860. 柠檬水找零 - 力扣(LeetCode)
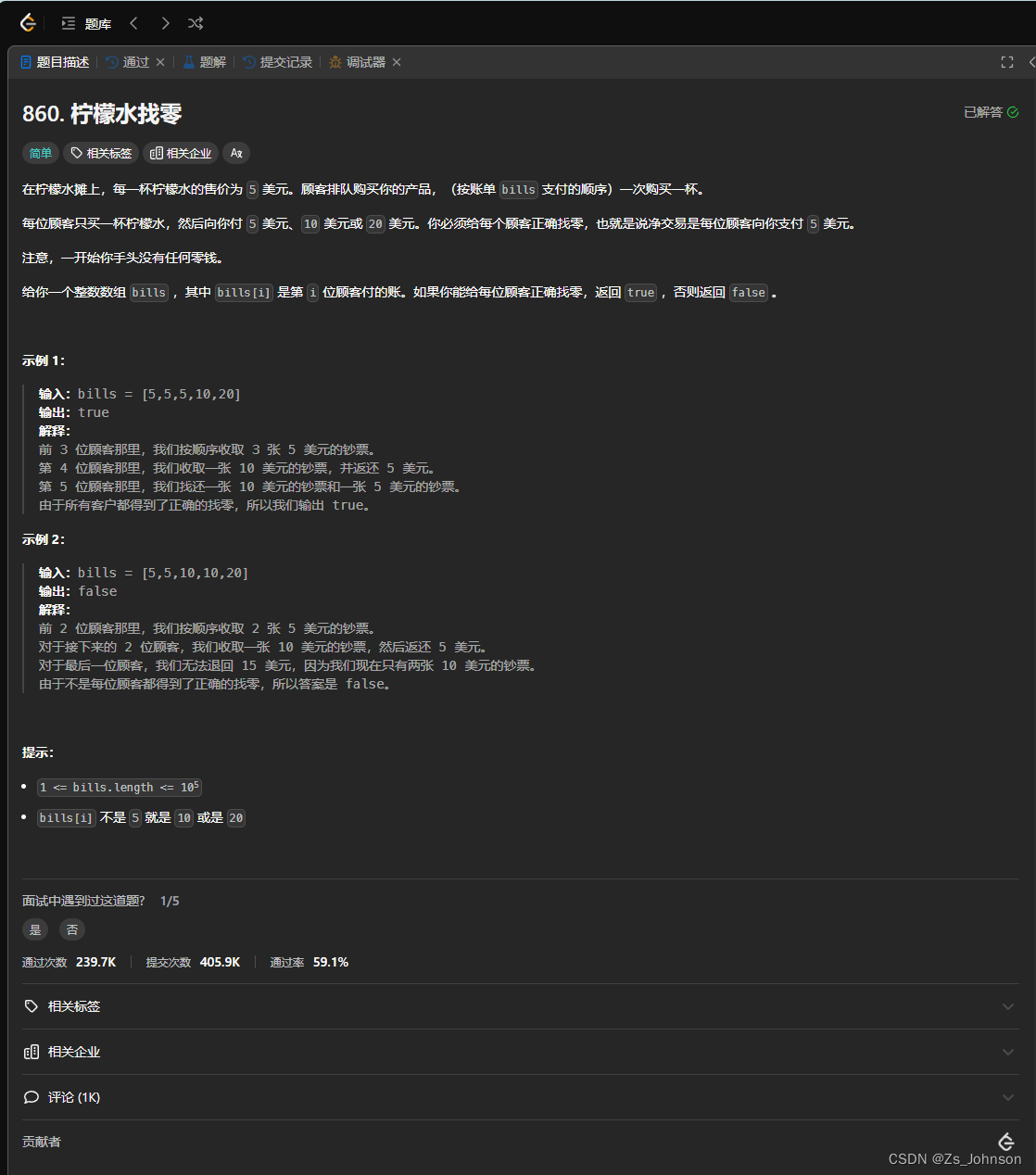
class Solution {
public boolean lemonadeChange(int[] bills) {
int fiveCount = 0;
int tenCount = 0;
for(int i=0;i<bills.length;i++){
if(bills[i] == 5){
fiveCount++;
}else if(bills[i] == 10){
fiveCount--;
tenCount++;
}else{
if(tenCount>0){
tenCount--;
fiveCount--;
}else{
fiveCount-=3;
}
}
if(fiveCount<0 || tenCount<0){
return false;
}
}
return true;
}
}
406. 根据身高重建队列 - 力扣(LeetCode)
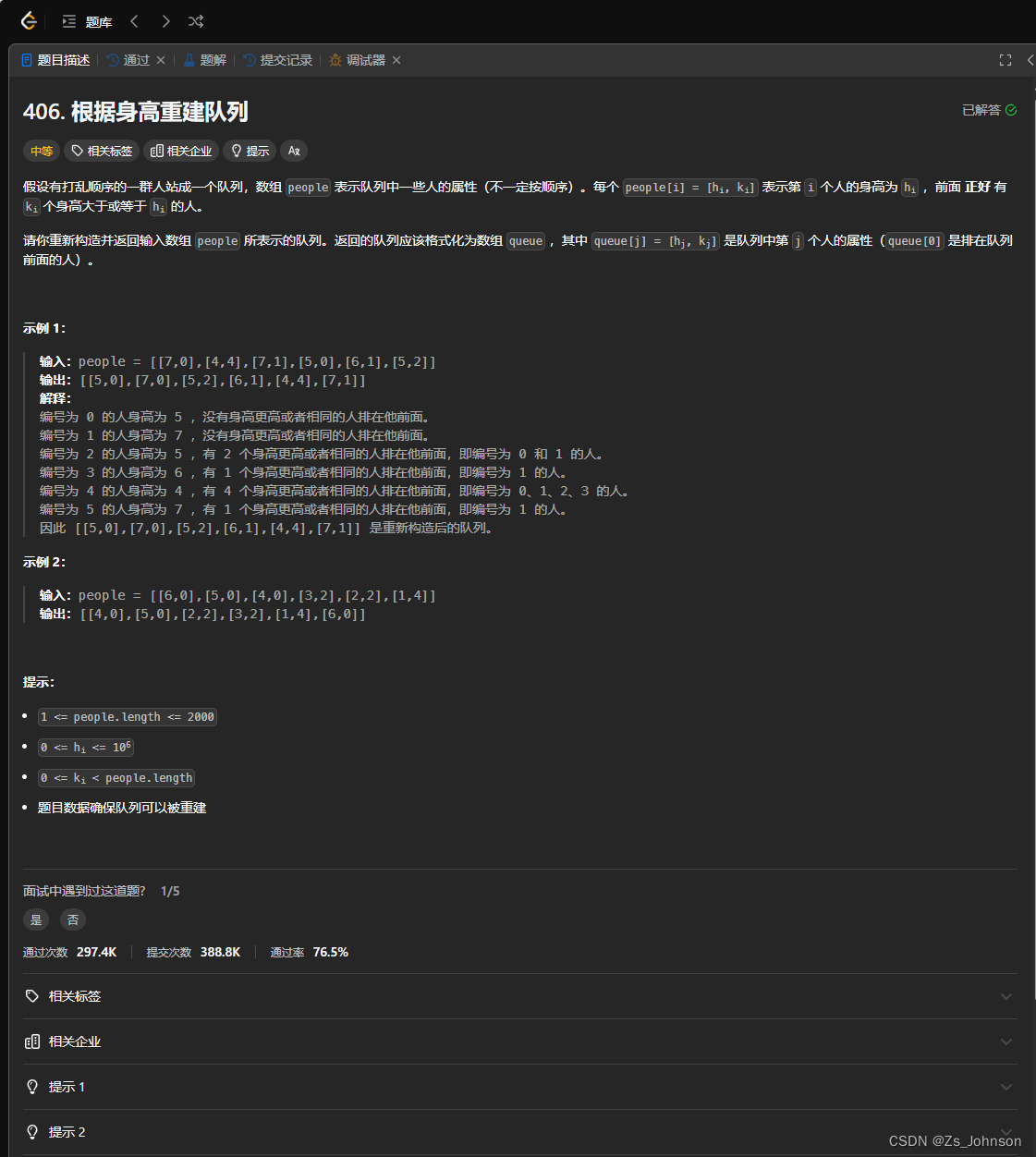
class Solution {
public int[][] reconstructQueue(int[][] people) {
//按身高进行排序
Arrays.sort(people, (o1, o2) -> {
if(o1[0] != o2[0]){
return o2[0] - o1[0];
}else{
return o1[1] - o2[1];
}
});
ArrayList<int[]> result = new ArrayList<>();
for(int i=0;i<people.length;i++){
result.add(people[i][1],people[i]);
}
return result.toArray(new int[people.length][2]);
}
}
738. 单调递增的数字 - 力扣(LeetCode)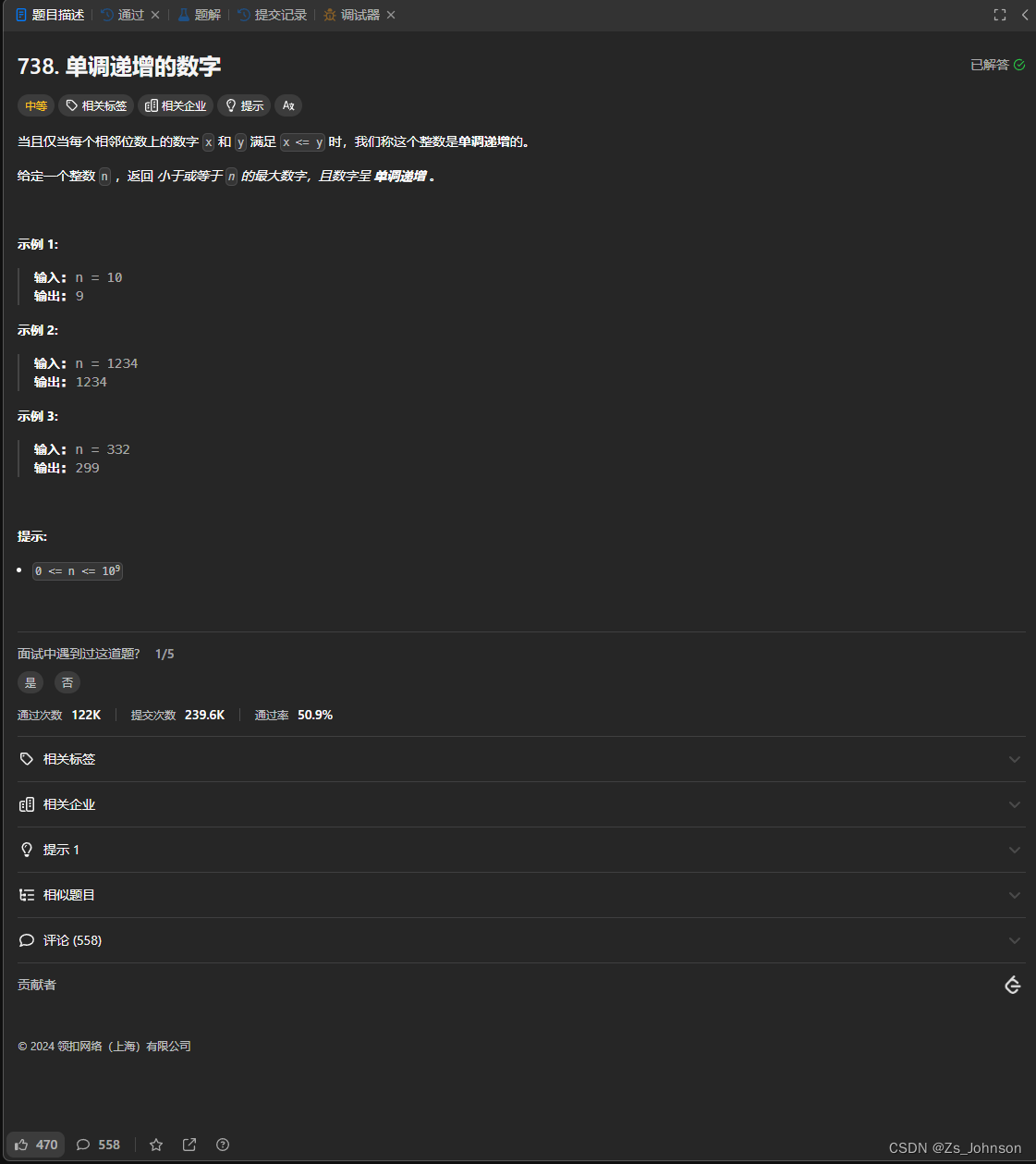
class Solution {
public int monotoneIncreasingDigits(int n) {
if(n<10){
return n;
}
char[] chars = (n + "").toCharArray();
for(int i=chars.length-1;i>=1;i--){
if(chars[i]<chars[i-1]){
for(int j=i;j<chars.length;j++){
chars[j] = '9';
}
chars[i-1] = (char)(chars[i-1] - 1);
}
}
return Integer.valueOf(new String(chars));
}
}