【LeetCode】138. Copy List with Random Pointer 复制带随机指针的链表(Medium)(JAVA)
题目地址: https://leetcode.com/problems/copy-list-with-random-pointer/
题目描述:
A linked list is given such that each node contains an additional random pointer which could point to any node in the list or null.
Return a deep copy of the list.
The Linked List is represented in the input/output as a list of n nodes. Each node is represented as a pair of [val, random_index] where:
- val: an integer representing Node.val
- random_index: the index of the node (range from 0 to n-1) where random pointer points to, or null if it does not point to any node.
Example 1:
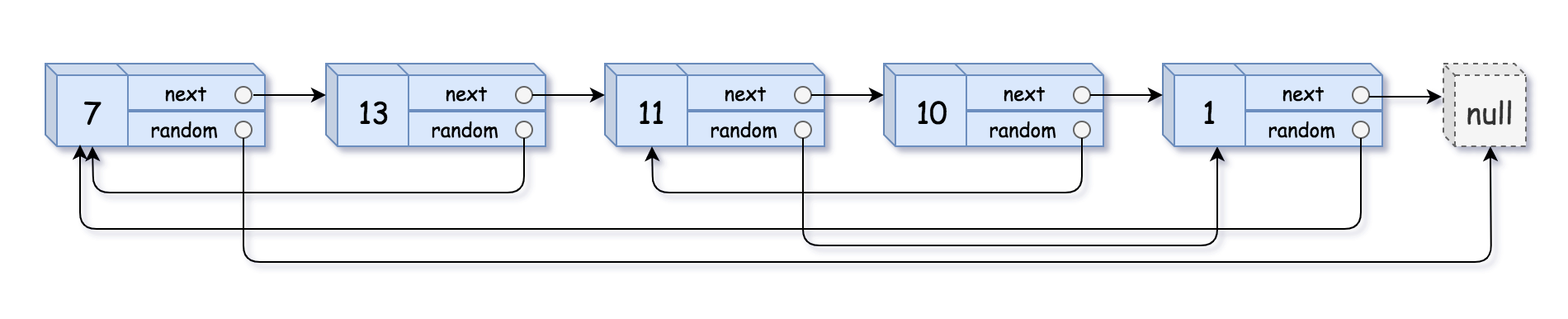
Input: head = [[7,null],[13,0],[11,4],[10,2],[1,0]]
Output: [[7,null],[13,0],[11,4],[10,2],[1,0]]
Example 2:

Input: head = [[1,1],[2,1]]
Output: [[1,1],[2,1]]
Example 3:
Input: head = [[3,null],[3,0],[3,null]]
Output: [[3,null],[3,0],[3,null]]
Example 4:
Input: head = []
Output: []
Explanation: Given linked list is empty (null pointer), so return null.
Constraints:
- -10000 <= Node.val <= 10000
- Node.random is null or pointing to a node in the linked list.
- The number of nodes will not exceed 1000.
题目大意
给定一个链表,每个节点包含一个额外增加的随机指针,该指针可以指向链表中的任何节点或空节点。
要求返回这个链表的 深拷贝。
我们用一个由 n 个节点组成的链表来表示输入/输出中的链表。每个节点用一个 [val, random_index] 表示:
- val:一个表示 Node.val 的整数。
- random_index:随机指针指向的节点索引(范围从 0 到 n-1);如果不指向任何节点,则为 null 。
解题方法
- 考深拷贝的问题,可能存在环,问题就是如何解开环
- 用一个 map 把当前 node 和 深拷贝的 copy 的结果存起来,下次再遇到直接拿来用即可
class Solution {
Map<Node, Node> map = new HashMap<>();
public Node copyRandomList(Node head) {
if (head == null) return null;
if (map.get(head) != null) return map.get(head);
Node copy = new Node(head.val);
map.put(head, copy);
copy.next = copyRandomList(head.next);
copy.random = copyRandomList(head.random);
return copy;
}
}
执行耗时:0 ms,击败了100.00% 的Java用户
内存消耗:38.7 MB,击败了28.44% 的Java用户
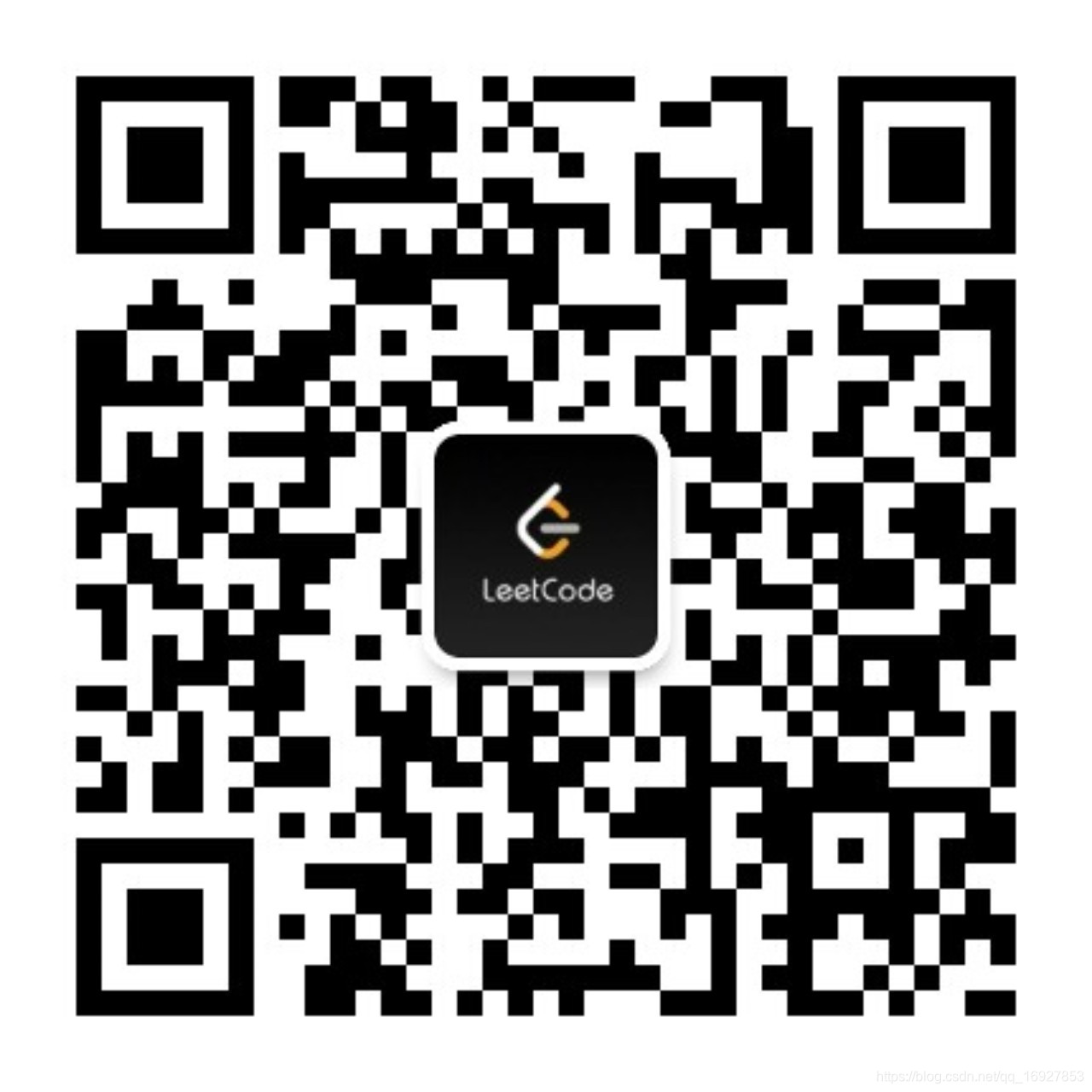