转自:http://blog.csdn.net/qq1712088151/article/details/7357476?locationNum=12&fps=1
堆栈和队列都是线性表结构。只不过堆栈的逻辑数据特点是先进后出(FILO),而队列的逻辑数据特点是先进先出(FIFO)。我们先用数组来存放这两种数据结构,也就是线性的存储结构。
请看下例:
- public class StackT {
- private int maxSize; // 堆栈的大小
- private int[] stackArray;
- private int top; // 堆栈顶部指针
- public StackT(int s) {
- maxSize = s; // 初始化数组大小
- stackArray = new int[maxSize]; // 初始化一个数组
- top = -1;
- }
- public void push(int j){
- if (!isFull())
- stackArray[++top] = j;
- else
- return;
- }
- public int pop(){
- return stackArray[top--];
- }
- public int peek(){// 得到栈顶的数据而不是出栈
- return stackArray[top];
- }
- public boolean isEmpty(){
- return (top == -1);
- }
- public boolean isFull(){
- return (top == maxSize - 1);
- }
- public String toString(){
- StringBuffer sb = new StringBuffer();
- for (int i = top; i >= 0; i--)
- sb.append("" + stackArray[i] + "\n");
- return sb.toString();
- }
- } // end class StackX
- class StackApp {
- public static void main(String[] args) {
- StackT theStack = new StackT(10); // 初始化一个堆栈
- theStack.push(20);
- theStack.push(40);
- theStack.push(60);
- theStack.push(80);
- System.out.println(theStack);
- System.out.println("");
- } // end main()
- } // end class StackApp
运行的结果如图:
队列的实现(循环队列):
- public class Queue {
- private int maxSize; // 表示队列的大小
- private int[] queArr; // 用数组来存放有队列的数据
- private int front; // 取数据的下标
- private int rear; // 存数据的下标
- private int nItems; // 记录存放的数据个数
- public Queue(int s) {
- maxSize = s;
- queArr = new int[maxSize];
- front = 0;
- rear = -1;
- nItems = 0;
- }
- public void insert(int j) { // 增加数据的方法
- if (isFull())
- return;
- // 如果下标到达数组顶部的话,让rear指向数组的第一个位置之前
- if (rear == maxSize - 1)
- rear = -1;
- queArr[++rear] = j; // increment rear and insert
- nItems++; // one more item
- }
- public int remove(){
- int temp = queArr[front++];
- // 如果下标到达数组顶部的话,让front指向数组的第一个位置
- if (front == maxSize)
- front = 0;
- nItems--;
- return temp;
- }
- public int peekFront(){// 只是返回最前面那个元素的值,并不删除
- return queArr[front];
- }
- public boolean isEmpty() {
- return (nItems == 0);
- }
- public boolean isFull() {
- return (nItems == maxSize);
- }
- public int size() {
- return nItems;
- }
- } // end class Queue
- class QueueApp {
- public static void main(String[] args) {
- Queue theQueue = new Queue(5);
- theQueue.insert(10); // 插入4个数据
- theQueue.insert(20);
- theQueue.insert(30);
- theQueue.insert(40);
- theQueue.remove(); // 删除(10, 20, 30)
- theQueue.remove();
- theQueue.remove();
- theQueue.insert(50); // 再插入4个数据
- theQueue.insert(60);
- theQueue.insert(70);
- theQueue.insert(80);
- while (!theQueue.isEmpty())
- // 取出所有数据
- System.out.println(theQueue.remove());
- }
- }
运行的结果如图:
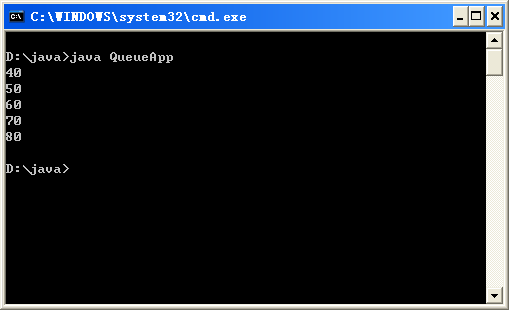