【LeetCode】 115. Distinct Subsequences 不同的子序列(Hard)(JAVA)
题目地址: https://leetcode.com/problems/distinct-subsequences/
题目描述:
Given two strings s and t, return the number of distinct subsequences of s which equals t.
A string’s subsequence is a new string formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., “ACE” is a subsequence of “ABCDE” while “AEC” is not).
It’s guaranteed the answer fits on a 32-bit signed integer.
Example 1:
Input: S = "rabbbit", T = "rabbit"
Output: 3
Explanation:
As shown below, there are 3 ways you can generate "rabbit" from S.
(The caret symbol ^ means the chosen letters)
rabbbit
^^^^ ^^
rabbbit
^^ ^^^^
rabbbit
^^^ ^^^
Example 2:
Input: S = "babgbag", T = "bag"
Output: 5
Explanation:
As shown below, there are 5 ways you can generate "bag" from S.
(The caret symbol ^ means the chosen letters)
babgbag
^^ ^
babgbag
^^ ^
babgbag
^ ^^
babgbag
^ ^^
babgbag
^^^
题目大意
给定一个字符串 S 和一个字符串 T,计算在 S 的子序列中 T 出现的个数。
一个字符串的一个子序列是指,通过删除一些(也可以不删除)字符且不干扰剩余字符相对位置所组成的新字符串。(例如,“ACE” 是 “ABCDE” 的一个子序列,而 “AEC” 不是)
题目数据保证答案符合 32 位带符号整数范围。
解题方法
- 看到两个字符串 S 和 T ,就应该想到用动态规划的方式
- 找出动态规划的动态函数: dp[s.length() + 1][t.length() + 1] (因为 s 和 t 都可能为空字符串,所以要 +1 用来表示空字符串的情况)
- 如果 j == 0, 表示 t 为空字符串,所以只有一种方式,就是 s 字符串的字符删光
- 如果 i == 0,表示 s 为空字符串,所以如果 t 字符串不为空,就 0 方式
- 如果 s.charAt(i - 1) == t.charAt(j - 1), 表示当前字符相等,有两种方式: 1.s 和 t 字符串各减一个字符;2. 只有 s 减一个字符
- 如果 s.charAt(i - 1) != t.charAt(j - 1), 表示当前字符不相等,只有一种方式: s 减一个字符
class Solution {
public int numDistinct(String s, String t) {
int[][] dp = new int[s.length() + 1][t.length() + 1];
for (int i = 0; i <= s.length(); i++) {
for (int j = 0; j <= t.length(); j++) {
if (j == 0) {
dp[i][j] = 1;
} else if (i == 0) {
dp[i][j] = 0;
} else if (s.charAt(i - 1) == t.charAt(j - 1)) {
dp[i][j] = dp[i - 1][j - 1] + dp[i - 1][j];
} else {
dp[i][j] = dp[i - 1][j];
}
}
}
return dp[s.length()][t.length()];
}
}
执行耗时:6 ms,击败了86.80% 的Java用户
内存消耗:36.9 MB,击败了88.98% 的Java用户
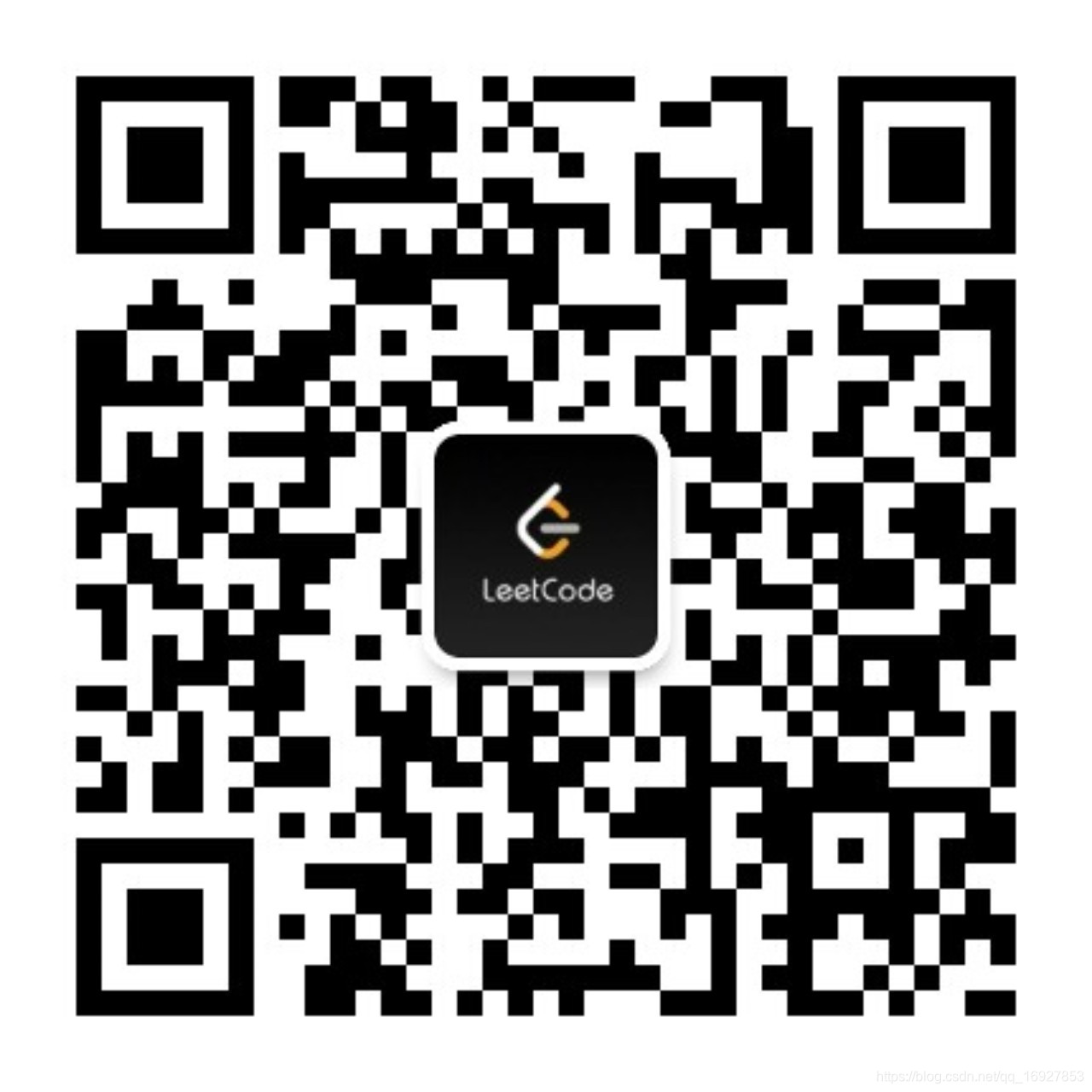