generator mysql反射生成(两种实现)
项目目录结构
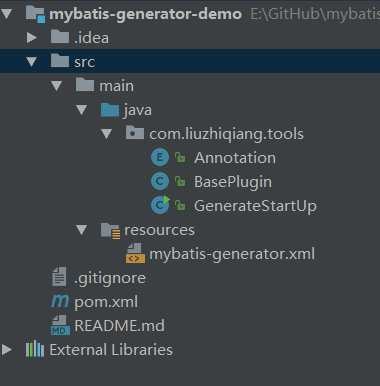
mybatis-generator文件
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE generatorConfiguration
PUBLIC "-//mybatis.org//DTD MyBatis Generator Configuration 1.0//EN"
"http://mybatis.org/dtd/mybatis-generator-config_1_0.dtd">
<generatorConfiguration>
<context id="DB2Tables" targetRuntime="MyBatis3">
<!-- <plugin>元素用来定义一个插件。插件用于扩展或修改通过MyBatis Generator (MBG)代码生成器生成的代码。 -->
<plugin type="com.liuzhiqiang.tools.BasePlugin" />
<commentGenerator>
<property name="javaFileEncoding" value="UTF-8"/>
<!-- 是否去除自动生成的注释 true:是 : false:否 -->
<property name="suppressAllComments" value="true" />
<property name="suppressDate" value="true" />
</commentGenerator>
<!--数据库链接地址账号密码-->
<jdbcConnection
driverClass="com.mysql.jdbc.Driver"
connectionURL="jdbc:mysql://127.0.0.1:3306/springcloud"
userId="数据库账号"
password="数据库密码"
/>
<!--生成Model实体类存放位置-->
<javaModelGenerator targetPackage="com.liuzhiqiang.domain.sysRole" targetProject="src/main/java">
<property name="enableSubPackages" value="true"/>
<property name="trimStrings" value="true"/>
</javaModelGenerator>
<!--生成映射文件xml存放位置-->
<sqlMapGenerator targetPackage="mappers.sysRole" targetProject="src/main/resources">
<property name="enableSubPackages" value="true"/>
</sqlMapGenerator>
<!--生成mapper接口存放位置-->
<!-- 客户端代码,生成易于使用的针对Model对象和XML配置文件 的代码
type="ANNOTATEDMAPPER",生成Java Model 和基于注解的Mapper对象
type="MIXEDMAPPER",生成基于注解的Java Model 和相应的Mapper对象
type="XMLMAPPER",生成SQLMap XML文件和独立的Mapper接口
-->
<javaClientGenerator type="XMLMAPPER" targetPackage="com.liuzhiqiang.mapper.sysRole" targetProject="src/main/java">
<property name="enableSubPackages" value="true"/>
</javaClientGenerator>
<!--生成对应表及类名-->
<table tableName="sys_role"
domainObjectName="SysRole"
enableCountByExample="false"
enableUpdateByExample="false"
enableDeleteByExample="false"
enableSelectByExample="false"
selectByExampleQueryId="false">
</table>
</context>
</generatorConfiguration>
plugin元素用来定义一个插件。插件用于扩展或修改通过MyBatis Generator (MBG)代码生成器生成的代码
mybatis-generator-core 提供了常用的插件 PluginAdapter 要定制 生成的实体类 接口 及sql 我们只要继承PluginAdapter 并重写相对应的内部方法就好了
简单介绍本文中用到的几个方法 及 参数说明
参数
topLevelClass 对应生成的类
Interface 对应生成的接口
Field 对应生成的属性
Method 对应生成的方法
参数对象的常用方法
addAnnotation 增加注解
addImportedTypes 增加导入的包
addJavaDocLine 增加注释
setType 修改属性类型
introspectedTable 数据库表映射信息
introspectedColumn 数据库字段映射信息
PluginAdapter 提供的接口
modelBaseRecordClassGenerated 修改实体类在此方法
clientGenerated 修改生成的mapper接口
modelFieldGenerated 修改生成的属性
modelSetterMethodGenerated 修改set方法 false不生成
modelGetterMethodGenerated 修改get方法 false不生成
clientDeleteByPrimaryKeyMethodGenerated 对应mapper接口方法 DeleteByPrimaryKey方法
client*****MethodGenerated 对应mapper接口内的各个方法
新建BasePlugin类 并继承 PluginAdapter。新建枚举类Annotation
本类中实现的业务主要有 实体类增加lombok 及 swagger2注解, 日期类型格式化注解,tinyint 转 Integer类型,增加类注释,接口注释,属性注释,方法注释,mapper接口增加@Mapper注解
package com.liuzhiqiang.tools;
/**
* 开发公司:青岛海豚数据技术有限公司
* 版权:青岛海豚数据技术有限公司
* <p>
* Annotation
*
* @author 刘志强
* @created Create Time: 2019/1/16
*/
public enum Annotation {
DATA("@Data", "lombok.Data"),
Mapper("@Mapper", "org.apache.ibatis.annotations.Mapper"),
Param("@Param", "org.apache.ibatis.annotations.Param"),
ApiModel("@ApiModel", "io.swagger.annotations.ApiModel"),
ApiModelProperty("@ApiModelProperty", "io.swagger.annotations.ApiModelProperty"),
JsonFormat("@JsonFormat", "com.fasterxml.jackson.annotation.JsonFormat");
private String annotation;
private String clazz;
Annotation(String annotation, String clazz) {
this.annotation = annotation;
this.clazz = clazz;
}
public String getAnnotation() {
return annotation;
}
public String getClazz() {
return clazz;
}
}
package com.liuzhiqiang.tools;
import org.apache.commons.lang.StringUtils;
import org.mybatis.generator.api.IntrospectedColumn;
import org.mybatis.generator.api.IntrospectedTable;
import org.mybatis.generator.api.PluginAdapter;
import org.mybatis.generator.api.dom.java.*;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* 开发公司:青岛海豚数据技术有限公司
* 版权:青岛海豚数据技术有限公司
* <p>
* BasePlugin
*
* @author 刘志强
* @created Create Time: 2019/1/16
*/
public class BasePlugin extends PluginAdapter {
public boolean validate(List<String> list) {
return true;
}
/**
* 修改实体类
* @param topLevelClass
* @param introspectedTable
* @return
*/
public boolean modelBaseRecordClassGenerated(TopLevelClass topLevelClass, IntrospectedTable introspectedTable) {
classAnnotation(topLevelClass,null);
Set<FullyQualifiedJavaType> set = new HashSet<FullyQualifiedJavaType>();
set.add(new FullyQualifiedJavaType(Annotation.ApiModel.getClazz()));
set.add(new FullyQualifiedJavaType(Annotation.DATA.getClazz()));
topLevelClass.addImportedTypes(set);
topLevelClass.addAnnotation(Annotation.ApiModel.getAnnotation() + "(value=\"" + topLevelClass.getType() + "\",description=\"" + introspectedTable.getRemarks() + "\")");
topLevelClass.addAnnotation(Annotation.DATA.getAnnotation() + "()");
return super.modelBaseRecordClassGenerated(topLevelClass, introspectedTable);
}
/**
* 修改mapper接口
* @param interfaze
* @param topLevelClass
* @param introspectedTable
* @return
*/
public boolean clientGenerated(Interface interfaze, TopLevelClass topLevelClass, IntrospectedTable introspectedTable) {
interfazeAnnotation(interfaze, null);
Set<FullyQualifiedJavaType> set = new HashSet<FullyQualifiedJavaType>();
set.add(new FullyQualifiedJavaType(Annotation.Mapper.getClazz()));
interfaze.addImportedTypes(set);
interfaze.addAnnotation(Annotation.Mapper.getAnnotation() + "()");
return super.clientGenerated(interfaze,topLevelClass,introspectedTable);
}
/**
* 实体类字段
* @param field
* @param topLevelClass
* @param introspectedColumn
* @param introspectedTable
* @param modelClassType
* @return
*/
@Override
public boolean modelFieldGe