业务需求
- 学校计算中心目前有四个规格不同的机房,由于管理的不便,特开发一套机房预约管理系统,以供老师管理和学生预约。
- 预约时间为下一周的周1到周5,可以选择上/下午时间段,以及具体的座位号,预约记录随时可取消。
预设身份
- 【学生】行为:申请预约、查看/取消预约。
- 【教师】行为:审核预约。
- 【管理员】行为:查看/添加/删除身份账号信息、查看/清空预约、查看/修改机房布局。
- 用户进入系统,选择身份类型,输入用户信息进行验证,通过后进入各自的子菜单。
- 用户信息包括用户ID、姓名和密码。
机房信息
编号 | 容量:布局 |
---|
1 | 36:6*6 |
2 | 96:8*12 |
3 | 48:6*8 |
4 | 24 :6*4 |
预约设置
- 读取预约文件并初始化容器记录。
- 可调取updateOrder()实时更新预约记录并存储至文件。
class OrderFile
{
public:
OrderFile();
void updateOrder();
int m_Size;
map<int, map<string, string> > m_orderData;
};
OrderFile::OrderFile()
{
this->m_Size = 0;
ifstream ifs;
ifs.open(ORDER_FILE, ios::in);
if (!ifs.is_open())
{
cout << "No File!" << endl;
ifs.close();
return;
}
string date;
string interval;
string stuId;
string stuName;
string roomId;
string deskId;
string status;
this->m_Size;
while (ifs >> date && ifs >> interval && ifs >> stuId && ifs >> stuName && ifs >> roomId && ifs >> deskId && ifs >> status)
{
string key;
string value;
map<string, string> m;
int pos = date.find(":");
if (pos != -1)
{
value = date.substr(pos + 1, date.size() - pos - 1);
m.insert(make_pair("date", value));
}
pos = interval.find(":");
if (pos != -1)
{
value = interval.substr(pos + 1, interval.size() - pos - 1);
m.insert(make_pair("interval", value));
}
pos = stuId.find(":");
if (pos != -1)
{
value = stuId.substr(pos + 1, stuId.size() - pos - 1);
m.insert(make_pair("stuId", value));
}
pos = stuName.find(":");
if (pos != -1)
{
value = stuName.substr(pos + 1, stuName.size() - pos - 1);
m.insert(make_pair("stuName", value));
}
pos = roomId.find(":");
if (pos != -1)
{
value = roomId.substr(pos + 1, roomId.size() - pos - 1);
m.insert(make_pair("roomId", value));
}
pos = deskId.find(":");
if (pos != -1)
{
value = deskId.substr(pos + 1, deskId.size() - pos - 1);
m.insert(make_pair("deskId", value));
}
pos = status.find(":");
if (pos != -1)
{
value = status.substr(pos + 1, status.size() - pos - 1);
m.insert(make_pair("status", value));
}
this->m_orderData.insert(make_pair(this->m_Size, m));
this->m_Size++;
}
ifs.close();
}
void OrderFile::updateOrder()
{
ofstream ofs(ORDER_FILE, ios::out | ios::trunc);
if(this->m_Size == 0)
{
return;
}
for (int i = 0; i < this->m_Size; i++)
{
ofs << "date:" << this->m_orderData[i]["date"] << " ";
ofs << "interval:" << this->m_orderData[i]["interval"] << " ";
ofs << "stuId:" << this->m_orderData[i]["stuId"] << " ";
ofs << "stuName:" << this->m_orderData[i]["stuName"] << " ";
ofs << "roomId:" << this->m_orderData[i]["roomId"] << " ";
ofs << "deskId:" << this->m_orderData[i]["deskId"] << " ";
ofs << "status:" << this->m_orderData[i]["status"] << endl;
}
ofs.close();
}
机房设置
- 初始化机房信息,可绘制机房布局。
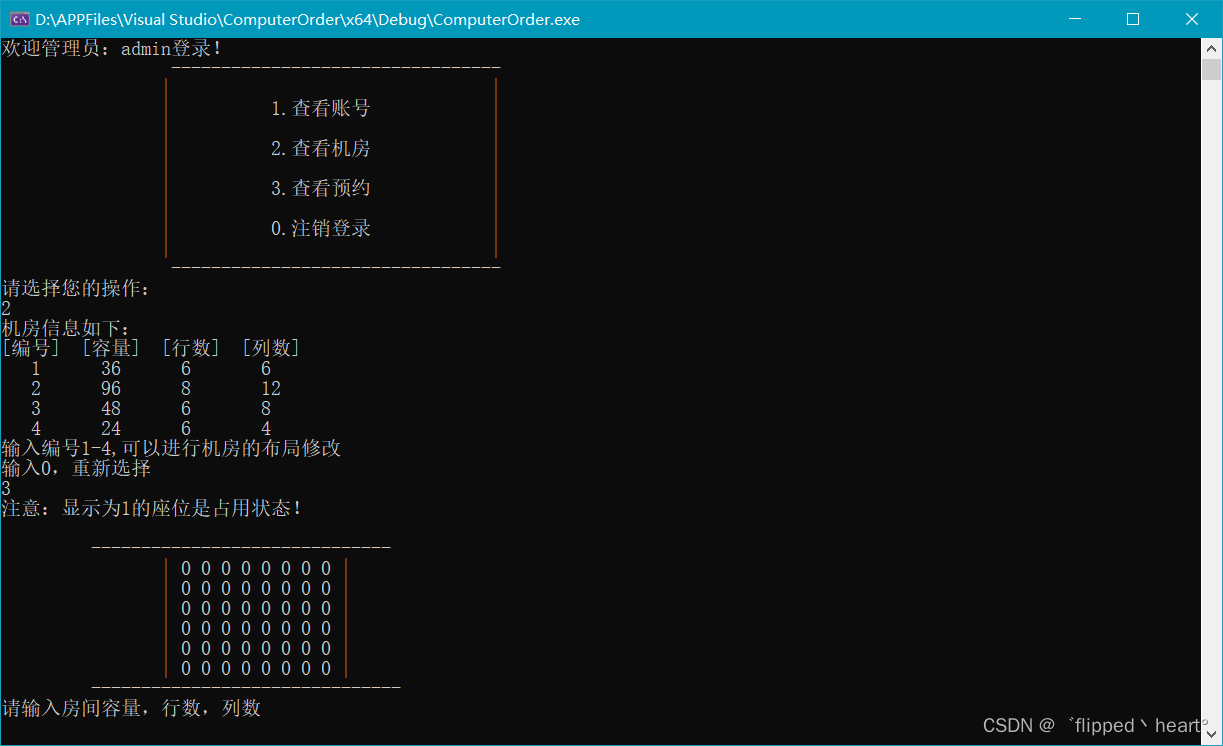
class ComputerRoom
{
public:
ComputerRoom(): m_ComId(0), m_MaxNum(0), row(0), col(0), layout("") {};
int m_ComId;
int m_MaxNum;
int row;
int col;
string layout;
void printLayout() const;
};
void ComputerRoom::printLayout() const
{
cout << "注意:显示为1的座位是占用状态!" << endl;
cout << "\n\t ------------------------------ " << endl;
for (int i = 0; i < this->row; i++)
{
cout << "\t\t| ";
for (int j = 0; j < this->col; j++)
{
cout << this->layout[j + i * this->col] << " ";
}
cout << "|\n";
}
cout << "\t ------------------------------- " << endl;
}
身份基类
- 定义纯虚函数openMenu()实现用户自定义菜单,可以在不同的身份子类中进行个性化定制。
- 定义静态机房和预约容器,保证各用户数据一致性。
class Identify
{
public:
virtual void openMenu() = 0;
string m_Name;
string m_Pwd;
static vector<ComputerRoom> vCom;
static OrderFile of;
}
学生
- 进入子菜单后,选择申请预约功能:选择下周内的时间(周1-周5),时间段(上午、下午),选择机房、选择座位号,确认预约信息。
- 选择查看预约功能:查看已预约的记录,可以对正在审核或者审核通过的记录进行取消。
- 选择注销功能:返回上一级登录界面。
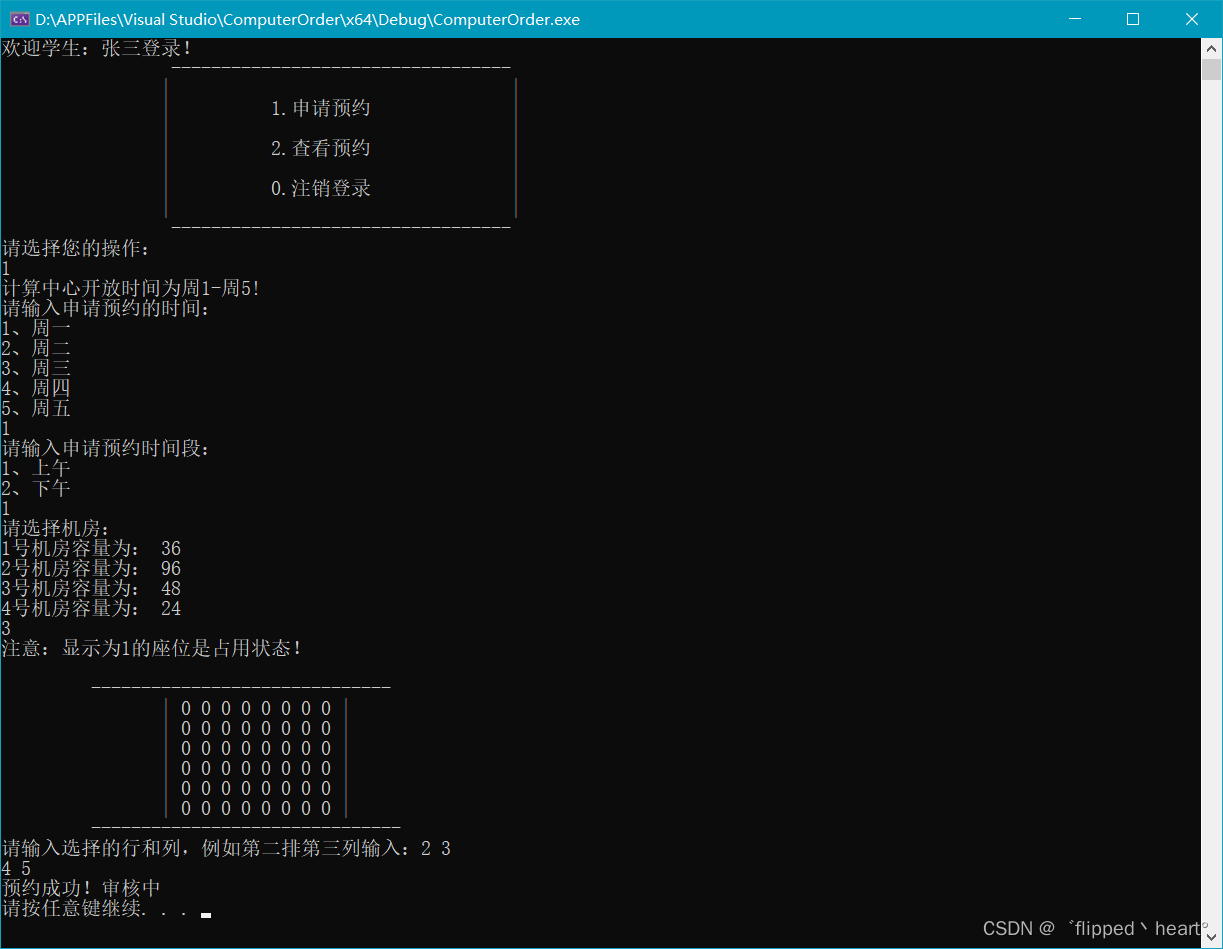
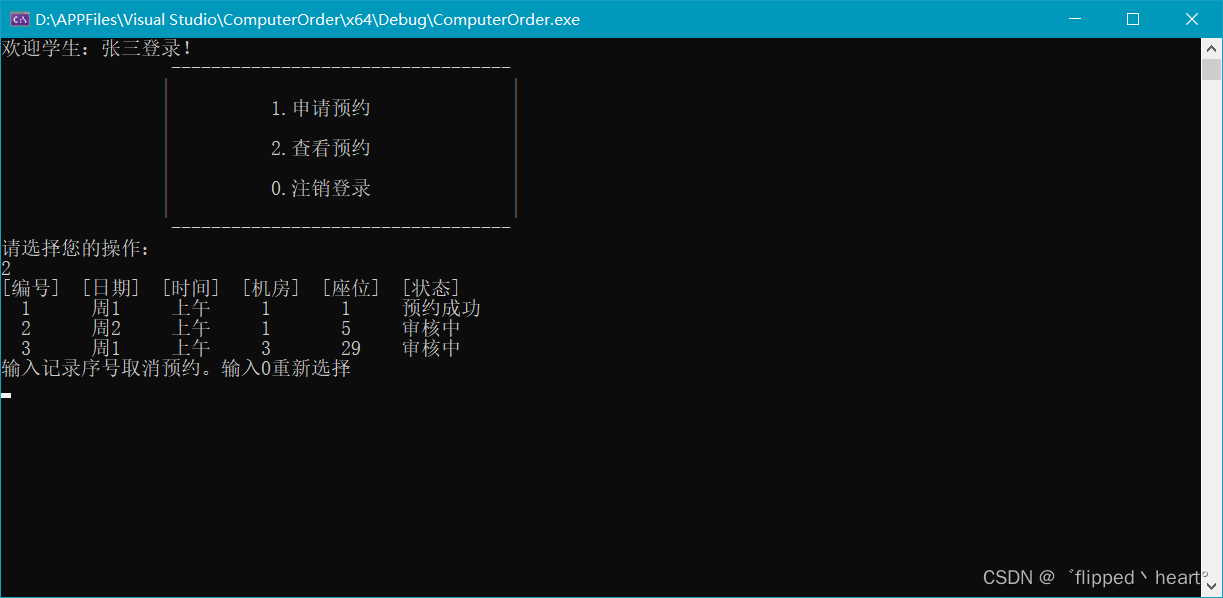
class Student: public Identify
{
public:
Student();
Student(const int& uid,const string& name,const string& pwd);
virtual void openMenu();
void applyOrder();
void showOrder();
void cancelOrder(const int& id);
void updateComputer() const;
int uid;
};
Student::Student()
{
this->uid = 0;
this->m_Name = "";
this->m_Pwd = "";
}
Student::Student(const int& uid, const string& name, const string& pwd)
{
this->udi = uid;
this->m_Name = name;
this->m_Pwd = pwd;
}
void Student::openMenu()
{
cout << "欢迎学生:" << this->m_Name << "登录!" << endl;
cout << "\t\t ----------------------------------\n";
cout << "\t\t| |\n";
cout << "\t\t| 1.申请预约 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 2.查看预约 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 0.注销登录 |\n";
cout << "\t\t| |\n";
cout << "\t\t ----------------------------------\n";
cout << "请选择您的操作: " << endl;
}
void Student::applyOrder()
{
int date = 0;
int interval = 0;
int room = 0;
cout << "计算中心开放时间为周1-周5!" << endl;
cout << "请输入申请预约的时间:" << endl;
cout << "1、周一" << endl;
cout << "2、周二" << endl;
cout << "3、周三" << endl;
cout << "4、周四" << endl;
cout << "5、周五" << endl;
while(true)
{
cin>>date;
if(date>=1 && date<=5)
{
break;
}
cout<<"Try again!"<<endl;
}
cout << "请输入申请预约时间段:" << endl;
cout << "1、上午" << endl;
cout << "2、下午" << endl;
while(true)
{
cin>>interval;
if(interval>=1 && interval<=2)
{
break;
}
cout<<"Try again!"<<endl;
}
cout << "请选择机房:" << endl;
for (int i = 0; i < ComputerRoom::num; i++)
{
cout << Identity::vCom[i].m_ComId << "号机房容量为: " << Identity::vCom[i].m_MaxNum << endl;
}
while (true)
{
cin >> room;
if (room >= 1 && room <= this->vCom.size())
{
break;
}
cout<<"Try again!"<<endl;
}
int T_room = (date - 1) * ComputerRoom::num * 2 + (interval - 1) * ComputerRoom::num + room;
Identity::vCom[T_room - 1].printLayout();
int r, l, t = 0;
cout << "请输入选择的行和列,例如第二排第三列输入:2 3" << endl;
while (true)
{
cin >> r >> l;
if (r <= Identity::vCom[T_room - 1].row && r >= 1 && l >= 1 && l <= Identity::vCom[T_room - 1].col)
{
t = l + (r - 1) * Identity::vCom[T_room - 1].col;
if (t <= Identity::vCom[T_room - 1].m_MaxNum)
{
if (Identity::vCom[T_room - 1].layout[t - 1] == '1')
{
cout << "该位置已被占用,请重新选择" << endl;
}
else
{
break;
}
}
else
{
cout<<"Try again!"<<endl;
}
}
else
{
cout<<"Try again!"<<endl;
}
}
bool flag = false;
for (int i = 0; i < Identity::of.m_Size; i++)
{
int f_date = atoi(Identity::of.m_orderData[i]["date"].c_str());
int f_interval = atoi(Identity::of.m_orderData[i]["interval"].c_str());
int f_uid = atoi(Identity::of.m_orderData[i]["stuId"].c_str());
string f_name = Identity::of.m_orderData[i]["stuName"];
int f_room = atoi(Identity::of.m_orderData[i]["roomId"].c_str());
int f_desk = atoi(Identity::of.m_orderData[i]["deskId"].c_str());
int f_status = atoi(Identity::of.m_orderData[i]["status"].c_str());
if (f_date == date && f_interval == interval && f_room == room && f_uid == this->uid)
{
if (f_status == 1 || f_status == -1 || f_status == 0)
{
Identity::of.m_orderData[i]["deskId"] = to_string(t);
Identity::of.m_orderData[i]["status"] = "1";
Identity::vCom[T_room - 1].layout[f_desk - 1] = '0';
Identity::vCom[T_room - 1].layout[t - 1] = '1';
cout << "预约成功!审核中!" << endl;
}
else if(f_status == 2)
{
cout << "预约失败!已完成该预约!" << endl;
}
flag = true;
break;
}
}
if (!flag)
{
string key;
string value;
map<string, string> m;
m.insert(make_pair("date", to_string(date)));
m.insert(make_pair("interval", to_string(interval)));
m.insert(make_pair("stuId", to_string(this->uid)));
m.insert(make_pair("stuName", this->m_Name));
m.insert(make_pair("roomId", to_string(room)));
m.insert(make_pair("deskId", to_string(t)));
m.insert(make_pair("status", "1"));
Identity::of.m_orderData.insert(make_pair(Identity::of.m_Size, m));
Identity::of.m_Size++;
Identity::vCom[T_room - 1].layout[t - 1] = '1';
cout << "预约成功!审核中" << endl;
}
Identity::of.updateOrder();
this->updateComputer();
system("pause");
system("cls");
}
void Student::updateComputer() const
{
ofstream ofs;
ofs.open(COMPUTER_FILE, ios::out | ios::trunc);
if (!ofs.is_open())
{
cout << "文件读取失败" << endl;
return;
}
for (vector<ComputerRoom>::iterator it = Identity::vCom.begin(); it != Identity::vCom.end(); it++)
{
ofs << it->m_ComId << " " << it->m_MaxNum << " " << it->row << " " << it->col << " " << it->layout << endl;
}
ofs.close();
}
void Student::showOrder()
{
if (Identity::of.m_Size == 0)
{
cout << "无预约记录!" << endl;
system("pause");
system("cls");
return;
}
cout << "[编号]\t[日期]\t[时间]\t[机房]\t[座位]\t[状态]" << endl;
int index = 1;
for (int i = 0; i < Identity::of.m_Size; i++)
{
if (this->uid == atoi(Identity::of.m_orderData[i]["stuId"].c_str()))
{
cout << " " << index++;
cout << "\t 周" << Identity::of.m_orderData[i]["date"];
cout << "\t " << (Identity::of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << "\t " << Identity::of.m_orderData[i]["roomId"];
cout << "\t " << Identity::of.m_orderData[i]["deskId"];
string status = "\t";
if (Identity::of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
else if (Identity::of.m_orderData[i]["status"] == "2")
{
status += "预约成功";
}
else if (Identity::of.m_orderData[i]["status"] == "-1")
{
status += "预约失败,审核未通过";
}
else
{
status += "预约已取消";
}
cout << status << endl;
}
}
if (index == 1)
{
cout << "无预约记录!" << endl;
system("pause");
system("cls");
}
else
{
int orderId;
cout << "输入记录序号取消预约。输入0重新选择" << endl;
cin >> orderId;
if (orderId)
{
cout << "确认取消?输入1确认,输入0重新选择" << endl;
int flag = 0;
cin >> flag;
if (flag)
{
this->cancelOrder(orderId);
}
else
{
system("pause");
system("cls");
}
}
else
{
system("pause");
system("cls");
}
}
}
void Student::cancelOrder(const int& orderId)
{
if (Identity::of.m_Size == 0 )
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
int index = 1;
for (int i = 0; i < Identity::of.m_Size; i++)
{
if (this->uid == atoi(Identity::of.m_orderData[i]["stuId"].c_str()))
{
if (index == orderId)
{
if (Identity::of.m_orderData[i]["status"] == "1" || Identity::of.m_orderData[i]["status"] == "2")
{
Identity::of.m_orderData[i]["status"] = "0";
int f_room = atoi(Identity::of.m_orderData[i]["roomId"].c_str());
int f_desk = atoi(Identity::of.m_orderData[i]["deskId"].c_str());
Identity::vCom[f_room - 1].layout[f_desk - 1] = '0';
cout << "取消成功" << endl;
}
else
{
cout << "不能取消" <<endl;
}
break;
}
else
{
index++;
}
}
}
Identity::of.updateOrder();
this->updateComputer();
system("pause");
system("cls");
}
教师
- 进入子菜单后,选择查看预约功能:可以查看所有学生的预约信息,可选择未完成审核的记录进行审核,可按照实际情况选择通过或不通过。
- 选择注销功能:返回上一级登录界面。
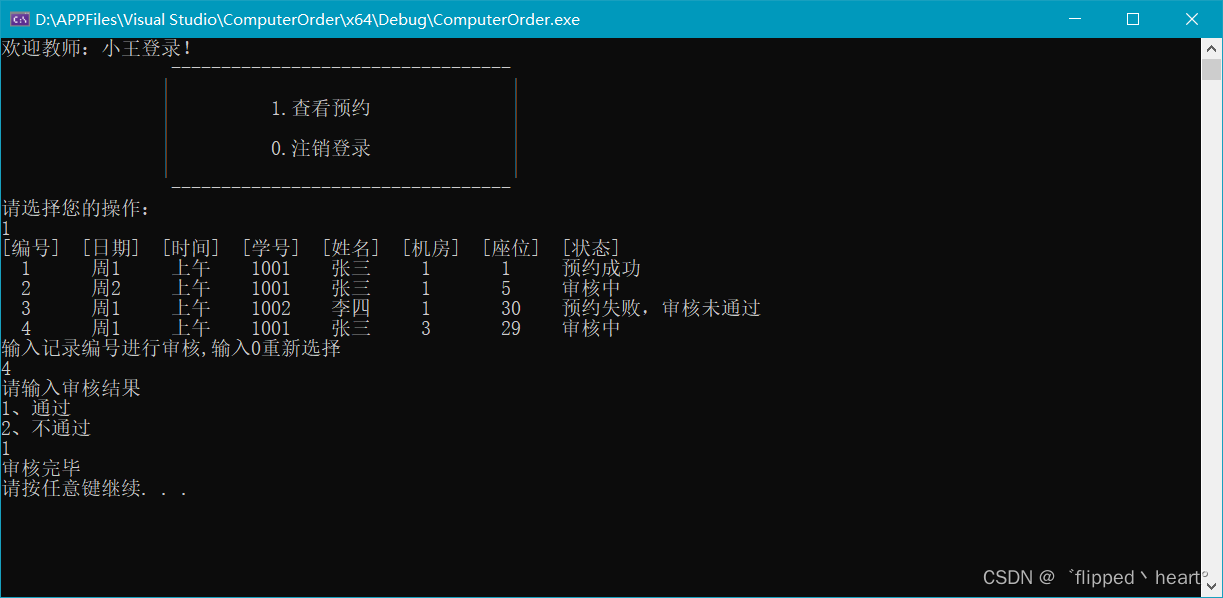
class Teacher: public Identify
{
public:
Teacher();
Teacher(const int& tid, const string& name, const string& pwd);
virtual void openMenu();
void showOrder();
void auditOrder(const int& id);
int tid;
};
Teacher::Teacher
{
this->tid = 0;
this->m_Name = "";
this->m_Pwd = "";
}
Teacher::Teacher(const int& tid, const string& name, const string& pwd)
{
this->tid = tid;
this->m_Name = name;
this->m_Pwd = pwd;
}
void Teacher::openMenu()
{
cout << "欢迎教师:" << this->m_Name << "登录!" << endl;
cout << "\t\t ----------------------------------\n";
cout << "\t\t| |\n";
cout << "\t\t| 1.查看预约 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 0.注销登录 |\n";
cout << "\t\t| |\n";
cout << "\t\t ----------------------------------\n";
cout << "请选择您的操作: " << endl;
}
void Teacher::showOrder()
{
if (Identity::of.m_Size == 0)
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
cout << "[编号]\t[日期]\t[时间]\t[学号]\t[姓名]\t[机房]\t[座位]\t[状态]" << endl;
for (int i = 0; i < Identity::of.m_Size; i++)
{
cout << " " << i + 1;
cout << "\t 周" << Identity::of.m_orderData[i]["date"];
cout << "\t " << (Identity::of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << "\t " << Identity::of.m_orderData[i]["stuId"];
cout << "\t " << Identity::of.m_orderData[i]["stuName"];
cout << "\t " << Identity::of.m_orderData[i]["roomId"];
cout << "\t " << Identity::of.m_orderData[i]["deskId"];
string status = "\t";
if (Identity::of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
else if (Identity::of.m_orderData[i]["status"] == "2")
{
status += "预约成功";
}
else if (Identity::of.m_orderData[i]["status"] == "-1")
{
status += "预约失败,审核未通过";
}
else
{
status += "预约已取消";
}
cout << status << endl;
}
int id;
cout << "输入记录编号进行审核,输入0重新选择" << endl;
cin >> id;
if (id > 0)
{
this->auditOrder(id);
}
else
{
system("pause");
system("cls");
}
}
void Teacher::auditOrder(const int& orderId)
{
if (Identity::of.m_Size == 0)
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
if (orderId > Identity::of.m_Size && orderId < 1)
{
cout << "编号有误" << endl;
system("pause");
system("cls");
return;
}
for (int i = 0; i < Identity::of.m_Size; i++)
{
if (i + 1 == orderId)
{
if (Identity::of.m_orderData[i]["status"] == "1")
{
int res;
cout << "请输入审核结果" << endl;
cout << "1、通过" << endl;
cout << "2、不通过" << endl;
cin >> res;
if (res == 1)
{
Identity::of.m_orderData[i]["status"] = "2";
}
else
{
Identity::of.m_orderData[i]["status"] = "-1";
}
Identity::of.updateOrder();
cout << "审核完毕" << endl;
}
else
{
cout<<"该记录不能审核" << endl;
}
break;
}
}
system("pause");
system("cls");
}
管理员
- 进入子菜单后,选择查看账号功能:可以查看所有学生和教师的账号信息,可删除或者添加账号。
- 选择查看预约功能:可以查看所有的预约信息,可选择是否清空所有的预约信息。
- 选择查看机房功能:可以查看所有的机房信息,可选择相应的机房信息及进行布局修改。
- 选择注销功能:返回上一级登录界面。
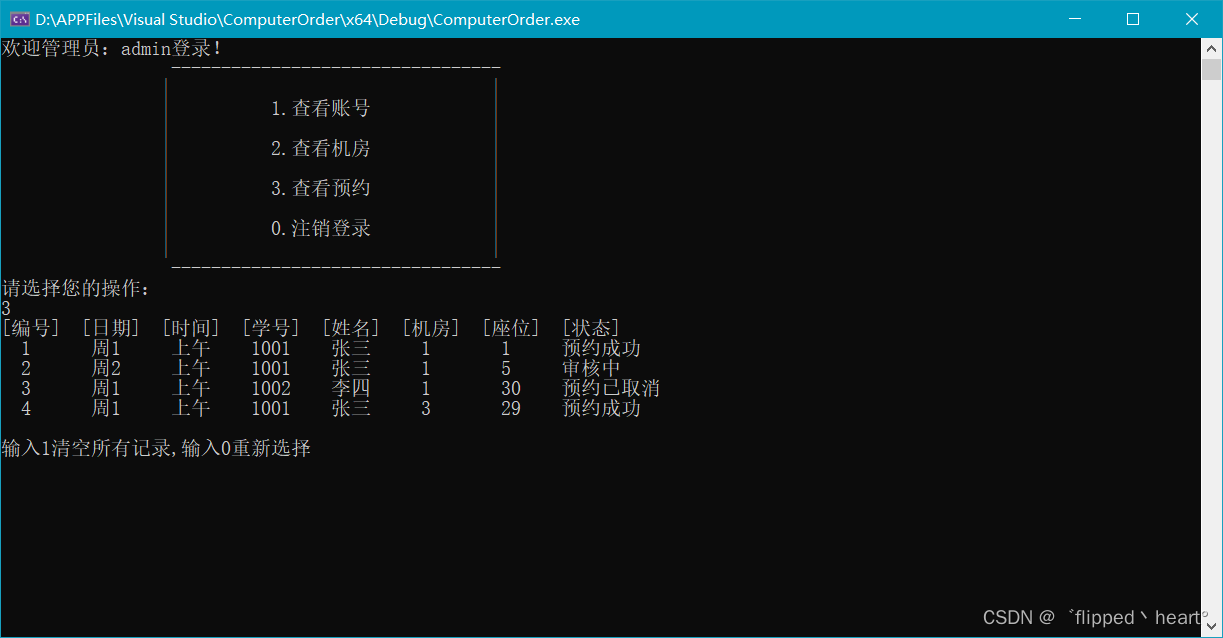
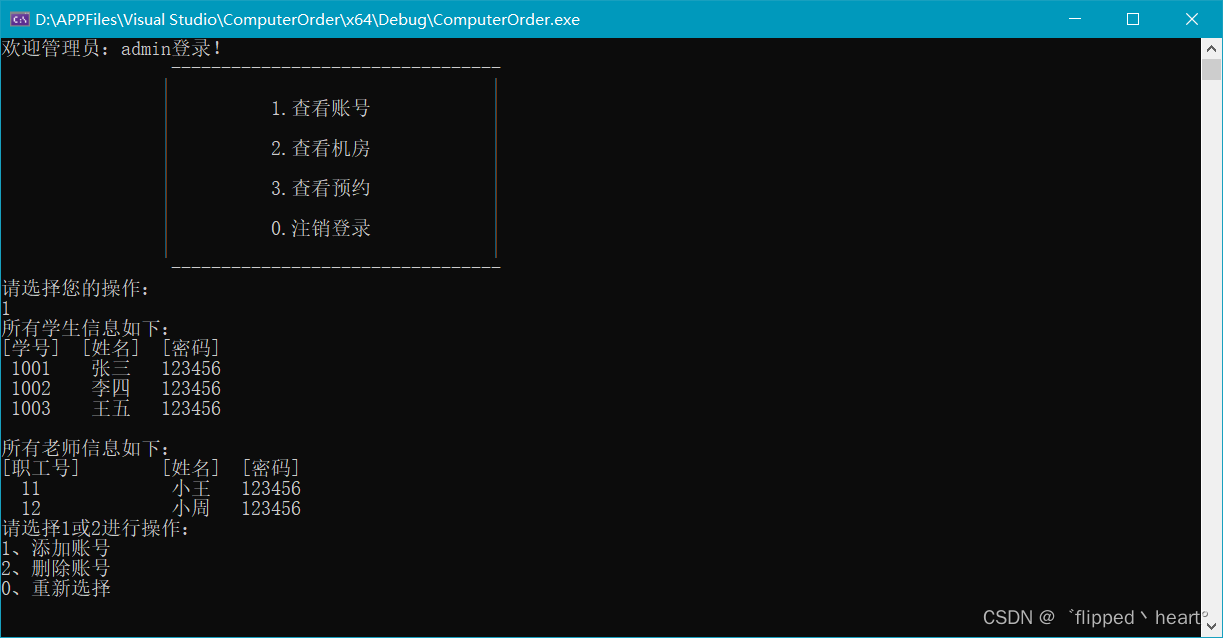
class Manager: public Identify
{
public:
Manager();
Manager(const string& name, const string& pwd);
virtual void openMenu();
void initVector();
void addPerson();
bool checkRepeat(const int& id, const int& type) const;
void showPerson();
void cleanPerson(const string& fileName) const;
void updatePerson(const string& fileName, const int& type) const;
void delPerson();
void showOrder() const;
void showComputer();
void alterRoom(const int& n);
void updateComputer() const;
private:
static vector<Student> vStu;
static vector<Teacher> vTea;
};
vector<Student> Manager::vStu;
vector<Teacher> Manager::vTea;
Manager::Manager()
{
this->m_Name = "";
this->m_Pwd = "";
}
Manager::Manager(const string& name, const string& pwd)
{
this->m_Name = name;
this->m_Pwd= pwd;
this->initVector();
}
void Manager::openMenu()
{
cout << "欢迎管理员:" << this->m_Name << "登录!" << endl;
cout << "\t\t ---------------------------------\n";
cout << "\t\t| |\n";
cout << "\t\t| 1.查看账号 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 2.查看机房 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 3.查看预约 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 0.注销登录 |\n";
cout << "\t\t| |\n";
cout << "\t\t ---------------------------------\n";
cout << "请选择您的操作: " << endl;
}
void Manager::initVector()
{
this->vStu.clear();
this->vTea.clear();
ifstream ifs;
ifs.open(STUDENT_FILE, ios::in);
if (!ifs.is_open())
{
cout << "文件读取失败" << endl;
return;
}
Student s;
while (ifs >> s.uid && ifs >> s.m_Name && ifs >> s.m_Pwd)
{
this->vStu.push_back(s);
}
ifs.close();
ifs.open(TEACHER_FILE, ios::in);
if (!ifs.is_open())
{
cout << "文件读取失败" << endl;
return;
}
Teacher t;
while (ifs >> t.tid && ifs >> t.m_Name && ifs >> t.m_Pwd)
{
this->vTea.push_back(t);
}
ifs.close();
}
void Manager::cleanPerson(const string& fileName) const
{
ofstream ofs(fileName, ios::trunc);
ofs.close();
cout << "清空成功!" << endl;
system("pause");
system("cls");
}
void Manager::updatePerson(const string& fileName, const int& type) const
{
ofstream ofs;
ofs.open(fileName, ios::out | ios::app);
if (!ofs.is_open())
{
cout << "文件读取失败" << endl;
return;
}
if (type == 1)
{
for (vector<Student>::iterator it = this->vStu.begin(); it != this->vStu.end(); it++)
{
ofs << it->uid << " " << it->m_Name << " " << it->m_Pwd << " " << endl;
}
}
else
{
for (vector<Teacher>::iterator it = this->vTea.begin(); it != this->vTea.end(); it++)
{
ofs << it->tid << " " << it->m_Name << " " << it->m_Pwd << " " << endl;
}
}
cout << "文件更新成功" << endl;
ofs.close();
}
void Manager::showPerson()
{
cout << "所有学生信息如下:" << endl;
cout << "[学号]\t[姓名]\t[密码]"<<endl;
for (vector<Student>::iterator i = this->vStu.begin(); i != this->vStu.end(); i++)
{
cout <<" " << i->uid << "\t " << i->m_Name << "\t" << i->m_Pwd << endl;
}
cout << "\n所有老师信息如下:" << endl;
cout << "[职工号]\t[姓名]\t[密码]" << endl;
for (vector<Teacher>::iterator i = this->vTea.begin(); i != this->vTea.end(); i++)
{
cout <<" "<< i->tid << "\t\t " << i->m_Name << "\t" << i->m_Pwd << endl;
}
cout << "请选择1或2进行操作:" << endl;
cout << "1、添加账号" << endl;
cout << "2、删除账号" << endl;
cout << "0、重新选择" << endl;
int select = 0;
cin >> select;
if (select == 1)
{
this->addPerson();
}
else if (select == 2)
{
this->delPerson();
}
else
{
system("pause");
system("cls");
}
}
void Manager::addPerson()
{
cout << "请输入添加账号类型" << endl;
cout << "1、学生" << endl;
cout << "2、老师" << endl;
string fileName;
string tip;
string errorTip;
int select = 0;
cin >> select;
if (select == 1)
{
fileName = STUDENT_FILE;
tip = "请输入学号:";
errorTip = "学号重复,请重新输入";
}
else
{
fileName = TEACHER_FILE;
tip = "请输入职工编号:";
errorTip = "职工号重复,请重新输入";
}
ofstream ofs;
ofs.open(fileName, ios::out | ios::app);
int id;
string name;
string pwd;
cout << tip << endl;
while (true)
{
cin >> id;
if (this->checkRepeat(id, select))
{
cout << errorTip << endl;
}
else
{
break;
}
}
cout << "请输入姓名: " << endl;
cin >> name;
cout << "请输入密码: " << endl;
cin >> pwd;
ofs << id << " " << name << " " << pwd << " " << endl;
cout << "添加成功" << endl;
system("pause");
system("cls");
ofs.close();
this->initVector();
}
bool Manager::checkRepeat(const int& id, const int& type) const
{
if (type == 1)
{
for (vector<Student>::iterator it = this->vStu.begin(); it != this->vStu.end(); it++)
{
if (id == it->uid)
{
return true;
}
}
}
else
{
for (vector<Teacher>::iterator it = this->vTea.begin(); it != this->vTea.end(); it++)
{
if (id == it->tid)
{
return true;
}
}
}
return false;
}
void Manager::delPerson()
{
cout << "请输入删除账号类型" << endl;
cout << "1、学生" << endl;
cout << "2、老师" << endl;
string fileName;
string tip;
string errorTip;
int type = 0;
cin >> type;
if (type == 1)
{
fileName = STUDENT_FILE;
tip = "请输入学号:";
errorTip = "学号错误,请重新输入";
}
else
{
fileName = TEACHER_FILE;
tip = "请输入职工编号:";
errorTip = "职工号错误,请重新输入";
}
int id;
cout << tip << endl;
bool flag = false;
while (!flag)
{
cin >> id;
if (type == 1)
{
for (vector<Student>::iterator it = this->vStu.begin(); it != this->vStu.end(); it++)
{
if (id == it->uid)
{
this->vStu.erase(it);
flag = true;
break;
}
}
cout << errorTip << endl;
}
else
{
for (vector<Teacher>::iterator it = this->vTea.begin(); it != this->vTea.end(); it++)
{
if (id == it->tid)
{
this->vTea.erase(it);
flag = true;
break;
}
}
cout << errorTip << endl;
}
}
cout << "删除成功" << endl;
this->cleanPerson(fileName);
this->updatePerson(fileName, type);
system("pause");
system("cls");
}
void Manager::updateComputer() const
{
ofstream ofs;
ofs.open(COMPUTER_FILE, ios::out | ios::trunc);
if (!ofs.is_open())
{
cout << "文件读取失败" << endl;
return;
}
for (vector<ComputerRoom>::iterator it = Identity::vCom.begin(); it != Identity::vCom.end(); it++)
{
ofs << it->m_ComId << " " << it->m_MaxNum << " " << it->row << " "<<it->col << " "<<it->layout << endl;
}
ofs.close();
}
void Manager::showComputer()
{
cout << "机房信息如下:" << endl;
cout << "[编号]\t[容量]\t[行数]\t[列数]" << endl;
int m = 0;
for (vector<ComputerRoom>::iterator it = Identity::vCom.begin(); m < ComputerRoom::num; it++, m++)
{
cout <<" "<< it->m_ComId << "\t " << it->m_MaxNum << "\t " << it->row<<"\t " << it->col <<endl;
}
cout << "输入编号1-" << ComputerRoom::num << ",可以进行机房的布局修改" << endl;
cout << "输入0,重新选择" << endl;
int n = 0;
cin >> n;
if (n)
{
Identity::vCom[n - 1].printLayout();
this->alterRoom(n);
}
else
{
system("pause");
system("cls");
}
}
void Manager::alterRoom(const int& n)
{
cout << "请输入房间容量,行数,列数" << endl;
int new_size, new_col, new_row;
cin >> new_size >> new_row >> new_col;
if (new_size <= new_row * new_col && new_size > 0 && new_row > 0 && new_col > 0)
{
for (int i = 0; i < ComputerRoom::num * 10; i++)
{
if (i % ComputerRoom::num == n - 1)
{
Identity::vCom[i].row = new_row;
Identity::vCom[i].col = new_col;
Identity::vCom[i].m_MaxNum = new_size;
string t(new_size, '0');
Identity::vCom[i].layout.assign(t);
}
}
cout << "修改成功,返回上一级界面" << endl;
this->updateComputer();
this->showComputer();
}
else
{
cout << "修改失败,返回上一级界面" << endl;
system("pause");
system("cls");
}
}
void Manager::showOrder() const
{
if (Identity::of.m_Size == 0)
{
cout << "无预约记录" << endl;
system("pause");
system("cls");
return;
}
cout << "[编号]\t[日期]\t[时间]\t[学号]\t[姓名]\t[机房]\t[座位]\t[状态]" << endl;
for (int i = 0; i < Identity::of.m_Size; i++)
{
cout <<" "<< i + 1;
cout << "\t 周" << Identity::of.m_orderData[i]["date"];
cout << "\t " << (Identity::of.m_orderData[i]["interval"] == "1" ? "上午" : "下午");
cout << "\t " << Identity::of.m_orderData[i]["stuId"];
cout << "\t "<< Identity::of.m_orderData[i]["stuName"];
cout << "\t " << Identity::of.m_orderData[i]["roomId"];
cout << "\t " << Identity::of.m_orderData[i]["deskId"];
string status = "\t";
if (Identity::of.m_orderData[i]["status"] == "1")
{
status += "审核中";
}
else if (Identity::of.m_orderData[i]["status"] == "2")
{
status += "预约成功";
}
else if (Identity::of.m_orderData[i]["status"] == "0")
{
status += "预约失败";
}
else
{
status += "预约已取消";
}
cout << status << endl;
}
cout << "\n输入1清空所有记录,输入0重新选择" << endl;
int f = 1;
cin >> f;
if (f)
{
cout << "\n操作完成!" << endl;
Identity::of.m_orderData.clear();
Identity::of.m_Size = 0;
Identity::of.updateOrder();
for (vector<ComputerRoom>::iterator it = Identity::vCom.begin(); it != Identity::vCom.end(); it++)
{
string t(it->m_MaxNum, '0');
it->layout.assign(t);
}
this->updateComputer();
}
system("pause");
system("cls");
}
重点问题
- 用户信息、机房信息和预约信息以txt形式保存于本地。
- 管理员需要在进入系统后初始化教师和学生容器。
全局模块
#define ADMIN_FILE "admin.txt"
#define STUDENT_FILE "student.txt"
#define TEACHER_FILE "teacher.txt"
#define COMPUTER_FILE "computerRoom.txt"
#define ORDER_FILE "order.txt"
登录模块
- 选择用户类型后进入到验证信息环节,读取相应的txt文件进行信息比对,成功则进入子菜单。
- 期间需要先初始化基类指针,而后指向不同子类的实例,调用重写后的虚函数。
- 在进入每个子菜单后,需要定义子类指针接管基类指针指向的对象。
- 在进入系统后初始化机房和预约容器。
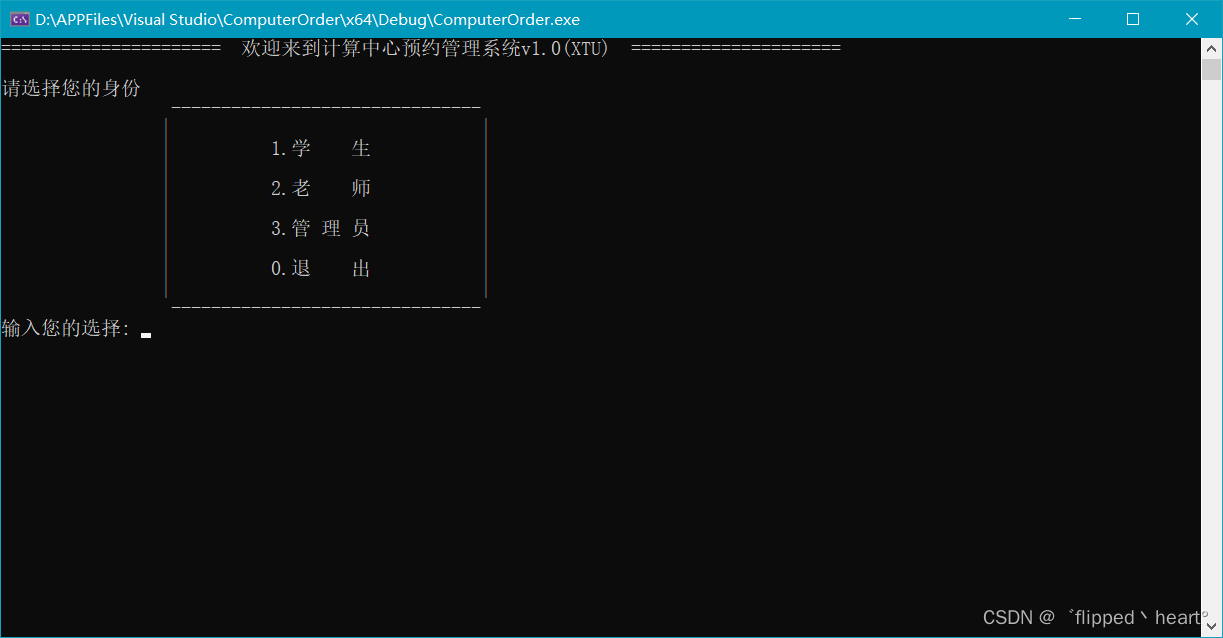
vector<ComputerRoom> Identity::vCom;
int ComputerRoom::num = 4;
OrderFile Identity::of;
void initComputerRoom()
{
ifstream ifs;
ifs.open(COMPUTER_FILE, ios::in);
if (!ifs.is_open())
{
cout << "No File!" << endl;
ifs.close();
return;
}
ComputerRoom com;
Identity::vCom.clear();
while (ifs >> com.m_ComId && ifs >> com.m_MaxNum && ifs >> com.row && ifs >> com.col && ifs >> com.layout)
{
Identity::vCom.push_back(com);
}
ifs.close();
}
void Login(const string& fileName, const int& type)
{
Identity * person = NULL;
ifstream ifs;
ifs.open(fileName, ios::in);
if (!ifs.is_open())
{
cout << "文件不存在" << endl;
ifs.close();
return;
}
int u_id = 0;
string name;
string pwd;
if (type == 1)
{
cout << "请输入你的学号:" << endl;
cin >> u_id;
}
else if (type == 2)
{
cout << "请输入您的职工号: " << endl;
cin >> u_id;
}
cout << "请输入用户名:" << endl;
cin >> name;
cout << "请输入密码:" << endl;
cin >> pwd;
if (type == 1)
{
int fId;
string fName;
string fPwd;
while (ifs >> fId && ifs >> fName && ifs >> fPwd )
{
if (fId == u_id && fName == name && fPwd == pwd)
{
cout << "学生验证登录成功!" << endl;
system("pause");
system("cls");
person = new Student(u_id, name, pwd);
studentMenu(person);
return;
}
}
}
else if (type == 2)
{
int fId;
string fName;
string fPwd;
while (ifs >> fId && ifs >> fName && ifs >> fPwd)
{
if (fId == u_id && fName == name && fPwd == pwd)
{
cout << "教师验证登录成功!" << endl;
system("pause");
system("cls");
person = new Teacher(u_id, name, pwd);
teacherMenu(person);
return;
}
}
}
else if (type == 3)
{
string fName;
string fPwd;
while (ifs >> fName && ifs >> fPwd)
{
if (name == fName && pwd == fPwd)
{
cout << "管理员验证登录成功!" << endl;
system("pause");
system("cls");
person = new Manager(name, pwd);
managerMenu(person);
return;
}
}
}
cout << "验证登录失败!" << endl;
system("pause");
system("cls");
return;
}
void studentMenu(Identify *student)
{
while(true)
{
student->openMenu();
Student *p= (Student *)student;
int select=0;
cin>>select;
if(select == 1)
{
p->applyOrder();
}
else if(select == 2)
{
p->showOrder();
}
else
{
delete student;
cout << "注销成功" << endl;
system("pause");
system("cls");
return;
}
}
}
void teacherMenu(Identify *teacher)
{
while(true)
{
teacher->openMenu();
Teacher *p= (Teacher *)teacher;
int select;
cin>>select;
if(select == 1)
{
p->showOrder();
}
else
{
delete teacher;
cout << "注销成功" << endl;
system("pause");
system("cls");
return;
}
}
}
void managerMenu(Identify *manager)
{
while(true)
{
manager->openMenu();
Manager *p= (Manager *)manager;
int select;
cin>>select;
if(select == 1)
{
p->showPerson();
}
else if(select == 2)
{
p->showComputer()
}
else if(select == 3)
{
p->showOrder()
}
else
{
delete manager;
cout << "注销成功" << endl;
system("pause");
system("cls");
return;
}
}
}
int main()
{
int select = 0;
while (true)
{
cout << "====================== 欢迎来到计算中心预约管理系统v1.0(XTU) =====================" << endl;
cout << endl << "请选择您的身份" << endl;
cout << "\t\t -------------------------------\n";
cout << "\t\t| |\n";
cout << "\t\t| 1.学 生 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 2.老 师 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 3.管 理 员 |\n";
cout << "\t\t| |\n";
cout << "\t\t| 0.退 出 |\n";
cout << "\t\t| |\n";
cout << "\t\t -------------------------------\n";
cout << "输入您的选择: ";
cin >> select;
initComputerRoom();
switch (select)
{
case 1:
Login(STUDENT_FILE, 1);
break;
case 2:
Login(TEACHER_FILE, 2);
break;
case 3:
Login(ADMIN_FILE, 3);
break;
case 0:
cout << "欢迎下一次使用" << endl;
system("pause");
return 0;
break;
default:
cout << "输入有误,请重新选择!" << endl;
system("pause");
system("cls");
break;
}
}
system("pause");
return 0;
}
代码文件