisTemplate; this.authenticationManager = authenticationManager; //是否只接受post请求,如果是,则其他请求就会走认证失败的方法 this.setPostOnly(false); this.setRequiresAuthenticationRequestMatcher(new AntPathRequestMatcher("/admin/acl/login", "POST")); }
获取表单提交用户名和密码@Override public Authentication attemptAuthentication(HttpServletRequest request, HttpServletResponse response) throws AuthenticationException { //获取表单提交数据 //Jackson的方法:从ajax中直接读值封装成bean try { User user = new ObjectMapper().readValue(request.getInputStream(), User.class); //调取userDetailsService的数据库查询 return authenticationManager.authenticate( new UsernamePasswordAuthenticationToken( user.getUsername(), user.getPassword(), new ArrayList<>())); } catch (IOException e) { e.printStackTrace(); throw new RuntimeException(); } }
- 认证成功调用的方法
@Override protected void successfulAuthentication(HttpServletRequest request, HttpServletResponse response, FilterChain chain, Authentication authResult) throws IOException, ServletException { //principal就是认证之后的userDetail,包含权限信息 SecurityUser user = (SecurityUser) authResult.getPrincipal(); //根据用户名生成token String token = tokenManager.createToken(user.getCurrentUserInfo().getUsername()); //把用户信息和用户权限列表放到redis中去 redisTemplate.opsForValue().set(user.getCurrentUserInfo().getUsername(), user.getPermissionValueList()); //返回token ResponseUtil.out(response, R.ok().data("token", token)); }
- 认证失败调用的方法
@Override protected void unsuccessfulAuthentication(HttpServletRequest request, HttpServletResponse response, AuthenticationException failed) throws IOException, ServletException { ResponseUtil.out(response, R.error()); }
2、实现UserDetailsService
- 重写loadUserByUsername方法,进行密码的校验与权限列表的查询与赋值
3、授权过滤器(TokenAuthFilter)
- 每次用户发起请求都会经过授权过滤器
- 继承BasicAuthenticationFilter
@Override protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws IOException, ServletException { //获取当前认证成功用户权限信息 UsernamePasswordAuthenticationToken authRequest = getAuthentication(request); //判断:如果有权限信息,放在权限上下文中 if (authRequest != null) { SecurityContextHolder.getContext().setAuthentication(authRequest); } chain.doFilter(request, response); }
- 再从request的请求头中获取token,token中获取用户名,然后从redis根据用户名获取权限列表
private UsernamePasswordAuthenticationToken getAuthentication(HttpServletRequest request) { //从header获取token String token = request.getHeader("token"); if (token != null) { //从token获取用户名 String username = tokenManager.getUserInfoFromToken(token); //从redis获取对应权限列表信息 List<String> permissionValueList = (List<String>) redisTemplate.opsForValue().get(username); //Collection<? extends GrantedAuthority> authorities Collection<GrantedAuthority> authorities = new ArrayList<>(); for (String permissionValue : permissionValueList) { SimpleGrantedAuthority auth = new SimpleGrantedAuthority(permissionValue); authorities.add(auth); } return new UsernamePasswordAuthenticationToken(username, token, authorities); } return null; }
4、Spring Security使用一个Authentication对象来描述当前用户的相关信息。
- SecurityContextHolder中持有的是当前用户的SecurityContext,而SecurityContext持有的是代表当前用户相关信息的Authentication的引用。
- 在与系统交互的过程中,Spring Security会自动为我们创建相应的Authentication对象,然后赋值给当前的SecurityContext。
- 但是往往我们需要在程序中获取当前用户的相关信息,通过以下方法,进而获取其他相关信息。
String username = SecurityContextHolder.getContext().getAuthentication().getName();
7、Redis
- 首页数据通过Redis做缓存
- Redis数据类型:
- Set
- List
- Hash
- String
- zset
Redis做缓存,什么样的数据适合使用Redis做缓存?
经常访问,但不经常修改的数据;如主页
8、Nginx
- 方向代理服务器
- 请求转发、负载均衡、动静分离
9、OAuth2+JWT
- 针对特定问题的解决方案
- Jwt制定一种规则生成字符串,包括:三部分
- JWT头
- 有效载荷(用户信息)
- 防伪标志
10、HttpClient
- 模拟浏览器,请发请求响应的工具
- 项目中应用场景:微信登录获取扫描人信息,微信支付查询支付状态
11、Cookie
- 特点:
- 客户端技术,存储在浏览器、客户端中
- 每次发送请求,都会带着cookie
- cookie是会话级别
- cookie有默认有效时长:默认关闭浏览器就不存在了
- 也可以设置时长:setMaxAge方法
- 应用场景:
- 设置10天内免登录
12、微信登录、微信支付(见上)
13、阿里云OSS
- 文件存储
- 添加讲师,上次讲师头像
14、阿里云视频点播
- 视频上传、视频删除、视频播放
- 整合阿里云视频播放器播放
- 使用视频播放凭证播放
15、阿里云短信服务
- 注册时,发送手机验证码,存储到redis中校验
16、Git
- 代码提交到远程的Git仓库中
17、Docker+Jenkins
- 手动打包
- idea工具打包
- 自动化部署过程