引入依赖
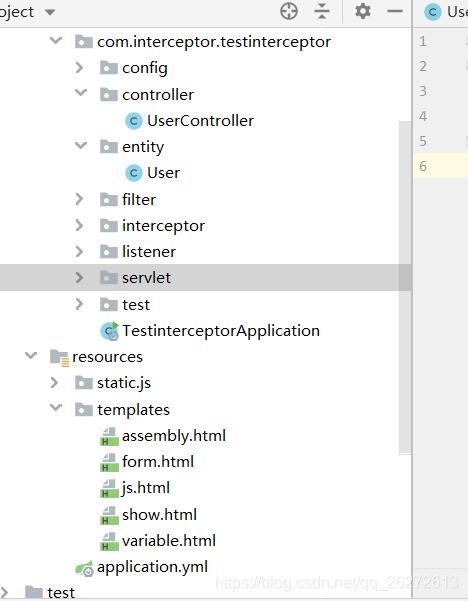
<!--添加thymeleaf依赖-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!--引入验证依赖-->
<dependency>
<groupId>org.hibernate.validator</groupId>
<artifactId>hibernate-validator</artifactId>
<version>6.0.18.Final</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.12</version>
</dependency>
application.yml
spring:
thymeleaf:
cache: false # 禁用缓存(建议开发关闭,生产环境开启)
prefix: classpath:/templates/ # 配置静态模板引擎的位置
suffix: .html # 配置后缀名称
UserController
package com.interceptor.testinterceptor.controller;
import com.interceptor.testinterceptor.entity.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.ui.ModelMap;
import org.springframework.validation.BindingResult;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.servlet.ModelAndView;
import javax.validation.Valid;
import java.util.*;
@Controller
@RequestMapping("user")
public class UserController {
@GetMapping("show")
public String test(Model model) {
model.addAttribute("data","模板数据");
return "show";
}
@GetMapping("user")
public ModelAndView user() {
ModelAndView mv = new ModelAndView();
mv.setViewName("variable");
User user = new User();
user.setId(3430L);
user.setName("小雪");
user.setAge(30);
mv.addObject("user",user);
return mv;
}
@GetMapping("add")
public String add() {
return "form";
}
@GetMapping("js")
public String js(ModelMap modelMap) {
modelMap.put("data","js中获取值");
return "js";
}
@PostMapping("save")
public String save(@Valid User user, BindingResult result, ModelMap modelMap) {
//System.out.println(result.getAllErrors().size());
//result.getFieldErrors().stream().forEach(n-> System.out.println(n.getField()+","+n.getDefaultMessage()));
if (result.hasErrors()) {
result.getFieldErrors().stream().forEach(n-> modelMap.put(n.getField(),n.getDefaultMessage()));
modelMap.remove("user");
} else {
modelMap.put("user", user);
}
return "form";
}
@GetMapping("assembly")
public String assembly(Model model) {
// 创建List集合
List<User> userList = Arrays.asList(
new User(10001L,"王五",43),
new User(10002L,"张柳",20),
new User(10003L,"骑闪",24),
new User(10004L,"刘昂",64)
);
// 创建Map集合
Map<Integer, User> userMap = new HashMap<>();
userMap.put(40001, new User(30001L,"王五",43));
userMap.put(40002, new User(30002L,"五阿哥",54));
userMap.put(40003, new User(30003L,"砌墙图",14));
// 创建复杂集合
// 创建复杂集合List->Map->List->User
List<Map<Integer, List<User>>> mapList = new ArrayList<>();
for (int i = 0; i < 2; i++) {
Map<Integer, List<User>> map = new HashMap<>();
for (int i1 = 0; i1 < 2; i1++) {
List<User> users = new ArrayList<>();
for (int i2 = 0; i2 < 3; i2++) {
User user = new User(i2 + 11L, "张三" + i2, 32 + i);
users.add(user);
}
map.put(i1+1, users);
}
mapList.add(map);
}
model.addAttribute("users", userList);
model.addAttribute("userMap", userMap);
model.addAttribute("mapList", mapList);
return "assembly";
}
}
User
package com.interceptor.testinterceptor.entity;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.hibernate.validator.constraints.Range;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class User {
@NotNull(message="ID不能为空")
private Long id;
@Size(max = 15,min = 6,message = "名字长度必须为{min}~{max}之间")
private String name;
@Range(min = 18,max = 80,message = "年龄必须在{min}~{max}之间")
@NotNull(message = "年龄不能为空")
private Integer age;
}
assembly.html
<!DOCTYPE HTML>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<title>集合处理</title>
</head>
<body>
<fieldset>
<legend>List集合</legend>
<div th:each="user,stat:${users}">
编号: <span th:text="${user.id}"></span><br>
姓名:<span th:text="${user.name}"></span><br>
年龄:<span th:text="${user.age}"></span><br>
<hr>
</div>
</fieldset>
<fieldset>
<legend>Map集合</legend>
<div th:each="map,stat:${userMap}">
行号: <span th:text="${stat.count}"></span><br>
编号: <span th:text="${map.value.id}"></span><br>
姓名:<span th:text="${map.value.name}"></span><br>
年龄:<span th:text="${map.value.age}"></span><br>
<hr>
</div>
</fieldset>
<fieldset>
<legend>List->map-list-user复杂集合的变量</legend>
<div th:each="map1:${mapList}">
<div th:each="userList:${map1.values()}">
<div th:each="user:${userList}">
编号: <span th:text="${user.id}"></span><br>
姓名:<span th:text="${user.name}"></span><br>
年龄:<span th:text="${user.age}"></span><br>
<span th:if="${user.age eq 31}">有小孩了吗?</span>
<span th:unless="${user.age eq 31}">谈朋友了吗?</span>
<span th:switch="${user.id}">
<span th:case="11">111</span>
<span th:case="12">112</span>
<span th:case="13">113</span>
</span>
<br/>
------------------------------------------------
</div>
<hr>
</div>
</div>
</fieldset>
</body>
</html>
form.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>表单元素</title>
</head>
<body>
<form method="post" th:action="@{/user/save}">
编号: <input type="text" th:onblur="alert(11111)" th:name="id"> <span style="color:red;font-size: 9pt" th:text="${id}"></span><br/>
姓名: <input type="text" th:name="name"> <span style="color:red;font-size: 9pt" th:text="${name}"></span><br/>
年龄: <input type="text" th:name="age"> <span style="color:red;font-size: 9pt" th:text="${age}"></span><br/>
<input type="submit" th:value="提交">
</form>
<fieldset th:if="${user != null}">
<legend>添加的数据</legend>
编号: <span th:text="${user.id}"></span><br>
姓名:<span th:text="${user.name}"></span><br>
年龄:<span th:text="${user.age}"></span><br>
</fieldset>
</body>
</html>
js.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>JS相关</title>
<script type="text/javascript" th:src="@{/js/jquery-3.3.1.min.js}"></script>
<script type="text/javascript">
$(function(){
alert($("#data").text());
});
</script>
</head>
<body>
<h2>简单引入</h2>
<div th:id="data" th:text="刀刀9999"></div>
<h2>内敛标签</h2>
<fieldset>
<legend>th:inline</legend>
<p th:inline="text">
数据:[[${data}]]
</p>
<p>外部数据:[[${data}]]</p>
</fieldset>
<fieldset>
<legend>内敛脚本:th:javascript</legend>
<script type="text/javascript" th:inline="javascript">
function show() {
alert([[${data}]]);
}
</script>
<div th:onclick="show()"><a href="javascript:;">显示数据</a></div>
</fieldset>
</body>
</html>
show.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>简单应用</title>
</head>
<body>
<div th:text="${data}"></div>
<div th:text="${data}"></div>
<div th:text="${data}"></div>
</body>
</html>
variable.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>变量表达式</title>
</head>
<body>
<h2>对象变量</h2>
<fieldset>
<legend>标准变量表达式${}【推荐使用】</legend>
编号: <span th:text="${user.id}"></span><br>
姓名:<span th:text="${user.name}"></span><br>
年龄:<span th:text="${user.age}"></span><br>
</fieldset>
<fieldset th:object="${user}">
<legend>选择变量表达式*{}</legend>
编号: <span th:text="*{id}"></span><br>
姓名:<span th:text="*{name}"></span><br>
年龄:<span th:text="*{age}"></span><br>
<!--不存在的属性:<span th:text="*{age1}"></span><br>-->
</fieldset>
<fieldset >
<legend>混合表达式*{} 【建议不使用】</legend>
编号: <span th:text="*{user.id}"></span><br>
姓名:<span th:text="*{user.name}"></span><br>
年龄:<span th:text="*{user.age}"></span><br>
</fieldset>
<h2>URL路径变量</h2>
<fieldset>
<legend>绝对路径@{.....}</legend>
<a th:href="@{https://www.sina.com}">跳转到新浪</a><br/>
<a th:href="@{http://localhost:8080/user/show}">访问初始主页</a><br/>
</fieldset>
<fieldset>
<legend>相对路径@{.....}</legend>
<a th:href="@{/user/show}">访问初始主页->相对路径</a><br/>
<a th:href="@{/user/user}">标准变量页面->相对路径</a><br/>
</fieldset>
<fieldset>
<legend>相对路径@{.....} 带参数</legend>
<!--http://localhost:8080/user/show?id=1001-->
<a th:href="@{/user/show(id=${user.id})}">URL带一个参数</a><br/>
<!--http://localhost:8080/user/show?id=1001&name=zhagnsan-->
<a th:href="@{/user/show(id=${user.id},name=${user.name})}">URL带多个参数</a><br/>
<a th:href="@{'/user/show/'+${user.id}+'/'+${user.name}}">RESTful方式</a><br/>
<a th:href="@{/user/user/{id}(id=${user.id})}">RESTful方式->占位符替换[针对单个id处理]</a><br/>
</fieldset>
<h2>获取页面路径</h2>
<div th:text="${#httpServletRequest.getRequestURL() +'?'+ #httpServletRequest.getQueryString() }"></div>
<div th:text="${#httpServletRequest.requestURL +'?'+ #httpServletRequest.queryString }"></div>
</body>
</html>