中英对照版,本人只对一些关键部分进行翻译
英文原版网址 https://www.odoo.com/documentation/8.0/howtos/web.html
This guide is about creating modules for Odoo's web client.
该指南是创建一个web客户端的模块指南
To create websites with Odoo, see Building a Website; to add business capabilities or extend existing business systems of Odoo, see Building a Module.
Warning
This guide assumes knowledge of:
- Javascript basics and good practices
- jQuery
- Underscore.js
It also requires an installed Odoo, and Git.
A Simple Module(一个简单的模块)
Let's start with a simple Odoo module holding basic web component configuration and letting us test the web framework.
我们开始创建一个简单的odoo模块,包括了基础的web组件设置并且我们会对其web框架进行测试
The example module is available online and can be downloaded using the following command:
这个例子的源代码我们可以从网上得到并且下载,你可以通过以下的git命令获取
$ git clone http://github.com/odoo/petstore
This will create a petstore
folder wherever you executed the command. You then need to add that folder to Odoo's addons path
, create a new database and install the oepetstore
module.
运行改命令后你会在你运行指令的地方得到一个叫petstore的文件夹,你需要将该文件夹放置到你的odoo脚本代码的addons 文件夹中,并安装该模块
If you browse the petstore
folder, you should see the following content:
如果你查看petstore文件夹可以看到以下结构
oepetstore
|-- images
| |-- alligator.jpg
| |-- ball.jpg
| |-- crazy_circle.jpg
| |-- fish.jpg
| `-- mice.jpg
|-- __init__.py
|-- oepetstore.message_of_the_day.csv
|-- __openerp__.py
|-- petstore_data.xml
|-- petstore.py
|-- petstore.xml
`-- static
`-- src
|-- css
| `-- petstore.css
|-- js
| `-- petstore.js
`-- xml
`-- petstore.xml
The module already holds various server customizations. We'll come back to these later, for now let's focus on the web-related content, in the static
folder.
该模块已经包含了需要的多种服务器端定义,我们回头再了解,现在我们主要关注一下与web相关的内容,主要在static文件中
Files used in the "web" side of an Odoo module must be placed in a static
folder so they are available to a web browser, files outside that folder can not be fetched by browsers. The src/css
, src/js
and src/xml
sub-folders are conventional and not strictly necessary.
在Odoo模块中关于“web”的的文件必须放在一个叫static的文件夹,以便它们可用于Web浏览器,如果放到其他文件中就无法通过浏览器获取。在src/ CSS,SRC/ JS和src/ XML子文件是常规的存在的,但也不是绝对必要的。
- currently empty, will hold the CSS for pet store content
- 现在是空的,我们放置css的相关内容
- Mostly empty, will hold QWeb templates
- 基本空,我们放置qweb模板
-
The most important (and interesting) part, contains the logic of the application (or at least its web-browser side) as javascript. It should currently look like:
oepetstore/static/css/petstore.css
oepetstore/static/xml/petstore.xml
oepetstore/static/js/petstore.js
openerp.oepetstore = function(instance, local) { var _t = instance.web._t, _lt = instance.web._lt; var QWeb = instance.web.qweb; local.HomePage = instance.Widget.extend({ start: function() { console.log("pet store home page loaded"); }, }); instance.web.client_actions.add( 'petstore.homepage', 'instance.oepetstore.HomePage'); }Which only prints a small message in the browser's console.
这段代码运行的效果只是在浏览器端的控制台打印了一段小信息
Warning(注意)
All JavaScript files are concatenated and minified to improve application load time.
注意,odoo中所有的js文件都会使用级联和压缩的方式来形成一个js文件来提高应用加载的速度
One of the drawback is debugging becomes more difficult as individual files disappear and the code is made significantly less readable. It is possible to disable this process by enabling the "developer mode": log into your Odoo instance (user admin password admin by default) open the user menu (in the top-right corner of the Odoo screen) and select About Odoo then Activate the developer mode:
简单翻译下意思:因为js文件的合并和压缩再加载到你前端的原因,为了调试js代码,你必须打开odoo的开发者模式,此时你加载的将不再是压缩和混合后的js代码,你就可以进行调试
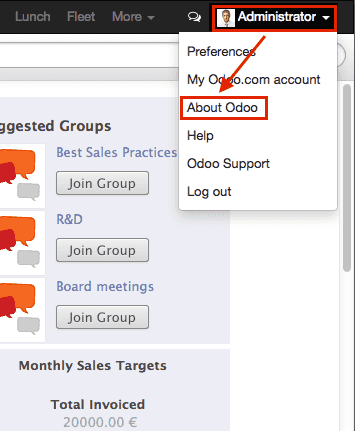
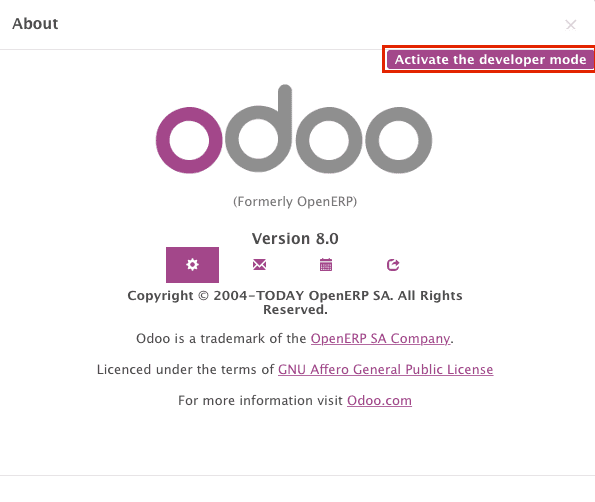
This will reload the web client with optimizations disabled, making development and debugging significantly more comfortable.
选择开发者模式会对页面再次进行加载
Odoo JavaScript Module
Javascript doesn't have built-in modules. As a result variables defined in different files are all mashed together and may conflict. This has given rise to various module patterns used to build clean namespaces and limit risks of naming conflicts.
JavaScript没有内置模块。其结果是,在不同的文件中定义的变量会混淆在一起,并可能会发生冲突。对于那些遵循保持清洁命名空间,限制命名冲突的模块编码方式来说,这种混淆将会产生极大的风险。
The Odoo framework uses one such pattern to define modules within web addons, in order to both namespace code and correctly order its loading.
在odoo框架中,对于web插件我们遵循一个定义模块的模式,该模式将会让我们很好地命名我们的代码,并且正确加载
oepetstore/static/js/petstore.js
contains a module declaration:
在oepetstore/static/js/petstore.js中我们这样定义模块
openerp.oepetstore = function(instance, local) { local.xxx = ...; }
openerp
variable. The function's name must be the same as the addon (in this case
oepetstore
) so the framework can find it, and automatically initialize it.
在odoo的web模块中,模块将会定义为全局变量openerp下的一个函数,这个函数的名字必须和addon下的模块名字意义(例如这里的oepetstore),这样odoo才能找到,并进行自动的初始化。
When the web client loads your module it will call the root function and provide two parameters:
但浏览器加载你的模块时,它会调用你的根函数(上面的那个函数),并为你提供两个变量:
- the first parameter is the current instance of the Odoo web client, it gives access to various capabilities defined by the Odoo (translations, network services) as well as objects defined by the core or by other modules.
- 第一个变量为odoo web客户端的当前实例,通过该变量,你可以访问到odoo定义的一些功能(如翻译,网络服务)。同样,你也可以通过该变量访问到其他addone模块和odoo核心定义的对象。
- the second parameter is your own local namespace automatically created by the web client. Objects and variables which should be accessible from outside your module (either because the Odoo web client needs to call them or because others may want to customize them) should be set inside that namespace.
未完待续。。。。