原题题目
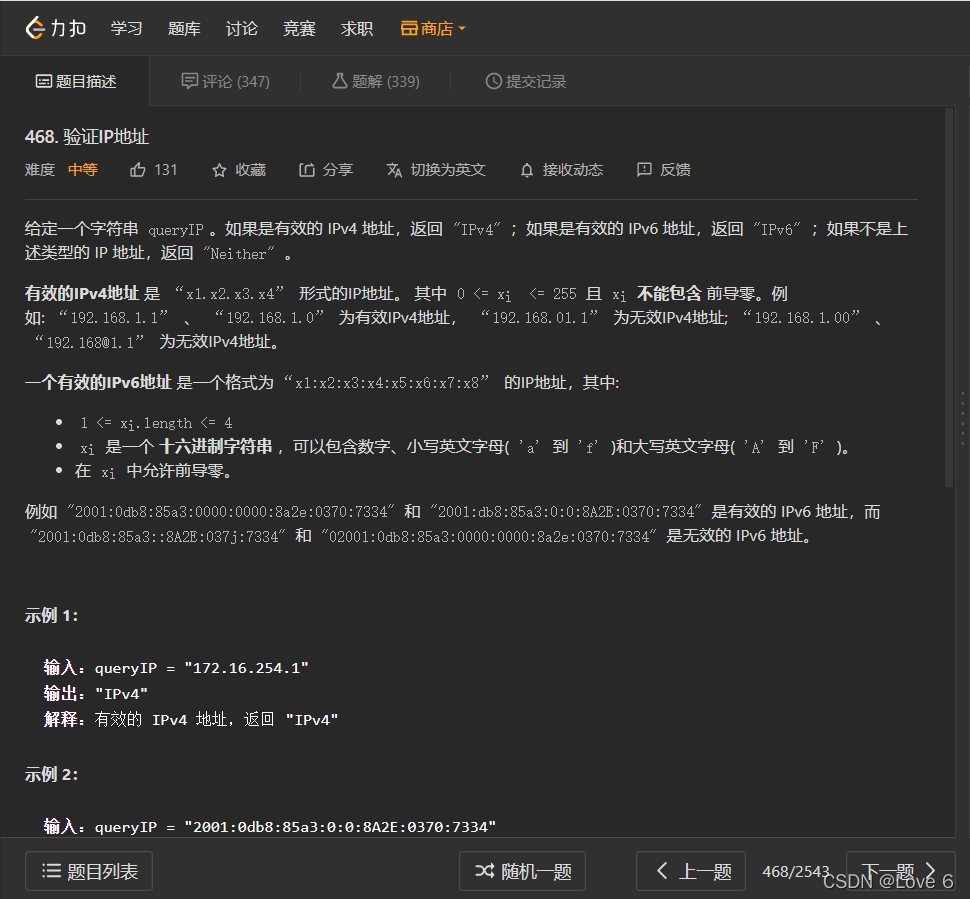
代码实现(首刷自解 面试遇到这种题 那就太逆天了)
class Solution {
public:
string judge_ip(const string& queryIP,int pos,int times,int ip_type)
{
if(pos == queryIP.size())
{
if(ip_type == 0 && times == 4) return "IPv4";
else if(ip_type == 1 && times == 8) return "IPv6";
else return "Neither";
return "";
}
if(!isalnum(queryIP[pos])) return "Neither";
string tmp;
int size = queryIP.size();
while(pos < size)
{
if(tmp.size() > 4) return "Neither";
if(!isalnum(queryIP[pos])) break;
if(queryIP[pos] > 'f' && queryIP[pos] < 'z' || queryIP[pos] > 'F' && queryIP[pos] < 'Z')
return "Neither";
if(!ip_type && !isdigit(queryIP[pos])) return "Neither";
tmp += queryIP[pos++];
}
if(queryIP[pos] != ':' && queryIP[pos] != '.')
return "Neither";
if(ip_type == 1 && queryIP[pos] != ':' || ip_type == 0 && queryIP[pos] != '.')
return "Neither";
if(ip_type == -1)
{
ip_type = (queryIP[pos] == '.' ? 0 : 1);
if(!ip_type)
{
for(const auto& chr:tmp)
{
if(!isdigit(chr))
return "Neither";
}
}
}
if(!ip_type)
{
auto num = stoi(tmp);
if(!tmp.size() || num < 0 || num > 255 || tmp[0] == '0' && tmp.size() > 1)
return "Neither";
}
else
{
if(tmp.size() < 1 || tmp.size() > 4) return "Neither";
}
return judge_ip(queryIP,pos + 1,times + 1,ip_type);
}
string validIPAddress(string queryIP) {
char add_chr = 0;
for(const auto& chr:queryIP)
{
if(chr == '.' || chr == ':')
{
add_chr = chr;
break;
}
}
queryIP += add_chr;
return judge_ip(queryIP,0,0,-1);
}
};
代码实现(二刷自解 DAY 12 golang)
func judgeIPv4(queryIP string) string {
items := strings.Split(queryIP, ".")
if len(items) != 4 {
return "Neither"
}
for _, ip := range items {
intip, err := strconv.Atoi(ip)
if err != nil {
return "Neither"
}
if intip < 0 || intip > 255 || intip == 0 && len(ip) > 1 || intip != 0 && ip[0] == '0' {
return "Neither"
}
}
return "IPv4"
}
func judgeIPv6(queryIP string) string {
items := strings.Split(queryIP, ":")
if len(items) != 8 {
return "Neither"
}
for _, ip := range items {
if iplen := len(ip); iplen <= 0 || iplen > 4 {
return "Neither"
}
for _, chr := range ip {
if !(chr >= 'a' && chr <= 'f') && !(chr >= 'A' && chr <= 'F') && !(chr >= '0' && chr <= '9') {
return "Neither"
}
}
}
return "IPv6"
}
func validIPAddress(queryIP string) string {
ret := "Neither"
if strings.Contains(queryIP, ".") {
ret = judgeIPv4(queryIP)
}
if strings.Contains(queryIP, ":") {
ret = judgeIPv6(queryIP)
}
return ret
}