vue自定义loading组件
首先看一下实现效果
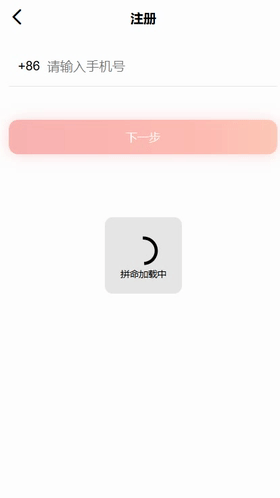
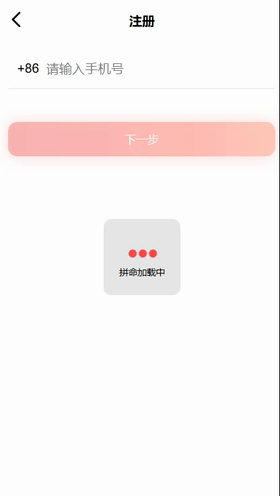
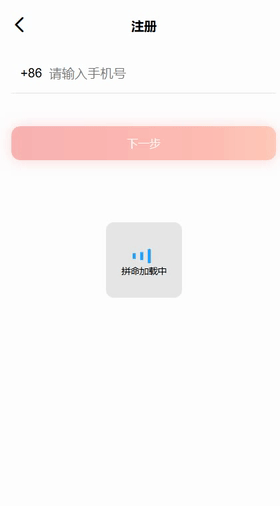
下面就来实现这个组件吧
1.先看一下目录结构
一般情况下,每一个组件都新建一个新的文件夹,里面包含对应的vue文件,和js文件,如下图:
2.实现组件代码
loading.vue代码
大部分的代码主要是为了实现loading的动画,如果嫌代码太多可以将代码封装一下。
<template>
<div v-if="isShowLoading" class="loading-container">
<div class="loading-box">
<div v-if="seletedType === 'balls' " style="fill: rgb(255, 73, 73); width: 40px; height: 40px;">
<svg x="0px" y="0px" viewBox="0 0 30 30" style="enable-background:new 0 0 50 50;" xml:space="preserve">
<path transform="translate(-8 0)" d="M4 12 A4 4 0 0 0 4 20 A4 4 0 0 0 4 12">
<animateTransform attributeName="transform" type="translate" values="-8 0; 2 0; 2 0;" dur="0.8s" repeatCount="indefinite" begin="0" keyTimes="0;.25;1" keySplines="0.2 0.2 0.4 0.8;0.2 0.6 0.4 0.8" calcMode="spline"></animateTransform>
</path>
<path transform="translate(2 0)" d="M4 12 A4 4 0 0 0 4 20 A4 4 0 0 0 4 12">
<animateTransform attributeName="transform" type="translate" values="2 0; 12 0; 12 0;" dur="0.8s" repeatCount="indefinite" begin="0" keyTimes="0;.35;1" keySplines="0.2 0.2 0.4 0.8;0.2 0.6 0.4 0.8" calcMode="spline"></animateTransform>
</path>
<path transform="translate(12 0)" d="M4 12 A4 4 0 0 0 4 20 A4 4 0 0 0 4 12">
<animateTransform attributeName="transform" type="translate" values="12 0; 22 0; 22 0;" dur="0.8s" repeatCount="indefinite" begin="0" keyTimes="0;.45;1" keySplines="0.2 0.2 0.4 0.8;0.2 0.6 0.4 0.8" calcMode="spline"></animateTransform>
</path>
<path transform="translate(24 0)" d="M4 12 A4 4 0 0 0 4 20 A4 4 0 0 0 4 12">
<animateTransform attributeName="transform" type="translate" values="22 0; 32 0; 32 0;" dur="0.8s" repeatCount="indefinite" begin="0" keyTimes="0;.55;1" keySplines="0.2 0.2 0.4 0.8;0.2 0.6 0.4 0.8" calcMode="spline"></animateTransform>
</path>
</svg>
</div>
<div v-if="seletedType === 'bars' " style="fill: rgb(32, 160, 255); width: 30px; height: 30px;">
<svg x="0px" y="0px" viewBox="0 0 30 30" style="enable-background:new 0 0 50 50;" xml:space="preserve">
<rect x="0" y="13" width="4" height="5">
<animate attributeName="height" attributeType="XML" values="5;21;5" begin="0s" dur="0.6s" repeatCount="indefinite"></animate>
<animate attributeName="y" attributeType="XML" values="13; 5; 13" begin="0s" dur="0.6s" repeatCount="indefinite"></animate>
</rect>
<rect x="10" y="13" width="4" height="5">
<animate attributeName="height" attributeType="XML" values="5;21;5" begin="0.15s" dur="0.6s" repeatCount="indefinite"></animate>
<animate attributeName="y" attributeType="XML" values="13; 5; 13" begin="0.15s" dur="0.6s" repeatCount="indefinite"></animate>
</rect>
<rect x="20" y="13" width="4" height="5">
<animate attributeName="height" attributeType="XML" values="5;21;5" begin="0.3s" dur="0.6s" repeatCount="indefinite"></animate>
<animate attributeName="y" attributeType="XML" values="13; 5; 13" begin="0.3s" dur="0.6s" repeatCount="indefinite"></animate>
</rect>
</svg>
</div>
<div v-if="seletedType === 'spin' " style="fill: black; width: 50px; height: 50px;">
<svg x="0px" y="0px" viewBox="0 0 50 50" style="enable-background:new 0 0 50 50;" xml:space="preserve">
<path d="M43.935,25.145c0-10.318-8.364-18.683-18.683-18.683c-10.318,0-18.683,8.365-18.683,18.683h4.068c0-8.071,6.543-14.615,14.615-14.615c8.072,0,14.615,6.543,14.615,14.615H43.935z">
<animateTransform attributeType="xml" attributeName="transform" type="rotate" from="0 25 25" to="360 25 25" dur="0.6s" repeatCount="indefinite"></animateTransform>
</path>
</svg>
</div>
<span class="loading-txt">{{content}}</span>
</div>
</div>
</template>
<script>
export default {
data () {
return {
isShowLoading: false,
content: '',
seletedType: ''
}
}
}
</script>
<style scoped>
.loading-container {
display: flex;
justify-content: center;
align-items: center;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0);
z-index: 1000;
}
.loading-box {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
width: 100px;
height: 100px;
border-radius: 10px;
background: #e7e7e7;
}
.loading-txt {
font-size: 12px;
color: #000;
}
</style>
js代码
import Vue from 'Vue'
import LoadingComponent from './loading.vue'
const Loading = {}
let showLoading = false // 存储loading显示状态
let loadingNode = null // 存储loading节点元素
const LoadingConstructor = Vue.extend(LoadingComponent)
Loading.install = function (Vue) {
Vue.prototype.$loading = function (tips,type, method) {
if (method === 'hide') {
loadingNode.isShowLoading = showLoading = false
} else {
if (showLoading) {
return
}
if(type != 'spin' && type != 'balls' && type != 'bars'){
type = 'balls';
}
loadingNode = new LoadingConstructor({
data: {
isShowLoading: showLoading,
content: tips,
seletedType: type
}
})
loadingNode.$mount()
document.body.appendChild(loadingNode.$el)
loadingNode.isShowLoading = showLoading = true
}
};
['show', 'hide'].forEach(function (method) {
Vue.prototype.$loading[method] = function (tips, type) {
return Vue.prototype.$loading(tips, type,method)
}
})
}
export default Loading
以上就是这个组件的全部代码,创建文件后直接把代码拷进去就行了。
创建完这个组件后,我们开始使用它吧。
3.使用过程
因为在一个项目中,loading组件可能要使用到多处地方,所以我们在main.js中把它定义为全局组件。
在main.js文件中导入这个组件
import Loading from './components/loading/loading'//注意路径是否正确
Vue.use(Loading)
导入这个组件后,我们就可以直接使用这个组件了,简单吧
使用方法
1.显示loading的方法
this.$loading.show('拼命加载中','ball');
该方法传递两个参数,第一个参数是显示的文本信息,第二个参数是设置动画的类型。若是不传参数的话则默认空的文本信息和ball动画效果(即“小球”滚动效果)。
第二个参数可以传递的值有:spin、ball、bars。效果实现依次为顶部的效果展示。若传递的值错误则默认为ball。
2.隐藏loading的方法
this.$loading.hide();