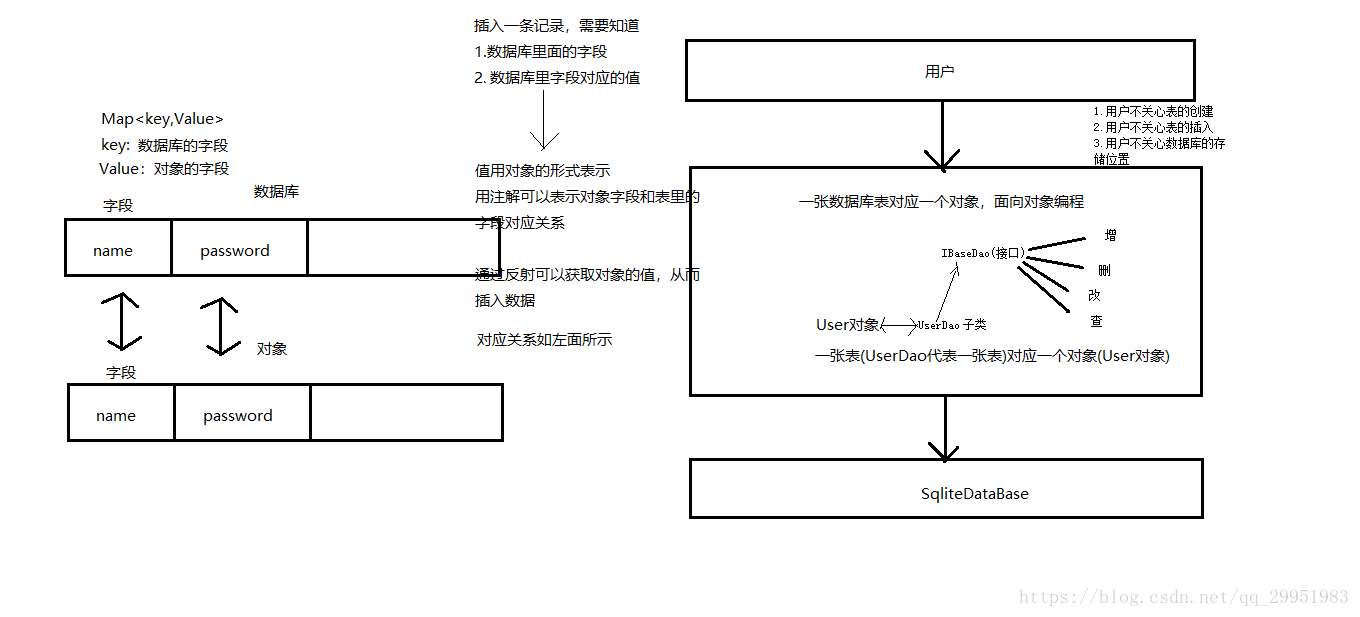
/**
* 抽象接口,定义增删改查等操作
* @param <T>
*/
public interface IBaseDao<T> {
Long inserrt(T entity);
int update(T entity, T where);
}
/**
* 用于通过对象的字段获取对应数据库的字段名称
*/
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
public @interface DbFiled {
String value();
}
/**
* 用于获取表名
*/
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface DbTable {
String value();
}
@DbTable("tb_user")
public class User {
@DbFiled("name")
public String name;
@DbFiled("password")
public String password;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
/**
* 对应的表
*/
public class UserDao extends BaseDao {
@Override
public String createDataBase() {
return "create table if not exists tb_user(name varchar(20),password varchar(10))";
}
}
/**
* 核心类
* 实现了增删改查接口的方法
* 确立了表字段和表对象字段的对应关系
* @param <T>
*/
public abstract class BaseDao<T> implements IBaseDao<T> {
private SQLiteDatabase sqLiteDatabase;
private boolean isInit = false;
private String tableName;
private Class<T> entityClass;
private Map<String, Field> cacheMap = new HashMap();;
public synchronized void init(Class<T> entity, SQLiteDatabase sqLiteDatabase) {
if (!isInit) {
this.sqLiteDatabase = sqLiteDatabase;
this.tableName = entity.getAnnotation(DbTable.class).value();
this.entityClass = entity;
sqLiteDatabase.execSQL(createDataBase());
initCacheMap();
}
}
/**
* 找到数据库字段和表对象(User对象)对应的子段,创建Map
*/
protected void initCacheMap() {
String sql = "select * from " + this.tableName + " limit 1,0";
Cursor cursor = null;
try {
cursor = this.sqLiteDatabase.rawQuery(sql, null);
String[] columnNames = cursor.getColumnNames();
Field[] columnFields = entityClass.getFields();
for (Field field : columnFields) {
field.setAccessible(true);
}
for (String columnName : columnNames) {
for (Field field : columnFields) {
String filedName;
if (field.getAnnotation(DbFiled.class) != null) {
filedName = field.getAnnotation(DbFiled.class).value();
} else {
filedName = field.getName();
}
if (columnName.equals(filedName)) {
cacheMap.put(columnName, field);
}
}
}
} catch (Exception e) {
} finally {
cursor.close();
}
}
public abstract String createDataBase();
@Override
public Long inserrt(T entity) {
Map<String, String> map = getValues(entity);
ContentValues contentvalues = getContentValues(map);
Long result = sqLiteDatabase.insert(tableName, null, contentvalues);
return result;
}
/**
* 返回ContentValues
* @param map key是数据库的字段名称,Value是需要插入的值
* @return ContentValues
*/
private ContentValues getContentValues(Map<String, String> map) {
ContentValues contentvalues = new ContentValues();
Set keys = map.keySet();
Iterator iterator = keys.iterator();
while (iterator.hasNext()) {
String key = (String) iterator.next();
String value = map.get(key);
if (value != null) {
contentvalues.put(key, value);
}
}
return contentvalues;
}
/**
* 将表对象的的field值取出,作为Value
* 将field对象的数据库字段名取出,作为Key
* @param entity 表对象(User)
* @return Map
*/
public Map getValues(T entity) {
HashMap<String, String> result = new HashMap<>();
Iterator fieldsIterator = cacheMap.values().iterator();
while (fieldsIterator.hasNext()) {
Field colmunToFiled = (Field) fieldsIterator.next();
String cacheKey = null;
String cacheValue = null;
if (colmunToFiled.getAnnotation(DbFiled.class) != null) {
cacheKey = colmunToFiled.getAnnotation(DbFiled.class).value();
} else {
cacheKey = colmunToFiled.getName();
}
try {
if (null == colmunToFiled.get(entity)) {
continue;
}
cacheValue = colmunToFiled.get(entity).toString();
} catch (Exception e) {
e.printStackTrace();
}
result.put(cacheKey, cacheValue);
}
return result;
}
@Override
public int update(T entity, T where) {
int result = -1;
Map values = getValues(entity);
Condition condition = new Condition(getValues(where));
result = sqLiteDatabase.update(tableName, getContentValues(values),
condition.whereClauser, condition.WhereArgs);
return result;
}
}
/**
* 用于拼接查询语句
*/
public class Condition {
public String whereClauser;
public String[] WhereArgs;
public Condition(Map<String, String> map) {
ArrayList list = new ArrayList();
StringBuilder stringBuilder = new StringBuilder();
stringBuilder.append(" 1=1 ");
Set keys = map.keySet();
Iterator iterator = keys.iterator();
while (iterator.hasNext()) {
String key = (String) iterator.next();
String value = map.get(key);
if (null != value) {
stringBuilder.append(" and " + key + " =? ");
list.add(value);
}
}
this.whereClauser = stringBuilder.toString();
this.WhereArgs = (String[]) list.toArray(new String[list.size()]);
}
}
public class MainActivity extends AppCompatActivity {
BaseDao baseDao;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
baseDao = DaoManagerFactory.instance().getDataHelper(UserDao.class, User.class);
}
public void insert(View view) {
User user = new User();
user.setName("李四");
user.setPassword("123456");
baseDao.inserrt(user);
}
}
/**
* 工厂类
*/
public class DaoManagerFactory {
private String path;
private static DaoManagerFactory instance = new DaoManagerFactory(new File(Environment.getExternalStorageDirectory(), "ligic.db"));
private SQLiteDatabase sqLiteDatabase;
private DaoManagerFactory(File file) {
this.path = file.getAbsolutePath();
openDataBase();
}
private void openDataBase() {
this.sqLiteDatabase = SQLiteDatabase.openOrCreateDatabase(path, null);
}
public synchronized <T extends BaseDao, M> T getDataHelper(Class<T> clazz, Class<M> entityClass) {
T baseDao = null;
try {
baseDao = clazz.newInstance();
baseDao.init(entityClass, sqLiteDatabase);
} catch (InstantiationException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
}
return baseDao;
}
public static DaoManagerFactory instance() {
return instance;
}
}