背景:ai测试精度结果文件有5w行,跑测试的时候,需要逐个文件打开读最后一行的数据,拷贝放在结果表中,比较耗时,经常做回归测试,抽了点时间把这个读写数据的过程自动化了,同时和bench表对应网络的标准bench数据进行对比,得到是否pass的最终测试结果,一并写入了结果表中,实测已经可用,特记录一下,以备后用 解决的技术问题包括: 1、获取指定路径下的文件名称 2、匹配字符串 3、txt文件读指定行、匹配字符串 4、根据单元格内容查找excel中单元格所在的行号 ************************************代码分隔符************************** # coding:utf-8 import xlwt import xlrd import pandas as pd from os import listdir '''说明: 1、功能说明:用于自动从精度测试结果txt文件中获取精度、从精度bench表中获取bench、计算精度gap、计算精度测试结果,并写入到结果表result.xls中;适用于c++接口01_getresult_c++.py 2、使用说明: a.将bench和本文件拷贝至本地,并运行本文件,本bench表目前只包含pytorch网络bench,其他网络需根据bench表格式修改为 被测网络bench b.可能需要修改的参数: bench.xlsx文件内容 path:此目录为结果文件txt文件所在的路径,也可以是某个指定路径,如E:\\automation\\demo result_file:结果文件的路径及文件名,根据实际情况填写,如E:\\automation\\demo\\result.xls ''' #定义benchfile名称、结果文件名称 path="." #此目录为结果文件txt文件所在的路径,也可以是某个指定路径,如E:\\automation\\demo bench_file ='bench.xlsx' #指定bench文件的名称及路径,可以是绝对路径,如E:\\automation\\demo\\bench.xlsx workbook = xlrd.open_workbook(bench_file) sheet1 = workbook.sheet_by_index(0) #定义网络模型集合和quant方式集合 models = ["inceptionV3", "resnet18", "resnet34", "resnet50", "resnet101", "resnet152", "squeezenet1_0", "squeezenet1_1", "densenet121", "densenet169", "densenet201", "densenet161", "shufflenet_v2_x1_0", "shufflenet_v2_x0_5", "mobilenet_v2", "resnext50_32x4d", "resnext101_32x8d", "wide_resnet50_2", "wide_resnet101_2", "gluon_resnet50_v1b", "gluon_resnet50_v1c", "gluon_resnet50_v1d", "mnasnet0_5", "mnasnet1_0"] quants = ["max", "sigma", "percentile", "kl_divergence"] ##初始化:定义结果表表明及表头:网络、quant类型、bench、精度结果、精度bench、判断结果 workbook = xlwt.Workbook(encoding='utf-8', style_compression=0) sheet = workbook.add_sheet('test', cell_overwrite_ok=True) result_file = "result.xls" # 结果文件的路径及文件名,根据实际情况填写,如E:\\automation\\demo\\result.xls sheet.write(0, 0, 'no') sheet.write(0, 1, 'net') sheet.write(0, 2, 'quant') sheet.write(0, 3, 'Top1_bench') sheet.write(0, 4, 'Top5_bench') sheet.write(0, 5, 'Top1') sheet.write(0, 6, 'Top5') sheet.write(0, 7, 'Top1_gap') sheet.write(0, 8, 'Top5_gap') sheet.write(0, 9, 'test_result') #根据文件名称和单元格内容,获取单元格所在的行号 def find_row(num_value,file_name): demo_df = pd.read_excel(file_name) for indexs in demo_df.index: for i in range(len(demo_df.loc[indexs].values)): if (str(demo_df.loc[indexs].values[i]) == num_value): row = str(indexs+2).rstrip('L') return row # 获取指定目录下(不包括子目录)的所有txt格式文件 def get_file_name(filepath): #print(listdir(filepath)) txtfile = [] for f in listdir(filepath): if ".txt" in f: txtfile.append(f) return txtfile txt_file = get_file_name(path) #获取所有txt文件名 i = 1 #处理每个txt文件,得到精度值,并计算差值,写入到结果表中 for file in txt_file: fname = file.split('.')[0] print(fname) #net = fname.split('_')[1] #quant = fname.split('_')[3] with open(file, 'r') as f: # 打开文件 # 向结果表种写入网络名称、quant方式 sheet.write(i, 0, i) #sheet.write(i, 1, net) #sheet.write(i, 2, quant) # 获取精度测试结果,获取最后一行的精度值,并写入值结果表中,适用c++接口 lines = f.readlines() # 读取所有行 first_line = lines[0] # 取第一行 last_line = lines[-1] # 取最后一行 top1 = last_line.split(' ')[-2].split(":")[1].replace('Prec', '') top5 = last_line.split(' ')[-1].split(":")[-1] sheet.write(i, 5, top1) sheet.write(i, 6, top5) # 通过txt文件名称与网络名称字典匹配,得出网络名称 m = 0 for model in models: # print("aaa") # print( "aaa" + model) if model in fname: net = model # print("bbb"+net) m = m + 1 sheet.write(i, 1, net) # 通过txt文件名称与网络名称字典匹配,获取quant方式 j = 0 for q in quants: if q in fname: quant = q j = j + 1 sheet.write(i, 2, quant) # 通过网络名称,从bench表中获取该网络的bench,并写入到结果表中 row_num = int(find_row(net, bench_file)) -1 print(row_num) print(sheet1.cell(row_num, 3)) print(sheet1.cell(row_num, 4)) top1_bench = sheet1.cell(row_num, 3).value top5_bench = sheet1.cell(row_num, 4).value sheet.write(i, 3, top1_bench) sheet.write(i, 4, top5_bench) #计算bench_gap、是否通过,并写入结果表 top1_gap = top1_bench - float(top1) top5_gap = top5_bench - float(top5) sheet.write(i, 7, top1_gap) sheet.write(i, 8, top5_gap) if top1_gap <= 1 and top1_gap <= 1: result = "pass" else: result = "fail" sheet.write(i, 9, result) i = i+1 workbook.save(result_file)
AI测试自动化脚本:解析结果txt文件字段并保存至excel文件
于 2022-03-09 15:40:13 首次发布
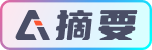