数据结构之顺序栈实现
代码及思想
#include <iostream>
#include <cstdio>
#include <cstdlib>
using namespace std;
#define MAXSIZE 100
#define INCREASE 10
#define OK 1
#define ERROR 0
typedef int ElemType;
typedef int Status;
typedef struct
{
ElemType *data;
ElemType top;
int stackSize;
}SqStack;
Status SqStackInit(SqStack &S);
bool IsEmpty(SqStack &S);
Status GetTop(SqStack &S,ElemType &x);
Status Push(SqStack &S,ElemType x);
Status Pop(SqStack &S,ElemType &x);
int SqStackLength(SqStack S);
void SqStackTraverse(SqStack S);
void SqStackDestroy(SqStack &S);
Status SqStackInit(SqStack &S)
{
S.data = (ElemType *)malloc(MAXSIZE * sizeof(ElemType));
if(!S.data)
return ERROR;
S.top = -1;
S.stackSize = MAXSIZE;
return OK;
}
bool IsEmpty(SqStack &S)
{
return S.top == -1 ? true : false;
}
Status GetTop(SqStack &S,ElemType &x)
{
if(IsEmpty(S))
return ERROR;
x = S.data[S.top];
return OK;
}
Status Push(SqStack &S,ElemType x)
{
if(S.top >= S.stackSize)
{
S.data = (ElemType *)realloc(S.data,(S.stackSize + INCREASE));
if(!S.data)
return ERROR;
S.stackSize += INCREASE;
}
S.data[++S.top] = x;
return OK;
}
Status Pop(SqStack &S,ElemType &x)
{
if(IsEmpty(S))
{
return ERROR;
}
x = S.data[S.top--];
return OK;
}
int StackLength(SqStack S)
{
return S.top+1;
}
void StackTraverse(SqStack S)
{
while(S.top != -1)
{
cout<<S.data[S.top]<<" ";
S.top--;
}
cout<<endl;
}
void SqStackDestroy(SqStack &S)
{
free(S.data);
S.top = -1;
S.stackSize = MAXSIZE;
}
int main()
{
SqStack S;
int topNum,popNum=0;
SqStackInit(S);
cout<<"1、初始化输入1-10 "<<endl;
for(int i=1;i<11;i++)
Push(S,i);
StackTraverse(S);
cout<<"*栈长为"<<StackLength(S)<<endl;
cout<<endl;
GetTop(S,topNum);
cout<<"2、取栈顶元素"<<"*为:"<<topNum<<endl;
cout<<endl;
Pop(S,popNum);
cout<<"3、输出栈顶元素为"<<popNum<<endl;
StackTraverse(S);
cout<<"**栈长为"<<StackLength(S)<<endl;
SqStackDestroy(S);
return 0;
}
测试截图
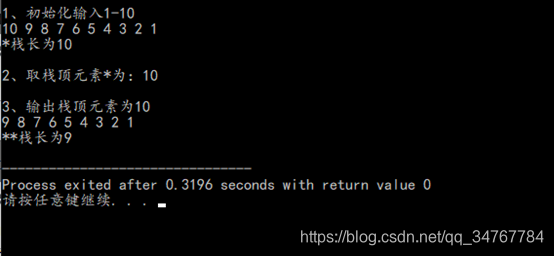