一:题目
你好,我是悦创。
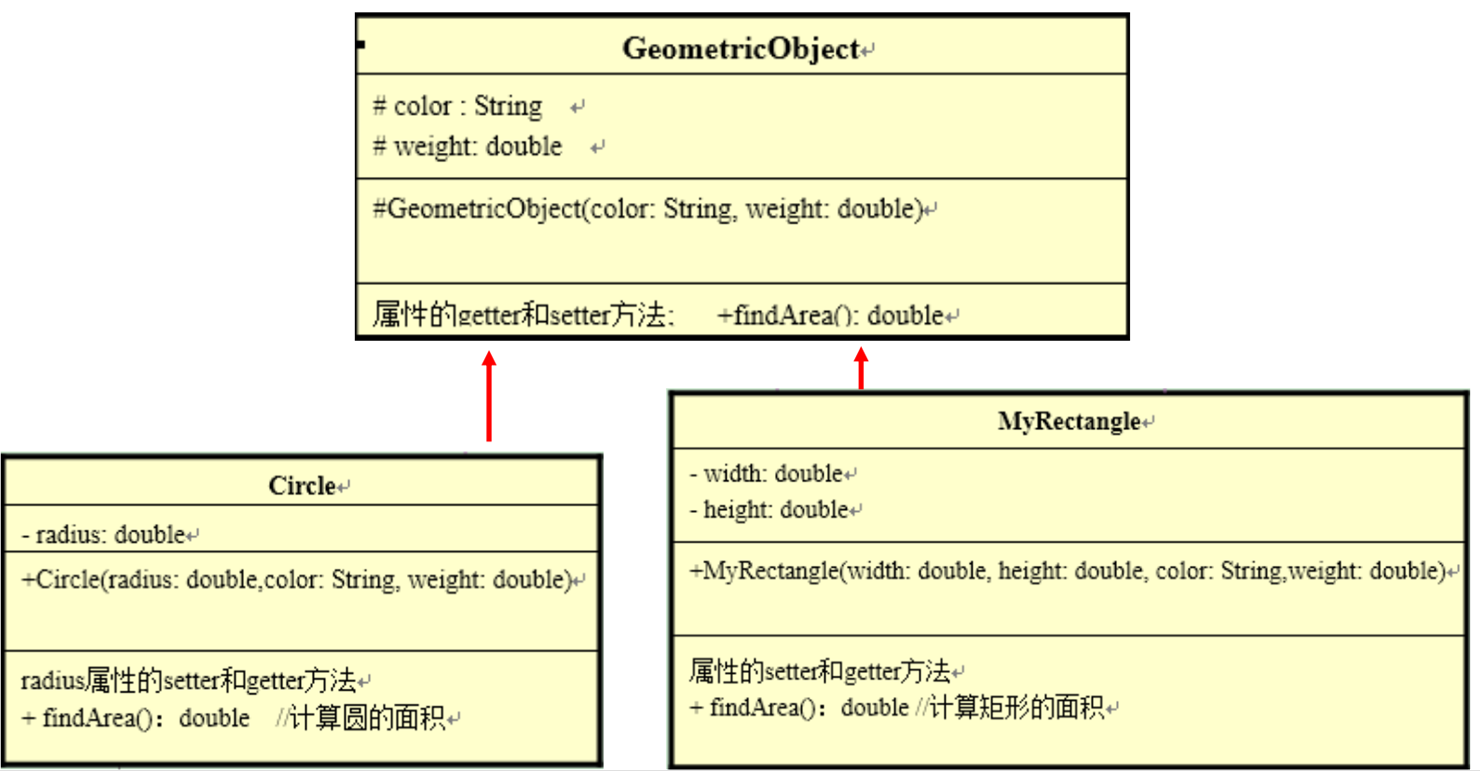
定义三个类:
- 父类
GeometricObject
代表几何形状 - 子类
Circle
代表圆形,MyRectangle
代表矩形 - 定义一个测试类
GeometricTest
,编写equalsArea
方法测试两个对象的面积是否相等(注意方法的参数类型,利用动态绑定技术),编写displayGeometricObject
方法显示对象的面积 - 注意方法的参数类型,利用动态绑定技术。
二:代码如下:
1. 父类:GeometricObject
// project = 'GeometricObject', file_name = 'GeometricObject', author = 'AI悦创(黄家宝)'
// time = '2020/3/29 13:31', product_name = Sublime Text3
// # code is far away from bugs with the god animal protecting
// I love animals. They taste delicious.
public class GeometricObject {
protected double weight;
protected String color;
public GeometricObject(double weight, String color){
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
public double findArea(){
return 0.0;
}
}
2. 子类 Circle 圆形
// project = 'Circle', file_name = 'Circle', author = 'AI悦创(黄家宝)'
// time = '2020/3/29 13:40', product_name = Sublime Text3
// # code is far away from bugs with the god animal protecting
// I love animals. They taste delicious.
public class Circle extends GeometricObject {
private double radius;
public Circle(double weight, String color, double radius) {
super(weight, color);
this.radius=radius;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double findArea(){
return 3014*radius*radius;
}
}
3. MyRectangle 代表矩形
// project = 'MyRectangle', file_name = 'MyRectangle', author = 'AI悦创(黄家宝)'
// time = '2020/3/29 13:45', product_name = Sublime Text3
// # code is far away from bugs with the god animal protecting
// I love animals. They taste delicious.
public class MyRectangle extends GeometricObject {
private double width;
private double height;
public MyRectangle(double weight, String color, double width, double height) {
super(weight, color);
this.height=height;
this.width=width;
}
public double getWidth() {
return width;
}
public void setWidth(double width) {
this.width = width;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double findArea(){
return height*width;
}
}
4. 测试类 GeometricTest
// project = 'GeometricTest', file_name = 'GeometricTest', author = 'AI悦创(黄家宝)'
// time = '2020/3/29 13:50', product_name = Sublime Text3
// # code is far away from bugs with the god animal protecting
// I love animals. They taste delicious.
public class GeometricTest {
public static void main(String[] args) {
GeometricTest t = new GeometricTest();
Circle c1 = new Circle(5.2, "black", 7.5);
Circle c2 = new Circle(5.2, "white", 7.5);
MyRectangle m1 = new MyRectangle(2.1,"red",5.7,6.6);
t.displayGeometricObject(c1);
boolean b1 = t.equalsArea(c1, c2);
boolean b2 = t.equalsArea(c1, m1);
System.out.println(b1);
System.out.println(b2);
}
public boolean equalsArea(GeometricObject g1, GeometricObject g2){
return g1.findArea() == g2.findArea();
}
public void displayGeometricObject(GeometricObject g){
System.out.println(g.findArea());
}
}