例1.在随机数组中查找输入的数据
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define _CRT_SECURE_NO_DEPRECATE;
constexpr int Num = 20;
int main()
{
int arr[Num], x, n, i;
int f = -1;
srand(time(NULL));
for (int i = 0; i < Num; i++)
{
arr[i] = rand() / 1000;
}
printf("输入要查找的整数:");
scanf_s("%d", &x);
for (int i = 0; i < arr[i]; i++)
{
if (x == arr[i])
{
f = i;
break;
}
}
printf("\n随机生成的数据序列\n");
for (int i = 0; i < Num; i++)
{
printf("%d", arr[i]);
printf(" ");
}
printf("\n\n");
if (f < 0)
{
printf("没找到数据:%d\n", x);
}
else
{
printf("数据:%d 位于数组的第 %d个元素处.\n", x, f + 1);
}
system("pause");
return 0;
}
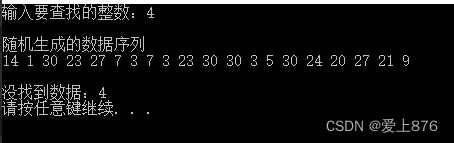
例2.顺序表
#include <stdio.h>
#include <string.h>
constexpr int MAXLEN = 20;
typedef struct
{
char key[20];
char name[20];
int age;
} DATA;
typedef struct
{
DATA listData[MAXLEN + 1];
int listLen;
}SLType;
void SLInit(SLType *pSL)
{
pSL->listLen = 0;
}
int SLLength(SLType *SL)
{
return (SL->listLen);
}
int SLInsert(SLType *pSL, int n, DATA data)
{
int i = 0;
if (pSL->listLen >= MAXLEN)
{
printf("顺序表已满,不能插入结点!\n");
return 0;
}
if (n < 1 || (n > pSL->listLen - 1))
{
printf("插入元素序号错误,不能插入元素");
return 0;
}
for (int i = pSL->listLen; i >= n; i--)
{
pSL->listData[i + 1] = pSL->listData[i];
}
pSL->listData[n] = data;
pSL->listLen++;
return 1;
}
int SLAdd(SLType *pSL, DATA data)
{
if (pSL->listLen >= MAXLEN)
{
printf("顺序表已满, 不能再添加结点了!");
return 0;
}
pSL->listData[++pSL->listLen] = data;
return 1;
}
int SLDelate(SLType *pSL, int n)
{
int i;
if (n<1 || n>pSL->listLen + 1)
{
printf("删除结点序号错误,不能删除结点!\n");
return 0;
}
for (int i = n; i < pSL ->listLen; i++)
{
pSL->listData[i] = pSL->listData[i + 1];
}
pSL->listLen--;
return 1;
}
DATA *SLFindByName(SLType *pSL, int n)
{
if (n<1 || n>pSL->listLen + 1)
{
printf("结点序号错误,不能返回结点!\n");
return nullptr;
}
return &pSL->listData[n];
}
int SLFindByCont(SLType *pSL, char* key)
{
int i;
for (i = 1; i <= pSL->listLen; i++)
{
if (strcmp(pSL->listData[i].key, key) == 0)
{
return i;
}
}
return 0;
}
int SLAll(SLType *pSL)
{
int i;
for (int i = 1; i < pSL->listLen; i++)
{
printf("(%s, %s, %d)\n", pSL->listData[i].key, pSL->listData[i].name, pSL->listData[i].age);
}
return 1;
}
int main()
{
int i;
SLType sl;
DATA data;
DATA *pData;
char key[10];
printf("顺序操作演示!\n");
SLInit(&sl);
do
{
printf("输入添加的结点(学号 姓名 年龄)");
fflush(stdin); // 清空输入缓冲区
scanf_s("%s%s%d", &data.key, &data.name, &data.age);
if (data.age)
{
if (!SLAdd(&sl, data))
{
break;
}
}
else
{
break;
}
} while (1);
printf("\n顺序表中的结点顺序为:\n");
SLAll(&sl);
}
3.链表
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct
{
char key[10];
char name[20];
int age;
} Data;
typedef struct Node
{
Data nodeData;
struct Node* nextNode;
}CLType;
CLType *CLAddEnd(CLType *pHead, Data nodeData)
{
CLType *node, *htemp;
if (!(node = (CLType *)malloc(sizeof(CLType))))
{
printf("申请内存失败!\n");
return NULL;
}
else
{
node->nodeData = nodeData;
node->nextNode = nullptr;
if (pHead == nullptr)
{
pHead = node;
return pHead;
}
htemp = pHead;
while (htemp->nextNode != nullptr)
{
htemp = htemp->nextNode;
}
htemp->nextNode = node;
return pHead;
}
}
CLType *CLAddFirst(CLType *pHead, Data nodeData)
{
CLType *node;
if (!(node = (CLType *)malloc(sizeof(CLType))))
{
printf("查找内存失败!\n");
return nullptr;
}
else
{
node->nodeData = nodeData;
node->nextNode = pHead;
pHead = node;
return pHead;
}
}
CLType *CLFindNode(CLType *pHead, char *key)
{
CLType *pHtemp = nullptr;
pHtemp = pHead;
while(pHtemp)
{
if (strcmp(pHtemp->nodeData.key, key) == 0)
{
return pHtemp;
}
pHtemp = pHtemp->nextNode;
}
return nullptr;
}
CLType* CLInsertNode(CLType *pHead, char* findKey, Data nodeData)
{
CLType *pNode, *pHtemp;
if (!(pNode = (CLType*)malloc(sizeof(CLType))))
{
printf("申请内存失败!\n");
return 0;
}
pNode->nodeData = nodeData;
pHtemp = CLFindNode(pHead, findKey);
if (pHtemp)
{
pNode = pHtemp->nextNode;
pHtemp->nextNode = pNode;
}
else
{
printf("未找到正确的插入位置!\n");
free(pNode);
}
return pNode;
}
int CLDealateNode(CLType *pHead, char *key)
{
CLType *pNode, *pHtemp;
pHtemp = pHead;
while (pHtemp)
{
if (strcmp(pHtemp->nodeData.key, key) == 0)
{
free(pHtemp);
return 1;
}
else
{
pHtemp = pHtemp->nextNode;
}
}
return 0;
}
int CLLength(CLType *pHead)
{
CLType *pHtemp = nullptr;
int len = 0;
pHtemp = pHead;
while (pHtemp)
{
len++;
pHtemp = pHtemp->nextNode;
}
return len;
}
void CLAllNode(CLType *pHead)
{
CLType *pHtemp = nullptr;
Data nodeData;
pHtemp = pHead;
printf("当前链表共有%d个结点。链表数据如下:\n", CLLength(pHead));
while (pHtemp)
{
nodeData = pHtemp->nodeData;
printf("结点(%s%s%d)\n", nodeData.key, nodeData.name, nodeData.age);
pHtemp = pHtemp->nextNode;
}
}
void main()
{
CLType *pNode, *pHead = nullptr;
Data nodeData;
char key[10], findkey[10];
printf("链表测试,先输入链表中的数据,格式为:关键字 姓名 年龄\n");
do
{
fflush(stdin);
scanf_s("%s", nodeData.key);
if (strcmp(nodeData.key, "0") == 0)
{
break;
}
else
{
scanf_s("%s%d", nodeData.name, nodeData.age);
pHead = CLAddEnd(pHead, nodeData);
}
} while (1);
CLAllNode(pHead);
}
4.栈
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
constexpr int MAXLEN = 50;
typedef struct
{
char name[20];
int age;
} Data;
typedef struct Node
{
Data data[MAXLEN +1];
int top;
}StackType;
StackType *STInit()
{
StackType *pHead;
if (pHead = (StackType*)malloc(sizeof(StackType)))
{
pHead->top = 0;
return 0;
}
return nullptr;
}
int StIsEmpty(StackType *pSt)
{
int t;
t = (pSt->top == 0);
return t;
}
int STIsFull(StackType *s)
{
int t;
t = (s->top == MAXLEN);
return t;
}
void STClear(StackType *s)
{
s->top = 0;
}
void STFree(StackType *s)
{
if (s)
{
free(s);
}
}
int PushST(StackType *s, Data data)
{
if ((s->top + 1) > MAXLEN)
{
printf("栈溢出");
return 0;
}
s->data[++s->top] = data;
return 1;
}
Data PopST(StackType *s)
{
if (s->top == 0)
{
printf("栈为空!\n");
exit(0);
}
return (s->data[s->top]);
}
void main()
{
}
5.队列结构
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
constexpr int QUEUELEN = 15;
typedef struct
{
char name[10];
int age;
}Data;
typedef struct
{
Data data[QUEUELEN];
int head;
int tail;
}SQType;
SQType *SQTypeInit()
{
SQType *pSq;
if (pSq = (SQType *)malloc(sizeof(SQType)))
{
pSq->head = 0;
pSq->tail = 0;
return pSq;
}
else
{
return nullptr;
}
}
int SQTypeIsEmpty(SQType *pSq)
{
int temp;
temp = (pSq->head == pSq->tail);
return temp;
}
int SQTypeIsFull(SQType *pSq)
{
int temp;
temp = (pSq->tail == QUEUELEN);
return temp;
}
void SQTypeClear(SQType *pSq)
{
pSq->head = 0;
pSq->tail = 0;
}
void SQTypeFree(SQType *pSq)
{
if (pSq != nullptr)
{
free(pSq);
}
}
void InSQType(SQType *pSq, Data data)
{
if (pSq->tail == QUEUELEN)
{
printf("队列已满!操作失败!");
return;
}
else
{
pSq->data[pSq ->tail++] = data;
}
}
Data *OutSQType(SQType *pSq)
{
if (pSq->head == pSq->tail)
{
printf("\n队列已空!操作失败!\n");
exit(0);
}
else
{
return &(pSq->data[pSq->head++]);
}
}
Data *PeekSQType(SQType *pSq)
{
if (SQTypeIsEmpty(pSq))
{
printf("\n 空队列!\n");
return nullptr;
}
else
{
return &(pSq->data[pSq->head]);
}
}
int SQTypeLen(SQType *pSq)
{
int temp = 0;
temp = pSq->tail - pSq->head;
return temp;
}
void main()
{
}