#include <algorithm>
#include <cmath>
#include <iostream>
#define eps (1e-8)
using namespace std;
struct Elem
{
double coef;
int exp;
bool operator<(const Elem &b) { return exp < b.exp; }
};
struct Node
{
Elem data;
Node *next;
};
class Polynomial
{
public:
Polynomial();
Polynomial(const Elem a[], int n);
Polynomial(const Polynomial &b);
~Polynomial();
friend Polynomial operator+(const Polynomial &a, const Polynomial &b);
friend ostream &operator<<(ostream &out, const Polynomial &b);
private:
Node *first;
};
int main()
{
// // test input and output polynomial
// Elem a[10];
// int n;
// cout << "Pleace input polynomial A." << endl;
// cin >> n;
// for (int i = 0; i < n; i++)
// cin >> a[i].coef >> a[i].exp;
// sort(a, a + n);
// Polynomial A(a,n);
// cout<<A;
// input two polynomials named A and B
Elem a[10], b[10];
int n, m;
cout << "Pleace input polynomial A.\n";
cin >> n;
for (int i = 0; i < n; i++)
cin >> a[i].coef >> a[i].exp;
sort(a, a + n);
cout << "Pleace input polynomial B.\n";
cin >> m;
for (int i = 0; i < m; i++)
cin >> b[i].coef >> b[i].exp;
sort(b, b + m);
// calculate A + B and output
Polynomial A(a, n), B(b, m);
Polynomial C = A + B;
cout << "Answer of A + B:\n";
cout << C;
return 0;
}
Polynomial::Polynomial()
{
first = new Node{0, 0, NULL};
}
Polynomial::Polynomial(const Elem a[], int n)
{
first = new Node{0, 0, NULL};
Node *p = first, *s;
for (int i = 0; i < n; i++)
{
if (abs(a[i].coef) < eps)
continue;
s = new Node{a[i], p->next}, p->next = s, p = s;
}
}
Polynomial::Polynomial(const Polynomial &b)
{
first = new Node{0, 0, NULL};
Node *p = first, *q = b.first->next, *s;
for (; q; q = q->next)
s = new Node{q->data, p->next}, p->next = s, p = s;
}
Polynomial::~Polynomial()
{
while (first)
{
Node *tmp = first;
first = first->next;
delete tmp;
}
}
Polynomial operator+(const Polynomial &a, const Polynomial &b)
{
Polynomial c(a);
Node *p = c.first->next, *q = b.first->next, *pre = c.first;
while (p && q)
{
if (p->data.exp < q->data.exp)
pre = p, p = p->next;
else if (p->data.exp > q->data.exp)
{
Node *s = new Node{q->data, p};
pre->next = s;
pre = s;
q = q->next;
}
else
{
p->data.coef += q->data.coef;
if (abs(p->data.coef) < eps)
{
Node *tmp = p;
p = p->next;
pre->next = p;
delete tmp;
}
else
pre = p, p = p->next;
q = q->next;
}
}
for (p = pre; q; q = q->next)
{
Node *s = new Node{q->data, NULL};
p->next = s;
p = s;
}
return c;
}
inline char getSign(const Elem &x)
{
return x.coef < -eps ? '-' : '+';
}
inline char *getVarible(const Elem &x)
{
static char s[20];
if (x.exp == 0)
sprintf(s, "\0");
else if (x.exp == 1)
sprintf(s, "x");
else if (x.exp >= 2)
sprintf(s, "x^%d", x.exp);
else
sprintf(s, "x^(%d)", x.exp);
return s;
}
inline void write(ostream &out, const Elem &x)
{
out << (x.coef < -eps ? '-' : '\0');
if (abs(abs(x.coef) - 1) > eps || !x.exp)
out << abs(x.coef);
out << getVarible(x);
}
inline void writeWithSign(ostream &out, const Elem &x)
{
out << getSign(x);
if (abs(abs(x.coef) - 1) > eps || !x.exp)
out << abs(x.coef);
out << getVarible(x);
}
ostream &operator<<(ostream &out, const Polynomial &b)
{
Node *p = b.first->next;
if (!p)
out << 0;
else
{
write(out, p->data);
p = p->next;
for (; p; p = p->next)
writeWithSign(out, p->data);
}
out << endl;
return out;
}
多项式加法
最新推荐文章于 2024-04-24 19:54:25 发布
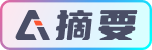