第十章 JavaScript操作BOM对象
BOM模型
浏览器对象模型 (Browser Object Model (BOM)) 使 JavaScript 有能力与浏览器对话
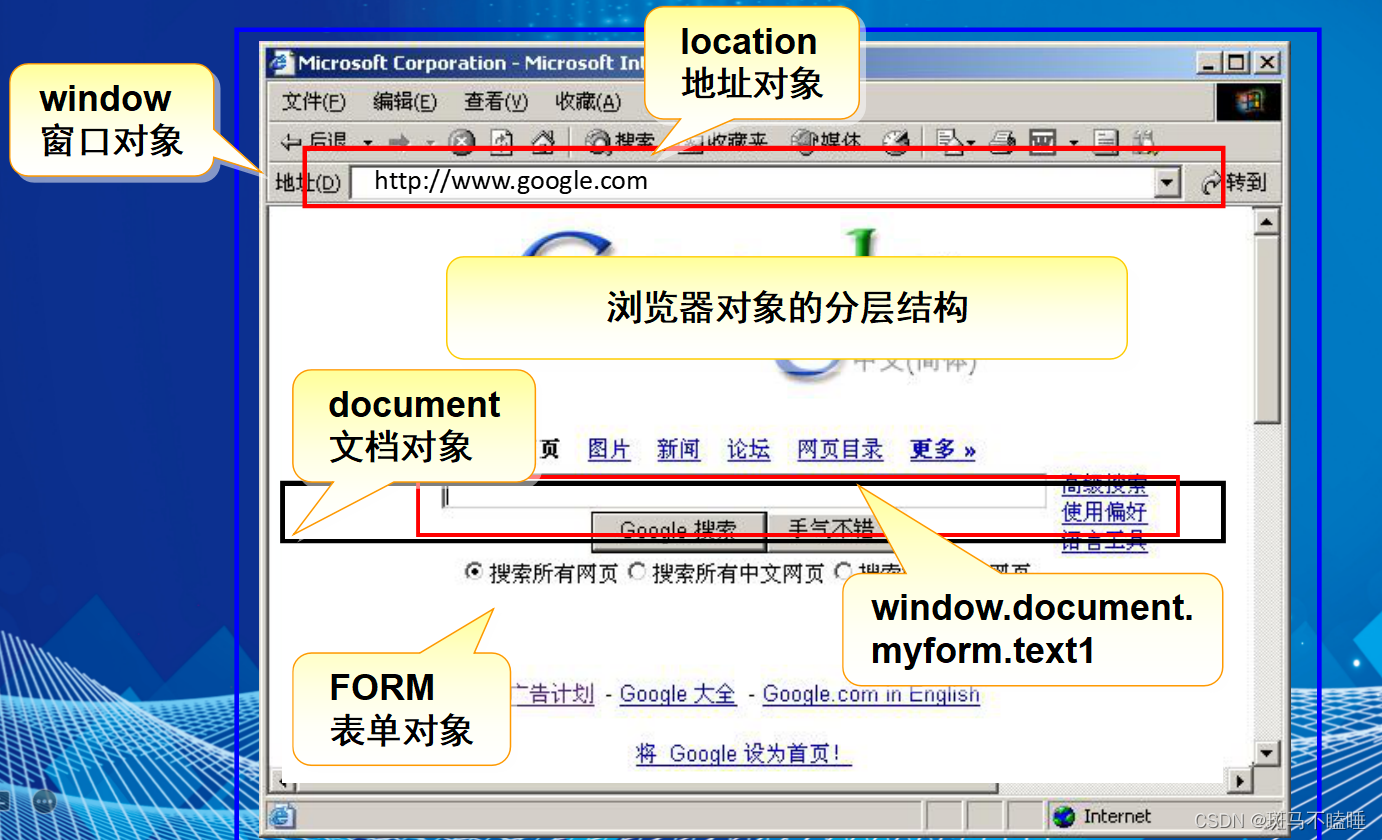
BOM提供了独立于内容的、可以与浏览器窗口进行互动的对象结构
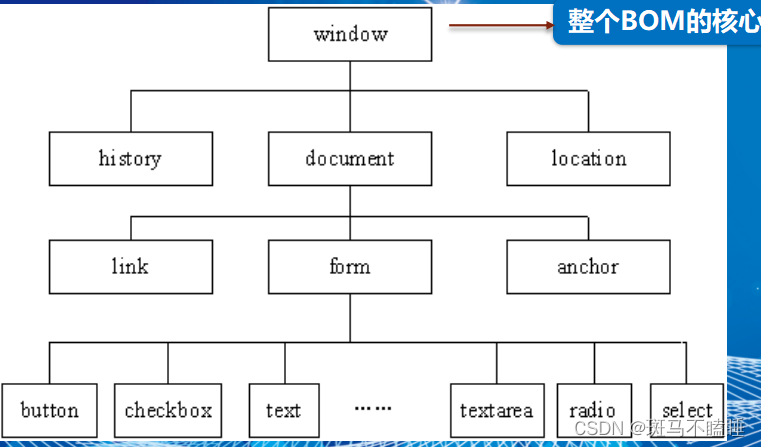
BOM可实现功能
弹出新的浏览器窗口
移动、关闭浏览器窗口以及调整窗口的大小
页面的前进、后退
window对象
常用属性
属性名称 | 说 明 |
---|
history | 有关客户访问过的URL的信息 |
location | 有关当前 URL 的信息 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>window</title>
<script>
function a(){
window.location="https://www.2345.com/tg36612.htm";
}
</script>
</head>
<body>
<button onclick="a()">2345</button>
</body>
</html>
常用的方法
方法名称 | 说 明 |
---|
prompt( ) | 显示可提示用户输入的对话框 |
alert( ) | 显示带有一个提示信息和一个确定按钮的警示框 |
confirm( ) | 显示一个带有提示信息、确定和取消按钮的对话框 |
close( ) | 关闭浏览器窗口 |
open( ) | 打开一个新的浏览器窗口,加载给定 URL 所指定的文档 |
setTimeout( ) | 在指定的毫秒数后调用函数或计算表达式 |
setInterval( ) | 按照指定的周期(以毫秒计)来调用函数或表达式 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>常用方法</title>
<script>
function a(){
var a=prompt("吃了么?","没有");
alert(a);
}
function b(){
var flag=confirm("是否删除?");
alert(flag)
}
function c(){
window.close();
}
function d(){
window.open("https://www.2345.com/tg36612.htm","新窗口",
"height=300px,width=300px");
}
function e(){
setTimeout("window.close()",5000);
}
function f(){
var now=new Date();
document.getElementById("h1").innerHTML=now;
}
var inter;
function g(){
inter=setInterval("f()",1000);
}
function h(){
clearInterval(inter);
}
</script>
</head>
<body onload="f()">
<button onclick="a()">**显示可提示用户输入的对话框**</button>
<button onclick="b()">删除</button>
<button onclick="c()">关闭浏览器</button>
<button onclick="d()">打开新窗口</button>
<button onclick="e()">5s后关闭窗口</button>
<button onclick="g()">开启时钟</button>
<button onclick="h()">关闭时钟</button>
<h1 id="h1"></h1>
</body>
</html>
open属性
属性名称 | 说 明 |
---|
height、width | 窗口文档显示区的高度、宽度。以像素计 |
left、top | 窗口的x坐标、y坐标。以像素计 |
toolbar=yes | no |1 | 0 | 是否显示浏览器的工具栏。黙认是yes |
scrollbars=yes | no |1 | 0 | 是否显示滚动条。黙认是yes |
location=yes | no |1 | 0 | 是否显示地址地段。黙认是yes |
status=yes | no |1 | 0 | 是否添加状态栏。黙认是yes |
menubar=yes | no |1 | 0 | 是否显示菜单栏。黙认是yes |
resizable=yes | no |1 | 0 | 窗口是否可调节尺寸。黙认是yes |
titlebar=yes | no |1 | 0 | 是否显示标题栏。黙认是yes |
fullscreen=yes | no |1 | 0 | 是否使用全屏模式显示浏览器。黙认是no。处于全屏模式的窗口必须同时处于剧院模式 |
history对象
名称 | 说 明 |
---|
back() | 加载 history 对象列表中的前一个URL |
forward() | 加载 history 对象列表中的下一个URL |
go() | 加载 history 对象列表中的某个具体URL |
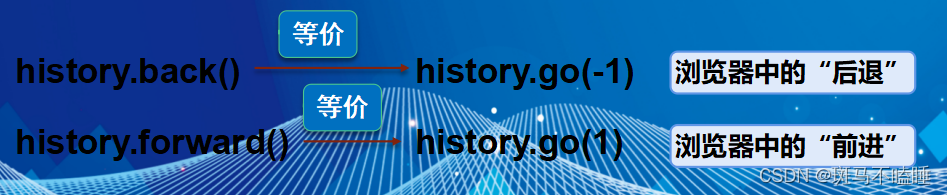
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script>
function a(){
history.forward();
}
function b(){
history.back();
}
</script>
</head>
<body>
<a href="c.html">进C</a>
<button onclick="a()">前进</button>
<button onclick="b()">后退</button>
</body>
</html>
location对象
名称 | 说 明 |
---|
host | 设置或返回主机名和当前URL的端口号 |
hostname | 设置或返回当前URL的主机名 |
href | 设置或返回完整的URL |
名称 | 说 明 |
---|
reload() | 重新加载当前文档 |
replace() | 用新的文档替换当前文档 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script>
function a(){
location.replace("b.html");
}
function b(){
location.reload();
}
function c(){
alert(location.host+"<br>"
+location.hostname+"<br>"
+location.href
);
}
</script>
</head>
<body>
<input type="text"/><br>
<button onclick="a()">替换</button>
<button onclick="b()">刷新</button>
<button onclick="c()">显示</button>
</body>
</html>
Document对象
名称 | 说 明 |
---|
referrer | 返回载入当前文档的URL |
URL | 返回当前文档的URL |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>document</title>
<script>
function a(){
alert(document.referrer);
alert(document.URL);
}
</script>
</head>
<body>
<button onclick="a()">显示</button>
</body>
</html>
Document对象的常用方法
名称 | 说 明 |
---|
getElementById() | 返回对拥有指定id的第一个对象的引用 |
getElementsByName() | 返回带有指定名称的对象的集合 |
getElementsByTagName() | 返回带有指定标签名的对象的集合 |
write() | 向文档写文本、HTML表达式或JavaScript代码 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script>
function a(){
var a= document.getElementById("h1");
a.innerHTML="<a href='#'>hello qiang!</a>"
}
function b(){
var strs=document.getElementsByName("h2");
for(i=0;i<strs.length;i++){
var a= strs[i].innerText;
alert(a);
}
}
function c(){
var h2=document.getElementsByTagName("h2");
h2[0].innerHTML="<span style='color:red'>你好 强!</span>";
h2[1].innerHTML="<span style='color:red'>你好 弱!</span>"
}
function d(){
var h2=document.getElementsByClassName("a");
h2[0].innerHTML="<span style='color:red'>你好 强!</span>";
h2[1].innerHTML="<span style='color:red'>你好 弱!</span>"
}
</script>
</head>
<body>
<h1 id="h1"></h1>
<h1 name="h2"><a href='#'>hello qiang!</a></h1>
<h1 name="h2">hello yuan</h1>
<h2 class="a"></h2>
<h2 class="a"></h2>
<button onclick="a()">按钮</button>
<button onclick="b()">按钮</button>
<button onclick="c()">按钮</button>
<button onclick="d()">按钮</button>
</body>
</html>
复选框的全选
checked属性值
选中:true
未选中:false
使用getElementsByName()方法访问同名复选框
将“全选”复选框的checked属性值赋值给每个复
选框的checked属性
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>复选</title>
<script>
function aa(){
var ck=document.getElementById("ck1");
var cks=document.getElementsByName("ck");
for(i=0;i<cks.length;i++){
cks[i].checked=ck.checked;
}
}
</script>
</head>
<body>
<form action="#" method="POST">
<table border="1">
<tr>
<td>
<input type="checkbox" name="a" onchange="aa()" id="ck1"/>
</td>
<td>id</td>
<td>name</td>
</tr>
<tr>
<td>
<input type="checkbox" name="ck"/>
</td>
<td>1</td>
<td>张三</td>
</tr>
<tr>
<td>
<input type="checkbox" name="ck"/>
</td>
<td>1</td>
<td>张三</td>
</tr>
<tr>
<td>
<input type="checkbox" name="ck"/>
</td>
<td>2</td>
<td>张三3</td>
</tr>
<tr>
<td>
<input type="checkbox" name="ck"/>
</td>
<td>3</td>
<td>张2三</td>
</tr>
</table>
</form>
</body>
</html>
JavaScript内置对象
Array:用于在单独的变量名中存储一系列的值
String:用于支持对字符串的处理
Math:用于执行常用的数学任务,它包含了若干个数字常量和函数
Date:用于操作日期和时间
Date
方法 | 说 明 |
---|
getDate() | 返回 Date 对象的一个月中的每一天,其值介于1~31之间 |
getDay() | 返回 Date 对象的星期中的每一天,其值介于0~6之间 |
getHours() | 返回 Date 对象的小时数,其值介于0~23之间 |
getMinutes() | 返回 Date 对象的分钟数,其值介于0~59之间 |
getSeconds() | 返回 Date 对象的秒数,其值介于0~59之间 |
getMonth() | 返回 Date 对象的月份,其值介于0~11之间 |
getFullYear() | 返回 Date 对象的年份,其值为4位数 |
getTime() | 返回自某一时刻(1970年1月1日)以来的毫秒数 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>日期</title>
<script>
var now=new Date();
document.write("<h1>"+now+"</h1>");
now.setHours(12);
now.setMinutes(30);
document.write("<h1>"+now+"</h1>");
document.write("<h1>"+now.getHours()+"</h1>");
document.write("<h1>"+now.getMinutes()+"</h1>");
document.write("<h1>"+now.getSeconds()+"</h1>");
</script>
</head>
<body>
</body>
</html>
Math对象
ceil() | 对数进行上舍入 | Math.ceil(25.5);返回26Math.ceil(-25.5);返回-25 |
---|
floor() | 对数进行下舍入 | Math.floor(25.5);返回25Math.floor(-25.5);返回-26 |
round() | 把数四舍五入为最接近的数 | Math.round(25.5);返回26Math.round(-25.5);返回-26 |
random() | 返回0~1之间的随机数 | Math.random();例如:0.6273608814137365 |
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<script>
var a=12.3;
var b=12.6;
var c=-12.3;
alert("上舍入:"+Math.ceil(c));
alert("下舍入:"+Math.floor(b));
alert("四舍五入:"+Math.round(b));
alert("随机数:"+Math.random())
</script>
</head>
<body>
</body>
</html>