SqlSession 作为MyBatis工作的主要顶层API,表示和数据库交互的会话,完成必要数据库增删改查功能 (对应下图)
Executor MyBatis执行器,是MyBatis 调度的核心,负责SQL语句的生成和查询缓存的维护
StatementHandler 封装了JDBC Statement操作,负责对JDBC statement 的操作,如设置参数、将Statement结果集转换成List集合。
ParameterHandler 负责对用户传递的参数转换成JDBC Statement 所需要的参数,
ResultSetHandler 负责将JDBC返回的ResultSet结果集对象转换成List类型的集合;
TypeHandler 负责java数据类型和jdbc数据类型之间的映射和转换
MappedStatement MappedStatement维护了一条<select|update|delete|insert>节点的封装,
SqlSource 负责根据用户传递的parameterObject,动态地生成SQL语句,将信息封装到BoundSql对象中,并返回
BoundSql 表示动态生成的SQL语句以及相应的参数信息
Configuration MyBatis所有的配置信息都维持在Configuration对象之中。
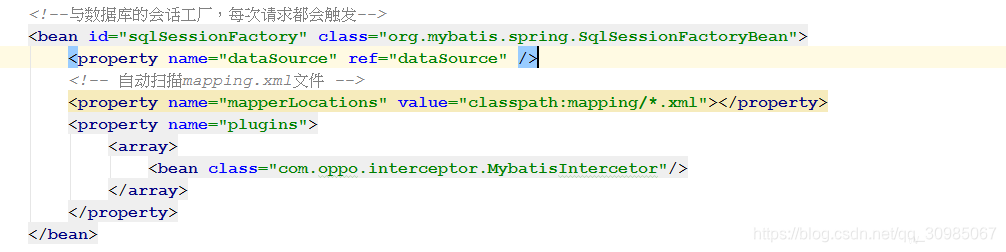
package com.oppo.interceptor;
import com.mysql.jdbc.Connection;
import com.oppo.pojo.Contract;
import org.apache.ibatis.executor.Executor;
import org.apache.ibatis.executor.statement.StatementHandler;
import org.apache.ibatis.mapping.*;
import org.apache.ibatis.plugin.*;
import org.apache.ibatis.session.ResultHandler;
import org.apache.ibatis.session.RowBounds;
import java.lang.reflect.Field;
import java.sql.Date;
import java.util.*;
@Intercepts({
@Signature(method = "query", type = Executor.class, args = {
MappedStatement.class, Object.class, RowBounds.class,
ResultHandler.class}),
})
public class MybatisIntercetor implements Interceptor {
public Object intercept(Invocation invocation) throws Throwable {
System.out.println("拦截的对象是:" + invocation.getTarget());
System.out.println("拦截方法是:" + invocation.getMethod().toString());
System.out.println("拦截参数是:" + invocation.getArgs().length);
/*里面封装了执行的方法名如:select,update,insert,delete*/
MappedStatement mappedStatement = (MappedStatement) invocation.getArgs()[0];
Object object = invocation.getArgs()[1];
//注解中method的值
String methodName = invocation.getMethod().getName();
//sql类型
SqlCommandType sqlCommandType = mappedStatement.getSqlCommandType();
Date currentDate = new Date(System.currentTimeMillis());
//对有要求的字段填值
Contract contract = null;
try {
/*重写过滤方法*/
contract=(Contract)object;
contract.setContractTempNumber("190528000195");
//BoundSql对象中封装了sql,以及参数
BoundSql boundSql = mappedStatement.getBoundSql(object);
String sql = boundSql.getSql() + " limit 0,1"; /*拼接sql*/
List<ParameterMapping> Parame = boundSql.getParameterMappings(); /*ParameterMapping中可以修改参数类型*/
System.out.print(Parame.get(0).getJdbcType());
invocation.getArgs()[0] = copyFromNewSql(mappedStatement,boundSql,sql, new ArrayList(boundSql.getParameterMappings()), object);
} catch (Exception e) {
e.printStackTrace();
}
return invocation.proceed();
}
private MappedStatement copyFromNewSql(MappedStatement ms, BoundSql boundSql,
String sql, List<ParameterMapping> parameterMappings, Object parameter) {
BoundSql newBoundSql = copyFromBoundSql(ms, boundSql, sql, parameterMappings, parameter);
SqlSource sqlSource = new BoundSqlSqlSource(newBoundSql);
return copyFromMappedStatement(ms, sqlSource);
}
public Object plugin(Object var1) {
System.out.println("插件方法1--将要包装的目标对象1:" + var1);
return Plugin.wrap(var1, this);
}
public void setProperties(Properties var1) {
System.out.print("==c==");
/*获取配置*/
}
private BoundSql copyFromBoundSql(MappedStatement ms, BoundSql boundSql,
String sql, List<ParameterMapping> parameterMappings, Object parameter) {
BoundSql newBoundSql = new BoundSql(ms.getConfiguration(),sql, parameterMappings, parameter);
for (ParameterMapping mapping : boundSql.getParameterMappings()) {
String prop = mapping.getProperty();
if (boundSql.hasAdditionalParameter(prop)) {
newBoundSql.setAdditionalParameter(prop, boundSql.getAdditionalParameter(prop));
}
}
return newBoundSql;
}
//see: MapperBuilderAssistant
private MappedStatement copyFromMappedStatement(MappedStatement ms, SqlSource newSqlSource) {
MappedStatement.Builder builder = new MappedStatement.Builder(ms.getConfiguration(),ms.getId(),newSqlSource,ms.getSqlCommandType());
builder.resource(ms.getResource());
builder.fetchSize(ms.getFetchSize());
builder.statementType(ms.getStatementType());
builder.keyGenerator(ms.getKeyGenerator());
if(ms.getKeyProperties() != null && ms.getKeyProperties().length !=0){
StringBuffer keyProperties = new StringBuffer();
for(String keyProperty : ms.getKeyProperties()){
keyProperties.append(keyProperty).append(",");
}
keyProperties.delete(keyProperties.length()-1, keyProperties.length());
builder.keyProperty(keyProperties.toString());
}
//setStatementTimeout()
builder.timeout(ms.getTimeout());
//setStatementResultMap()
builder.parameterMap(ms.getParameterMap());
//setStatementResultMap()
builder.resultMaps(ms.getResultMaps());
builder.resultSetType(ms.getResultSetType());
//setStatementCache()
builder.cache(ms.getCache());
builder.flushCacheRequired(ms.isFlushCacheRequired());
builder.useCache(ms.isUseCache());
return builder.build();
}
public static class BoundSqlSqlSource implements SqlSource {
private BoundSql boundSql;
public BoundSqlSqlSource(BoundSql boundSql) {
this.boundSql = boundSql;
}
public BoundSql getBoundSql(Object parameterObject) {
return boundSql;
}
}
}
推荐一个:https://blog.csdn.net/wwe4023/article/details/80557172