package com.matech.auditing.util;
import org.apache.commons.compress.archivers.zip.ZipArchiveEntry;
import org.apache.commons.compress.archivers.zip.ZipArchiveInputStream;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.io.InputStreamResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.URLEncoder;
import java.nio.charset.Charset;
import java.util.Enumeration;
import java.util.List;
import java.util.Objects;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import java.util.zip.ZipOutputStream;
/**
* @ author xf
* @ version 1.0
* @ description: 文件处理工具类
* @ date 2021/7/12 16:06
*/
@Component
public class FileUtil {
private static final Logger LOGGER = LoggerFactory.getLogger(FileUtil.class);
/**
* 删除文件夹
*
* @param dir 文件夹路径
*/
public static boolean deleteDir(File dir) {
if (dir.isDirectory()) {
String[] children = dir.list();
for (int i = 0; i < Objects.requireNonNull(children).length; i++) {
boolean success = deleteDir(new File(dir, children[i]));
if (!success) {
return false;
}
}
}
//删除空文件夹
return dir.delete();
}
/**
* 压缩文件
* @ param files
* @ param zipFilePath
* @ param fileName
*/
public static File fileToZip(List<File> files, String zipFilePath, String fileName) throws IOException, RuntimeException {
File zipFile = new File(zipFilePath + "/" + fileName + ".zip");
boolean b = new File(zipFilePath).mkdirs();
if(!b){
return null;
}
FileInputStream fis;
BufferedInputStream bis = null;
FileOutputStream fos;
ZipOutputStream zos = null;
try {
fos = new FileOutputStream(zipFile);
zos = new ZipOutputStream(new BufferedOutputStream(fos));
byte[] bufs = new byte[1024 * 10];
for (File file : files) {
//创建ZIP实体,并添加进压缩包
String name=file.getName();
if(file.isFile()){
if(files.contains(file.getParentFile())){
name = file.getParentFile().getName() + File.separator + name;
}
ZipEntry zipEntry = new ZipEntry(name);//压缩
zos.putNextEntry(zipEntry);//将每个压缩文件保存到zip文件中
//读取待压缩的文件并写进压缩包里
fis = new FileInputStream(file);
bis = new BufferedInputStream(fis, 1024 * 10);
int read;
while ((read = bis.read(bufs, 0, 1024 * 10)) != -1) {
zos.write(bufs, 0, read);
}
}else{
zos.putNextEntry(new ZipEntry(name + "/"));
}
}
} catch (FileNotFoundException e) {
e.printStackTrace();
throw new RuntimeException(e);
} finally {
//关闭流
try {
if (null != bis) bis.close();
if (null != zos) zos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
return zipFile;
}
/**
* 下载文件到默认浏览器
* @ param in 输入流
* @ param fileName 文件名称
*/
public static ResponseEntity<InputStreamResource> downloadFile(InputStream in, String fileName) {
try {
byte[] testBytes = new byte[in.available()];
HttpHeaders headers = new HttpHeaders();
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Content-Disposition", String.format("attachment; filename=\"%s\"", convertFileName(fileName)));
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
return ResponseEntity
.ok()
.headers(headers)
.contentLength(testBytes.length)
.contentType(MediaType.parseMediaType("application/octet-stream"))
.body(new InputStreamResource(in));
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
/**
* @ description: 下载文件
* @ param file
* @ return: void
* @ author xf
* @ date: 2021/7/13 13:57
*/
public static void downloadFile(File file,HttpServletResponse response) throws RuntimeException{
try{
String fileName = file.getName();
if(response == null)
response = ((ServletRequestAttributes) Objects.requireNonNull(RequestContextHolder.getRequestAttributes())).getResponse();
/* 设置文件ContentType类型,这样设置,会自动判断下载文件类型 */
assert response != null;
response.setContentType(MediaType.APPLICATION_OCTET_STREAM.toString());
response.setContentType("application/force-download");
response.setHeader("Content-Disposition", "attachment;fileName*=UTF-8''" + fileName);
response.addHeader("Access-Control-Expose-Headers", "Content-Disposition");
InputStream ins = new FileInputStream(file);
OutputStream os = response.getOutputStream();
byte[] b = new byte[1024];
int len;
while((len = ins.read(b)) > 0){
os.write(b,0,len);
}
os.flush();
os.close();
ins.close();
}catch (Exception e){
e.printStackTrace();
}
}
/**
* chinese code convert
* @ param fileName 文件名称
* @ return 文件名称
*/
public static String convertFileName(String fileName) {
if(isContainChinese(fileName)) {
try {
fileName = URLEncoder.encode(fileName, "gbk");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
}
return fileName;
}
/**
* 判断字符串中是否包含中文
* @ param str 待校验字符串
* @ return 是否为中文
* @ warn 不能校验是否为中文标点符号
*/
public static boolean isContainChinese(String str) {
Pattern p = Pattern.compile("[\u4e00-\u9fa5]");
Matcher m = p.matcher(str);
return m.find();
}
/**
* 将inputStream转化为file
* @param is 输入流
* @param file 要输出的文件目录
*/
public static void inputStream2File(InputStream is, File file) throws IOException {
try (OutputStream os = new FileOutputStream(file)) {
int len;
byte[] buffer = new byte[8192];
while ((len = is.read(buffer)) != -1) {
os.write(buffer, 0, len);
}
} finally {
if (is != null) {
is.close();
}
}
}
/**
* 将file转换为inputStream
* @ param file
* @ throws FileNotFoundException
*/
public static InputStream file2InputStream(File file) throws FileNotFoundException {
return new FileInputStream(file);
}
/**
* @ description: 解压压缩包文件
* @ param zipFile 压缩包文件
* @ param descDir 解压缩路径
* @ return: void
* @ author xf
* @ date: 2021/7/15 11:44
*/
public static void unArchivezip(File zipFile, String descDir) {
try (ZipArchiveInputStream inputStream = getZipFile(zipFile)) {
File pathFile = new File(descDir);
boolean b;
if (!pathFile.exists()) {
b = pathFile.mkdirs();
if(!b){
LOGGER.info("解压文件路径:{}", descDir + "创建失败");
return;
}
}
ZipArchiveEntry entry;
while ((entry = inputStream.getNextZipEntry()) != null) {
if (entry.isDirectory()) {
File directory = new File(descDir, entry.getName());
boolean b1 = directory.mkdirs();
if(!b1){
LOGGER.info("解压文件路径:{}", descDir + entry.getName());
}
} else {
try (OutputStream os = new BufferedOutputStream(new FileOutputStream(new File(descDir, entry.getName())))) {
//输出文件路径信息
LOGGER.info("解压文件的当前路径为:{}", descDir + entry.getName());
IOUtils.copy(inputStream, os);
}
}
}
final File[] files = pathFile.listFiles();
if (files != null && files.length == 1 && files[0].isDirectory()) {
// 说明只有一个文件夹
FileUtils.copyDirectory(files[0], pathFile);
//免得删除错误, 删除的文件必须在/data/demand/目录下。
boolean isValid = files[0].getPath().contains("/data/www/");
if (isValid) {
FileUtils.forceDelete(files[0]);
}
}
LOGGER.info("******************解压完毕********************");
} catch (Exception e) {
LOGGER.error("[unzip] 解压zip文件出错", e);
}
}
private static ZipArchiveInputStream getZipFile(File zipFile) throws Exception {
return new ZipArchiveInputStream(new BufferedInputStream(new FileInputStream(zipFile)));
}
/**
* 解压
* @ param zipFile 待解压的文件
* @ param unzipFilePath 解压后的文件存储路径
* @ throws Exception
*/
public static void unzip(File zipFile, String unzipFilePath) throws Exception {
//创建解压缩文件保存的路径
File unzipFileDir = new File(unzipFilePath);
if (!unzipFileDir.exists() || !unzipFileDir.isDirectory()) unzipFileDir.mkdirs();
//开始解压
ZipEntry entry;
String entryFilePath, entryDirPath;
File entryFile, entryDir;
int index, count;
byte[] buffer = new byte[1024];
BufferedInputStream bis;
BufferedOutputStream bos;
ZipFile zip = new ZipFile(zipFile, Charset.forName("GBK"));
Enumeration<ZipEntry> entries = (Enumeration<ZipEntry>)zip.entries();
//循环对压缩包里的每一个文件进行解压
while(entries.hasMoreElements()) {
// 获取下一个文件
entry = entries.nextElement();
if(entry.isDirectory()){
continue;
}else{
//构建压缩包中一个文件解压后保存的文件全路径
entryFilePath = unzipFilePath + File.separator + tranSlash(entry.getName());
}
//构建解压后保存的文件夹路径
index = entryFilePath.lastIndexOf(File.separator);
if (index != -1) {
entryDirPath = entryFilePath.substring(0, index);
}
else {
entryDirPath = "";
}
entryDir = new File(entryDirPath);
//如果文件夹路径不存在,则创建文件夹
if (!entryDir.exists() || !entryDir.isDirectory()) {
entryDir.mkdirs();
}
//创建解压文件
entryFile = new File(entryFilePath);
if (entryFile.exists()) {
//检测文件是否允许删除,如果不允许删除,将会抛出SecurityException
SecurityManager securityManager = new SecurityManager();
securityManager.checkDelete(entryFilePath);
//删除已存在的目标文件
entryFile.delete();
}
//写入文件
bos = new BufferedOutputStream(new FileOutputStream(entryFile));
bis = new BufferedInputStream(zip.getInputStream(entry));
while ((count = bis.read(buffer, 0, 1024)) != -1) {
bos.write(buffer, 0, count);
}
bos.flush();
bos.close();
}
}
/**
* @ description: 将 / 或 \ 转换为对应平台下的符号
* @ param str 字符串
* @ return: java.lang.String
* @ author xf
* @ date: 2021/7/14 15:02
*/
public static String tranSlash(String str){
return str.replace("\\",File.separator).replace("/",File.separator);
}
/**
* @ description: 删除文件夹
* @ param dir 目录
* @ return: void
* @ author xf
* @ date: 2021/7/13 14:20
*/
public static void deleteFile(File dir) {
File[] listFiles = dir.listFiles();
for(int i = 0; i< Objects.requireNonNull(listFiles).length; i++) {
File temp=listFiles[i];
if(temp.isDirectory()) {
deleteFile(temp);
}
temp.delete();
}
System.out.println("删除文件成功!");
}
}
File 工具类,避免重复写
最新推荐文章于 2022-07-29 18:27:26 发布
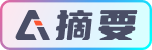