集成weex-gcanvas请参考Weex Gcanves集成和使用
先上图
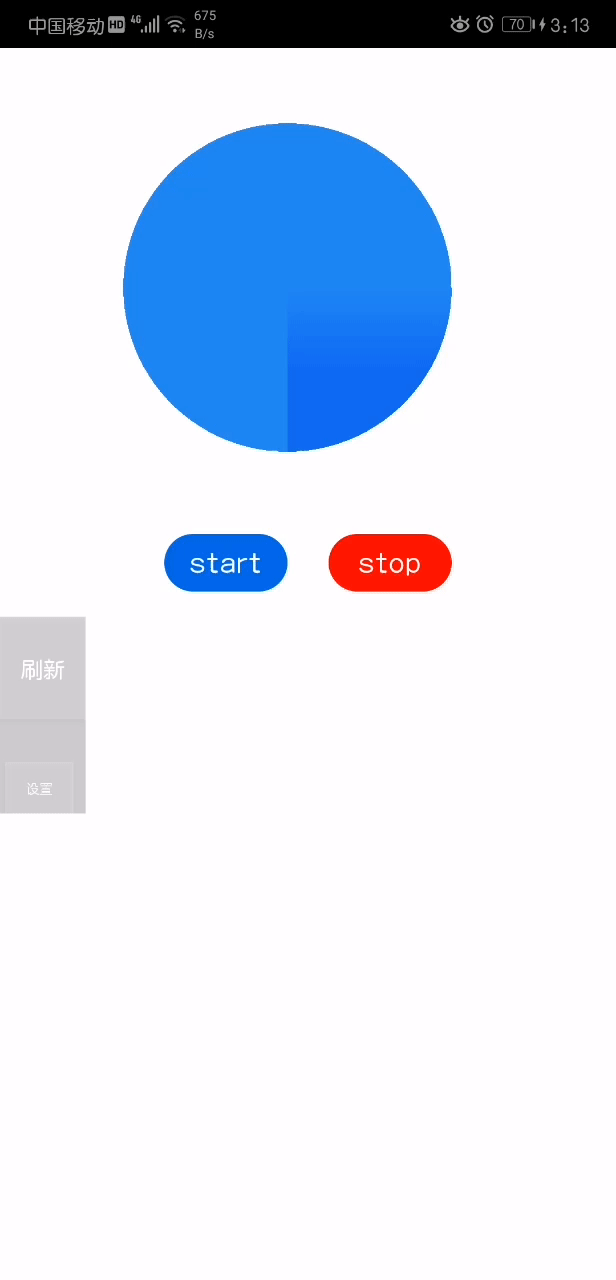
上代码:
<template>
<div ref="test" style="background-color: #ffffff">
<gcanvas @touchstart="touchstart" @touchmove="touchmove" @touchend="touchend" ref="areas"
style="width: 400px;height:400px;margin-left: 150px;
margin-top: 150px;border-radius: 200px;"></gcanvas>
<div style="width: 750px;flex-direction: row;align-items: center;justify-content: center">
<div class="button_start" @click="animation(1)">
<text style="color: white;font-size: 34px">start</text>
</div>
<div style="margin-left: 50px" class="button_stop" @click="animation(0)">
<text style="color: white;font-size: 34px">stop</text>
</div>
</div>
</div>
</template>
<style type="text/css" scoped>
.button_start{
height: 70px;width: 150px;background-color: #006ce7;margin-top: 100px;
border-radius: 35px;
align-items: center;
justify-content: center;
}
.button_stop{
height: 70px;width: 150px;background-color: #ff0000;margin-top: 100px;
border-radius: 35px;
align-items: center;
justify-content: center;
}
</style>
<script>
var libGCanvas=require("weex-gcanvas")
var animation=weex.requireModule('animation');
var modal=weex.requireModule("modal");
module.exports = {
data:{
startX:0,
startY:0,
currentX:0,
currentY:0,
context:{},
canvasObj:{},
angle:1,
doAnimation:0,
},
methods:{
animation(p){
this.doAnimation=p;
if(this.doAnimation){
this.exeAnimation();
}
},
touchstart(event){
this.startX=event.changedTouches[0].pageX;
this.startY=event.changedTouches[0].pageY;
this.context.strokeStyle = "#17acf6" ; //设置线的颜色状态
this.context.lineWidth = 10; //设置线宽状态
},
touchmove(event){
this.currentX=event.changedTouches[0].pageX;
this.currentY=event.changedTouches[0].pageY;
//进行绘制
this.context.moveTo(this.startX,this.startY);
this.context.lineTo(this.currentX,this.currentY);
this.context.stroke();
this.startX=event.changedTouches[0].pageX;
this.startY=event.changedTouches[0].pageY;
},
touchend(event){
// this.$navigator.push('login.js');
},
exeAnimation(){
let self=this;
animation.transition(self.$refs.areas,{
styles:{
transform:`rotate(${Math.PI*2*this.angle})`
},
duration:1000,
delay:0,
needLayout:false,
timingFunction:"linear"
},function () {
if(self.doAnimation){
self.angle++;
self.exeAnimation();
}
})
}
},
created: function () {
var self=this;
var globalEvent = weex.requireModule('globalEvent');
globalEvent.addEventListener("onPageInit", function (e) {
/*获取元素引用*/
var ref=self.$refs.areas;
/*通过元素引用获取canvas对象*/
self.canvasObj =libGCanvas.start(ref);
/*获取绘图所需的上下文,目前不支持3d*/
self.context = self.canvasObj.getContext('2d');
//绘制圆形
self.context.beginPath();
self.context.arc(200,200,200,0,2*Math.PI);
self.context.fillStyle="#268cf0";
self.context.fill();
//绘制渐变的扇形区域
self.context.beginPath();
self.context.moveTo(200,200);
self.context.arc(200,200,200,0,0.5*Math.PI);
var gradient=self.context.createLinearGradient(200,300,200,200);
gradient.addColorStop("0","#176cf0");
gradient.addColorStop("1.0","#268cf0");
self.context.fillStyle=gradient;
self.context.fill();
})
}
};
</script>