安装插件 npm install vue-scroller -d
main.js
import VueScroller from 'vue-scroller'
Vue.use(VueScroller)
<template>
<div class="wrap">
<div class="footer">
<div class="btn" @click="open = true">新增</div>
</div>
<div class="scrollerWrap">
<scroller :on-infinite="infinite" ref="myscroller" :noDataText="noDataText" class="item">
<div class="scrollerContent">
<div class="list" v-for="(item,i) in reportRecordList" :key="i">
<div class="cont">{{ item.name }}</div>
<div class="list-bot">
<div class="list-del" @click="handleDelete(i)">删除</div>
<div class="list-edit" @click="handleUpdate(i)">编辑</div>
</div>
</div>
</div>
</scroller>
</div>
<div class="mask" v-if="open"></div>
<div class="pop-box" v-if="open">
<div class="textare-wrap">
<el-input type="textarea" v-model="oname" class="otextare" resize="none"></el-input>
</div>
<div class="pop-footer">
<div class="b1" @click="cancel">取消</div>
<div class="b2" @click="submitForm">保存</div>
</div>
</div>
</div>
</template>
data里
oname: '',
// 是否显示弹出层
open: false,
// 用户信息
user: null,
// 查询参数
queryParams: {
pageNum: 1,
pageSize: 10
},
total: 0,
// 体测数据记录目录表格数据
reportRecordList: [],
noDate: false,//这是一个判断是否加载的开关
noDataText: '',//目前这个还没显示出来
methods里:
infinite(done) {
let that = this;
console.log(that.noDate)
if (!that.noDate) {
setTimeout(() => {
that.getList(done);
}, 300)
} else {
this.noDataText ="无更多数据"
that.$refs.myscroller.finishInfinite(true);//这个方法是不让它加载了,显示“没有更多数据”,要不然会一直转圈圈
}
},
/** 查询体测数据记录目录列表 */
getList(fn) {
this.loading = true;
listReportRecord(this.queryParams).then(response => {
//this.reportRecordList = response.rows;
let newDataList = this.reportRecordList.concat(response.rows)
this.reportRecordList = newDataList
this.total = response.total;
console.log("---")
console.log(newDataList.length >= this.total)
if (newDataList.length >= this.total) {
if(fn) fn(true);
this.noDate = true
} else {
if(fn) fn();
this.queryParams.pageNum++;//下拉一次页数+1
this.noDate = false
}
this.loading = false;
});
},
<style scoped>
.wrap{ min-height: 100%; background: #f8f8f8;}
.list{ margin: 0 15px 10px 15px; padding: 15px; box-sizing: border-box; background: #fff; font-size: 14px; border-radius: 10px;}
.list-bot{ margin-top: 15px; display: flex; justify-content: flex-end;}
.list-edit,.list-del{ margin-left: 15px; padding: 0 15px; height: 30px; line-height: 30px; border: 1px solid #ddd; border-radius: 15px;}
.list-del{ color: #ccc;}
.cont{ color: #333;}
.footer{ position: fixed; bottom: 0; left: 0; z-index: 99; display: flex; justify-content: center; align-items: center; width: 100%; height: 50px; background: #fff; border-top: 1px solid #f8f8f8;}
.footer .btn{ padding: 0 20px; height: 36px; line-height: 36px; background: #33a7ff; font-size: 16; color: #fff; border-radius: 18px;}
.mask{ position: fixed; top: 0; right: 0; bottom: 0; left: 0; z-index: 998; background: rgba(0,0,0,0.3);}
.pop-box{ position: fixed; top: 50%; left: 50%; z-index: 999; transform: translate(-50%, -50%); box-sizing: border-box; width: 90%; background: #fff; border-radius: 10px;}
.pop-footer{ display: flex; height: 50px; border-top: 1px solid #f8f8f8;}
.otextare{ padding: 15px 15px 10px 15px}
.otextare /deep/ .el-textarea__inner{ height: 200px;}
.pop-footer .b1,.pop-footer .b2{ flex: 1; text-align: center; line-height: 50px; font-size: 16px; }
.pop-footer .b1{ border-right: 1px solid #f8f8f8; color: #999;}
.pop-footer .b2{ color: #33a7ff;}
.scrollerWrap{
position:absolute;
width:100%;
height: calc(100% - 70px);
top: 10px;
bottom: 0;
}
.scrollerContent{ height: 100%;}
</style>
个人记录 仅供参考
vue 使用vue-scroller 列表滑动到底部加载更多数据
于 2024-03-02 10:27:43 首次发布
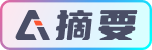