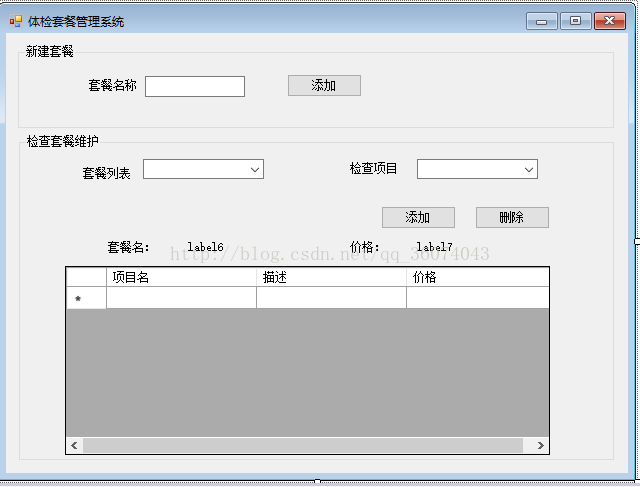
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 深入.Net_第五章_体检套餐
{
public partial class Form1 : Form
{
public List<HealthCheckItem> progrannerList = new List<HealthCheckItem>();
public Dictionary<string, HealthCheckSet> HealthSet = new Dictionary<string, HealthCheckSet>();
Dictionary<string, HealthCheckItem> AllItem = new Dictionary<string, HealthCheckItem>();
Dictionary<string, HealthCheckItem> items = new Dictionary<string, HealthCheckItem>();
//采用泛型集合保存 所有 的体检项目
List<HealthCheckItem> AllItems = new List<HealthCheckItem>();
//采用泛型集合保存 套餐 中的的体检项目
List<HealthCheckItem> items = new List<HealthCheckItem>();
//使用字典保存套餐集合
public Dictionary<string, HealthCheckSet> HealthSet = new Dictionary<string, HealthCheckSet>();
HealthCheckItem sg, ti, gan, B, shili;
HealthCheckSet set = new HealthCheckSet();
public Form1()
{
InitializeComponent();
}
public void BindGril(List<HealthCheckSet> list)
{
this.dataGridView1.DataSource = new BindingList<HealthCheckSet>(list);
}
//删除数据
private void button3_Click(object sender, EventArgs e)
{
string se = this.comboBox1.Text;
if (this.dataGridView1.SelectedRows.Count==0)
{
MessageBox.Show("没有选择删除项");
return;
}
//获取选中的索引
int index =this.dataGridView1.SelectedRows[0].Index;
this.HealthSet[se].items.RemoveAt(index);
//重新计算价格
this.HealthSet[se].CalPrice();
UpdateSet(HealthSet[se]);
this.lblSetName.Text=set.Name;
this.lblSetPrice.Text=set.Price.ToString();
MessageBox.Show("删除成功");
}
private void Form1_Load(object sender, EventArgs e)
{
this.lblSetName.Text = "";
this.lblSetPrice.Text = "";
Add();
Student();
}
public void Add() {
sg = new HealthCheckItem("身高", "用于检查身高", 5);
ti = new HealthCheckItem("体重", "用于检查体重", 5);
gan = new HealthCheckItem("肝功能", "用于检查肝功能", 50);
B = new HealthCheckItem("B超", "用于检查B超", 30);
shili = new HealthCheckItem("视力", "用于检查视力", 50);
AllItems.Add(sg);
AllItems.Add(ti);
AllItems.Add(gan);
AllItems.Add(B);
AllItems.Add(shili);
this.comboBox2.Items.Add("请选择");
foreach (HealthCheckItem item in AllItems) {
comboBox2.Items.Add(item.Name);
}
this.comboBox2.SelectedIndex = 0;
}
//入学体检套餐
public void Student() {
items = new List<HealthCheckItem>();
items.Add(sg);
items.Add(ti);
items.Add(gan);
set = new HealthCheckSet("入学体检", items);
set.CalPrice();
this.HealthSet.Add("入学体检", set);
this.comboBox1.Items.Clear();
this.comboBox1.Items.Add("请选择");
foreach (string key in HealthSet.Keys) {
this.comboBox1.Items.Add(key);
//this.lblSetName.Text = items[key].ToString() ;
}
//默认选择第一项
this.comboBox1.SelectedIndex = 0;
}
private void UpdateSet(HealthCheckSet set)
{
this.dataGridView1.DataSource = new BindingList<HealthCheckItem>(set.items);
}
private void comboBox1_SelectedIndexChanged(object sender, EventArgs e)
{
string setName = this.comboBox1.Text;
if (setName == "请选择") {
this.dataGridView1.DataSource = new BindingList<HealthCheckItem>();
lblSetName.Text = "";
lblSetPrice.Text = "";
return;
}
//设置套餐名
lblSetName.Text = this.HealthSet[setName].Name;
// 设置套餐价格
lblSetPrice.Text = this.HealthSet[setName].Price.ToString();
UpdateSet(HealthSet[setName]);
// this.button3.Enabled=true;
}
private void button2_Click(object sender, EventArgs e)
{
if (this.comboBox2.SelectedIndex == 0) {
MessageBox.Show("请选择一个项目");
return;
}
string SLL = comboBox1.Text;
if (SLL=="请选择")
{
MessageBox.Show("请选择套餐!");
return;
}
int index = this.comboBox2.SelectedIndex - 1;
if (!this.HealthSet[SLL].items.Contains(AllItems[index]))
{
this.HealthSet[SLL].items.Add(AllItems[index]);
this.HealthSet[SLL].CalPrice();
UpdateSet(this.HealthSet[SLL]);
this.lblSetName.Text = this.HealthSet[SLL].Name;
this.lblSetPrice.Text = this.HealthSet[SLL].Price.ToString();
MessageBox.Show("添加成功", "提示", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
else {
MessageBox.Show("该项目存在","提示",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
}
/// <summary>
/// 更新套餐,检查项目
/// </summary>
/// <param name="set"></param>
private void button1_Click(object sender, EventArgs e)
{
if (this.textBox1.Text == "")
{
MessageBox.Show("请输入要添加的套餐名称");
return;
}
HealthCheckSet se = new HealthCheckSet();
this.HealthSet.Add(this.textBox1.Text, se);
this.AddSet();
this.comboBox1.SelectedIndex = this.HealthSet.Count;
MessageBox.Show("添加成功");
}
public void AddSet()
{
this.comboBox1.Items.Clear();
this.comboBox1.Items.Add("请选择");
foreach (string item in HealthSet.Keys)
{
this.comboBox1.Items.Add(item);
}
this.comboBox1.SelectedIndex = 0;
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 深入.Net_第五章_体检套餐
{
public class HealthCheckItem
{
public string Name { get; set; }
public string Description { get; set; }
public int Price { get; set; }
public HealthCheckItem()
{
}
public HealthCheckItem(string name, string ds, int price)
{
this.Name = name;
this.Description = ds;
this.Price = price;
}
} }
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace 深入.Net_第五章_体检套餐
{
public class HealthCheckSet
{
public string Name { get; set; }
public int Price { get; set; }
public List<HealthCheckItem> items { get; set; }
public HealthCheckSet()
{
items = new List<HealthCheckItem>();
}
public HealthCheckSet(string name, List<HealthCheckItem> item)
{
this.Name = name;
this.items = item;
}
public void CalPrice()
{
int totalPrice = 0;
foreach(HealthCheckItem item in this.items)
{
totalPrice += item.Price;
}
this.Price = totalPrice;
}
}
}