给出一个 32 位的有符号整数,你需要将这个整数中每位上的数字进行反转。
示例 1:
输入: 123
输出: 321
示例 2:
输入: -123
输出: -321
示例 3:
输入: 120
输出: 21
注意:
假设我们的环境只能存储得下 32 位的有符号整数,则其数值范围为 [−231, 231 − 1]。请根据这个假设,如果反转后整数溢出那么就返回 0。
方法一:(栈的思想,24ms)
1.
int reverse(int x) {
int rev=0,pop=0;
while(x!=0)
{
pop=x%10;
x=x/10;
if(rev>INT_MAX/10||(rev==INT_MAX/10&&pop>7))return 0;
if(rev<INT_MIN/10||(rev==INT_MIN/10&&pop<-8))return 0;
rev=rev*10+pop;
}return rev;
}
方法二(队列实现,
超时)
#include<stdio.h>
#include<stdlib.h>
typedef struct QueueNode{
int data;
struct QueueNode *next;
}QueueNode;
typedef struct Queued{
QueueNode *front;
QueueNode *last;
int num;
}Queuend,*Queue;
void InitQueue(Queue *p)
{
(*p)=(Queue)malloc(sizeof(Queuend));
(*p)->num=0;
(*p)->front=(*p)->last=NULL;
}
void InQueue(Queue p,int x)
{printf("开始执行InQueue\n");printf("增加元素为[%d]\n",x);
QueueNode *t=(QueueNode *)malloc(sizeof(QueueNode));
t->data=x;
t->next=NULL;printf("已经创建数值为[%d]的结点\n",t->data);
if(p->num==0)
{
p->front=p->last=t;
p->num++;
}
else
{
p->last->next=t;
p->last=t;
p->num++;
} printf("InQueue执行完成\n");printf("头为[%d],尾为[%d]\n",p->front->data,p->last->data);
}
int OutQueue(Queue p,int *e)
{printf("开始执行OutQueue\n");
{
(*e)=p->front->data;printf("[%d]",*e);
QueueNode *temp=p->front;
if(p->front==p->last)
{
p->front=p->last=NULL;
}
else
{
p->front=p->front->next;
}
p->num--;
free(temp);
return(*e);printf("OutQueu执行完成\n");
}return 0;
}
int reverse(int x) {
int flag=(x>0)?1:-1;
int ans=(x>0)?x:-x;
int n=ans;
Queue p;
InitQueue(&p);
while(n>0)
{
InQueue(p,n%10);
n=n/10;
}
int e=0;
ans=0;
while(p->num>0)
{
ans=ans*10+OutQueue(p,&e);
}
if(ans>2147483467)
return 0;
else
return flag*ans;
}
void main()
{
int x;
printf("请输入数字:\n");
scanf("%d",&x);
printf("\n结果为[%d]\n",reverse(x));
}
啊啊啊啊啊啊啊啊啊啊啊啊啊啊啊啊
没有图片啊啊
啊啊啊
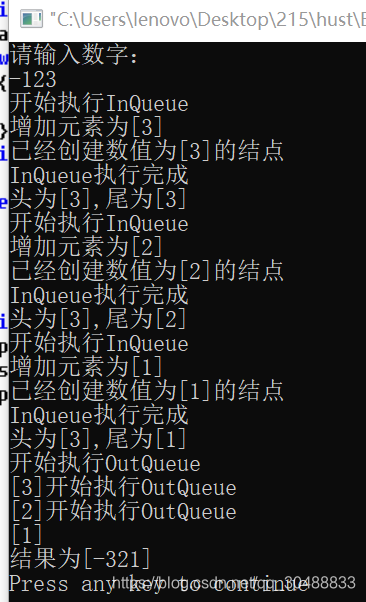
变式
请判断一个链表是否为回文链表。
示例 1:
输入: 1->2
输出: false
示例 2:
输入: 1->2->2->1
输出: true
方法一 (二级指针数组,28ms)
#include<stdio.h>
#include<stdlib.h>
struct ListNode {
int val;
struct ListNode *next;
};
bool isPalindrome(struct ListNode* head) {
struct ListNode *p,*q;//p往后,q往前
p=q=head;
int length=0;
while(q!=NULL){q=q->next;length++;}
if(length>0)
{
struct ListNode **num;
num=(struct ListNode **)malloc(length*sizeof(struct ListNode *));
q=p;
int i,j;
i=j=0;
for(i=0;i<length;i++)
{
num[i]=q;
q=q->next;
printf("num[%d]已成功存储[%d]\n",i,num[i]->val);
}
for(i=0,j=length-1;i<j;i++,j--)
{
if(num[i]->val!=num[j]->val)return false;
printf("第[%d]组已经成功比较\n",i+1);
}
return true;
}
return false;
}
void main()
{
struct ListNode *head=(struct ListNode *)malloc(sizeof(struct ListNode));
head->val =1;
struct ListNode *p=(struct ListNode *)malloc(sizeof(struct ListNode));
p->val=1;
head->next=p;
p->next=NULL;
if(isPalindrome(head)==false)printf("false\n");
else
printf("true\n");
}
方法二(评论区搬砖,快慢指针+逆序)
其一,find mid node 使用快慢指针找到链表中点。 其二,reverse 逆序后半部分。 其三,check 从头、中点,开始比较是否相同。
bool isPalindrome(ListNode* head) {//O(n)、O(1)
ListNode* slow = head, *fast = head, *prev = nullptr;
while (fast&&fast->next){//find mid node
slow = slow->next;
fast = fast->next ? fast->next->next: fast->next;
}
while (slow){//reverse
ListNode* ovn = slow->next;
slow->next = prev;
prev = slow;
slow = ovn;
}
while (head && prev){//check
if (head->val != prev->val){
return false;
}
head = head->next;
prev = prev->next;
}
return true;
}
明日预告maybe
验证回文串&&翻转链表etc
1.给定一个字符串,验证它是否是回文串,只考虑字母和数字字符,可以忽略字母的大小写。
说明:本题中,我们将空字符串定义为有效的回文串。
示例 1:
输入: "A man, a plan, a canal: Panama"
输出: true
示例 2:
输入: "race a car"
输出: false
2.反转一个单链表。
示例:
输入: 1->2->3->4->5->NULL
输出: 5->4->3->2->1->NULL
进阶:
你可以迭代或递归地反转链表。你能否用两种方法解决这道题?