【LeetCode】189. Rotate Array 旋转数组(Medium)(JAVA)
题目地址: https://leetcode.com/problems/rotate-array/
题目描述:
Given an array, rotate the array to the right by k steps, where k is non-negative.
Follow up:
- Try to come up as many solutions as you can, there are at least 3 different ways to solve this problem.
- Could you do it in-place with O(1) extra space?
Example 1:
Input: nums = [1,2,3,4,5,6,7], k = 3
Output: [5,6,7,1,2,3,4]
Explanation:
rotate 1 steps to the right: [7,1,2,3,4,5,6]
rotate 2 steps to the right: [6,7,1,2,3,4,5]
rotate 3 steps to the right: [5,6,7,1,2,3,4]
Example 2:
Input: nums = [-1,-100,3,99], k = 2
Output: [3,99,-1,-100]
Explanation:
rotate 1 steps to the right: [99,-1,-100,3]
rotate 2 steps to the right: [3,99,-1,-100]
Constraints:
- 1 <= nums.length <= 2 * 10^4
- -2^31 <= nums[i] <= 2^31 - 1
- 0 <= k <= 10^5
题目大意
给定一个数组,将数组中的元素向右移动 k 个位置,其中 k 是非负数。
说明:
- 尽可能想出更多的解决方案,至少有三种不同的方法可以解决这个问题。
- 要求使用空间复杂度为 O(1) 的 原地 算法。
解题方法
方法一
- 用一个额外的数组来存
- 时间复杂度: O(n), 空间复杂度: O(n)
class Solution {
public void rotate(int[] nums, int k) {
if (nums.length == 0) return;
k = k % nums.length;
int[] res = new int[nums.length];
for (int i = 0; i < k; i++) {
res[i] = nums[nums.length - k + i];
}
for (int i = k; i < nums.length; i++) {
res[i] = nums[i - k];
}
for (int i = 0; i < nums.length; i++) {
nums[i] = res[i];
}
}
}
执行耗时:9 ms,击败了54.92% 的Java用户
内存消耗:41.1 MB,击败了16.91% 的Java用户
方法二
- 每次都移动一位
- 时间复杂度: O(kn),空间复杂度: O(1)
class Solution {
public void rotate(int[] nums, int k) {
if (nums.length <= 1) return;
k = k % nums.length;
if (k == 0) return;
int temp = nums[nums.length - 1];
for (int i = nums.length - 1; i > 0; i--) {
nums[i] = nums[i - 1];
}
nums[0] = temp;
rotate(nums, k - 1);
}
}
执行耗时:265 ms,击败了20.95% 的Java用户
内存消耗:39.9 MB,击败了5.13% 的Java用户
方法三
- 用环的方式,位置 0 -> k -> 2k -> 3k,直到 nk >= num.length; 再从 nk % num.length --> nk % num.length + k; 最终回到 0
- 最难判断的何时该停止交换,用一个 count 来计数,个数够了就停止
class Solution {
public void rotate(int[] nums, int k) {
if (nums.length <= 1) return;
k = k % nums.length;
if (k == 0) return;
int count = 0;
for (int i = 0; i < k && count < nums.length; i++) {
int pre = nums[i];
int next = (i + k) % nums.length;
while (next != i) {
int temp = nums[next];
nums[next] = pre;
pre = temp;
next = (next + k) % nums.length;
count++;
}
nums[i] = pre;
count++;
}
}
}
执行耗时:0 ms,击败了100.00% 的Java用户
内存消耗:39 MB,击败了75.85% 的Java用户
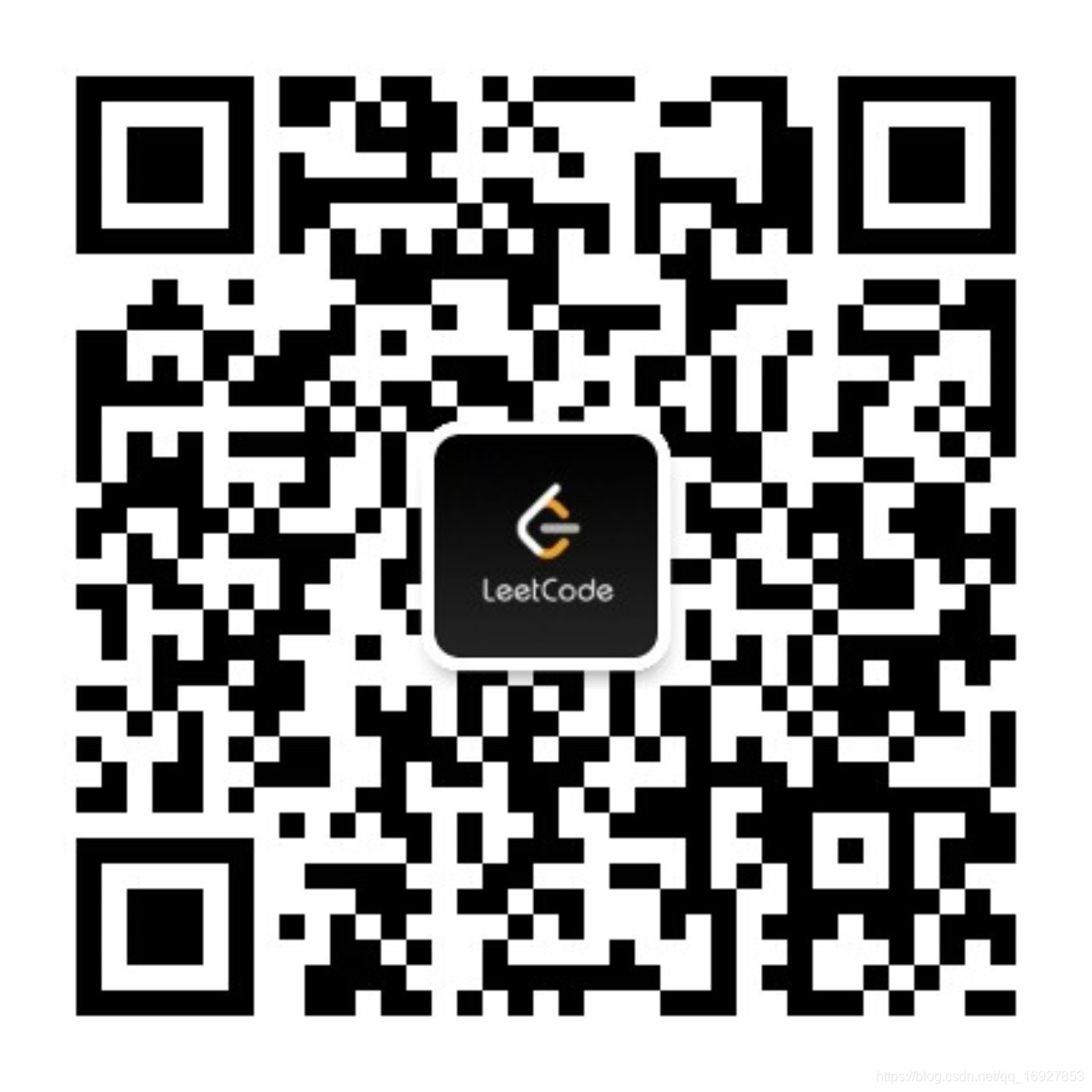