【LeetCode】151. Reverse Words in a String 翻转字符串里的单词(Medium)(JAVA)
题目地址: https://leetcode.com/problems/reverse-words-in-a-string/
题目描述:
Given an input string s, reverse the order of the words.
A word is defined as a sequence of non-space characters. The words in s will be separated by at least one space.
Return a string of the words in reverse order concatenated by a single space.
Note that s may contain leading or trailing spaces or multiple spaces between two words. The returned string should only have a single space separating the words. Do not include any extra spaces.
Example 1:
Input: s = "the sky is blue"
Output: "blue is sky the"
Example 2:
Input: s = " hello world "
Output: "world hello"
Explanation: Your reversed string should not contain leading or trailing spaces.
Example 3:
Input: s = "a good example"
Output: "example good a"
Explanation: You need to reduce multiple spaces between two words to a single space in the reversed string.
Example 4:
Input: s = " Bob Loves Alice "
Output: "Alice Loves Bob"
Example 5:
Input: s = "Alice does not even like bob"
Output: "bob like even not does Alice"
Constraints:
- 1 <= s.length <= 10^4
- s contains English letters (upper-case and lower-case), digits, and spaces ’ '.
- There is at least one word in s.
Follow up:
- Could you solve it in-place with O(1) extra space?
题目大意
给定一个字符串,逐个翻转字符串中的每个单词。
说明:
- 无空格字符构成一个 单词 。
- 输入字符串可以在前面或者后面包含多余的空格,但是反转后的字符不能包括。
- 如果两个单词间有多余的空格,将反转后单词间的空格减少到只含一个。
解题方法
- 用一个 StringBuilder res 来表示,遇到字符串(用 count 计数来判断当前字符串长度和区分是否遇到了空格),就在 res 前面插入
- 最后返回结果,注意最后一个字符串的情况
class Solution {
public String reverseWords(String s) {
StringBuilder res = new StringBuilder();
int count = 0;
for (int i = 0; i < s.length(); i++) {
if (s.charAt(i) == ' ') {
if (count == 0) continue;
if (res.length() == 0) {
res.append(s.substring(i - count, i));
} else {
res.insert(0, s.substring(i - count, i) + " ");
}
count = 0;
} else {
count++;
}
}
if (count > 0) {
if (res.length() == 0) {
res.append(s.substring(s.length() - count, s.length()));
} else {
res.insert(0, s.substring(s.length() - count, s.length()) + " ");
}
}
return res.toString();
}
}
执行耗时:7 ms,击败了55.25% 的Java用户
内存消耗:38.4 MB,击败了94.04% 的Java用户
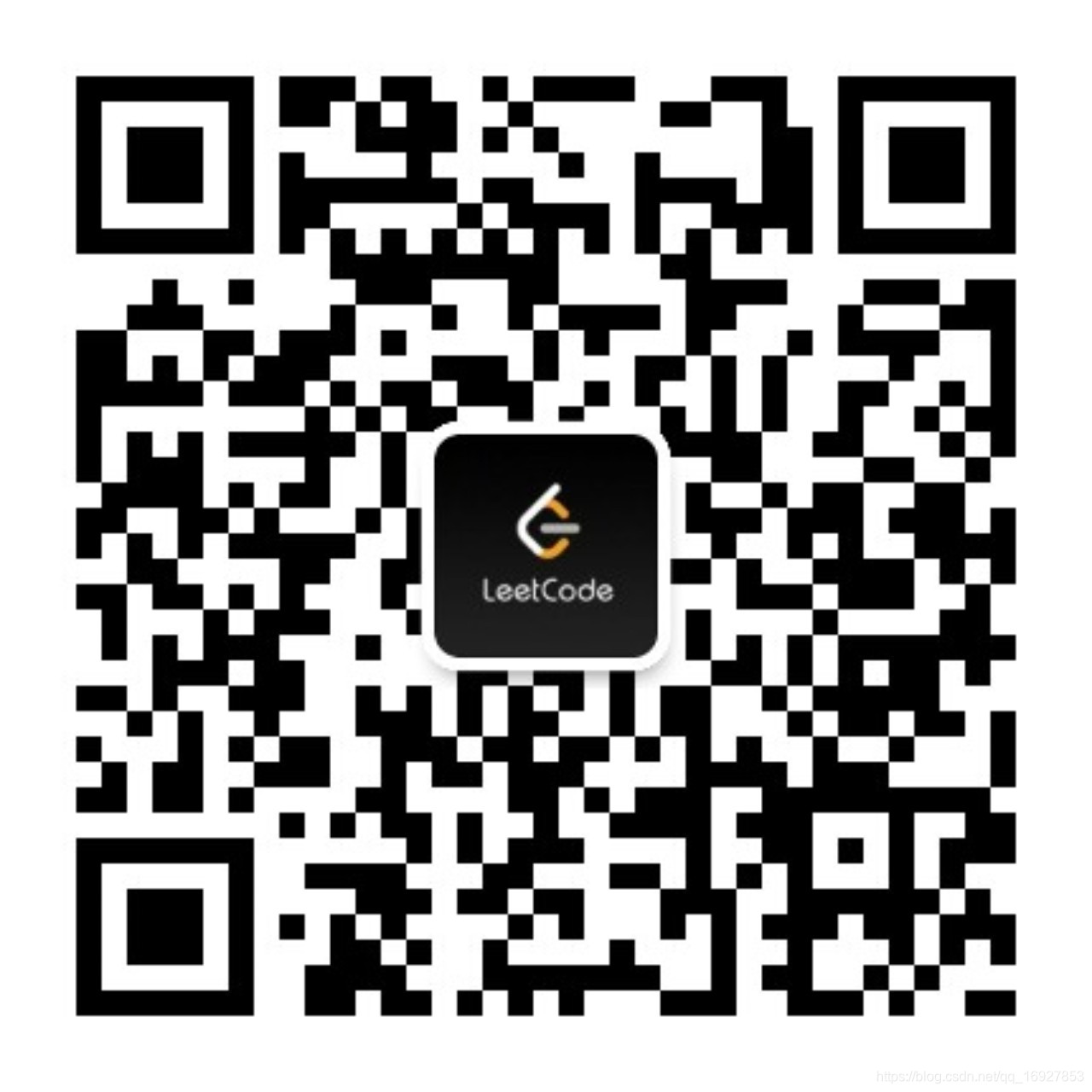