LeetCode1600. 皇位继承顺序(208场周赛) 原题链接:皇位继承顺序
1. 题意
继承顺序:king->长子->长孙,长子的所有后代完了才轮到长子的兄弟。
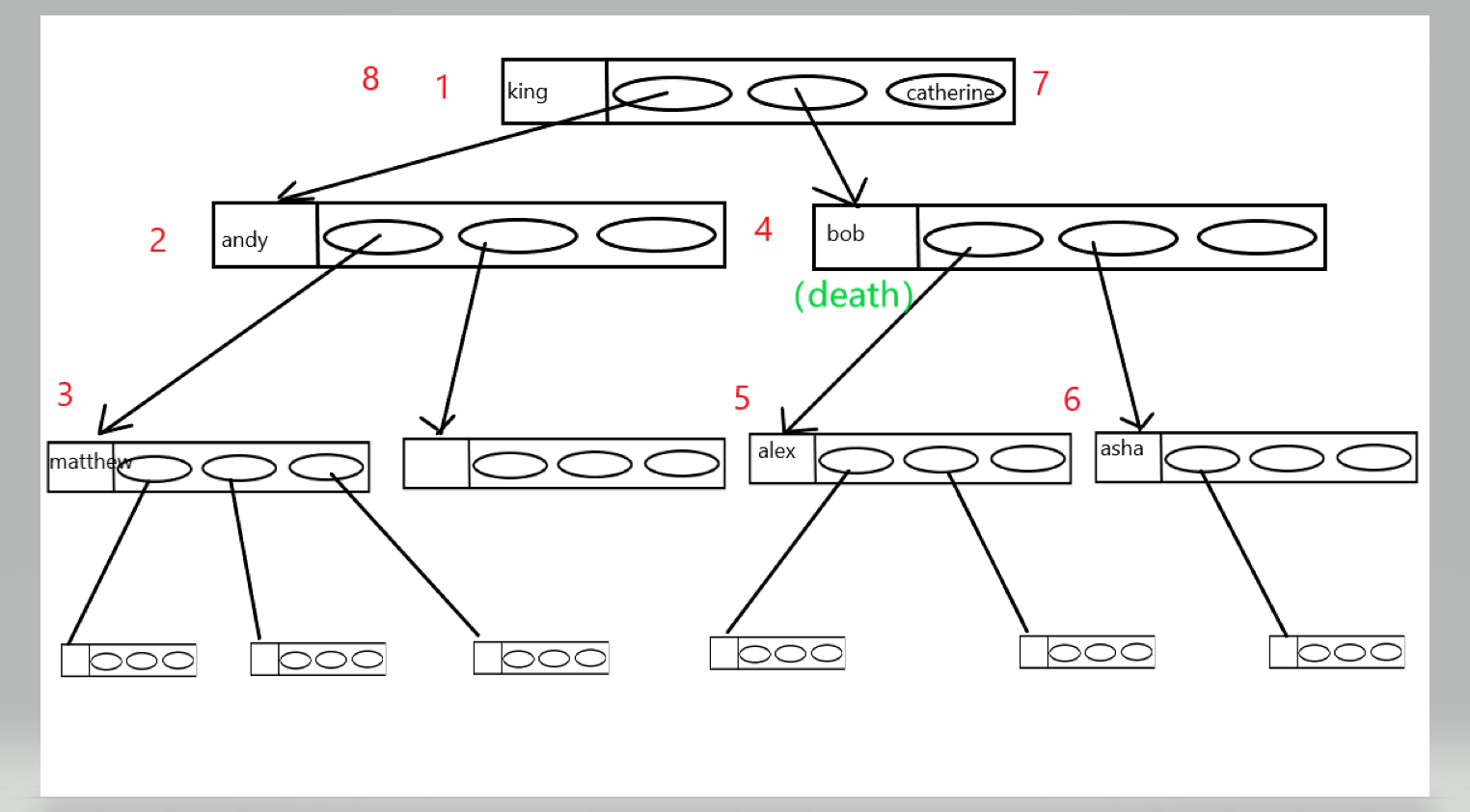
2. 思路
容易想到的是建棵N叉树,然后返回顺序为先序遍历(麻烦)。
简单一点的做法可以:
- 通过king索引出他的所有儿子,每个儿子索引出相应的孙子
- 父子关系,可以使用 unordered_map<string, vector<string>> 来记录,key 为父亲名字,value 为按顺序的孩子名字,
父亲添加儿子的同时每个儿子建一个<"名字",vector<"儿子">>对放入map。 - 使用 unordered_set<string> 来标记死亡情况
- 根据父子关系,可以进行深度优先遍历,这样在 dfs 时,根据死亡情况来输出即可
3. 代码注释
变量定义的解释
unordered_map<string, vector<string>> maps
记录族谱
string:父亲名字 vector<string>儿子们名字unordered_set<string> sets
记录已经去世的人
参考代码
void dfs(vector<string>& str, unordered_map<string, vector<string>>& maps,
unordered_set<string>& sets, string temp)
{
if (sets.find(temp) == sets.end())
str.push_back(temp);
auto item = maps.find(temp);
for (auto i : item->second)
dfs(str, maps, sets, i);
}
class ThroneInheritance {
public:
unordered_map<string, vector<string>> maps; //记录族谱
unordered_set<string> sets; //记录death的
public:
ThroneInheritance(string kingName) {
maps.emplace(kingName, vector<string>());
}
void birth(string parentName, string childName) {
auto item = maps.find(parentName);
item->second.push_back(childName); //加入父亲的儿子数组
maps.emplace(childName, vector<string>()); //儿子之后会变成父亲
}
void death(string name) {
sets.insert(name);
}
vector<string> getInheritanceOrder() {
vector<string> str;
dfs(str, maps, sets, "king"); //从king开始索引
return str;
}
};
测试代码
int main()
{
ThroneInheritance *t = new ThroneInheritance("king"); // 继承顺序:king
t->birth("king", "andy"); // 继承顺序:king > andy
t->birth("king", "bob"); // 继承顺序:king > andy > bob
t->birth("king", "catherine"); // 继承顺序:king > andy > bob > catherine
t->birth("andy", "matthew"); // 继承顺序:king > andy > matthew > bob > catherine
t->birth("bob", "alex"); // 继承顺序:king > andy > matthew > bob > alex > catherine
t->birth("bob", "asha"); // 继承顺序:king > andy > matthew > bob > alex > asha > catherine
t->getInheritanceOrder(); // 返回 ["king", "andy", "matthew", "bob", "alex", "asha", "catherine"]
t->death("bob"); // 继承顺序:king > andy > matthew > bob(已经去世)> alex > asha > catherine
/*
t->getInheritanceOrder(); // 返回 ["king", "andy", "matthew", "alex", "asha", "catherine"]
*/
vector<string> str=t->getInheritanceOrder(); // 返回 ["king", "andy", "matthew", "alex", "asha", "catherine"]
for (auto i : str)
cout << i << " ";
cout << endl;
return 0;
}
测试图片
