一、命令模式
- 定义:
将“请求”封装成对象,以便使用不同的请求
- 补充解释:解决了应用程序中对象的职责以及他们之间的通信方式,使发送者和接收者完全解耦,
发送者和接收者之间没有没有直接关系,下命令的对象只知道如何发送请求,不知道如何完成请求
- 类型:行为型
- 适用场景:
- 请求调用者和请求接收者需要解耦,使得
调用者和接收者不直接交互
- 需要抽象出等待执行的行为
- 优点:
- 缺点:
- 相关设计模式
- 命令模式和
备忘录模式
:经常结合起来使用,可以用备忘录模式保存命令的历史记录,所以保存了历史记录,就能够调取上一个命令或上上个命令
二、Coding
public class Course {
private String name;
public Course(String name) {
this.name = name;
}
public void open() {
System.out.println(this.name + "课程开放");
}
public void close() {
System.out.println(this.name + "课程关闭");
}
}
public interface Command {
void execute();
}
public class CloseCourseCommand implements Command {
private Course course;
public CloseCourseCommand(Course course) {
this.course = course;
}
@Override
public void execute() {
course.close();
}
}
public class OpenCourseCommand implements Command {
private Course course;
public OpenCourseCommand(Course course) {
this.course = course;
}
@Override
public void execute() {
course.open();
}
}
public class Staff {
private List<Command> commandList = new ArrayList<Command>();
public void addCommand(Command command) {
commandList.add(command);
}
public void executeCommands() {
for (Command command : commandList) {
command.execute();
}
commandList.clear();
}
}
public class Test {
public static void main(String[] args) {
Course course = new Course("Java设计模式");
OpenCourseCommand openCourseCommand = new OpenCourseCommand(course);
CloseCourseCommand closeCourseCommand = new CloseCourseCommand(course);
Staff staff = new Staff();
staff.addCommand(openCourseCommand);
staff.addCommand(closeCourseCommand);
staff.executeCommands();
}
}
Java设计模式课程开放
Java设计模式课程关闭
- UML类图:
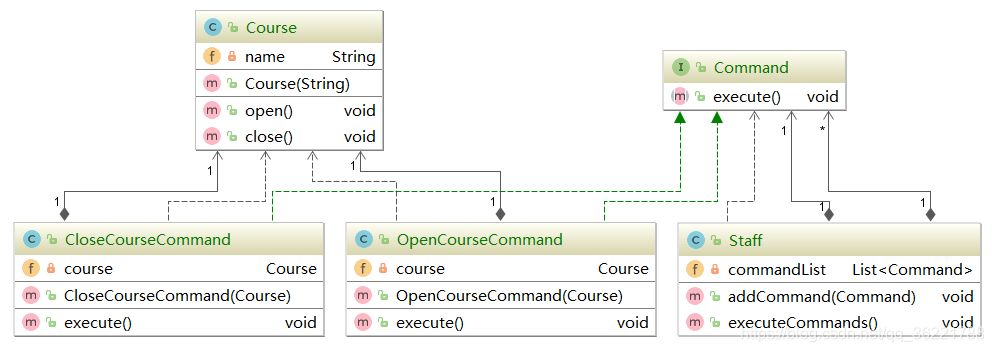
- 说明:首先打开和关闭命令实现了
Command
接口,而 Staff
里面可能有一个或多个命令且有顺序,两个命令和具体的课程 Course
是组合关系。
三、源码中的应用
- java.lang.Runnable:这个接口应该很熟悉了,实现这个接口创建线程,可以简单理解为Runnable是一个简单命令,如果线程实现了Runnable,里面的实现就是具体执行的命令。