日期格式化
simpleDateFormat(已过期)
SimpleDateFormat dateFormat=new SimpleDateFormat("yyyy-MM-dd");
y表示年,M表示月,d表示日
LocalDateTime格式化
LocalDateTime.now().format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"))
分割字符串
根据"-"分割字符串
payMonthpayMonth.split("-");
把前台字符串(%9837297423%89374%irdgo%783)转换为中文
new String(URLDecoder.decode(getPara(2),"utf-8"));
搜索某个字符在整个字符串的下标位置
注:下标从0开始
str.indexOf(String string);
返回值为-1则表示str中没有string这个字符
截取第N次出现的字符串
public static int getCharacterPosition(String string , int i){
//这里是获取"/"符号的位置
Matcher slashMatcher = Pattern.compile("/").matcher(string);
int mIdx = 0;
while(slashMatcher.find()) {
mIdx++;
//当"/"符号第三次出现的位置
if(mIdx == i){
break;
}
}
return slashMatcher.start();
}
判断两个值是否相等
object.equals(object)
字符串非空判断
StringUtils.isNotBlank(string);
此方法等于(string!=null)
Stringutils.isNotEmpty(string);
此方法等于(string!=null && string!= “”)
修改list的某个下标的值
list.set(index,value)
判断对象类型
object1 instanceof Integer
时间加减
Date date = new Date();//获取当前时间
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.add(Calendar.YEAR, -1);//当前时间减去一年,即一年前的时间
calendar.add(Calendar.MONTH, -1);//当前时间前去一个月,即一个月前的时间
calendar.getTime();//获取一年前的时间,或者一个月前的时间
查询系统变量
Map<String, String> getenv = System.getenv();
返回参数解释
USERPROFILE | 用户目录 |
---|---|
USERDNSDOMAIN | 用户域 |
PATHEXT | 可执行后缀 |
JAVA_HOME | Java安装目录 |
TEMP | 用户临时文件目录 |
SystemDrive | 系统盘符 |
ProgramFiles | 默认程序目录 |
USERDOMAIN | 帐户的域的名称 |
ALLUSERSPROFILE | 用户公共目录 |
SESSIONNAME | Session名称 |
TMP | 临时目录 |
Path | path环境变量 |
CLASSPATH | classpath环境变量 |
PROCESSOR_ARCHITECTURE | 处理器体系结构 |
os | 操作系统 |
PROCESSOR_LEVEL | 处理级别 |
COMPUTERNAME | 计算机名 |
Windir | 系统安装目录 |
SystemRoot | 系统启动目录 |
USERNAME | 用户名 |
ComSpec | 命令行解释器可执行程序的准确路径 |
APPDATA | 应用程序数据目录 |
前台传回一串HTML字符串,后台截取fieldname内的值
String string="<input name='NEWFIELD' type='text' title='文本框' value='' fieldname='aaaaa' plugins='text' orghide='0' fieldflow='0' orgalign='left' orgwidth='150' orgtype='text' style='text-align: left; width: 150px;'/><span plugins='radios' title='单选' name='NEWFIELD' fieldname='ddd' fieldflow='0'><input name='NEWFIELD' value='男' type='radio'/>男 <input name='NEWFIELD' value='女' type='radio'/>女</span><textarea title='多行文本框' name='NEWFIELD' plugins='textarea' value='' fieldname='bbb' fieldflow='0' orgrich='0' orgfontsize='' orgwidth='300' orgheight='80' style='width:300px;height:80px;'></textarea> <select name='NEWFIELD' title='下拉菜单' plugins='select' size='1' fieldname='ccc' fieldflow='0' orgwidth='150' style='width: 150px;'><option value='男'></option><lect> <span plugins='checkboxs' title='复选框' fieldname='hhh' fieldflow='0'><input name='newcheckbox' value='g' type='checkbox' fieldname='_1'/>g </span>";
String sb=new String();
List<String> create=new ArrayList<>();
int k=0;
String s2="";
String s3="";
for(int i=0;i<=string.length();i++){
if(i==0){
sb=string.substring(string.indexOf("fieldname='"));
System.out.println("进去了");
}
else {
System.out.println(sb);
sb=sb.substring(sb.indexOf("fieldname='"));
System.out.println("出来了");
}
s3=sb.substring(sb.indexOf("'")+1);
sb=sb.substring(sb.indexOf("'")+1);
s3=sb.substring(0,sb.indexOf("'"));
create.add(s3);
}
System.out.println(create);
获取随机四位整数
(int)((Math.random()*9+1)*1000)
随机生成指定范围的整数
Random random = new Random();
int randomNum = random.nextInt(61);
遍历map
for (Map.Entry<String, String> entry : result.entrySet()) {
System.out.println("Key = " + entry.getKey() + ", Value = " + entry.getValue());
}
map.putIfAbsent(object a,object b)作用
如果a非空,则不存入b;如果a为空,则存入b
参数按照ascⅡ表排序
StringBuffer notGenerate = new StringBuffer();
final LinkedList<String> paramMapKeyList = new LinkedList<>();
for (Map.Entry<String, Object> entry : paramMap.entrySet()) {
if (!entry.getKey().equals("sign")) {
paramMapKeyList.add(entry.getKey());
}
}
//排序
paramMapKeyList.sort(String.CASE_INSENSITIVE_ORDER);
for (String paramMapKey : paramMapKeyList) {
for (Map.Entry<String, Object> entry : paramMap.entrySet()) {
if (entry.getKey().equals(paramMapKey)) {
notGenerate.append(entry.getKey().toLowerCase());
if (entry.getValue() != null) {
if (entry.getValue() instanceof String) {
notGenerate.append(entry.getValue());
} else if (entry.getValue() instanceof Integer) {
notGenerate.append(entry.getValue());
} else {
notGenerate.append(JSONObject.toJSONString(entry.getValue()));
}
}
}
}
}
大小写转换
String test="SHA34cccddee";
System.out.println(test.toUpperCase());//小写转大写
String test="SHA34cccddee";
System.out.println(test.toLowerCase());//大写转小写
json字符串转javabean对象
new GsonBuilder().create().fromJson(data,GaodeProvinceJson.class);
腾讯签名获取方法
private final static String CHARSET = "UTF-8";
public static String sign(String s, String key, String method) throws Exception {
Mac mac = Mac.getInstance(method);
SecretKeySpec secretKeySpec = new SecretKeySpec(key.getBytes(CHARSET), mac.getAlgorithm());
mac.init(secretKeySpec);
byte[] hash = mac.doFinal(s.getBytes(CHARSET));
return DatatypeConverter.printBase64Binary(hash);
}
public static String getStringToSign(TreeMap<String, Object> params) {
StringBuilder s2s = new StringBuilder("GETcvm.tencentcloudapi.com/?");
// 签名时要求对参数进行字典排序,此处用TreeMap保证顺序
for (String k : params.keySet()) {
s2s.append(k).append("=").append(params.get(k).toString()).append("&");
}
return s2s.toString().substring(0, s2s.length() - 1);
}
public static String getUrl(TreeMap<String, Object> params) throws UnsupportedEncodingException {
StringBuilder url = new StringBuilder("https://cvm.tencentcloudapi.com/?");
// 实际请求的url中对参数顺序没有要求
for (String k : params.keySet()) {
// 需要对请求串进行urlencode,由于key都是英文字母,故此处仅对其value进行urlencode
url.append(k).append("=").append(URLEncoder.encode(params.get(k).toString(), CHARSET)).append("&");
}
return url.toString().substring(0, url.length() - 1);
}
public static void main(String[] args) throws Exception {
TreeMap<String, Object> params = new TreeMap<String, Object>(); // TreeMap可以自动排序
// 实际调用时应当使用随机数,例如:params.put("Nonce", new Random().nextInt(java.lang.Integer.MAX_VALUE));
params.put("Nonce", new Random().nextInt(Integer.MAX_VALUE)); // 公共参数
// 实际调用时应当使用系统当前时间,例如: params.put("Timestamp", System.currentTimeMillis() / 1000);
params.put("Timestamp", System.currentTimeMillis()/1000); // 公共参数
params.put("SecretId", "AKIDY0gIm8BQOEDdx7B2qX6WZYQDsywkPDtQ"); // 公共参数
params.put("Action", "DescribeInstances"); // 公共参数
params.put("Version", "2017-03-12"); // 公共参数
params.put("Region", "ap-guangzhou"); // 公共参数
params.put("Limit", 20); // 业务参数
params.put("Offset", 0); // 业务参数
params.put("InstanceIds.0", "ins-09dx96dg"); // 业务参数
params.put("Signature", sign(getStringToSign(params), "c7Wowx0xrdGk5GItpeLTUQGxVvFPm2BB", "HmacSHA1")); // 公共参数,第一个为SecretKey
System.out.println(getUrl(params));
}
微信手机号解密
pom
<dependency>
<groupId>org.bouncycastle</groupId>
<artifactId>bcprov-jdk15on</artifactId>
<version>1.57</version>
</dependency>
解密工具类
package com.adp.api.utils;
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import org.bouncycastle.util.Arrays;
import org.bouncycastle.util.encoders.Base64;
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.security.Key;
import java.security.Security;
public class WxUtil {
public static String getPhoneNumber(String encryptDataB64, String sessionKeyB64, String ivB64) {
return new String(
decryptOfDiyIV(
Base64.decode(encryptDataB64),
Base64.decode(sessionKeyB64),
Base64.decode(ivB64)
)
);
}
private static final String KEY_ALGORITHM = "AES";
private static final String ALGORITHM_STR = "AES/CBC/PKCS7Padding";
private static Key key;
private static Cipher cipher;
private static void init(byte[] keyBytes) {
// 如果密钥不足16位,那么就补足. 这个if 中的内容很重要
int base = 16;
if (keyBytes.length % base != 0) {
int groups = keyBytes.length / base + (keyBytes.length % base != 0 ? 1 : 0);
byte[] temp = new byte[groups * base];
Arrays.fill(temp, (byte) 0);
System.arraycopy(keyBytes, 0, temp, 0, keyBytes.length);
keyBytes = temp;
}
// 初始化
Security.addProvider(new BouncyCastleProvider());
// 转化成JAVA的密钥格式
key = new SecretKeySpec(keyBytes, KEY_ALGORITHM);
try {
// 初始化cipher
cipher = Cipher.getInstance(ALGORITHM_STR, "BC");
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 解密方法
*
* @param encryptedData 要解密的字符串
* @param keyBytes 解密密钥
* @param ivs 自定义对称解密算法初始向量 iv
* @return 解密后的字节数组
*/
private static byte[] decryptOfDiyIV(byte[] encryptedData, byte[] keyBytes, byte[] ivs) {
byte[] encryptedText = null;
init(keyBytes);
try {
cipher.init(Cipher.DECRYPT_MODE, key, new IvParameterSpec(ivs));
encryptedText = cipher.doFinal(encryptedData);
} catch (Exception e) {
e.printStackTrace();
}
return encryptedText;
}
}
加密算法
AES加密算法解密
/**
*
* 解密用户分享群信息
*
* 对称解密使用的算法为 AES-128-CBC,数据采用PKCS#7填充。
* 对称解密的目标密文为 Base64_Decode(encryptedData)。
* 对称解密秘钥 aeskey = Base64_Decode(session_key), aeskey 是16字节。
* 对称解密算法初始向量 为Base64_Decode(iv),其中iv由数据接口返回。
*
* @param encryptedData 包括敏感数据在内的完整用户信息的加密数据
* @param key 数据进行加密签名的密钥
* @param iv 加密算法的初始向量
* @return
*/
public JSONObject decodeGroupInfo(String encryptedData,String key, String iv){
if(null != encryptedData && null != key && null != iv) {
// 被加密的数据
byte[] dataByte = Base64.decode(encryptedData);
// 加密秘钥
byte[] keyByte = Base64.decode(key);
// 偏移量
byte[] ivByte = Base64.decode(iv);
try {
// 如果密钥不足16位,那么就补足. 这个if 中的内容很重要
int base = 16;
if (keyByte.length % base != 0) {
int groups = keyByte.length / base + (keyByte.length % base != 0 ? 1 : 0);
byte[] temp = new byte[groups * base];
Arrays.fill(temp, (byte) 0);
System.arraycopy(keyByte, 0, temp, 0, keyByte.length);
keyByte = temp;
}
// 初始化
Security.addProvider(new BouncyCastleProvider());
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS7Padding","BC");
SecretKeySpec spec = new SecretKeySpec(keyByte, "AES");
AlgorithmParameters parameters = AlgorithmParameters.getInstance("AES");
parameters.init(new IvParameterSpec(ivByte));
cipher.init(Cipher.DECRYPT_MODE, spec, parameters);// 初始化
byte[] resultByte = cipher.doFinal(dataByte);
if (null != resultByte && resultByte.length > 0) {
String result = new String(resultByte, "UTF-8");
return JSONObject.parseObject(result);
}
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
} catch (NoSuchPaddingException e) {
e.printStackTrace();
} catch (InvalidParameterSpecException e) {
e.printStackTrace();
} catch (IllegalBlockSizeException e) {
e.printStackTrace();
} catch (BadPaddingException e) {
e.printStackTrace();
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
} catch (InvalidKeyException e) {
e.printStackTrace();
} catch (InvalidAlgorithmParameterException e) {
e.printStackTrace();
} catch (NoSuchProviderException e) {
e.printStackTrace();
}
return null;
}else {
return null;
}
}
byte+1加密
String ss = "hhhjjjkkk";
final String[] split = ss.split("");
final StringBuffer stringBuffer = new StringBuffer();
for (int i = 0; i < split.length; i += 1) {
final byte[] bytes = split[i].getBytes();
for (int k = 0; k < bytes.length; k++) {
bytes[k] = (bytes[k] -= i);
}
split[i] = new String(bytes);
stringBuffer.append(split[i]);
}
System.out.println(stringBuffer.toString());
final StringBuffer decryptStringBuffer = new StringBuffer();
final String[] decryptSplit = stringBuffer.toString().split("");
for (int i = 0; i < decryptSplit.length; i++) {
final byte[] bytes = decryptSplit[i].getBytes();
for (int k = 0; k < bytes.length; k++) {
bytes[k] = (bytes[k] += i);
}
decryptStringBuffer.append(new String(bytes));
}
System.out.println(decryptStringBuffer.toString());
DES加密
加密成密文
private final static String SECRET_KEY_INTANCE_DESC="DES";
/**
* des加密
* @param str 待加密的字符串
* @param key 密钥
* @return 加密后的字符串
*/
public static String encryption(String str, String key){
key = DigestUtils.md5DigestAsHex(key.getBytes());
final SecureRandom secureRandom = new SecureRandom();
try {
//创建密钥规则
final DESKeySpec desKey = new DESKeySpec(key.getBytes());
//创建加密工厂
final SecretKeyFactory secretKeyFactory = SecretKeyFactory.getInstance("DESC");
//按照密钥规则生成密钥
final SecretKey secretKey = secretKeyFactory.generateSecret(desKey);
//加密对象
final Cipher cipher = Cipher.getInstance("DES");
//初始化加密对象需要的属性
cipher.init(Cipher.ENCRYPT_MODE,secretKey,secureRandom);
//开始加密
final byte[] result = cipher.doFinal(str.getBytes());
return new BASE64Encoder().encode(result);
}catch (Exception e){
e.printStackTrace();
}
return null;
}
解密
private final static String SECRET_KEY_INTANCE_DESC="DES";
public static String decrypt(String str, String key){
key = DigestUtils.md5DigestAsHex(key.getBytes());
final SecureRandom secureRandom = new SecureRandom();
try {
//创建密钥规则
final DESKeySpec keySpec = new DESKeySpec(key.getBytes());
//创建加密工厂
final SecretKeyFactory secretKeyFactory = SecretKeyFactory.getInstance("DESC");
//按照密钥规则生成密钥
final SecretKey secretKey = secretKeyFactory.generateSecret(keySpec);
//加密对象
final Cipher cipher = Cipher.getInstance("DESC");
//初始化加密对象需要的属性
cipher.init(Cipher.DECRYPT_MODE, secretKey, secureRandom);
//base64对
final byte[] result = new BASE64Decoder().decodeBuffer(str);
final byte[] bytes = cipher.doFinal(result);
return new String(bytes);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
生成字符串
生成随机APPID
StringBuffer shortBuffer = new StringBuffer();
String uuid = UUID.randomUUID().toString().replace("-", "");
for (int i = 0; i < 8; i++) {
String str = uuid.substring(i * 4, i * 4 + 4);
int x = Integer.parseInt(str, 16);
shortBuffer.append(chars[x % 0x3E]);
}
return shortBuffer.toString();
生成密钥
try {
String[] array = new String[]{appId, SERVER_NAME};
StringBuffer sb = new StringBuffer();
// 字符串排序
Arrays.sort(array);
for (int i = 0; i < array.length; i++) {
sb.append(array[i]);
}
String str = sb.toString();
MessageDigest md = MessageDigest.getInstance("SHA-1");
md.update(str.getBytes());
byte[] digest = md.digest();
StringBuffer hexstr = new StringBuffer();
String shaHex = "";
for (int i = 0; i < digest.length; i++) {
shaHex = Integer.toHexString(digest[i] & 0xFF);
if (shaHex.length() < 2) {
hexstr.append(0);
}
hexstr.append(shaHex);
}
return hexstr.toString();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
throw new RuntimeException();
}将二进制转成16进制
生成密码
private static final byte INDEX_NUMBER = 0;
private static final byte INDEX_LETTER = 1;
private static final byte INDEX_SPECIAL_CHAR = 2;
/** 特殊符号 */
private static final char[] SPECIAL_CHARS = { '`', '~', '!', '@', '#', '$', '%', '^', '&', '*', '(', ')', '-', '_', '=', '+', '[', ']', '{', '}', '\\', '|', ';', ':', '\'', '"', ',', '<', '.', '>', '/', '?' };
/**
* 按一定的概率生成一个随机的N位(N>=3)密码,必须由字母数字特殊符号组成,三者缺一不可
*
* @param len
* 密码长度,必须大于等于3
* @param genChances
* 分别是生成数字、字母、特殊符号的概率
* @return 生成的随机密码
*/
private static char[] generateRandomPassword(final int len, final byte[] paramGenChances) throws IllegalArgumentException {
if (len < 3) {
throw new IllegalArgumentException("len must not smaller than 3, but now is " + len);
}
final char[] password = new char[len];
// 之所以该复制一份是为了使函数不对外产生影响
final byte[] genChances = paramGenChances.clone();
final byte[] genNums = new byte[genChances.length];
for (byte i = 0; i < genChances.length; i++) {
genNums[i] = 0;
}
final Random random = new Random();
int r;
for (int i = 0; i < len; i++) {
adjustGenChance(len, i, genChances, genNums);
byte index = getPasswordCharType(random, genChances);
genNums[index]++;
switch (index) {
case INDEX_NUMBER:
password[i] = (char) ('0' + random.nextInt(10));
break;
case INDEX_LETTER:
r = random.nextInt(52);
if (r < 26) {
password[i] = (char) ('A' + r);
} else {
password[i] = (char) ('a' + r - 26);
}
break;
case INDEX_SPECIAL_CHAR:
r = random.nextInt(SPECIAL_CHARS.length);
password[i] = SPECIAL_CHARS[r];
break;
default:
password[i] = ' ';
break;
}
}
logChances(genNums);
return password;
}
/**
* 根据当前需要生成密码字符的位置,动态调整生成概率
*
* @param len
* 待生成的总长度
* @param index
* 当前位置
* @param genChances
* 分别是生成数字、字母、特殊符号的概率
* @param genNums
* 这些类型已经生成过的次数
*/
private static void adjustGenChance(final int len, final int index, final byte[] genChances, final byte[] genNums) {
final int leftCount = len - index;
byte notGenCount = 0;
for (byte i = 0; i < genChances.length; i++) {
if (genNums[i] == 0) {
notGenCount++;
}
}
if (notGenCount > 0 && leftCount < genChances.length && leftCount == notGenCount) {
for (byte i = 0; i < genChances.length; i++) {
if (genNums[i] > 0) {
genChances[i] = 0;
}
}
}
}
/**
* 获取该密码字符的类型
*
* @param random
* 随机数生成器
* @param genChances
* 分别是生成数字、字母、特殊符号的概率
* @return 密码字符的类型
*/
private static byte getPasswordCharType(final Random random, final byte[] genChances) {
int total = 0;
byte i = 0;
for (; i < genChances.length; i++) {
total += genChances[i];
}
int r = random.nextInt(total);
for (i = 0; i < genChances.length; r -= genChances[i], i++) {
if (r < genChances[i]) {
break;
}
}
return i;
}
public static void main(String[] args) {
test1();
test2();
}
/**
* 打印生成密码中各类字符的个数
*/
private static void logChances(byte[] genNums) {
StringBuilder sb = new StringBuilder();
sb.append("{");
for (byte i = 0; i < genNums.length; i++) {
sb.append(genNums[i]);
if (i != genNums.length - 1) {
sb.append(", ");
}
}
sb.append("}");
System.out.println(sb.toString());
}
private static void test1() {
byte[] genChances = { 1, 1, 1 };
for (int i = 3; i < 200; i += 30) {
try {
char[] password = generateRandomPassword(i, genChances);
System.out.println(password);
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
}
System.out.println();
}
private static void test2() {
byte[] genChances = { 2, 5, 3 };
for (int i = 3; i < 200; i += 30) {
try {
char[] password = generateRandomPassword(i, genChances);
System.out.println(password);
} catch (IllegalArgumentException e) {
e.printStackTrace();
}
}
System.out.println();
}
转换进制
将二进制转成16进制
StringBuffer sb = new StringBuffer();
for (int i = 0; i < buf.length; i++) {
String hex = Integer.toHexString(buf[i] & 0xFF);
if (hex.length() == 1) {
hex = '0' + hex;
}
sb.append(hex.toUpperCase());
}
return sb.toString();
将16进制转为2进制
if (hexStr.length() < 1)
return null;
byte[] result = new byte[hexStr.length() / 2];
for (int i = 0; i < hexStr.length() / 2; i++) {
int high = Integer.parseInt(hexStr.substring(i * 2, i * 2 + 1), 16);
int low = Integer.parseInt(hexStr.substring(i * 2 + 1, i * 2 + 2), 16);
result[i] = (byte) (high * 16 + low);
}
return result;
计算指数(i的n次方)
new BigDecimal(10).pow(i).longValue()
bigdecimal
加减乘除基本使用
//加法
bignum3 = bignum1.add(bignum2);
System.out.println("和 是:" + bignum3);
//减法
System.out.println("差 是:" + bignum3);
//乘法
bignum3 = bignum1.multiply(bignum2);
System.out.println("积 是:" + bignum3);
//除法
bignum3 = bignum1.divide(bignum2);
System.out.println("商 是:" + bignum3);
//比较
int i=a.compareTo(b);
// i<0——>a比b小
// i=0——>a与b相等
// i>0——>a比b大
//判断是否为零
tradeRule.getSingleMinCommission().equals(BigDecimal.ZERO)
舍入模式
CEILING | 向正无限大方向舍入。 |
---|---|
DOWN | 向零方向舍入。 |
FLOOR | 向负无限大方向舍入。 |
HALF_DOWN | 向最接近数字方向舍入,如果与两个相邻数字的距离相等,则向下舍入。 |
HALF_EVEN | 向最接近数字方向舍入,如果与两个相邻数字的距离相等,则向相邻的偶数舍入。 |
HALF_UP | 向最接近数字方向舍入,如果与两个相邻数字的距离相等,则向上舍入。 |
UNNECESSARY | 断言具有精确结果。 |
使用
new BigDecimal("10").divide(new BigDecimal("3"),3, RoundingMode.FLOOR).doubleValue();//第一个参数是除数,第二个参数是保留位数,第三个参数是舍入模式常量
注意
1、所得结果无限小数,例如——10/3,结果为3.3333333,该结果无限故报此错误
Non-terminating decimal expansion; no exact representable decimal result
解决方案
divide方法内传参舍入模式,如
new BigDecimal("10").divide(new BigDecimal("3"), RoundingMode.HALF_EVEN).doubleValue()
匹配星号
private boolean xinghao(String str, String regx) {
int strLen = str.length();
int regxLen = regx.length();
int regxIndex = regx.indexOf("*");
switch (regxIndex) {
//如果regx不存在星号,则直接匹配str与regx是否相等
case -1:
if (regx.equals(str)) {
return true;
} else {
return false;
}
//regx第一个符号是星号
case 0:
//regx的长度为1,直接返回true
if(regxLen==1){
return true;
}
boolean isMatch = false;
int p=0;
//循环匹配str每个与regx的第二个字符是否相等,并记录相等字符的位数
for (int i = 0; i < strLen; i++) {
if ( str.charAt(i)==regx.charAt(regxIndex + 1) || regx.charAt(regxIndex + 1) == '*') {
isMatch=true;
p=i;
break;
}
}
//假如str每个字符与regx第二个字符不相等,则返回false
if (!isMatch) {
return false;
} else {
//假如str与regx相等
//如果str与regx相等位数=str的长度,直接返回true
if(p==strLen){
return true;
}
//str=截取str与regx的位数到str长度位置,regx=星号后一位到regx长度的位置
return xinghao(str.substring(p,strLen),regx.substring(regxIndex+1,regxLen));
}
//regx的*的位置大于等于1
default:
//循环判断str每个字符是否等于regx到*号之前的字符,如果循环中有一个不匹配则立刻返回false
for(int i=0;i<regxIndex;i++){
if(str.charAt(i)!=regx.charAt(i)){
return false;
}
}
//上述循环都相等,则str截取从*后到str的长度之间的字符,regx截取*后到regx长度之间的字符
return xinghao(str.substring(regxIndex,strLen),regx.substring(regxIndex,regxLen));
}
}
反射
第一种方法
Shop shop = super.getShopService().selectByPrimaryKey(Integer.valueOf(param.getId()));
Field[] fields = shop.getClass().getDeclaredFields();
LinkedMap linkedMap = new LinkedMap();
for (Field field : fields) {
String name=field.getName();
name = name.substring(0,1).toUpperCase()+name.substring(1);
linkedMap.put(field.getName(), shop.getClass().getMethod("get"+ name).invoke(shop));
}
linkedMap.put("attention", super.getShopAttentionService().isAttention(user.getId(), shop.getId()));
第二种方法
Field[] fields = obj.getClass().getDeclaredFields();
for(Field field:fields){
String fieldName = field.getName();
field.setAccessible(true);//私有属性设置访问权限
System.out.println(fieldName+":"+field.get(obj));
}
反射调用setter
ExternalOperationParam param = new ExternalOperationParam();
Method[] methods = param.getClass().getMethods();
for (Method method : methods) {
System.out.println(method.getName() + ":" + method.getName().indexOf("set"));
System.out.println(method.getName().substring(3));
if (method.getName().indexOf("set") >= 0) {
//getMethod(),第一个参数为方法名,第二个及之后的参数为参数列表类型
Method met = param.getClass().getMethod("set" + method.getName().substring(3), String.class);
//invoke(),调用方法
met.invoke(param, map.get(method.getName().substring(3)));
}
}
反射赋值
Test t = new Test();
Field f = t.getClass().getDeclaredField("name");
f.setAccessible(true);
f.set(t, "this is test1");
System.out.println(t.getName());
复制对象(注:两个对象的成员名相同的类型必须一致)
/**
* 复制属性,从source复制到end
*
* @param source 复制类
* @param end 目标类
*/
public static void copyObj(Object source, Object end) throws IntrospectionException, InvocationTargetException,
IllegalAccessException {
Method[] sourceMethods = source.getClass().getMethods();
Field[] sourceFields = source.getClass().getDeclaredFields();//获取source所有属性值
Method[] endMethods = end.getClass().getMethods();
Field[] endFields = end.getClass().getDeclaredFields();//获取end所有属性值
for (Field field : sourceFields) {
PropertyDescriptor endDescriptor = new PropertyDescriptor(field.getName(), end.getClass());
Method endDescriptorWriteMethod = endDescriptor.getWriteMethod();
PropertyDescriptor sourceDescriptor = new PropertyDescriptor(field.getName(), source.getClass());
Method sourceDescriptorReadMethod = sourceDescriptor.getReadMethod();
if (endDescriptorWriteMethod!=null){
endDescriptorWriteMethod.invoke(end,sourceDescriptorReadMethod.invoke(source));
}
}
}
获取包名下的所有类
Reflections
Reflections reflections = new Reflections("com.dcfs.smartaibank.video.qc.property");
Set<Class<? extends QcBaseConfig>> baseConfigSet = reflections.getSubTypesOf(QcBaseConfig.class);
ArrayList<QcBaseConfig> qcBaseConfigs = new ArrayList<>();
try {
for (Class<? extends QcBaseConfig> baseConfig : baseConfigSet) {
if(!baseConfig.isInterface()){//如果不是接口的话
QcBaseConfig qcBaseConfig = baseConfig.newInstance();
}
}
} catch (InstantiationException | IllegalAccessException | NoSuchFieldException e) {
e.printStackTrace();
}
System.out.println(qcBaseConfigs);
复制对象(克隆对象)
package org.springframework.beans中的BeanUtils.copyProperties(A,B)
是A中的值赋给B
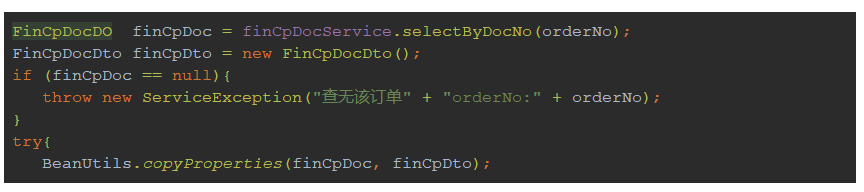
package org.apache.commons.beanutils的BeanUtils.copyProperties(A,B)
是B中的值付给A
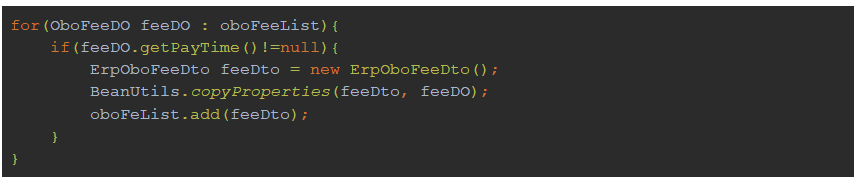
beancopier复制
final BeanCopier copier1 = BeanCopier.create(matchInfo.getClass(), matchInfo2.getClass(), false);
copier1.copy(matchInfo, matchInfo2, null);//第一个为源对象,第二个为目标对象
okhttp使用
实例化客户端
private final static OkHttpClient OK_HTTP_CLIENT=new OkHttpClient();
get请求
final Request request = new Request.Builder()
.url("http://127.0.0.1:8848/nacos/v1/cs/configs?dataId=nacos.cfg.dataId&group=test")
.get()
.build();
post请求
表单请求
RequestBody requestBody = new FormBody.Builder()
.add("search", "Jurassic Park")
.build();
Request request = new Request.Builder()
.url("https://en.wikipedia.org/w/index.php")
.post(requestBody)
.build();
发送请求并接收响应
final Response response = OK_HTTP_CLIENT.newCall(request).execute();
return response.body().string();
Jackson
基本使用
实例化ObjectMapper
final private ObjectMapper OBJECT_MAPPER=new ObjectMapper();
Map(实体类)转json
OBJECT_MAPPER.convertValue(entry.getValue(), ZuulRouteEntity.class);
json转实体类
OBJECT_MAPPER.readValue("aa",ZuulRouteEntity.class)
单引号转实体类
添加策略JsonParser.Feature.ALLOW_SINGLE_QUOTES
OBJECT_MAPPER.config(JsonParser.Feature.ALLOW_SINGLE_QUOTES,true)
json转list
Arrays.asList(OBJECT_MAPPER.readValue(config,ZuulRouteEntity[].class))
添加转换策略
try{
objectMapper.setPropertyNamingStrategy(PropertyNamingStrategy.KEBAB_CASE);
}
finally{
objectMapper.setPropertyNamingStrategy(null)
}
转换策略总览
策略名 | java对象成员 | 转化后的属性名 |
---|---|---|
CamelCase | personId | persionId |
PascalCase | personId | PersonId |
SnakeCase | personId | person_id |
KebabCase | personId | person-id |
只比较时分秒
jdk8之前
java.sql.Time d1 = new java.sql.Time(10, 10, 10); //时,分,秒
java.sql.Time d2 = new java.sql.Time(11, 9, 45);
int i = d1.compareTo(d2);
i > 0 说明d1大
i=0说明 d1==d2
jdk8之后
/**
* 两个时间对比大小
*
* @param compareTime 时间1
* @param nowTime 时间2
* @return true=时间2比较大,false=时间1比较大
*/
@Override
public boolean checkNowTimeCompareTradeTime(Date compareTime, Date nowTime) {
TsTradeRule tradeRule = this.redisGetTrade();
//待比较时间
Calendar compareDateTime = new Calendar.Builder().setInstant(tradeRule.getAfternoonTradeStarttime()).build();
Calendar nowDateTime = new Calendar.Builder().setInstant(nowTime).build();
//当前时间
LocalTime nowLocalTime = LocalTime.of(nowDateTime.get(Calendar.HOUR_OF_DAY), nowDateTime.get(Calendar.MINUTE), nowDateTime.get(Calendar.SECOND));
LocalTime compareDateTimeLocalTime = LocalTime.of(compareDateTime.get(Calendar.HOUR_OF_DAY), compareDateTime.get(Calendar.MINUTE), compareDateTime.get(Calendar.SECOND));
return nowLocalTime.compareTo(compareDateTimeLocalTime) > 0;
}
true=时间2比较大,false=时间1比较大
不够位数自动往前补零
private static String autoGenericCode(String code, int num) {
String result = String.format("%0" + num + "d", Integer.parseInt(code) + 1);
return result;
}
解析xml文件
dom解析
package com.cxx.xml;
import org.w3c.dom.*;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
/**
* @Author: cxx
* Dom操作xml
* @Date: 2018/5/29 20:19
*/
public class DomDemo {
//用Element方式
public static void element(NodeList list){
for (int i = 0; i <list.getLength() ; i++) {
Element element = (Element) list.item(i);
NodeList childNodes = element.getChildNodes();
for (int j = 0; j <childNodes.getLength() ; j++) {
if (childNodes.item(j).getNodeType()==Node.ELEMENT_NODE) {
//获取节点
System.out.print(childNodes.item(j).getNodeName() + ":");
//获取节点值
System.out.println(childNodes.item(j).getFirstChild().getNodeValue());
}
}
}
}
public static void node(NodeList list){
for (int i = 0; i <list.getLength() ; i++) {
Node node = list.item(i);
NodeList childNodes = node.getChildNodes();
for (int j = 0; j <childNodes.getLength() ; j++) {
if (childNodes.item(j).getNodeType()==Node.ELEMENT_NODE) {
System.out.print(childNodes.item(j).getNodeName() + ":");
System.out.println(childNodes.item(j).getFirstChild().getNodeValue());
}
}
}
}
public static void main(String[] args) {
//1.创建DocumentBuilderFactory对象
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
//2.创建DocumentBuilder对象
try {
DocumentBuilder builder = factory.newDocumentBuilder();
Document d = builder.parse("src/main/resources/demo.xml");
NodeList sList = d.getElementsByTagName("student");
//element(sList);
node(sList);
} catch (Exception e) {
e.printStackTrace();
}
}
}
dom4j方式
pom
<!-- https://mvnrepository.com/artifact/org.dom4j/dom4j -->
<dependency>
<groupId>org.dom4j</groupId>
<artifactId>dom4j</artifactId>
<version>2.1.1</version>
</dependency>
实现
import org.dom4j.*;
public class Test {
public static void main(String[] args) {
try {
Document document = DocumentHelper.parseText("aaa");
//获取element子元素
Element batchId = document.getRootElement().element("batchId").element("sdfsdf");
//获取属性
Attribute aaa = batchId.attribute("aaa");
//获取属性值
batchId.attributeValue("aaa");
} catch (DocumentException e) {
e.printStackTrace();
}
}
}
HttpUrlConnection调用外部接口
public static String postUrl(String urlStr, String json) {
try {
//请求
URL url = new URL(urlStr);
HttpsURLConnection connection = (HttpsURLConnection) url.openConnection();
//后续可以用connection.inputStream()
connection.setDoInput(true);
//后续可以用connection.outputStream()传输json或者表单数据
connection.setDoOutput(true);
//设置请求方法
connection.setRequestMethod(HttpMethod.POST.name());
//添加header
connection.setRequestProperty("Content-Type", "application/json");
connection.setUseCaches(false);
//连接服务器超时时间
connection.setConnectTimeout(1000 * 10);
//从服务器获取响应超时时间
connection.setReadTimeout(1000 * 30);
//是否自动处理重定向
connection.setInstanceFollowRedirects(true);
connection.connect();
//转换json
DataOutputStream outputStream = new DataOutputStream(connection.getOutputStream());
outputStream.write(json.getBytes());
outputStream.close();
//响应
InputStream inputStream = null;
BufferedReader reader = null;
inputStream = connection.getInputStream();
reader = new BufferedReader(new InputStreamReader(inputStream));
String lines;
StringBuffer sb = new StringBuffer();
while ((lines = reader.readLine()) != null) {
lines = new String(lines.getBytes(), StandardCharsets.UTF_8);
sb.append(lines);
}
return sb.toString();
} catch (MalformedURLException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
根据图片url流下载图片到本地并返回文件地址
/**
* 请求别人的图片url地址,获得文件流
*
* @param imgUrl
* @return
*/
public static InputStream getInputStream(String imgUrl) {
InputStream inputStream = null;
HttpURLConnection httpURLConnection = null;
try {
URL url = new URL(imgUrl);
httpURLConnection = (HttpURLConnection)url.openConnection();
// 设置网络连接超时时间
httpURLConnection.setConnectTimeout(3000);
// 设置应用程序要从网络连接读取数据
httpURLConnection.setDoInput(true);
httpURLConnection.setRequestMethod("GET");
int responseCode = httpURLConnection.getResponseCode();
if (responseCode == 200) {
// 从服务器返回一个输入流
inputStream = httpURLConnection.getInputStream();
}
} catch (MalformedURLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return inputStream;
}
base64
base64转问文件
文件转base64
public static String file2Base64(File file) {
if(file==null) {
return null;
}
String base64 = null;
FileInputStream fin = null;
try {
fin = new FileInputStream(file);
byte[] buff = new byte[fin.available()];
fin.read(buff);
base64 = Base64.encode(buff);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fin != null) {
try {
fin.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
return base64;
}