电影列表
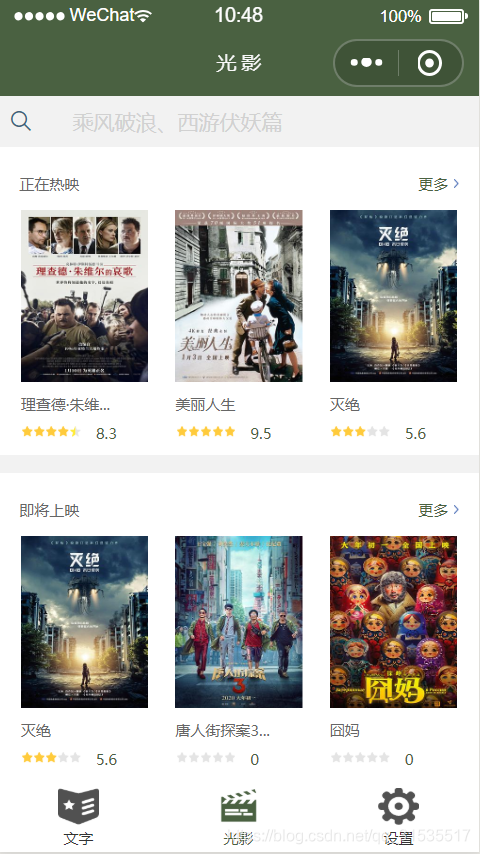
自定义星级列表
定义星星组件
<template name="starsTpl">
<view class="stars-container">
<view class="stars">
<block wx:for="{{stars}}" wx:for-item="i">
<image wx:if="{{i===1}}" src="/images/icon/wx_app_star.png"></image>
<image wx:elif="{{i===0.5}}" src="/images/icon/wx_app_star@half.png"></image>
<image wx:else="{{i===0}}" src="/images/icon/wx_app_star@none.png"></image>
</block>
</view>
<text class="star-score">{{score}}</text>
</view>
</template>
定义星星样式
.stars-container {
display: flex;
flex-direction: row;
}
.stars {
display: flex;
flex-direction: row;
height: 17rpx;
margin-right: 24rpx;
margin-top: 6rpx;
}
.stars image {
padding-left: 3rpx;
height: 17rpx;
width: 17rpx;
}
.star-score{
color: #4A6141;
}
定义电影列表item组件
编写电影item组件布局
<import src="../stars/stars-tpl.wxml" />
<template name="movieTpl">
<view class="movie-container" catchtap="onMovieTap" data-movie-id="{{movieId}}">
<image class="movie-img" src="{{coverageUrl}}"></image>
<text class="movie-title">{{title}}</text>
<template is="starsTpl" data="{{stars:stars, score: average}}" />
</view>
</template>
电影列表item组件样式
@import "../stars/stars-tpl.wxss";
.movie-container {
display: flex;
flex-direction: column;
padding: 0 22rpx;
}
.movie-img {
width: 200rpx;
height: 270rpx;
padding-bottom: 20rpx;
}
.movie-title{
margin-bottom: 16rpx;
font-size: 24rpx;
}
编写列表页的组件
编写列表页的组件布局
<import src="../single-movie/movie-tpl.wxml" />
<template name="movieListTpl">
<view class="movie-list-container">
<view class="inner-container">
<view class="movie-head">
<text class="slogan">{{categoryTitle}}</text>
<view catchtap="onMoreTap" class="more" data-category="{{categoryTitle}}">
<text class="more-text">更多</text>
<image class="more-img" src="/images/icon/wx_app_arrow_right.png"></image>
</view>
</view>
<view class="movies-container">
<block wx:for="{{movies}}" wx:for-item="movie">
<template is="movieTpl" data="{{...movie}}"/>
</block>
</view>
</view>
</view>
</template>
编写列表页的组件样式
@import "../single-movie/movie-tpl.wxss";
.movie-list-container {
background-color: #fff;
display: flex;
flex-direction: column;
}
.inner-container{
margin: 0 auto 20rpx;
}
.movie-head {
padding: 30rpx 20rpx 22rpx;
}
.slogan {
font-size: 24rpx;
}
.more {
float: right;
}
.more-text {
vertical-align: middle;
margin-right: 10rpx;
color: #4A6141;
}
.more-img {
width: 9rpx;
height: 16rpx;
vertical-align: middle;
}
.movies-container{
display:flex;
flex-direction: row;
}
编写电影页面
编写电影页组件布局
<import src="movie-list/movie-list-tpl.wxml" />
<import src="movie-grid/movie-grid-tpl.wxml" />
<view class="search">
<icon type="search" class="search-img" size="13" color="#405f80"></icon>
<input type="text" placeholder="唐人街探案3、囧妈"
placeholder-class="placeholder" bindfocus="onBindFocus" value="{{inputValue}}"
bindconfirm="onBindConfirm"/>
<image wx:if="{{searchPanelShow}}" src="/images/icon/wx_app_xx.png" class="xx-img" catchtap="onCancelImgTap"></image>
</view>
<view class="container" wx:if="{{containerShow}}">
<view class="movies-template">
<template is="movieListTpl" data="{{...inTheaters}}" />
</view>
<view class="movies-template">
<template is="movieListTpl" data="{{...comingSoon}}" />
</view>
<view class="movies-template">
<template is="movieListTpl" data="{{...top250}}"/>
</view>
</view>
<view class="search-panel" wx:if="{{searchPanelShow}}">
<template is="movieGridTpl" data="{{...searchResult}}"/>
</view>
编写电影页组件样式
@import "movie-list/movie-list-tpl.wxss";
@import "movie-grid/movie-grid-tpl.wxss";
.container {
background-color: #f2f2f2;
}
.movies-template {
margin-bottom: 30rpx;
}
.search {
background-color: #f2f2f2;
height: 80rpx;
width: 100%;
display: flex;
flex-direction: row;
}
.search-img {
margin: auto 0 auto 20rpx;
}
.search input {
height: 100%;
width: 600rpx;
margin-left: 20px;
font-size: 28rpx;
}
.placeholder {
font-size: 14px;
color: #d1d1d1;
margin-left: 20rpx;
}
.search-panel{
position:absolute;
top:80rpx;
}
.xx-img{
height: 30rpx;
width: 30rpx;
margin:auto 0 auto 10rpx;
}
接口的调用
定义域名
在app.js中添加域名
属性 | 解析 |
---|---|
App({
onLaunch: function () {
var storageData = wx.getStorageSync('postList');
if (!storageData) {
var dataObj = require("data/data.js")
wx.clearStorageSync();
wx.setStorageSync('postList', dataObj.postList);
}
},
globalData: {
g_isPlayingMusic: false,
g_currentMusicPostId: null,
doubanBase: "http://api.douban.com",
g_userInfo: null
}
})
使用域名访问接口
var util = require('../../util/util.js')
var app = getApp();
Page({
data: {
inTheaters: {},
comingSoon: {},
top250: {},
containerShow: true,
searchPanelShow: false,
searchResult: {},
},
onLoad: function (event) {
var inTheatersUrl = app.globalData.doubanBase +
"/v2/movie/in_theaters" + "?apikey=0b2bdeda43b5688921839c8ecb20399b&start=0&count=3";
var comingSoonUrl = app.globalData.doubanBase +
"/v2/movie/coming_soon" + "?apikey=0b2bdeda43b5688921839c8ecb20399b&start=0&count=3";
var top250Url = app.globalData.doubanBase +
"/v2/movie/top250" + "?apikey=0b2bdeda43b5688921839c8ecb20399b&start=0&count=3";
wx.showNavigationBarLoading();
console.log('show');
this.getMovieListData(inTheatersUrl, "inTheaters", "正在热映");
this.getMovieListData(comingSoonUrl, "comingSoon", "即将上映");
this.getMovieListData(top250Url, "top250", "豆瓣Top250");
},
getMovieListData: function (url, settedKey, categoryTitle) {
var that = this;
wx.request({
url: url,
method: 'GET',
header: {
"content-type": "json"
},
success: function (res) {
that.processDoubanData(res.data, settedKey, categoryTitle)
},
fail: function (error) {
// fail
console.log(error)
}
})
},
processDoubanData: function (moviesDouban, settedKey,
categoryTitle) {
var movies = [];
console.log(moviesDouban)
for (var idx in moviesDouban.subjects) {
var subject = moviesDouban.subjects[idx];
var title = subject.title;
if (title.length >= 6) {
title = title.substring(0, 6) + "...";
}
// [1,1,1,1,1] [1,1,1,0,0]
var temp = {
stars: util.convertToStarsArray(subject.rating.stars),
title: title,
average: subject.rating.average,
coverageUrl: subject.images.large,
movieId: subject.id
}
movies.push(temp)
}
var readyData = {};
readyData[settedKey] = {
categoryTitle: categoryTitle,
movies: movies
}
this.setData(readyData);
console.log('hide');
wx.hideNavigationBarLoading();
},
onMoreTap: function (event) {
var category = event.currentTarget.dataset.category;
wx.navigateTo({
url: "more-movie/more-movie?apikey=0b2bdeda43b5688921839c8ecb20399b&category=" + category
})
},
onMovieTap: function (event) {
var movieId = event.currentTarget.dataset.movieId;
wx.navigateTo({
url: "movie-detail/movie-detail?apikey=0b2bdeda43b5688921839c8ecb20399b&id=" + movieId
})
},
onBindFocus: function (event) {
this.setData({
containerShow: false,
searchPanelShow: true
})
},
onCancelImgTap: function (event) {
this.setData({
containerShow: true,
searchPanelShow: false,
searchResult: {},
inputValue: ''
}
)
},
onBindConfirm: function (event) {
var keyWord = event.detail.value;
var searchUrl = app.globalData.doubanBase +
"/v2/movie/search?q=" + keyWord + "&apikey=0b2bdeda43b5688921839c8ecb20399b";
this.getMovieListData(searchUrl, "searchResult", "");
}
})