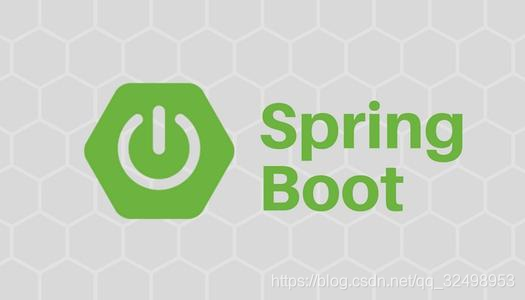
1.SpringBoot快速入门
1.简化Spring应用开发的一个框架
2.整个Spring技术栈的大整合
3.J2EE开发的一站式解决方案
1.1 环境准备
-
jdk8:Spring Boot 推荐jdk7及以上;
-
maven3.x:maven 3.3以上的版本;
-
SpringBoot 1.5.9 RELEASE;
-
IntelliJ IDEA;
1.1.1 MAVEN 设置全局的JDK
给maven的setting.xml配置文件的profiles标签添加如下内容:
<profile>
<id>jdk1.8</id>
<activation>
<activeByDefault>true</activeByDefault>
<jdk>1.8</jdk>
</activation>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<maven.compiler.compilerVersion>1.8</maven.compiler.compilerVersion>
</properties>
</profile>
1.1.2 IDEA MAVEN 配置
1.2 Spring Boot HelloWorld
功能描述:
浏览器发送hello请求,服务器接受请求并处理,响应Hello World字符串;
1.2.1 创建一个MAVEN工程
1.2.2 导入spring boot 相关的依赖
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
1.2.3 编写主程序类
/**
* @SpringBootApplication 来标注一个主程序类,说明这是一个主程序类
*/
@SpringBootApplication
public class HelloWorldMainApplication {
public static void main(String[] args) {
// Spring 应用启动起来
SpringApplication.run(HelloWorldMainApplication.class, args);
}
}
1.2.4 编写Controller,相关的Service
@Controller
public class HelloController {
@ResponseBody
@RequestMapping("/hello")
public String sayHello() {
return "hello world";
}
}
1.2.5 运行主程序
1.2.6 简化部署
<!-- 该插件可以将应用打包成可执行文件 -->
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
通过Maven 的“package”将这个应用打成jar包,直接使用java -jar的命令进行执行;
1.3 Hello World 探究
1.3.1 POM文件
1.父项目
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.9.RELEASE</version>
</parent>
<!--spring-boot-starter-parent的父项目才是真正管理Spring Boot应用里面的所有依赖版-->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>1.5.9.RELEASE</version>
<relativePath>../spring-boot-dependencies</relativePath>
</parent>
spring-boot-dependencies 是Spring Boot的仲裁中心;
有了spring-boot-dependencies管理以后导入受管理的依赖默认是不需要写版本的;
2.启动器
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
spring-boot-starter-web:
spring-boot-starter-web:spring-boot场景启动器,帮我们导入了web模块正常运行所依赖的组件;
Spring Boot将所有的功能场景都抽取出来,做成一个个的starters(启动器),只需要在项目里面引入这些starter 相关场景的所有依赖都会导入进来。要用什么功能就导入什么场景的启动器
1.3.2 主程序类,主入口类
/**
* @SpringBootApplication 来标注一个主程序类,说明1这是一个主程序类
*/
@SpringBootApplication
public class HelloWorldMainApplication {
public static void main(String[] args) {
// Spring 应用启动起来
SpringApplication.run(HelloWorldMainApplication.class, args);
}
}
@SpringBootApplicaton:Spring Boot 应用标注在某个类上面说明这个类是SpringBoot主配置类,SpringBoot就直接运行这个类的main方法来启动SpringBoot应用;
1. @SpringBootConfiguration
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@SpringBootConfiguration
@EnableAutoConfiguration
@ComponentScan(excludeFilters = {
@Filter(type = FilterType.CUSTOM, classes = TypeExcludeFilter.class),
@Filter(type = FilterType.CUSTOM, classes = AutoConfigurationExcludeFilter.class) })
public @interface SpringBootApplication {}
- @SpringBootConfiguration: Spring Boot的配置类,标注在某个类上,表示这个类是SpringBoot的配置类
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Configuration
public @interface SpringBootConfiguration {
}
- @Configuration: 配置类上来标注这个注解,配置文件,配置类也是容器中的一个组件;@Component
2.@EnableAutoConfiguration
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@SpringBootConfiguration
@EnableAutoConfiguration
@ComponentScan(excludeFilters = {
@Filter(type = FilterType.CUSTOM, classes = TypeExcludeFilter.class),
@Filter(type = FilterType.CUSTOM, classes = AutoConfigurationExcludeFilter.class) })
public @interface SpringBootApplication {}
@EnableAutoConfiguration: 开启自动配置功能,以前需要配置的动作,SpringBoot帮我们自动配置,
@EnableAutoConfiguration 告诉Spring Boot 开启自动配置功能,这样自动配置才能生效。
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@AutoConfigurationPackage
@Import(EnableAutoConfigurationImportSelector.class)
public @interface EnableAutoConfiguration {
@AutoConfigruationPackage: 自动配置包
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@Documented
@Inherited
@Import(AutoConfigurationPackages.Registrar.class)
public @interface AutoConfigurationPackage {}
@Improt(AutoConfigruationPackages.Registar.class): Spring的底层注解@Import,给容器中导入一个组件;导入的组件有AutoConfigurationPackages.Registrar.class,将主配置类(@SpringBootApplication标注的类)的所在包及下面所有子包里面的所有组件扫描到Spring容器;
@Import(EnableAutoConfigurationImportSelector.class): 给容器导入组件。
EnableAutoConfigurationImportSelector: 导入哪些组件的选择器;所有需要导入的组件以全类名的方式返回;这些组件就会被添加到容器中;会给容器中导入非常多的自动配置类(xxxAutoConfiguration);就是给容器中导入这个场景需要的所有组件, 并配置好这些组件;
有了自动配置类,免去了我们手动编写配置注入功能组件等的工作;
在EnableAutoConfigurationImportSelector的父类也就是 org.springframework.boot.autoconfigure.AutoConfigurationImportSelector#getCandidateConfigurations中
List<String> configurations = SpringFactoriesLoader.loadFactoryNames(
getSpringFactoriesLoaderFactoryClass(), getBeanClassLoader());
代码作用如下:
在SpringBoot启动的时候从类路径下的META-INF/Spring.factories中获取EnableAutoConfiguration指定的值,将 这些值作为自动配置类导入到容器中,自动配置类就生效,帮我们进行自动配置工作;以前我们需要自己配置的东 西,自动配置类都帮我们;
J2EE的整体整合解决方案和自动配置都在spring-boot-autoconfigure-version.jar;
1.4 使用 IDEA Spring Initializer快速创建Spring Boot项目
- IDE都支持使用Spring的项目创建向导快速创建一个Spring Boot项目;
- 选择我们需要的模块;向导会联网创建Spring Boot项目; 默认生成的Spring Boot项目;
1.4.1 创建步骤
步骤1
步骤2
步骤3
步骤4
步骤5
1.4.2 SpringBoot下resources文件结构
- static:保存所有的静态资源; js css images;
- templates :保存所有的模板页面;(Spring Boot默认jar包使用嵌入式的Tomcat,默认不支持JSP页 面);可以使用模板引擎(freemarker、thymeleaf);
- application.properties:Spring Boot应用的配置文件;可以修改一些默认设置;