package Hbase;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.Cell;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.HColumnDescriptor;
import org.apache.hadoop.hbase.HTableDescriptor;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.Connection;
import org.apache.hadoop.hbase.client.ConnectionFactory;
import org.apache.hadoop.hbase.client.HBaseAdmin;
import org.apache.hadoop.hbase.client.HTable;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.client.Table;
import org.apache.hadoop.hbase.util.Bytes;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class HbaseImport {
static Configuration config = null;
private static Connection connection = null;
private Table table = null;
public static void init(String zkIP) throws Exception {
config = HBaseConfiguration.create();// 配置
config.set("hbase.zookeeper.quorum", zkIP);// zookeeper地址
config.set("hbase.zookeeper.property.clientPort", "2181");// zookeeper端口
config.set("hbase.rpc.timeout", "1000");
//System.out.println(config.get("hbase.rpc.timeout"));
//System.out.println(config.get("zookeeper.znode.parent"));
connection = ConnectionFactory.createConnection(config);
}
public static void createTable(String tableName) {
HBaseAdmin admin = null;
try {
admin = (HBaseAdmin) connection.getAdmin();
//判断表是否存在`
if (admin.tableExists(tableName)) {
System.out.println("table already exists!");
return;
}
//Table table = connection.getTable(TableName.valueOf(tableName));
//HTableDescriptor tableDesc = table.getTableDescriptor();
HTableDescriptor tableDesc = new HTableDescriptor(TableName.valueOf(tableName));
HColumnDescriptor nameColumnDesc = new HColumnDescriptor("info");
tableDesc.addFamily(nameColumnDesc);
HColumnDescriptor addressColumnDesc = new HColumnDescriptor("other");
tableDesc.addFamily(addressColumnDesc);
//创建表
admin.createTable(tableDesc);
System.out.println("create table " + tableName + " ok.");
} catch (Exception e) {
e.printStackTrace();
System.out.println(tableName + " create failed");
} finally {
try {
if (admin != null) {
admin.close();
}
} catch (Exception e) {
e.printStackTrace();
System.out.println("close HBaseAdmin failed");
}
}
return;
}
public static void insertMany(String tableName,int num) throws Exception {
Table table = connection.getTable(TableName.valueOf(tableName));
String address[] = {"南京","上海","南通","北京","杭州","深圳","武汉","成都","天津","西安"};
String x[]={"赵","钱","孙","李","周","吴","王","许","李"};
String m[]={"一一","康","栋","建国","天意","小小","大大","天天","宁","静","无名","俊","安安","洒家","上","下"};
List<Put> puts = new ArrayList<Put>();
for(int i =0;i<num;i++){
String rowKey = Integer.toString(i);
Put put = new Put(Bytes.toBytes(rowKey));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("name"), Bytes.toBytes(x[getRandom(0,x.length-1)]+m[getRandom(0,m.length-1)]));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("sex"), Bytes.toBytes(1));
put.addColumn(Bytes.toBytes("info"), Bytes.toBytes("addres"), Bytes.toBytes(address[getRandom(0,address.length-1)]));
put.addColumn(Bytes.toBytes("other"), Bytes.toBytes("other"), Bytes.toBytes(getRandom(0,getRandom(0,99))));
puts.add(put);
if(i!=0 && i%1000000==0){
table.put(puts);
puts.clear();
System.out.println("success add 1000000 date to "+tableName);
}
}
table.put(puts);
}
public static int getRandom(int min, int max){
Random random = new Random();
int s = random.nextInt(max - min + 1) + min;
return s;
}
public static void scanTable(String tablename,String start,String end) {
try {
//HTable table = new HTable(config, tablename);
HTable table = (HTable) connection.getTable(TableName.valueOf(tablename));
Scan scan = new Scan();
scan.setStartRow(start.getBytes());
scan.setStopRow(end.getBytes());
ResultScanner scanner = table.getScanner(scan);
for (Result r = scanner.next(); r != null; r = scanner.next()) {
for (Cell value : r.rawCells()) {
System.out.println(Bytes.toString(value.getRowArray()) + ","
+ Bytes.toString(value.getFamilyArray()) + ","
+ Bytes.toString(value.getQualifierArray()) + ","
+ Bytes.toString(value.getValueArray()));
}
/*for (KeyValue kv : r.raw()) {
System.out.println(Bytes.toString(kv.getRow()) + ","
+ Bytes.toString(kv.getFamily()) + ","
+ Bytes.toString(kv.getQualifier()) + ","
+ Bytes.toString(kv.getValue()));
}*/
}
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) throws Exception {
String zk = args[0];
init(zk);
String tableName = args[1];
int num = Integer.valueOf(args[2]);
createTable(tableName);
insertMany(tableName,num);
connection.close();
}
}
Habse造数据
最新推荐文章于 2024-08-16 23:08:28 发布
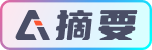