public function upload(): Response
{
$path = '/upload/' . date('Ymd', time()) . '/';
$name = $_FILES['file']['name'];
$tmp_name = $_FILES['file']['tmp_name'];
$error = $_FILES['file']['error'];
//过滤错误
if ($error) {
switch ($error) {
case 1:
$error_message = '您上传的文件超过了PHP.INI配置文件中UPLOAD_MAX-FILESIZE的大小';
break;
case 2:
$error_message = '您上传的文件超过了PHP.INI配置文件中的post_max_size的大小';
break;
case 3:
$error_message = '文件只被部分上传';
break;
case 4:
$error_message = '文件不能为空';
break;
default:
$error_message = '未知错误';
}
die($error_message);
}
$arr_name = explode('.', $name);
$hz = array_pop($arr_name);
$new_name = md5(time() . uniqid()) . '.' . $hz;
if (!file_exists($_SERVER['DOCUMENT_ROOT'] . $path)) {
mkdir($_SERVER['DOCUMENT_ROOT'] . $path, 0755, true);
}
if (move_uploaded_file($tmp_name, $_SERVER['DOCUMENT_ROOT'] . $path . $new_name)) {
return $this->buildSuccess([
'fileName' => $new_name,
'fileUrl' => $this->request->domain() . $path . $new_name
]);
} else {
return $this->buildFailed(ReturnCode::FILE_SAVE_ERROR, '文件上传失败');
}
}
/**
* 附件上传接口
* @return Response
*
*/
public function upload_file(): Response
{
$accessKeyId = Config::get('apiadmin.aliyun_oss.accessKeyId');//去阿里云后台获取秘钥
$accessKeySecret = Config::get('apiadmin.aliyun_oss.accessKeySecret');//去阿里云后台获取秘钥
$endpoint = Config::get('apiadmin.aliyun_oss.endpoint');//你的阿里云OSS地址
$bucket = Config::get('apiadmin.aliyun_oss.bucket');
$ossClient = new OssClient($accessKeyId, $accessKeySecret, $endpoint);
$name = $_FILES['file']['name'];
$tmp_name = $_FILES['file']['tmp_name']; ///tmp/phprNH2wN
$error = $_FILES['file']['error'];
//过滤错误
if ($error) {
switch ($error) {
case 1:
$error_message = '您上传的文件超过了PHP.INI配置文件中UPLOAD_MAX-FILESIZE的大小';
break;
case 2:
$error_message = '您上传的文件超过了PHP.INI配置文件中的post_max_size的大小';
break;
case 3:
$error_message = '文件只被部分上传';
break;
case 4:
$error_message = '文件不能为空';
break;
default:
$error_message = '未知错误';
}
die($error_message);
}
$arr_name = explode('.', $name);
$hz = array_pop($arr_name);
$str = str_replace('.'.$hz,'',$name);
$new_name = date('Ymdhis') . rand(100, 999) .$str. '.' . $hz;
$result = $ossClient->uploadFile($bucket, $new_name, $tmp_name);
if ($result){
return $this->buildSuccess([
'fileName' => $new_name,
'fileUrl' => $result['info']['url']
]);
}else{
return $this->buildFailed(ReturnCode::FILE_SAVE_ERROR, '文件上传失败');
}
}
/**
* 附件上传接口
* @return Response
*
*/
public function upload_file_v2(): Response
{
$accessKeyId = Config::get('apiadmin.aliyun_oss.accessKeyId');//去阿里云后台获取秘钥
$accessKeySecret = Config::get('apiadmin.aliyun_oss.accessKeySecret');//去阿里云后台获取秘钥
$endpoint = Config::get('apiadmin.aliyun_oss.endpoint');//你的阿里云OSS地址
$bucket = Config::get('apiadmin.aliyun_oss.bucket');
$ossClient = new OssClient($accessKeyId, $accessKeySecret, $endpoint);
$name = $_FILES['file']['name'];
$tmp_name = $_FILES['file']['tmp_name']; ///tmp/phprNH2wN
$error = $_FILES['file']['error'];
dd($_FILES);
//过滤错误
if ($error) {
switch ($error) {
case 1:
$error_message = '您上传的文件超过了PHP.INI配置文件中UPLOAD_MAX-FILESIZE的大小';
break;
case 2:
$error_message = '您上传的文件超过了PHP.INI配置文件中的post_max_size的大小';
break;
case 3:
$error_message = '文件只被部分上传';
break;
case 4:
$error_message = '文件不能为空';
break;
default:
$error_message = '未知错误';
}
die($error_message);
}
$arr_name = explode('.', $name);
$hz = array_pop($arr_name);
$new_name = date('Ymdhis') . rand(100, 999) . $arr_name[0] . '.' . $hz;
$result = $ossClient->uploadFile($bucket, $new_name, $tmp_name);
if ($result){
return $this->buildSuccess([
'fileName' => $new_name,
'fileUrl' => $result['info']['url']
]);
}else{
return $this->buildFailed(ReturnCode::FILE_SAVE_ERROR, '文件上传失败');
}
}
/**
* 富文本批量上传 至OSS
* @return Response
*/
public function editor_upload(): Response
{
$accessKeyId = Config::get('apiadmin.aliyun_oss.accessKeyId');//去阿里云后台获取秘钥
$accessKeySecret = Config::get('apiadmin.aliyun_oss.accessKeySecret');//去阿里云后台获取秘钥
$endpoint = Config::get('apiadmin.aliyun_oss.endpoint');//你的阿里云OSS地址
$bucket = Config::get('apiadmin.aliyun_oss.bucket');
$ossClient = new OssClient($accessKeyId, $accessKeySecret, $endpoint);
//获取表单上传文件
$files = request()->file();
//组装文件保存目录
$path = '/editorupload';
//定义允许上传文件后缀的数组
$suffix_config = [
//允许图片上传的后缀
'image' => 'jpg,jpeg,png,gif',
//允许上传文件的后缀
'file' => 'zip,gz,doc,txt,pdf,xls',
//...
];
//定义允许上传文件大小的数组
$size_config = [
//允许图片上传的大小
'image' => 10,
//允许文件上传的大小
'file' => 50
];
$arr = [];
try {
foreach ($files as $file) {
$suffix = $suffix_config['image'];
$size = $size_config['image'];
//验证器验证上传的文件
validate(['file' => [
//限制文件大小
'fileSize' => $size * 1024 * 1024,
//限制文件后缀
'fileExt' => $suffix
]], [
'file.fileSize' => '上传的文件大小不能超过' . $size . 'M',
'file.fileExt' => '请上传后缀为:' . $suffix . '的文件'
])->check(['file' => $file]);
$new_name = date('Ymdhis').md5(time() . uniqid());
// $tmp_name = $file->getFilename();
$tmp_path = $file->getPathname(); //tmp/phplRqsWW
$result = $ossClient->uploadFile($bucket, $new_name, $tmp_path);
if (!empty($result)) {
$arr[] = array(
'url' => $result['info']['url'],
'alt' => "",
'href' => ""
);
} else {
throw new Exception('上传失败');
}
}
} catch (ValidateException $e) {
return json(['errno' => -1, 'data' => []]);
}
return json(['errno' => 0, 'data' => $arr]);
}
/**
* 富文本批量上传
* @return Response
*/
public function editor_upload_local(): Response
{
//获取表单上传文件
$files = request()->file();
//组装文件保存目录
$path = '/editorupload';
if (!file_exists($_SERVER['DOCUMENT_ROOT'] . '/storage'.$path)) {
mkdir($_SERVER['DOCUMENT_ROOT'] . '/storage'.$path, 0755, true);
}
//定义允许上传文件后缀的数组
$suffix_config = [
//允许图片上传的后缀
'image' => 'jpg,jpeg,png,gif',
//允许上传文件的后缀
'file' => 'zip,gz,doc,txt,pdf,xls',
//...
];
//定义允许上传文件大小的数组
$size_config = [
//允许图片上传的大小
'image' => 10,
//允许文件上传的大小
'file' => 50
];
$arr = [];
try {
foreach ($files as $file) {
//从config/upload.php配置文件中读取允许上传的文件后缀和大小
$suffix = $suffix_config['image'];
$size = $size_config['image'];
//验证器验证上传的文件
validate(['file' => [
//限制文件大小
'fileSize' => $size * 1024 * 1024,
//限制文件后缀
'fileExt' => $suffix
]], [
'file.fileSize' => '上传的文件大小不能超过' . $size . 'M',
'file.fileExt' => '请上传后缀为:' . $suffix . '的文件'
])->check(['file' => $file]);
//上传文件到本地服务器
$filename = \think\facade\Filesystem::disk('public')->putFile($path, $file);
if (!empty($filename)) {
$src = '/storage/'.$filename;
// $this->request->domain() . '/v1' .
$arr[] = array(
'url' => $this->request->domain() . '/v1' .$src,
'alt' => "",
'href' => ""
);
} else {
throw new Exception('上传失败');
}
}
} catch (ValidateException $e) {
return json(['errno' => -1, 'data' => []]);
}
return json(['errno' => 0, 'data' => $arr]);
}
TP6 上传文件
最新推荐文章于 2024-02-14 22:38:12 发布
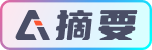