界面效果
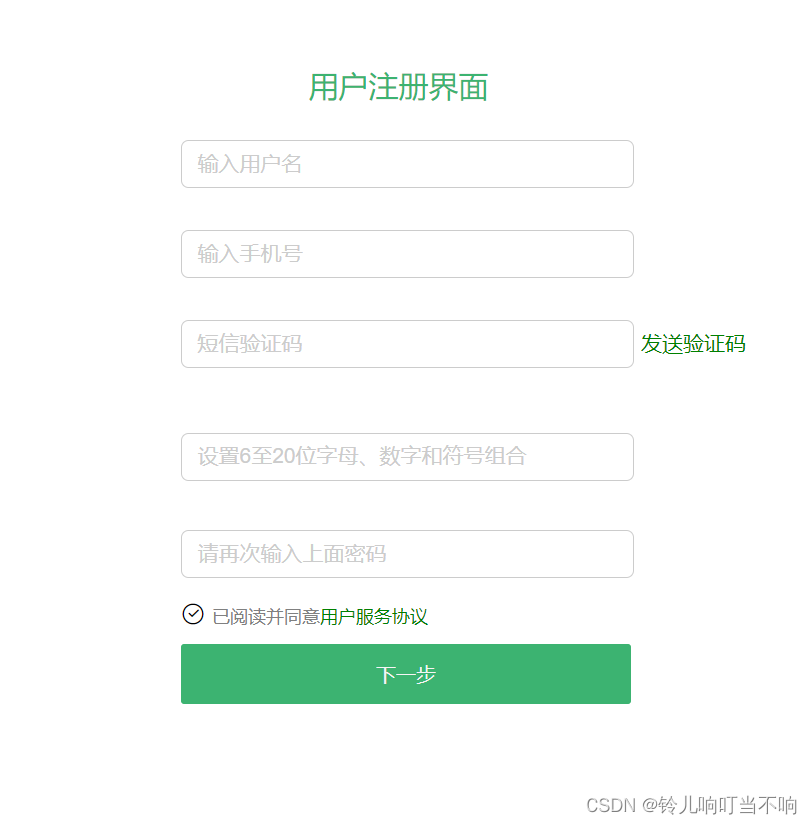
完整代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<link rel="stylesheet" href="http://at.alicdn.com/t/font_2143783_iq6z4ey5vu.css">
<style>
* {
margin: 0;
padding: 0;
}
body {
position: relative;
}
.title {
position: absolute;
top: 50px;
left: 585px;
font-size: 20px;
font-family: "微软雅黑";
color:#3cb371;
}
.register {
top: 100px;
position: absolute;
left: 500px;
}
.service {
color: green;
font-size: 11px;
}
.agree {
color: grey;
font-size: 11px;
}
.code {
color:green;
font-size: 14px;
}
/* 伪元素修改placeholder */
::-webkit-input-placeholder {
color: #ccc;
font-size: 14px;
/* 设置文本缩进 */
text-indent:10px;
}
input {
outline:none;
}
.item_one {
height: 60px;
}
.item_two {
height: 60px;
}
.item_three {
height: 60px;
}
.item_four {
height: 60px;
}
.item_five {
height: 60px;
}
input[name=username] {
height: 30px;
width: 300px;
border: 1px solid #ccc;
border-radius: 5px;
}
input[name=phone] {
height: 30px;
width: 300px;
border: 1px solid #ccc;
border-radius: 5px;
}
input[name=code] {
height: 30px;
width: 300px;
border: 1px solid #ccc;
border-radius: 5px;
}
input[name=password] {
height: 30px;
width: 300px;
border: 1px solid #ccc;
border-radius: 5px;
margin-top: 15px;
}
input[name=confirm] {
height: 30px;
width: 300px;
border: 1px solid #ccc;
border-radius: 5px;
margin-top: 20px;
}
.icon {
width: 300px;
margin-top: 5px;
}
span {
color:gray;
font-size: 14px;
margin-top: 20px;
}
.item_six {
margin-top: 10px;
}
button {
background-color: #3cb371;
border: none;
border-radius: 2px;
height: 40px;
width: 300px;
color: whitesmoke;
}
a {
text-decoration: none;
}
</style>
</head>
<body>
<div class="title">用户注册界面</div>
<form class="register">
<div class="item_one">
<input type="text" name="username" placeholder="输入用户名">
<span></span>
</div>
<div class="item_two">
<input type="text" name="phone" placeholder="输入手机号">
<span></span>
</div>
<div class="item_three">
<input type="text" name="code" placeholder="短信验证码">
<a href="#" class="code">发送验证码</a>
</div>
<div class="item_four">
<input type="password" name="password" placeholder="设置6至20位字母、数字和符号组合">
<span></span>
</div>
<div class="item_five">
<input type="password" name="confirm" placeholder="请再次输入上面密码">
<span></span>
</div>
<div class="icon">
<i class="iconfont icon-queren"></i> <span class="agree">已阅读并同意<span class="service">用户服务协议</span>
</div>
<div class="item_six">
<button type="submit">下一步</button>
</div>
</form>
<script>
// 发送验证码 用户点击之后,显示 30秒后重新获取
// 时间到了,自动改为重新获取
let code = document.querySelector('.code')
code.addEventListener('click',function(){
// 倒计时读秒操作
this.innerHTML = '30秒后重新获取'
let num = 30
let timer = setInterval(function(){
num--
num < 10 ? '0'+ num : num
code.innerHTML = `${num}秒重新获取`
if (num === 0){
code.innerHTML = `重新获取`
// 清除定时器
clearInterval(timer)
}
},1000)
})
// 用户名验证(注意封装函数 verify)
// 正则 /^[a-zA-Z0-9_]{6,10}$/
// 如果不符合要求,则出现提示信息,并return false 否则返回return true
// css属性选择器 [name=username]
let username = document.querySelector('input[name=username]')
username.addEventListener('change',verifyUser)
// 验证用户名的函数
function verifyUser(){
let span = username.nextElementSibling
// 开始验证 正则 1、定义规则 2、检测
let reg = /^([a-zA-Z0-9_]|[\u4e00-\u9fa5]){6,10}$/
if(!reg.test(username.value)){
// 验证错误
span.innerHTML = '请输入6~10个字符'
return false
}
// 合法
span.innerHTML = ''
return true
}
// 手机号验证
let phone = document.querySelector('input[name=phone]')
phone.addEventListener('change',verifyPhone)
// 验证用户名的函数
function verifyPhone(){
let span = phone.nextElementSibling
// 开始验证 正则 1、定义规则 2、检测
let reg = /^1(3[0-9]|5[0-3,5-9]|7[1-3,5-8]|8[0-9])\d{8}$/
if(!reg.test(phone.value)){
// 验证错误
span.innerHTML = '请输入11位的手机号码'
return false
}
// 合法
span.innerHTML = ''
return true
}
// 验证码验证
// 正则 /^\d{6}$/
let codeInput = document.querySelector('input[name=code]')
codeInput.addEventListener('change',verifyCode)
// 验证 验证码
function verifyCode(){
let span = codeInput.nextElementSibling
// 开始验证 正则 1、定义规则 2、检测
let reg = /^\d{6}$/
if(!reg.test(codeInput.value)){
// 验证错误
span.innerHTML = '请输入6位验证码'
return false
}
// 合法
span.innerHTML = ''
return true
}
// 密码验证
// 正则 /^[a-zA-Z0-9_]{6-20}$/
let password = document.querySelector('input[name=password]')
password.addEventListener('change',verifyPassWord)
// 验证 验证码
function verifyPassWord(){
let span = password.nextElementSibling
// 开始验证 正则 1、定义规则 2、检测
let reg = /^[a-zA-Z0-9_]{6,20}$/
if(!reg.test(password.value)){
// 验证错误
span.innerHTML = '请输入6~20位的密码'
return false
}
// 合法
span.innerHTML = ''
return true
}
// 再次密码验证
let confirm = document.querySelector('input[name=confirm]')
confirm.addEventListener('change',verifyConfirm)
function verifyConfirm(){
let span = confirm.nextElementSibling
if (confirm.value !== password.value ) {
span.innerHTML = '两次密码不一致'
return false
}
return true
}
// 切换类 同意模块
let icon = document.querySelector('.iconfont')
icon.addEventListener('click',function(){
icon.classList.toggle('icon-queren2')
})
// 需求:提交按钮.
// 提交之前先验证
let form = document.querySelector('form')
form.addEventListener('submit',function(e){
// 先阻止提交要先验证(阻止默认行为)
// 验证失败则阻止提交行为
// if( verifyUser() === false){
// e.preventDefault()
// }
if( !verifyUser()){
// 阻止提交
e.preventDefault()
}
if( !verifyPhone()){
// 阻止提交
e.preventDefault()
}
if( !verifyCode()){
// 阻止提交
e.preventDefault()
}
if( !verifyPassWord()){
// 阻止提交
e.preventDefault()
}
if( !verifyConfirm()){
// 阻止提交
e.preventDefault()
}
// 勾选模块
// classList.add() 添加
// classList.remove() 移除
// classList.toggle() 切换
// classList.contains() 检查是否包含 如果包含返回 true
if(!icon.classList.contains('icon-queren2')){
icon.
alert('请勾选同意协议')
e.preventDefault()
}
})
</script>
</body>
</html>