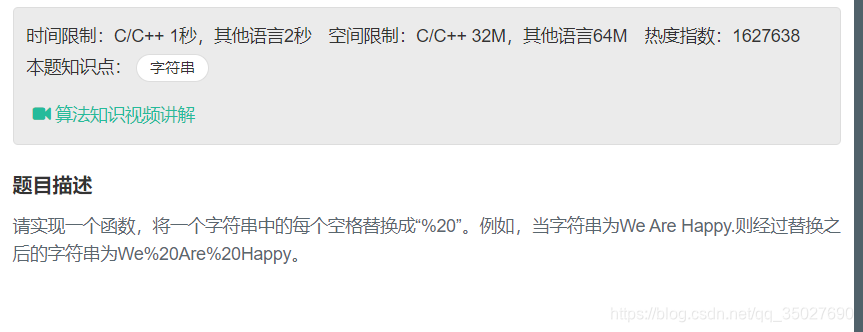
1 从前往后遍历
假如从前往后替换,那么遍历时,每出现一个空格,都需要将该空格后面的字符移动3个位置。
假若从后往前替换,在已知空格数的前提下,每个字符只需要移一次。
2 从后往前遍历
用双指针p1和p2,p1指向原字符串末尾,p2指向替换空格后字符串末尾;
每次循环时,将p1指向的内容赋给p2指向的内容;
当p1指向空格时,p2指向内容依次赋值为0,2,%。
直到p1=p2时,说明空格已经替换完了。
可以自己代入特殊情况验证。
#include<iostream>
using namespace std;
void replaceSpace(char *str,int length)
{
if(str == nullptr||length <=0) return; //用c++11编译
int space_count = 0;
for(int i = 0;i < length; i++)
{
if(str[i] == ' ')
space_count++;
}
if(!space_count) return;
int new_length = length + 2*space_count;
char *p1 = str + length;
char *p2 = str + new_length;
while(p1 != p2)
{
if(*p1 == ' ')
{
*p2-- = '0';
*p2-- = '2';
*p2-- = '%';
}
else
{
*p2-- = *p1;
}
p1--;
}
}
int main()
{
int i;
int length = 12;
char str[length] = {"we are happy"};
replaceSpace(str, length);
while(str[i] != '\0')
{
cout<<str[i];
i++;
}
return 0;
}
二刷时发现牛客网已经更新,现粘上代码
class Solution {
public:
/**
* 代码中的类名、方法名、参数名已经指定,请勿修改,直接返回方法规定的值即可
*
*
* @param s string字符串
* @return string字符串
*/
string replaceSpace(string s) {
// write code here
int count=0;
for(int i=0;i<s.size();i++)
{
if(s[i]==' ')
count++;
}
int i= s.size()-1;
int j=s.size()-1+2*count;
string s1(s.size()+2*count,'.');
while(i>=0)
{
if(s[i]== ' ')
{
s1[j]='0';
j--;
s1[j]='2';
j--;
s1[j]='%';
i--;
j--;
}
else
{
s1[j] = s[i];
i--;
j--;
}
}
return s1;
}
};
3 妙用STL 算法
利用string 中的replace 函数,找到空格后即替换。
class Solution {
public:
/**
* 代码中的类名、方法名、参数名已经指定,请勿修改,直接返回方法规定的值即可
*
*
* @param s string字符串
* @return string字符串
*/
string replaceSpace(string s) {
// write code here
if(s.empty()) return s;
for(int i=0; i<s.size();i++)
if(s[i]==' ')
s.replace(i,1,"%20");
return s;
}
};