一、拦截器接口
package com.example.study.interceptor;
public abstract class Interceptor {
abstract void before(ActionInvocation invocation);
String intercept(ActionInvocation invocation) {
before(invocation);
String result = invocation.invoke();
after(invocation);
return result;
}
abstract void after(ActionInvocation invocation);
public interface Action {
String execute();
}
public interface ActionInvocation {
String invoke();
}
}
二、拦截器具体实现
package com.example.study.interceptor;
public class TestInterceptor extends Interceptor {
@Override
public void before(ActionInvocation invocation) {
System.out.println("test before ---> ");
}
@Override
public void after(ActionInvocation invocation) {
System.out.println("test after ---> ");
}
}
package com.example.study.interceptor;
public class InitInterceptor extends Interceptor {
@Override
public void before(ActionInvocation invocation) {
System.out.println("init before ---> ");
}
@Override
public void after(ActionInvocation invocation) {
System.out.println("init after ---> ");
}
}
package com.example.study.interceptor;
public class LoginInterceptor extends Interceptor {
@Override
public void before(ActionInvocation invocation) {
System.out.println("login before ---> ");
}
@Override
public void after(ActionInvocation invocation) {
System.out.println("login after ---> ");
}
}
三、调度器具体实现
package com.example.study.interceptor;
import java.util.ArrayList;
import java.util.List;
public class ActionInvoationImpl implements Interceptor.ActionInvocation {
private int index = 0;
private Interceptor.Action action;
private List<Interceptor> interceptors = new ArrayList<>();
public void setAction(Interceptor.Action action) {
this.action = action;
}
public void addInterceptor(Interceptor interceptor) {
interceptors.add(interceptor);
}
@Override
public String invoke() {
String result = "";
if (index == interceptors.size()) {
result = action.execute();
} else {
Interceptor interceptor = interceptors.get(index);
index++;
result = interceptor.intercept(this);
}
return result;
}
}
四、具体调用
package com.example.study;
import com.example.study.interceptor.ActionInvoationImpl;
import com.example.study.interceptor.InitInterceptor;
import com.example.study.interceptor.Interceptor;
import com.example.study.interceptor.LoginInterceptor;
import com.example.study.interceptor.TestInterceptor;
public class MyClass {
public static void main(String[] args) {
LoginInterceptor loginInterceptor = new LoginInterceptor();
TestInterceptor testInterceptor = new TestInterceptor();
InitInterceptor initInterceptor = new InitInterceptor();
ActionInvoationImpl impl = new ActionInvoationImpl();
impl.addInterceptor(loginInterceptor);
impl.addInterceptor(testInterceptor);
impl.addInterceptor(initInterceptor);
Interceptor.Action action = new Interceptor.Action() {
@Override
public String execute() {
System.out.println("action execute ...");
return "hello world";
}
};
impl.setAction(action);
String result = impl.invoke();
System.out.println("Action result ---> " + result);
}
}
五、结果查看
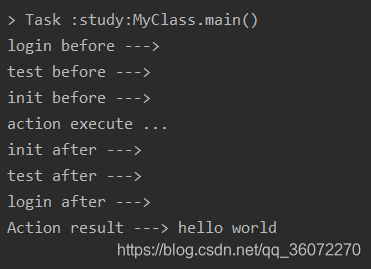