You are given two positive integers 𝑎a.
5 10 4 13 9 100 13 123 456 92 46
2 5 4 333 0
代码
#include<iostream>
#include<cstdio>
#include<cmath>
#include<cstring>
#include<algorithm>
#include<map>
#include<vector>
#include<queue>
#include<string>
using namespace std;
int main()
{
/* freopen("A.txt","r",stdin); */
/* freopen("Ans.txt","w",stdout); */
int t;
scanf("%d", &t);
while(t --)
{
int a,b;
scanf("%d %d", &a, &b);
if(a % b == 0)
printf("0\n");
else
printf("%d\n", (a/b + 1) * b - a);
}
return 0;
}
For the given integer $$$n$$$ ($$$n > 2$$$) let's write down all the strings of length $$$n$$$ which contain $$$n-2$$$ letters 'a' and two letters 'b' in lexicographical (alphabetical) order.
Recall that the string $$$s$$$ of length $$$n$$$ is lexicographically less than string $$$t$$$ of length $$$n$$$, if there exists such $$$i$$$ ($$$1 \le i \le n$$$), that $$$s_i < t_i$$$, and for any $$$j$$$ ($$$1 \le j < i$$$) $$$s_j = t_j$$$. The lexicographic comparison of strings is implemented by the operator < in modern programming languages.
For example, if $$$n=5$$$ the strings are (the order does matter):
- aaabb
- aabab
- aabba
- abaab
- ababa
- abbaa
- baaab
- baaba
- babaa
- bbaaa
It is easy to show that such a list of strings will contain exactly $$$\frac{n \cdot (n-1)}{2}$$$ strings.
You are given $$$n$$$ ($$$n > 2$$$) and $$$k$$$ ($$$1 \le k \le \frac{n \cdot (n-1)}{2}$$$). Print the $$$k$$$-th string from the list.
The input contains one or more test cases.
The first line contains one integer $$$t$$$ ($$$1 \le t \le 10^4$$$) — the number of test cases in the test. Then $$$t$$$ test cases follow.
Each test case is written on the the separate line containing two integers $$$n$$$ and $$$k$$$ ($$$3 \le n \le 10^5, 1 \le k \le \min(2\cdot10^9, \frac{n \cdot (n-1)}{2})$$$.
The sum of values $$$n$$$ over all test cases in the test doesn't exceed $$$10^5$$$.
For each test case print the $$$k$$$-th string from the list of all described above strings of length $$$n$$$. Strings in the list are sorted lexicographically (alphabetically).
7 5 1 5 2 5 8 5 10 3 1 3 2 20 100
aaabb aabab baaba bbaaa abb bab aaaaabaaaaabaaaaaaaa
思路
- 题意:给我们n个a字符序列,选择其中两个位置替换成b字符,得到的这个序列是所有的替换序列中 低k大的那么,输出这个序列
- 分析:其实 字符串的大小与两个b字符的位置密切相关,那么替换后最小的字符串就是两个 b都在所给的序列的最后两个位置,这个时候要想让得到的结果学列变大的话,我们可以让倒数第二b字符往前挪移1(此时这个b在第三个位置),那么倒数第一个b,此时有两个位置可以选择,要么选择倒数第一个,要么倒数第二个,选择倒数第二个比倒数第一个位置的得到的字符串更大,这样我们在 第二个b 在倒数第四个位置,在这个基础上在观察 第一个b 此时可以选择的位置,就可以找到规律了
代码
#include<iostream>
#include<cstdio>
#include<cmath>
#include<cstring>
#include<algorithm>
#include<map>
#include<vector>
#include<queue>
#include<string>
using namespace std;
int main()
{
/* freopen("A.txt","r",stdin); */
/* freopen("Ans.txt","w",stdout); */
int t;
scanf("%d", &t);
while(t --)
{
int a,b;
scanf("%d %d", &a, &b);
int i = 0;
while(b > 0)
{
i ++;
b -= i;
}
int p1 = a - i;
int p2 = a - (i + b) + 1;
for(int i = 1; i <= a; i ++)
{
if(i == p1 || i == p2)
printf("b");
else
printf("a");
}
printf("\n");
}
return 0;
}
A number is ternary if it contains only digits 00 is the minimum possible. If there are several answers, you can print any.
4 5 22222 5 21211 1 2 9 220222021
11111 11111 11000 10211 1 1 110111011 110111010
思路
- 分析:这个也是根据 所给的样例观察所怎么构造两个序列,要先让这个两个序列最大的尽可能的小,我们在构造的时候可以默认 把第一个序列在符合题意的基础上有意的把第一个构造的更大一些,当给力我们一个目标序列(这个目标序列中只包含 0 、1、2)之后我们从左到右一位一位的根据这一位的数值分类进行讨论就行了,如果这一位是 2、0,那么我们均分 2、0 到 相应的两个构造序列位置上就行了,如果是1 ,显然它不能均分,
对于第一个1
我们可以直接把它放到第一个构造序列中,那么另一个构造序列就是0,那么这时候要注意了,在这个分割以后无论我怎么分割目标序列某一位置的数,都不会影响两个构造序列的相对大小了,,,所以为了是最大的构造序列(这个时候就是第一个序列)尽可能的小,把剩下的数都分给 第二个序列就行了。
代码
#include<iostream>
#include<cstdio>
#include<cmath>
#include<cstring>
#include<algorithm>
#include<map>
#include<vector>
#include<queue>
#include<string>
using namespace std;
const int Len = 5e5 + 10;
int main()
{
/* freopen("A.txt","r",stdin); */
/* freopen("Ans.txt","w",stdout); */
int t;
scanf("%d", &t);
while(t --)
{
char a[Len],b[Len],c[Len];
int n;
scanf("%d", &n);
scanf("%s", a + 1);
b[1] = c[1] = '1';
int flag = 1;
int i;
for(i = 2; i <= n; i ++)
{
if(a[i] % 2 == 0 && flag)
{
b[i] = c[i] = (a[i] - '0')/2 + '0';
}
else if(flag)
{
flag = 0;
b[i] = a[i];
c[i] = '0';
}
else
{
b[i] = '0';
c[i] = a[i];
}
}
b[i] = c[i] = '\0';
printf("%s\n%s\n", b+1, c+1);
}
return 0;
}
The round carousel consists of $$$n$$$ figures of animals. Figures are numbered from $$$1$$$ to $$$n$$$ in order of the carousel moving. Thus, after the $$$n$$$-th figure the figure with the number $$$1$$$ follows. Each figure has its own type — the type of the animal corresponding to this figure (the horse, the tiger and so on). The type of animal of the $$$i$$$-th figure equals $$$t_i$$$.
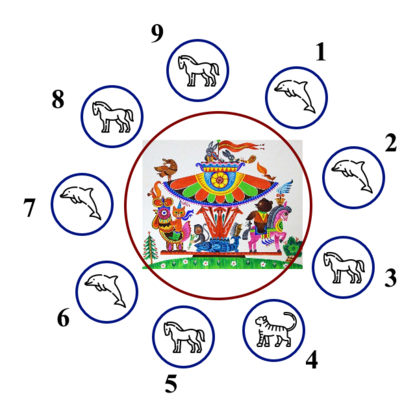
You want to color each figure in one of the colors. You think that it's boring if the carousel contains two different figures (with the distinct types of animals) going one right after another and colored in the same color.
Your task is to color the figures in such a way that the number of distinct colors used is the minimum possible and there are no figures of the different types going one right after another and colored in the same color. If you use exactly $$$k$$$ distinct colors, then the colors of figures should be denoted with integers from $$$1$$$ to $$$k$$$.
The input contains one or more test cases.
The first line contains one integer $$$q$$$ ($$$1 \le q \le 10^4$$$) — the number of test cases in the test. Then $$$q$$$ test cases follow. One test case is given on two lines.
The first line of the test case contains one integer $$$n$$$ ($$$3 \le n \le 2 \cdot 10^5$$$) — the number of figures in the carousel. Figures are numbered from $$$1$$$ to $$$n$$$ in order of carousel moving. Assume that after the $$$n$$$-th figure the figure $$$1$$$ goes.
The second line of the test case contains $$$n$$$ integers $$$t_1, t_2, \dots, t_n$$$ ($$$1 \le t_i \le 2 \cdot 10^5$$$), where $$$t_i$$$ is the type of the animal of the $$$i$$$-th figure.
The sum of $$$n$$$ over all test cases does not exceed $$$2\cdot10^5$$$.
Print $$$q$$$ answers, for each test case print two lines.
In the first line print one integer $$$k$$$ — the minimum possible number of distinct colors of figures.
In the second line print $$$n$$$ integers $$$c_1, c_2, \dots, c_n$$$ ($$$1 \le c_i \le k$$$), where $$$c_i$$$ is the color of the $$$i$$$-th figure. If there are several answers, you can print any.
4 5 1 2 1 2 2 6 1 2 2 1 2 2 5 1 2 1 2 3 3 10 10 10
2 1 2 1 2 2 2 2 1 2 1 2 1 3 2 3 2 3 1 1 1 1 1
思路
题意:给我们一个有n个数的序列,把这个序列首位相连,要求这个序列中相邻位置 如果是不同数字 不能够 图相邻的颜色,如果是相同的数字就可以涂成相同的颜色(⚠️相同数字也可以涂成不同的颜色),问我们最少需要几种颜色,才能图的符合题意
思路:分类讨论
1.如果序列全是相同的元素 -> 只需一种颜色
2.如果序列是元素个数是偶数 -> 只需两种颜色,间隔涂色 1 2 1 2 1 2 1 2
3.如果序列是奇数个元素
1.如果在这个序列中 有相邻两个元素重复 -> 把第一个重复的元素填成与之前那个相同,这样剩下的 就可当成偶数元素个数的图色方法了
2. 如果相邻位置没有重复的,那么我们接下来要讨论 这个序列的 首位元素是否相同
1.如果不同 -> 按 1 2 1 2 1 2 3 来填写
2.如果相同-> 按 1 2 1 2 1 来填写
代码
#include<iostream>
#include<cstdio>
using namespace std;
const int Len = 2e5 + 10;
int ar[Len];
int main()
{
/* freopen("A.txt","r",stdin); */
/* freopen("Res.txt","w", stdout); */
int t;
scanf("%d", &t);
while(t --)
{
int n;
scanf("%d", &n);
for(int i = 1; i <= n; i ++)
scanf("%d", &ar[i]);
bool all_same = 1;
for(int i = 2; i <= n; i ++)
if(ar[i] != ar[1])
{
all_same = 0;
break;
}
if(all_same)
{
printf("1\n");
for(int i = 1; i <= n; i ++)
printf("1 ");
}
else if(n%2)
{
bool is_repeat = 0;
for(int i = 2; i <= n; i ++)
if(ar[i] == ar[i - 1])
{
is_repeat = 1;
break;
}
if(is_repeat)
{
printf("2\n");
ar[0] = 1e9 + 7;
bool first_repeat = 1;
int last = 2;
for(int i = 1; i <= n; i ++)
{
if(ar[i] != ar[i - 1])
{
if(last == 1)
printf("2 "), last = 2;
else
printf("1 "), last = 1;
}
else if(first_repeat)
{
if(last == 1)
printf("1 ");
else
printf("2 ");
first_repeat = 0;
}
else
{
if(last == 1)
printf("2 "), last = 2;
else
printf("1 "), last = 1;
}
}
}
else
{
if(ar[1] != ar[n])
{
printf("3\n");
for(int i = 1; i <= n; i ++)
{
if(i == n)
printf("3");
else if(i%2)
printf("1 ");
else
printf("2 ");
}
}
else
{
printf("2\n");
for(int i = 1; i <= n; i ++)
{
if(i%2)
printf("1 ");
else
printf("2 ");
}
}
}
}
else
{
printf("2\n");
for(int i = 1; i <= n; i ++)
{
if(i%2)
printf("1 ");
else
printf("2 ");
}
}
printf("\n");
}
return 0;
}