Java中Itext生成Pdf,并给PdfCell添加图片
1. 页眉页脚类 _ 根据实际情况使用,如不需要可忽略此步
package com.mediinfo.test;
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.*;
import lombok.SneakyThrows;
import lombok.extern.slf4j.Slf4j;
import java.io.IOException;
import java.util.Date;
@Slf4j
public class PdfHeaderFooter extends PdfPageEventHelper {
private final static String FONT_PATH = "c:\\windows\\fonts\\SIMSUN.TTC,1";
private final static String logoPath = "D:\\1.jpg";
public PdfTemplate totalNumTemplate = null;
public void onEndPage(PdfWriter writer, Document docment){
try{
this.addPageHeader(writer, docment);
}catch(Exception e){
log.error("添加页眉出错", e);
}
try{
this.addPageFooter(writer, docment);
}catch(Exception e){
log.error("添加页脚出错", e);
}
}
private void addPageHeader(PdfWriter writer, Document docment) throws IOException, DocumentException {
BaseFont BASE_FONT = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", false);
Font textFont = new Font(BASE_FONT, 10f);
PdfPTable table = new PdfPTable(2);
table.setTotalWidth(PageSize.A4.getWidth()-60);
try {
Image logo = Image.getInstance(logoPath);
Phrase logoP = new Phrase("", textFont);
logoP.add(new Chunk(logo, 0, -5));
PdfPCell logoCell = new PdfPCell(logoP);
logoCell.disableBorderSide(13);
logoCell.setFixedHeight(20);
table.addCell(logoCell);
}catch (IOException e){
Phrase nameP = new Phrase("", textFont);
PdfPCell nameCell = new PdfPCell(nameP);
nameCell.disableBorderSide(13);
nameCell.setFixedHeight(20);
table.addCell(nameCell);
}
Phrase nameP = new Phrase("页眉右上角显示的内容", textFont);
PdfPCell nameCell = new PdfPCell(nameP);
nameCell.disableBorderSide(13);
nameCell.setFixedHeight(20);
nameCell.setHorizontalAlignment(Element.ALIGN_RIGHT);
nameCell.setVerticalAlignment(Element.ALIGN_MIDDLE);
table.addCell(nameCell);
table.writeSelectedRows(0, -1, 30, PageSize.A4.getHeight()-5, writer.getDirectContent());
}
private void addPageFooter(PdfWriter writer, Document docment) throws DocumentException, IOException {
BaseFont BASE_FONT = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", false);
Font textFont = new Font(BASE_FONT, 10f);
PdfPTable table = new PdfPTable(3);
table.setTotalWidth(PageSize.A4.getWidth()-60);
table.getDefaultCell().disableBorderSide(14);
table.getDefaultCell().setFixedHeight(40);
table.getDefaultCell().setVerticalAlignment(Element.ALIGN_MIDDLE);
table.addCell(new Phrase("admin", textFont));
if(null == totalNumTemplate){
totalNumTemplate = writer.getDirectContent().createTemplate(30, 16);
}
PdfPTable pageNumTable = new PdfPTable(2);
pageNumTable.setTotalWidth(new float[]{80f, 80f});
pageNumTable.setLockedWidth(true);
pageNumTable.setPaddingTop(-5f);
pageNumTable.getDefaultCell().disableBorderSide(15);
pageNumTable.getDefaultCell().setFixedHeight(16);
pageNumTable.getDefaultCell().setHorizontalAlignment(Element.ALIGN_RIGHT);
pageNumTable.getDefaultCell().setVerticalAlignment(Element.ALIGN_BOTTOM);
pageNumTable.addCell(new Phrase(writer.getPageNumber()+" / ", textFont));
Image totalNumImg = Image.getInstance(totalNumTemplate);
totalNumImg.setPaddingTop(-5f);
pageNumTable.getDefaultCell().setPaddingTop(-18f);
pageNumTable.getDefaultCell().setHorizontalAlignment(Element.ALIGN_LEFT);
pageNumTable.getDefaultCell().setVerticalAlignment(Element.ALIGN_TOP);
pageNumTable.addCell(totalNumImg);
table.addCell(pageNumTable);
table.addCell(new Phrase(String.valueOf(new Date()), textFont));
table.writeSelectedRows(0, -1, 30, 40, writer.getDirectContent());
}
@SneakyThrows
@Override
public void onCloseDocument(PdfWriter writer, Document docment){
BaseFont BASE_FONT = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", false);
Font textFont = new Font(BASE_FONT, 10f);
String totalNum = writer.getPageNumber() + "页";
totalNumTemplate.beginText();
totalNumTemplate.setFontAndSize(BASE_FONT, 5f);
totalNumTemplate.showText(totalNum);
totalNumTemplate.setHeight(16f);
totalNumTemplate.endText();
totalNumTemplate.closePath();
}
}
2. Pdf生成 [ PdfCell添加图片参考createHardwarePDF方法的第九行nineRowTalbe ]
package com.mediinfo.test;
import com.itextpdf.text.*;
import com.itextpdf.text.pdf.BaseFont;
import com.itextpdf.text.pdf.PdfPCell;
import com.itextpdf.text.pdf.PdfPTable;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.*;
public class PdfUtils {
public static void main(String[] args) throws Exception {
createHardwarePDF("d:/test.pdf");
}
private static PdfPCell mircoSoftFont(String str, Font font, int high, boolean alignCenter, boolean alignMidde) {
PdfPCell pdfPCell = new PdfPCell(new Phrase(str, font));
pdfPCell.setMinimumHeight(high);
pdfPCell.setUseAscender(true);
if (alignCenter) {
pdfPCell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
}
if (alignMidde) {
pdfPCell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
}
return pdfPCell;
}
private static PdfPCell mircoSoftFont(String str, Font font, int high, boolean alignCenter, boolean alignMidde, boolean haveColor) {
PdfPCell pdfPCell = new PdfPCell(new Phrase(str, font));
pdfPCell.setMinimumHeight(high);
pdfPCell.setUseAscender(true);
if (alignCenter) {
pdfPCell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
}
if (alignMidde) {
pdfPCell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
}
if (haveColor) {
pdfPCell.setBackgroundColor(new BaseColor(217, 217, 217));
}
return pdfPCell;
}
private static PdfPCell ImageSet(int high) throws Exception {
byte[] fileByte = toByteArray("D:\\2.jpg");
Jpeg jpeg = new Jpeg(fileByte);
jpeg.scaleAbsolute(70, 50);
PdfPCell pdfPCell = new PdfPCell(jpeg);
pdfPCell.setMinimumHeight(high);
pdfPCell.setUseAscender(true);
pdfPCell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
pdfPCell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
return pdfPCell;
}
public static void createHardwarePDF(String outputPath) throws Exception {
Document document = new Document(PageSize.A4, 20, 20, 30, 30);
PdfWriter writer = PdfWriter.getInstance(document, new FileOutputStream(outputPath));
BaseFont baseFont = BaseFont.createFont("STSong-Light", "UniGB-UCS2-H", BaseFont.NOT_EMBEDDED);
Font size14font = new Font(baseFont, 14, Font.NORMAL);
Font size10font = new Font(baseFont, 10, Font.BOLD);
document.open();
PdfHeaderFooter event = new PdfHeaderFooter();
writer.setPageEvent(event);
PdfPTable tableName = new PdfPTable(1);
tableName.setWidthPercentage(90);
PdfPCell titleCell = mircoSoftFont("个人信息", size14font, 80, true, true);
titleCell.disableBorderSide(15);
tableName.addCell(titleCell);
document.add(tableName);
PdfPTable secondRowTable = new PdfPTable(3);
secondRowTable.setWidthPercentage(90);
secondRowTable.setTotalWidth(new float[]{0.18f, 0.32f, 0.5f});
secondRowTable.addCell(mircoSoftFont(" 姓名: ", size10font, 50, false, true));
secondRowTable.addCell(mircoSoftFont("李晓明", size10font, 50, false, true));
secondRowTable.addCell(mircoSoftFont(" 出生日期: 1994年3月14日", size10font, 50, false, true));
document.add(secondRowTable);
PdfPTable thirdRowTable = new PdfPTable(3);
thirdRowTable.setWidthPercentage(90);
thirdRowTable.setTotalWidth(new float[]{0.18f, 0.32f, 0.5f});
thirdRowTable.addCell(mircoSoftFont(" 名族:", size10font, 50, false, true));
thirdRowTable.addCell(mircoSoftFont("汉族", size10font, 50, false, true));
thirdRowTable.addCell(mircoSoftFont(" 联系电话: 13888880000", size10font, 50, false, true));
document.add(thirdRowTable);
PdfPTable fourthRowTable = new PdfPTable(2);
fourthRowTable.setWidthPercentage(90);
fourthRowTable.setTotalWidth(new float[]{0.66f, 0.34f});
fourthRowTable.addCell(mircoSoftFont(" 个人描述 :", size10font, 175, false, false));
fourthRowTable.addCell(mircoSoftFont("个人特长 :", size10font, 175, false, false));
document.add(fourthRowTable);
PdfPTable fifthDetailName = new PdfPTable(1);
fifthDetailName.setWidthPercentage(90);
fifthDetailName.addCell(mircoSoftFont("获奖记录 :", size14font, 50, true, true));
document.add(fifthDetailName);
PdfPTable sisthRowTalbe = new PdfPTable(1);
sisthRowTalbe.setWidthPercentage(90);
sisthRowTalbe.addCell(mircoSoftFont(" 联系地址: " + "广东省广州市天河区XXXXXXXXXXXXXXXXXX", size10font, 50, false, true));
document.add(sisthRowTalbe);
PdfPTable seventhRowTalbe = new PdfPTable(1);
seventhRowTalbe.setWidthPercentage(90);
seventhRowTalbe.addCell(mircoSoftFont(" 毕业院校 ", size14font, 60, true, true));
document.add(seventhRowTalbe);
PdfPTable eiththRowTalbe = new PdfPTable(3);
eiththRowTalbe.setWidthPercentage(90);
eiththRowTalbe.setTotalWidth(new float[]{0.3f, 0.5f, 0.2f});
eiththRowTalbe.addCell(mircoSoftFont(" 毕业学校", size10font, 50, true, true, true));
eiththRowTalbe.addCell(mircoSoftFont(" 就读日期", size10font, 50, true, true, true));
eiththRowTalbe.addCell(mircoSoftFont(" 联系人", size10font, 50, true, true, true));
document.add(eiththRowTalbe);
String school = "XXX学校";
String time = "201909 - 2022-06";
String name = "陈某";
for (int i = 0; i < 2; i++) {
PdfPTable tempTable = new PdfPTable(3);
tempTable.setWidthPercentage(90);
tempTable.setTotalWidth(new float[]{0.3f, 0.5f, 0.2f});
tempTable.addCell(mircoSoftFont(school, size10font, 50, true, true));
tempTable.addCell(mircoSoftFont(time, size10font, 50, true, true));
tempTable.addCell(mircoSoftFont(name, size10font, 50, true, true));
document.add(tempTable);
}
PdfPTable nineRowTalbe = new PdfPTable(3);
nineRowTalbe.setWidthPercentage(90);
nineRowTalbe.setTotalWidth(new float[]{0.3f, 0.5f, 0.2f});
nineRowTalbe.addCell(mircoSoftFont(" test1", size10font, 50, true, true));
nineRowTalbe.addCell(mircoSoftFont(" test222", size10font, 50, true, true));
nineRowTalbe.addCell(ImageSet(50));
document.add(nineRowTalbe);
document.close();
writer.close();
}
public static byte[] toByteArray(String filename) throws IOException {
File f = new File(filename);
if (!f.exists()) {
throw new FileNotFoundException(filename);
}
ByteArrayOutputStream bos = new ByteArrayOutputStream((int) f.length());
BufferedInputStream in = null;
try {
in = new BufferedInputStream(new FileInputStream(f));
int buf_size = 1024;
byte[] buffer = new byte[buf_size];
int len = 0;
while (-1 != (len = in.read(buffer, 0, buf_size))) {
bos.write(buffer, 0, len);
}
return bos.toByteArray();
} catch (IOException e) {
e.printStackTrace();
throw e;
} finally {
try {
in.close();
} catch (IOException e) {
e.printStackTrace();
}
bos.close();
}
}
}
3. 实现效果如下图
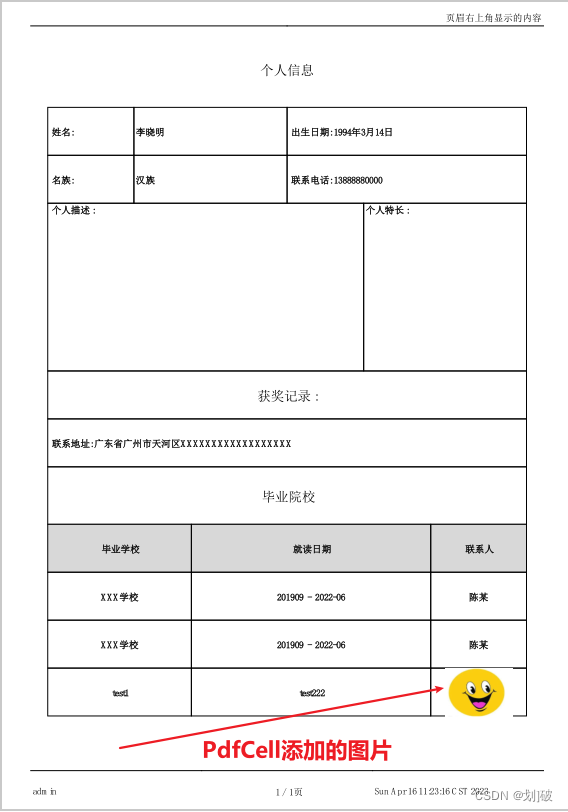