标准的输出流System.out、System.in
/**
* @date 2018/7/8 22:06
* 标准的输入输出流
* 标准的输出流 System.out(); 返回一个InputStream
* 标准的输入流 System.in(); 返回一个PrintStream
*/
public class StandardInputOrOutput {
public static void main(String[] args) {
BufferedReader br = null;
try {
InputStream is = System.in;
InputStreamReader isr = new InputStreamReader(is);
br = new BufferedReader(isr);
String str = null;
while (true){
System.out.println("请输入字符流");
str = br.readLine();
if (str.equalsIgnoreCase("e")){
break;
}
System.out.println(str.toUpperCase());
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (br != null) {
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
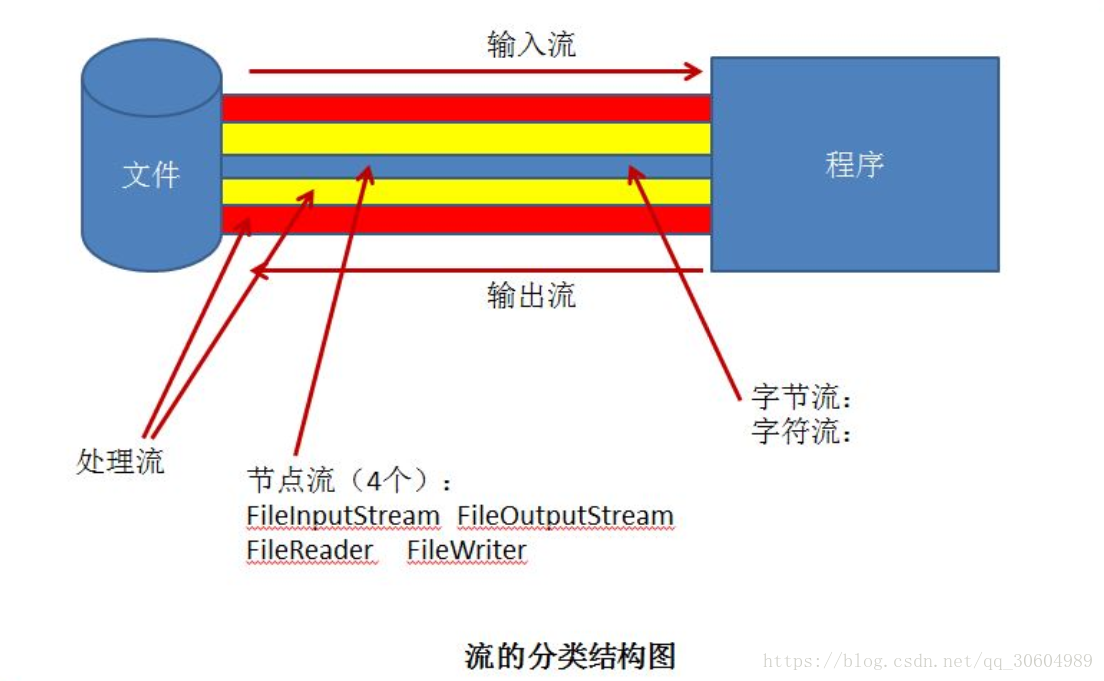
打印流
/**
* @date 2018/7/8 23:03
* 打印流:字节流:PrintStream 字符流:PrintWriter
*/
public class Print {
public static void main(String[] args) {
FileOutputStream fos = null;
try {
fos = new FileOutputStream(new File("F:/test/1.txt"));
} catch (FileNotFoundException e) {
e.printStackTrace();
}
//创建打印输出流,设置为自动刷新模式(写入换行符或字节“\n”时都会刷新输出缓冲区)
PrintStream ps = new PrintStream(fos);
if (ps!=null){
//把标准输出流(控制台输出)改成文件
System.setOut(ps);
}
//输出ASCII字符、
for (int i = 0; i < 255; i++) {
System.out.print((char)i);
if (i%50==0){
System.out.println();//换行
}
}
ps.close();
}
}
数据流
/**
* @author chenpeng
* @date 2018/7/8 23:21
* 数据流:用来处理基本数据类型、String、字节数组的数据:DataInputStream、DataOutputStream
*/
public class DataStream {
public static void main(String[] args) {
DataOutputStream dos = null;
try {
//创建连接到指定文件的数据输出流对象
FileOutputStream fos = new FileOutputStream("F:/test/1.txt");
dos = new DataOutputStream(fos);
//写UTF字符串
dos.writeUTF("I love you");
//写入布尔值
dos.writeBoolean(true);
//写入长整数
dos.writeLong(239742398);
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (dos!=null){
dos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}