<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>4.3.9.RELEASE</version>
</dependency>
public class MyController {
public MyService2 getMyService2() {
return myService2;
}
public void setMyService2(MyService2 myService2) {
this.myService2 = myService2;
}
private MyService myService;
private MyService2 myService2;
public MyController(MyService myService) {
this.myService = myService;
}
public String doSomething(int id, String name) {
System.out.println("id:" + id + "name:" + name);
doTry();
return "id>" + id + "name<" + name;
}
public void doTry() {
System.out.println(System.currentTimeMillis());
myService.doService();
myService2.doService();
}
}
-------------------------------------------------
public class MyService {
public void doService() {
System.out.println("service....");
}
}
------------------------------------------------------
public class MyService2 {
public void doService() {
System.out.println("service2....");
}
}
---------------------------------------------
public class MyController {
public MyService2 getMyService2() {
return myService2;
}
public void setMyService2(MyService2 myService2) {
this.myService2 = myService2;
}
private MyService myService;
private MyService2 myService2;
public MyController(MyService myService) {
this.myService = myService;
}
public String doSomething(int id, String name) {
System.out.println("id:" + id + "name:" + name);
doTry();
return "id>" + id + "name<" + name;
}
public void doTry() {
System.out.println(System.currentTimeMillis());
myService.doService();
myService2.doService();
}
}
-------------------------------------------
public class UserTO {
private String id;
private String name;
private int age;
private String gender;
}
<?xml version="1.0" encoding="UTF-8"?>
<beans default-lazy-init="true"
xmlns="http://www.springframework.org/schema/beans"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:util="http://www.springframework.org/schema/util"
xmlns:jpa="http://www.springframework.org/schema/data/jpa"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.3.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-4.3.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-4.3.xsd
http://www.springframework.org/schema/util
http://www.springframework.org/schema/util/spring-util-4.3.xsd
http://www.springframework.org/schema/data/jpa
http://www.springframework.org/schema/data/jpa/spring-jpa-1.3.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<!-- 配置bean -->
<bean id = "myService" class="com.feifan.service.MyService"></bean>
<bean id = "myService2" class="com.feifan.service.MyService2"></bean>
<bean id = "userTO" class="com.feifan.compent.UserTO"></bean>
<bean id = "myController" class="com.feifan.controller.MyController">
<!-- 构造注入参数 -->
<constructor-arg name="myService" ref="myService"></constructor-arg>
<!-- set 注入参数 -->
<property name="myService2" ref="myService2"></property>
</bean>
</beans>
public static void main(String[] args) {
ClassPathXmlApplicationContext cac = new ClassPathXmlApplicationContext("classpath:configs/spring-config.xml");
System.out.println(cac.getDisplayName());
System.out.println(cac.getBeanDefinitionCount());
((MyService)cac.getBean("myService")).doService();
System.out.println(((MyController)(cac.getBean("myController"))).doSomething(9, "f"));
System.out.println(cac.getBean("userTO"));
System.out.println(cac.getId());
cac.close();
}
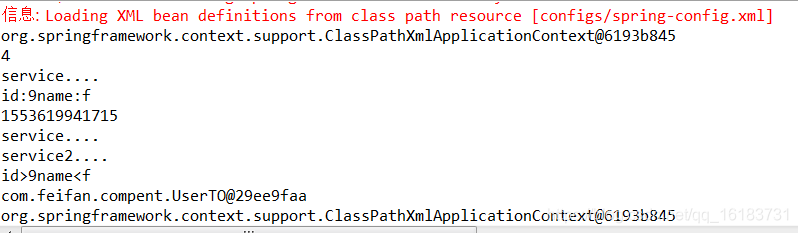