package com.wqh.fsrm.common.util;
import java.text.DecimalFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.time.temporal.ChronoUnit;
import java.util.Calendar;
import java.util.Date;
import java.util.Random;
import static java.time.format.DateTimeFormatter.ofPattern;
public class DateUtils {
/**
* Date 转 String
* @param date
* @param format 格式
* @return
*/
public static String dateToString(Date date,String format){
SimpleDateFormat sdf = new SimpleDateFormat(format);
String str = sdf.format(date);
return str;
}
/**
* String 转 Date
* @param str
* @param format 格式
* @return
*/
public static Date stringToDate(String str,String format){
SimpleDateFormat sdf = new SimpleDateFormat(format);
Date date = null;
try {
date = sdf.parse(str);
} catch (ParseException e) {
e.printStackTrace();
}
return date;
}
/**
* LocalDateTime 转 String
* @param localDateTime
* @param format
* @return
*/
public static String localDateTimeToString(LocalDateTime localDateTime,String format){
DateTimeFormatter df = DateTimeFormatter.ofPattern(format);
String str = df.format(localDateTime);
return str;
}
/**
* String 转 LocalDateTime
* @param str
* @param format
* @return
*/
public static LocalDateTime stringToLocalDateTime(String str,String format){
DateTimeFormatter df = DateTimeFormatter.ofPattern(format);
LocalDateTime localDateTime = LocalDateTime.parse(str,df);
return localDateTime;
}
/**
* Date 转 LocalDateTime
* @param date
* @return
*/
public static LocalDateTime dateToLocalDateTime(Date date){
Instant instant = date.toInstant();
ZoneId zoneId = ZoneId.systemDefault();
LocalDateTime localDateTime = instant.atZone(zoneId).toLocalDateTime();
return localDateTime;
}
/**
* LocalDateTime 转 Date
* @param localDateTime
* @return
*/
public static Date localDateTimeToDate(LocalDateTime localDateTime){
ZoneId zoneId = ZoneId.systemDefault();
ZonedDateTime zdt = localDateTime.atZone(zoneId);
Date date = Date.from(zdt.toInstant());
return date;
}
/**
* date 转 localDate
* @param date
* @return
*/
public static LocalDate dateToLocalDate(Date date){
Instant instant = date.toInstant();
ZoneId zoneId = ZoneId.systemDefault();
LocalDate localDate = instant.atZone(zoneId).toLocalDate();
return localDate;
}
/**
* localDate 转 date
* @param localDate
* @return
*/
public static Date localDateToDate(LocalDate localDate){
ZoneId zoneId = ZoneId.systemDefault();
ZonedDateTime zdt = localDate.atStartOfDay().atZone(zoneId);
Date date = Date.from(zdt.toInstant());
return date;
}
/**
* localDate 转 String
* @param localDate
* @param format
* @return
*/
public static String localDateToString(LocalDate localDate,String format){
DateTimeFormatter dtf = ofPattern(format);
String localDateString = dtf.format(localDate);
return localDateString;
}
/**
* string 转 localDate
* @param str
* @param format
* @return
*/
public static LocalDate stringToLocalDate(String str,String format){
DateTimeFormatter dtf = ofPattern(format);
LocalDate localDate = LocalDate.parse(str, dtf);
return localDate;
}
/**
* 获取到毫秒级时间戳
* @param localDateTime 具体时间
* @return long 毫秒级时间戳
*/
public static long toEpochMilli(LocalDateTime localDateTime){
return localDateTime.toInstant(ZoneOffset.of("+8")).toEpochMilli();
}
/**
* 毫秒级时间戳转 LocalDateTime
* @param epochMilli 毫秒级时间戳
* @return LocalDateTime
*/
public static LocalDateTime ofEpochMilli(long epochMilli){
return LocalDateTime.ofInstant(Instant.ofEpochMilli(epochMilli), ZoneOffset.of("+8"));
}
/**
* 获取到秒级时间戳
* @param localDateTime 具体时间
* @return long 秒级时间戳
*/
public static long toEpochSecond(LocalDateTime localDateTime){
return localDateTime.toEpochSecond(ZoneOffset.of("+8"));
}
/**
* 秒级时间戳转 LocalDateTime
* @param epochSecond 秒级时间戳
* @return LocalDateTime
*/
public static LocalDateTime ofEpochSecond(long epochSecond){
return LocalDateTime.ofEpochSecond(epochSecond, 0,ZoneOffset.of("+8"));
}
/**
* Date 增加 num 时间 num为负数时减少num时间
* @param date
* @param num
* @return
*/
public static Date addDate(Date date,int num){
Calendar cd = Calendar.getInstance();
cd.setTime(date);
cd.add(Calendar.DATE, num);//增加几天
//cd.add(Calendar.MONTH, num);//增加几个月
return cd.getTime();
}
/**
* 比较 date1 和 date2 大小 1 date1 大于 date2 -1 date1 小于 date 2
* @param date1
* @param date2
* @return
*/
public static int dateCompare(Date date1,Date date2){
if(date1.after(date2)){
return 1;
}else{
return -1;
}
}
/**
* 在当前时间上添加时间
* time 为毫秒long类型
* @param
* @return
*/
public static Date getDate(long time) {
long currentTime = System.currentTimeMillis();
currentTime+=time;
Date date = new Date(currentTime);
return date;
}
/**
* localDate 加减一天
* @param localDate
* @param days
* @return
*/
public static LocalDate localDateChangeDays(LocalDate localDate,int days){
return localDate.plusDays(days);
}
/**
* 计算两个localDateTime 相差天数
* @param localDateTimeMax
* @param localDateTimeMin
* @return
*/
public static Long localDateSubtract(LocalDateTime localDateTimeMax,LocalDateTime localDateTimeMin){
long days = localDateTimeMin.until(localDateTimeMax, ChronoUnit.DAYS);
return days;
}
/**
* 计算两个 localDateTime 相差
* @param localDateTime1
* @param localDateTime2
* @return
*/
public static Long localDateTimeDiffer(LocalDateTime localDateTime1,LocalDateTime localDateTime2,String type){
Duration duration = Duration.between(localDateTime1,localDateTime2);
Long differ = null;
if("days".equals(type)){
Long days = duration.toDays(); //相差的天数
differ = days;
}else if("hours".equals(type)){
Long hours = duration.toHours();//相差的小时数
differ = hours;
}else if("minutes".equals(type)){
Long minutes = duration.toMinutes();//相差的分钟数
differ = minutes;
}else if("millis".equals(type)){
Long millis = duration.toMillis();//相差毫秒数
differ = millis;
}else if("nanos".equals(type)){
Long nanos = duration.toNanos();//相差的纳秒数
differ = nanos;
}
return differ;
}
public void ShowTimeInterval(Date date1, Date date2) {
long lDate1 = date1.getTime();
long lDate2 = date2.getTime();
long diff = (lDate1 < lDate2) ? (lDate2 - lDate1) : (lDate1 - lDate2);
long day = diff / (24 * 60 * 60 * 1000);
long hour = diff / (60 * 60 * 1000) - day * 24;
long min = diff / (60 * 1000) - day * 24 * 60 - hour * 60;
long sec = diff / 1000 - day * 24 * 60 * 60 - hour * 60 * 60 - min * 60;
System.out.println("date1 与 date2 相差 " + day + "天" + hour + "小时" + min + "分" + sec + "秒");
}
public static String generateTimestampNo() {
String tradeNo = "";
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMddHHmmssSSS");
tradeNo += sdf.format(new Date());
Random rand = new Random(Thread.currentThread().getId() ^ System.nanoTime());
tradeNo += new DecimalFormat("0000").format(rand.nextInt(10000));
return tradeNo;
}
public static void main(String[] args) {
// LocalDateTime end = LocalDateTime.now();
// LocalDateTime start = stringToLocalDateTime("2021-03-03 12:00:00","yyyy-MM-dd HH:mm:ss");
// System.out.println("计算两个时间之差");
// //LocalDateTime end = LocalDateTime.now();
// Duration duration = Duration.between(start,end);
// long days = duration.toDays(); //相差的天数
// long hours = duration.toHours();//相差的小时数
// long minutes = duration.toMinutes();//相差的分钟数
// long millis = duration.toMillis();//相差毫秒数
// long nanos = duration.toNanos();//相差的纳秒数
//
// LocalDateTime lastDate = start.plusNanos(nanos);
// System.out.println(days);
// System.out.println(hours);
// System.out.println(minutes);
// System.out.println(millis);
// System.out.println(nanos);
// System.out.println(start);
// System.out.println(end);
// System.out.println(lastDate);
// System.out.println(toEpochSecond(LocalDateTime.now()));
// System.out.println(ofEpochSecond(1630119933L));
String str = DateUtils.localDateTimeToString(LocalDateTime.now(),"yyyy-MM-dd'T'HH:mm:ss'Z'");
System.out.println(str);
}
}
java DateUtils时间转化工具类
最新推荐文章于 2024-06-22 11:08:31 发布
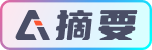