// 常用工具类
// npm i element-ui axios router
import axios from "axios";
import router from "@/router";
import { Message, Loading } from "element-ui";
/******************* 日期时间相关 ******************/
/**
* 获取年
* @param num 0:当前年
* @returns 年
*/
function getYear(num: number = 0) {
const t = new Date(new Date().getTime());
const year: number = t.getFullYear() - num;
const newTime = "" + year;
return newTime;
}
/**
* 获取月
* @param num 0:当前月
* @returns 月
*/
function getMonth(num: number = 0) {
const t = new Date(new Date().getTime() - 30 * 24 * 60 * 60 * 1000 * num);
const month = t.getMonth() + 1;
const newTime = month < 10 ? "0" + month : month;
return newTime;
}
/**
* 获取日
* @param num 0:当前日
* @returns 日
*/
function getDate(num: number = 0) {
const t = new Date(new Date().getTime() - 24 * 60 * 60 * 1000 * num);
const day = t.getDate();
const newTime = day < 10 ? "0" + day : day;
return newTime;
}
/**
* 获取年月
* @param num 0:当前年月
* @param splitSymbol 年月之间分隔符号
* @returns 年月
*/
function getYM(num: number = 0, splitSymbol: string = "-") {
const t = new Date(new Date().getTime() - 30 * 24 * 60 * 60 * 1000 * num);
const year: number = t.getFullYear();
const month = t.getMonth() + 1;
const newTime = year + splitSymbol + (month < 10 ? "0" + month : month);
return newTime;
}
/**
* 获取年月日
* @param num 0:当前年月日
* @param splitSymbol 年月日之间分隔符号
* @returns 年月日
*/
function getYMD(num: number = 0, splitSymbol: string = "-") {
const t = new Date(new Date().getTime() - 24 * 60 * 60 * 1000 * num);
const year: number = t.getFullYear();
const month = t.getMonth() + 1;
const day = t.getDate();
const newTime =
year +
splitSymbol +
(month < 10 ? "0" + month : month) +
splitSymbol +
(day < 10 ? "0" + day : day);
return newTime;
}
/**
* 获取年月日 00:00:00
* @param num 0:当前年月日00:00:00
* @param splitSymbol 年月日之间分隔符号
* @param splitSymbol2 时分秒之间分隔符号
* @returns 年月日 00:00:00
*/
function getYMD0(
num: number = 0,
splitSymbol: string = "-",
splitSymbol2: string = ":"
) {
const t = new Date(new Date().getTime() - 24 * 60 * 60 * 1000 * num);
const year: number = t.getFullYear();
const month = t.getMonth() + 1;
const day = t.getDate();
const newTime =
year +
splitSymbol +
(month < 10 ? "0" + month : month) +
splitSymbol +
(day < 10 ? "0" + day : day) +
` 00${splitSymbol2}00${splitSymbol2}00`;
return newTime;
}
/**
* 获取年月日 时
* @param num 0:当前年月日时
* @param splitSymbol 年月日分隔符号
* @returns 年月日时
*/
function getYMDH(num: number = 0, splitSymbol: string = "-") {
const t = new Date(new Date().getTime() - 24 * 60 * 60 * 1000 * num);
const year: number = t.getFullYear();
const month = t.getMonth() + 1;
const day = t.getDate();
const hour = t.getHours();
const newTime =
year +
splitSymbol +
(month < 10 ? "0" + month : month) +
splitSymbol +
(day < 10 ? "0" + day : day) +
" " +
(hour < 10 ? "0" + hour : hour);
return newTime;
}
/**
* 获取年月日 时分
* @param num 0:当前年月日时分
* @param splitSymbol 年月日分隔符号
* @param splitSymbol2 时分分隔符号
* @returns 年月日时分
*/
function getYMDHm(
num: number = 0,
splitSymbol: string = "-",
splitSymbol2: string = ":"
) {
const t = new Date(new Date().getTime() - 24 * 60 * 60 * 1000 * num);
const year: number = t.getFullYear();
const month = t.getMonth() + 1;
const day = t.getDate();
const hour = t.getHours();
const min = t.getMinutes();
const newTime =
year +
splitSymbol +
(month < 10 ? "0" + month : month) +
splitSymbol +
(day < 10 ? "0" + day : day) +
" " +
(hour < 10 ? "0" + hour : hour) +
splitSymbol2 +
(min < 10 ? "0" + min : min);
return newTime;
}
/**
* 获取年月 时分秒
* @param num 0:当前年月日时分秒
* @param splitSymbol 年月日分隔符号
* @param splitSymbol2 时分秒分隔符号
* @returns 年月日时分秒
*/
function getYMDHms(
num: number = 0,
splitSymbol: string = "-",
splitSymbol2: string = ":"
) {
const t = new Date(new Date().getTime() - 24 * 60 * 60 * 1000 * num);
const year: number = t.getFullYear();
const month = t.getMonth() + 1;
const day = t.getDate();
const hour = t.getHours();
const min = t.getMinutes();
const sec = t.getSeconds();
const newTime =
year +
splitSymbol +
(month < 10 ? "0" + month : month) +
splitSymbol +
(day < 10 ? "0" + day : day) +
" " +
(hour < 10 ? "0" + hour : hour) +
splitSymbol2 +
(min < 10 ? "0" + min : min) +
splitSymbol2 +
(sec < 10 ? "0" + sec : sec);
return newTime;
}
/************************ localStorage ************************/
/**
* 设置localStorage
* @param key 键
* @param value 值
*/
function setStorage(key: string, value: string) {
try {
window.localStorage.setItem(key, value);
} catch (err) {
console.log("setStorage=》=》=》", err);
}
}
/**
* 获取localStorage
* @param key 键
*/
function getStorage(key: string) {
try {
const value = window.localStorage.getItem(key);
return value;
} catch (err) {
console.log("getStorage=》=》=》", err);
}
}
/**
* 删除localStorage
* @param key 键
*/
function removeStorage(key: string) {
try {
window.localStorage.removeItem(key);
} catch (err) {
console.log("removeStorage=》=》=》", err);
}
}
/************************ axios 相关 ************************/
// 创建自定义axios实例
let instance: any;
function initAxios() {
instance = axios.create({
// baseURL: 'http://localhost:8081:8081/api',
headers: {
"Content-Type": "application/json;charset=UTF-8",
// 'Content-Type': 'x-www-form-urlencoded',
"Access-Control-Allow-Origin": "*",
"Access-Control-Allow-Credentials": true,
},
// 设置超时时间
timeout: 30 * 1000,
// 携带凭证
// withCredentials: true,
// 返回数据类型
// responseType: "json",
});
// 登录页路由
const loginPath = "/login";
/**
* request 请求拦截器=>请求发出前处理
*/
instance.interceptors.request.use(
(config: any) => {
// if (config.url != '/api/sys/login') {
// 发送请求时携带token
const token = getStorage("token");
if (token) {
// 动态设置请求头
config.headers.token = token;
} else {
if (window.location.hash !== "#" + loginPath) {
// 重定向到登录页面
router.push(loginPath);
}
}
// }
// post和put请求时,序列化入参
if (config.method === "post" || config.method === "put") {
config.data = config.data ? JSON.stringify(config.data) : "";
}
return config;
},
(error: any) => {
// 接口返回失败
console.log("接口请求失败=》=》=》", error.response);
// 判断请求超时
if (
error.code === "ECONNABORTED" &&
error.message.indexOf("timeout") !== -1
) {
console.log("接口请求失败=》=》=》timeout请求超时");
}
Promise.reject(error.response);
}
);
/**
* request 响应拦截器=>处理响应数据
*/
instance.interceptors.response.use(
(response: any) => {
const code = response.data.code;
if (code === 401) {
// 401 token失效 返回登录页面
router.push(loginPath);
}
return Promise.resolve(response);
},
(error: any) => {
// //请求失败,这个地方可以根据error.response.status统一处理一些界面逻辑,比如status为401未登录,可以进行重定向
// router.replace({
// path: '/login',
// query: { redirect: router.currentRoute.fullPath }
// });
return Promise.reject(error);
}
);
}
initAxios();
/**
* get 异步请求
* @param uri api
* @param params 请求参数
* @param successCallback 成功回调函数
* @param isLoading 默认不显示loading
*/
function getRequest(
uri: string,
params: any,
successCallback?: any,
isLoading: boolean = false
) {
// 显示loading
let loading: any;
if (isLoading) {
loading = Loading.service({
lock: true,
text: "拼命加载中...",
// spinner: 'el-icon-loading',
background: "rgba(0, 0, 0, 0.2)",
});
}
instance({
url: uri,
method: "get",
params,
})
.then((ret: any) => {
if (isLoading) {
loading.close(); // 关闭loading
}
if (ret.data.code === 200 && ret.data.success === true) {
successCallback(ret.data);
} else {
// Message.error(ret.data.msg);
Message({
message: ret.data.msg,
type: "warning",
});
}
})
.catch((err: any) => {
if (isLoading) {
loading.close(); // 关闭loading
}
console.log("请求失败=》=》=》", err);
});
}
/**
* post 异步请求
* @param uri api
* @param params 请求参数
* @param successCallback 成功回调函数
* @param isLoading 默认不显示loading
*/
function postRequest(
uri: string,
params: any,
successCallback?: any,
isLoading: boolean = false
) {
// 显示loading
let loading: any;
if (isLoading) {
loading = Loading.service({
lock: true,
text: "拼命加载中...",
// spinner: 'el-icon-loading',
background: "rgba(0, 0, 0, 0.2)",
});
}
// 请求对象
let req;
if (params.params) {
// params类型
req = instance({
url: uri,
method: "post",
params: params.params,
});
} else if (params.data) {
// data类型
req = instance({
url: uri,
method: "post",
data: params.data,
});
} else {
// 默认params类型
req = instance({
url: uri,
method: "post",
data: params,
});
}
req.then((ret: any) => {
if (isLoading) {
loading.close(); // 关闭loading
}
if (ret.data.code === 200 && ret.data.success === true) {
successCallback(ret.data);
} else {
// errorCallback(ret.data);
// Message.error(ret.data.msg);
Message({
message: ret.data.msg,
type: "warning",
});
}
}).catch((err: any) => {
if (isLoading) {
loading.close(); // 关闭loading
}
console.log("post请求失败=》=》=》", err);
});
}
/**
* get 异步请求 响应数据不是正规格式的
* @param uri api
* @param params 请求参数
* @param successCallback 成功回调函数
* @param isLoading 默认不显示loading
*/
function getRequestOther(
uri: string,
params: any,
successCallback?: any,
isLoading: boolean = false
) {
// 显示loading
let loading: any;
if (isLoading) {
loading = Loading.service({
lock: true,
text: "拼命加载中...",
// spinner: 'el-icon-loading',
background: "rgba(0, 0, 0, 0.2)",
});
}
instance({
url: uri,
method: "get",
params,
})
.then((ret: any) => {
if (isLoading) {
loading.close(); // 关闭loading
}
successCallback(ret);
})
.catch((err: any) => {
if (isLoading) {
loading.close(); // 关闭loading
}
console.log("get other 请求失败=》=》=》", err);
});
}
/************************ 处理数据 ************************/
/**
* 数组对象中根据键去重
* @param arr 遍历的数组
* @param key 需要去重的键
* @returns 根据key去重后的值组成的数组
* arr=[{name:1,value:2},{name:3,value:4},{name:5,value:6},{name:1,value:2},{name:7,value:8},{name:1,value:2},{name:9,value:10}]
* key='value'
* 输出 [2, 4, 6, 8, 10]
*/
function arrRemoveRepeat(arr: any, key: string) {
const map = new Map();
for (const item of arr) {
if (!map.has(item[key])) {
map.set(item[key], item);
}
}
const newArr = [];
for (const iterator of [...map.values()]) {
newArr.push(iterator[key]);
}
return newArr;
}
/**
* json对象转url参数
* @param parame 对象
* @returns url参数字符串
*/
function objToUrlParams(parame: any) {
const params = Object.keys(parame)
.map((key) => {
return key + "=" + encodeURIComponent(parame[key]);
})
.join("&");
return "?" + params;
}
/**
* 导出 Excel
* @param url api
* @param params 参数对象 {a:1,b:2}
*/
function exportExcel(url: string, params: any) {
// 方式一 动态创建a标签
// const link = document.createElement("a");
// link.style.display = "none";
// link.href = that.exportObj.url;
// link.click();
// 方式二 iframe的方式
const elemIF = document.createElement("iframe");
elemIF.src = url + objToUrlParams(params);
elemIF.style.display = "none";
document.body.appendChild(elemIF);
}
/**
* 合法uri
* @param textval url
* @returns boolean
*/
function validateURL(textval: string) {
const urlregex = /^(https?|ftp):\/\/([a-zA-Z0-9.-]+(:[a-zA-Z0-9.&%$-]+)*@)*((25[0-5]|2[0-4][0-9]|1[0-9]{2}|[1-9][0-9]?)(\.(25[0-5]|2[0-4][0-9]|1[0-9]{2}|[1-9]?[0-9])){3}|([a-zA-Z0-9-]+\.)*[a-zA-Z0-9-]+\.(com|edu|gov|int|mil|net|org|biz|arpa|info|name|pro|aero|coop|museum|[a-zA-Z]{2}))(:[0-9]+)*(\/($|[a-zA-Z0-9.,?'\\+&%$#=~_-]+))*$/;
return urlregex.test(textval);
}
/**
* 合法邮箱
* @param email 邮箱
* @returns boolean
*/
function isEmail(email: string) {
return /^([a-zA-Z0-9_-])+@([a-zA-Z0-9_-])+((.[a-zA-Z0-9_-]{2,3}){1,2})$/.test(
email
);
}
/**
* 手机号码
* @param s 手机号码
* @returns boolean
*/
function isMobile(s: string) {
return /^1[0-9]{10}$/.test(s);
}
/**
* 电话号码
* @param s 电话号码
* @returns boolean
*/
function isPhone(s: string) {
return /^([0-9]{3,4}-)?[0-9]{7,8}$/.test(s);
}
/************************ 导出 ************************/
export default {
getYear,
getMonth,
getDate,
getYM,
getYMD,
getYMD0,
getYMDH,
getYMDHm,
getYMDHms,
setStorage,
getStorage,
removeStorage,
arrRemoveRepeat,
objToUrlParams,
getRequest,
postRequest,
getRequestOther,
exportExcel,
};
js常用工具类
最新推荐文章于 2024-04-07 03:10:51 发布
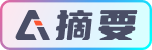