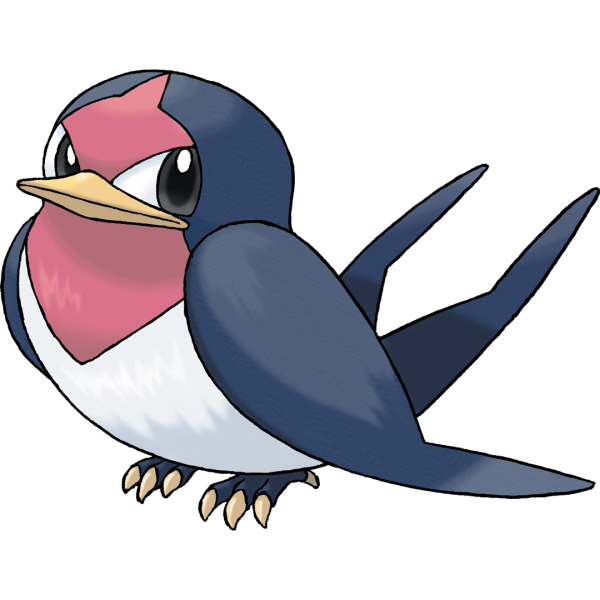
UITabBarController 和UINavigationController类似(可以相互嵌套使用),UITabBarController也可以管理多个控制器,完成控制器之间的切换
一般步骤就是下面这样
- 创建初始化UITabBarController
- 设置UIWindow的rootViewController为UITabBarController
- 创建相应的子控制器(viewcontroller)
- 把子控制器添加到UITabBarController
代码实例: 有六个标签的UITabBarController
首先是建立六个视图控制器并引入(在AppDelegate里面演示的)
#import"FirstViewController.h"
#import"SecondViewController.h"
#import"ThreeViewController.h"
#import"ForthViewController.h"
#import"FiveViewController.h"
#import"SixViewController.h"
创建Window:
self.window = [[UIWindow alloc] initWithFrame:[UIScreen mainScreen].bounds];
self.window.backgroundColor = [UIColor cyanColor];
[self.window makeKeyAndVisible];
创建子视图控制器:
//第一个标签
FirstViewController *firstVC = [FirstViewController new];
UINavigationController *firstNC = [[UINavigationController alloc] initWithRootViewController:firstVC];
// 第一种设置tabBar外观方法(系统样式)
// 参数1:系统tabbar外观方法(系统样式)
// 参数2:tabbarItem的tag值
firstNC.tabBarItem= [[UITabBarItem alloc] initWithTabBarSystemItem:(UITabBarSystemItemHistory) tag:101];
//第二个标签
SecondViewController *secondVC = [SecondViewController new];
UINavigationController *secondNC = [[UINavigationController alloc] initWithRootViewController:secondVC];
//第二种设置tabbar外观方法(自定义tabbar样式)
UIImage *secondNCimage = [UIImage imageNamed:@"carGary"];
//未选中时展示的图片
//图片不被渲染,保持图片本身样式
secondNCimage = [secondNCimage imageWithRenderingMode:(UIImageRenderingModeAlwaysOriginal)];
//选中时的图片
UIImage *secondSelectedImage = [UIImage imageNamed:@"carRed"];
secondSelectedImage = [secondSelectedImage imageWithRenderingMode:(UIImageRenderingModeAlwaysOriginal)];
//设置tabbarItem外观
//参数1:tabBar标题
//参数2:未选中时展示的图片
//参数3:选中时展示的图片
secondNC.tabBarItem = [[UITabBarItem alloc] initWithTitle:@"第二页" image:secondNCimage selectedImage:secondSelectedImage];
//第三个标签
ThreeViewController *threeVC = [ThreeViewController new];
UINavigationController *threeNC = [[UINavigationController alloc] initWithRootViewController:threeVC];
//第二种设置tabbar外观方法(自定义tabbar样式)
UIImage *threeNCimage = [UIImage imageNamed:@"findGray"];//未选中时展示的图片
//图片不被渲染,保持图片本身样式
threeNCimage = [threeNCimage imageWithRenderingMode:(UIImageRenderingModeAlwaysOriginal)];
//选中时的图片
UIImage *threeSelectedImage = [UIImage imageNamed:@"findRed"];
threeSelectedImage = [threeSelectedImage imageWithRenderingMode:(UIImageRenderingModeAlwaysOriginal)];
//设置tabbarItem外观
//参数1:tabBar标题
//参数2:未选中时展示的图片
//参数3:选中时展示的图片
threeNC.tabBarItem = [[UITabBarItem alloc] initWithTitle:@"第三页" image:threeNCimage selectedImage:threeSelectedImage];
//第四个标签
ForthViewController *fourthVC = [ForthViewController new];
UINavigationController *forthNC = [[UINavigationController alloc] initWithRootViewController:fourthVC];
//第二种设置tabbar外观方法(自定义tabbar样式)
UIImage *forthNCimage = [UIImage imageNamed:@"planeGary"];//未选中时展示的图片
//图片不被渲染,保持图片本身样式
forthNCimage = [forthNCimage imageWithRenderingMode:(UIImageRenderingModeAlwaysOriginal)];
//选中时的图片
UIImage *forthSelectedImage = [UIImage imageNamed:@"planeRed"];
forthSelectedImage = [forthSelectedImage imageWithRenderingMode:(UIImageRenderingModeAlwaysOriginal)];
//设置tabbarItem外观
//参数1:tabBar标题
//参数2:未选中时展示的图片
//参数3:选中时展示的图片
forthNC.tabBarItem = [[UITabBarItem alloc] initWithTitle:@"第四页" image:forthNCimage selectedImage:forthSelectedImage];
#pragma mark --- 当tabBar管理的试图控制器多于四个的时候
// tabBar 一般要求是四个控制器就足够
// 第五个标签
FifthViewController *fiveVC = [FifthViewController new];
UINavigationController *fiveNC = [[UINavigationController alloc] initWithRootViewController:fiveVC];
fiveNC.tabBarItem = [[UITabBarItem alloc] initWithTitle:@"第五页" image:forthNCimage selectedImage:forthSelectedImage];
// 第六个标签
SixViewController *sixVC = [SixViewController new];
UINavigationController *sixNC = [[UINavigationController alloc] initWithRootViewController:sixVC];
sixNC.tabBarItem = [[UITabBarItem alloc] initWithTitle:@"第六页" image:threeNCimage selectedImage:threeSelectedImage];
// 1:创建一个TabBarController
// TabBar高度是49;
UITabBarController *mainTabBar = [UITabBarController new];
// 2:设置TabBarController的子视图控制器数组
mainTabBar.viewControllers = @[firstNC,secondNC,threeNC,forthNC,fiveNC,sixNC];
// 3:将根视图控制器设置为TabBarController
[self.window setRootViewController:mainTabBar];
// 4:设置是否半透明
mainTabBar.tabBar.translucent=YES;
// TabBar属性
// 设置TabBar选中的颜色
[mainTabBar.tabBar setTintColor:[UIColor greenColor]];
// 设置TabBar背景颜色
[mainTabBar.tabBar setBarTintColor:[UIColor purpleColor]];
// 改变tabBar位置
// [secondNC.tabBarItem setTitlePositionAdjustment:UIOffsetMake(5, 5)];
// 设置进入应用默认选中第几个
mainTabBar.selectedIndex = 2;
// 设置全局外观
// 通过[UITabBar appearance]得到当前应用的UITabBar对象来设置tabBar外观
// 注意:设置全局外观最好在appdelegate里,否则会无效
// appearce本质是系统封装好的单例 小数据的情况下,没有必要使用单例;单例;单例是全局的,它的生命周期和程序一样长
// 使用appearce去修改相应的控件外观,应该在APPDelegate中进行设置,否则容易出现无效的情况.
[[UITabBar appearance] setBarTintColor:[UIColor blueColor]];
// 设置导航栏
[[UINavigationBar appearance] setTintColor:[UIColor yellowColor]];
// 设置代理人
mainTabBar.delegate = self;
//实现协议中的方法(< UITabBarControllerDelegate >)
//当点击某个标签时,(tabBarItem)触发该方法
-(void)tabBarController:(UITabBarController *)tabBarController didSelectViewController:(UIViewController *)viewController
{
NSLog(@"%ld",tabBarController.selectedIndex);
}