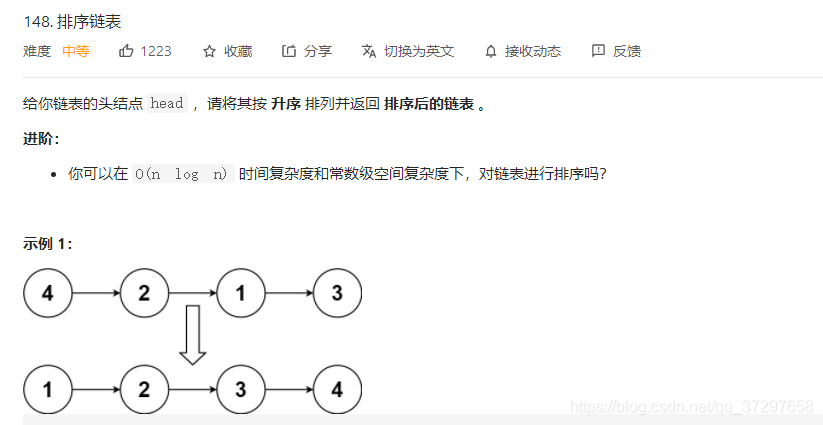
class Solution {
public:
static bool cmp(ListNode*l1,ListNode*l2){
return l1->val<l2->val;
}
ListNode* sortList(ListNode* head) {
if(!head)
return head;
vector<ListNode*>list;
ListNode*temp=head;
while(temp){
list.push_back(temp);
temp=temp->next;
}
sort(list.begin(),list.end(),cmp);
ListNode* dump=new ListNode(0);
ListNode* res=dump;
for(int i=0;i<list.size();i++){
dump->next=list[i];
dump=dump->next;
}
dump->next=nullptr;
return res->next;
}
};
class Solution {
public:
ListNode* sortList(ListNode* head) {
if (head == nullptr) return head;
vector<int> value;
ListNode* p = head;
while (p){
value.push_back(p->val);
p = p->next;
}
sort(value.begin(), value.end());
p = head;
int i = 0;
while (p){
p->val = value[i];
p = p->next;
i++;
}
return head;
}
};